1 User Management
1.1 User List Display
1.1.1 Page JS Analysis
- Life cycle functions
//Data Query Using Hook Function
mounted(){
this.getUserList()
}
- Get data function analysis
async getUserList(){
const {data: result} = await this.$http.get('/user/list',{
params: this.queryInfo
})
if(result.status !== 200) return this.$message.error("User list query failed")
this.userList = result.data.rows
this.total = result.data.total
console.log("Total Records:"+this.total)
},
1.1.2 Business Interface Description
- Request path: /user/list
- Request type: GET
- Request parameters: Receive in the background using a PageResult object
- Request case: http://localhost:8091/user/list?query= Query keyword &pageNum=1&pageSize=10
Parameter Name | Parameter Description | Remarks |
---|
query | Data queried by the user | Can be null |
pageNum | Page Number of Paging Queries | Must assign cannot be null |
pageSize | Number of Paging Queries | Must assign cannot be null |
- Response parameter: SysResult object needs to carry paging object PageResult
Parameter Name | Parameter Description | Remarks |
---|
status | status information | 200 indicates successful server request 201 indicates server exception |
msg | Prompt information returned by the server | Can be null |
data | Business data returned by the server | Return value PageResult object |
- Introduction to PageResult object
Parameter Name | Parameter type | Parameter Description | Remarks |
---|
query | String | Data queried by the user | Can be null |
pageNum | Integer | Query Pages | Cannot be null |
pageSize | Integer | Number of queries | Cannot be null |
total | Long | Total number of records queried | Cannot be null |
rows | Object | Results of Paging Query | Cannot be null |
{"status":200,
"msg":"Server call succeeded!",
"data":
{"query":"",
"pageNum":1,
"pageSize":2,
"total":4,
"rows":[
{"created":"2021-02-18T11:17:23.000+00:00",
"updated":"2021-03-26T06:47:20.000+00:00",
"id":1,
"username":"admin",
"password":"a66abb5684c45962d887564f08346e8d",
"phone":"13111112222",
"email":"1235678@qq.com",
"status":true,
"role":null
},
{"created":"2021-02-18T11:17:23.000+00:00",
"updated":"2021-03-13T08:50:30.000+00:00",
"id":2,
"username":"admin123",
"password":"a66abb5684c45962d887564f08346e8d",
"phone":"13111112223",
"email":"1235678@qq.com",
"status":false,
"role":null
}
]
}
}
1.1.3 Encapsulates PageResult objects
@Data
@Accessors(chain = true)
@NoArgsConstructor
@AllArgsConstructor
public class PageResult { //Encapsulate VO Objects
private String query;
private Integer pageNum;
private Integer pageSize;
private Long total;
private Object rows;
}
1.1.4 Edit UserController
/**
* Business description:
* 1. /user/list
* 2.Request type: GET
* 3.Parameter Receive: Background Receive with PageResult Object
* 3.Return value: SysResult<PageResult>
*/
@GetMapping("/list")
public SysResult getUserList(PageResult pageResult){//Parameter 3
//Total number of business queries. Number of page breaks.
pageResult = userService.getUserList(pageResult);
return SysResult.success(pageResult);//Parameter 5
}
1.1.5 Edit UserService
/**
* Require Query 1 Page 10 Items
* Features: Array Result Tip: Head without Tail
* Syntax: select * from user limit starting position, number of queries
* Page 1: select * from user limit 0,10-9
* Page 2: select * from user limit 10,10-19
* Page 3: select * from user limit 20,10 20-29
* Page N: select * from user limit (n-1)*10,10
* @param pageResult
* @return
*/
@Override
public PageResult getUserList(PageResult pageResult) {
//1. total number of records
long total = userMapper.getTotal();
//2. Paged data
int size = pageResult.getPageSize();
int start = (pageResult.getPageNum() - 1) * size;
List<User> rows = userMapper.findUserListByPage(start,size);
return pageResult.setTotal(total).setRows(rows);
}
1.1.6 Edit UserMapper interface
@Select("select * from user limit #{start},#{size}")
List<User> findUserListByPage(@Param("start") int start,@Param("size") int size);
1.1.7 Page Effect Display
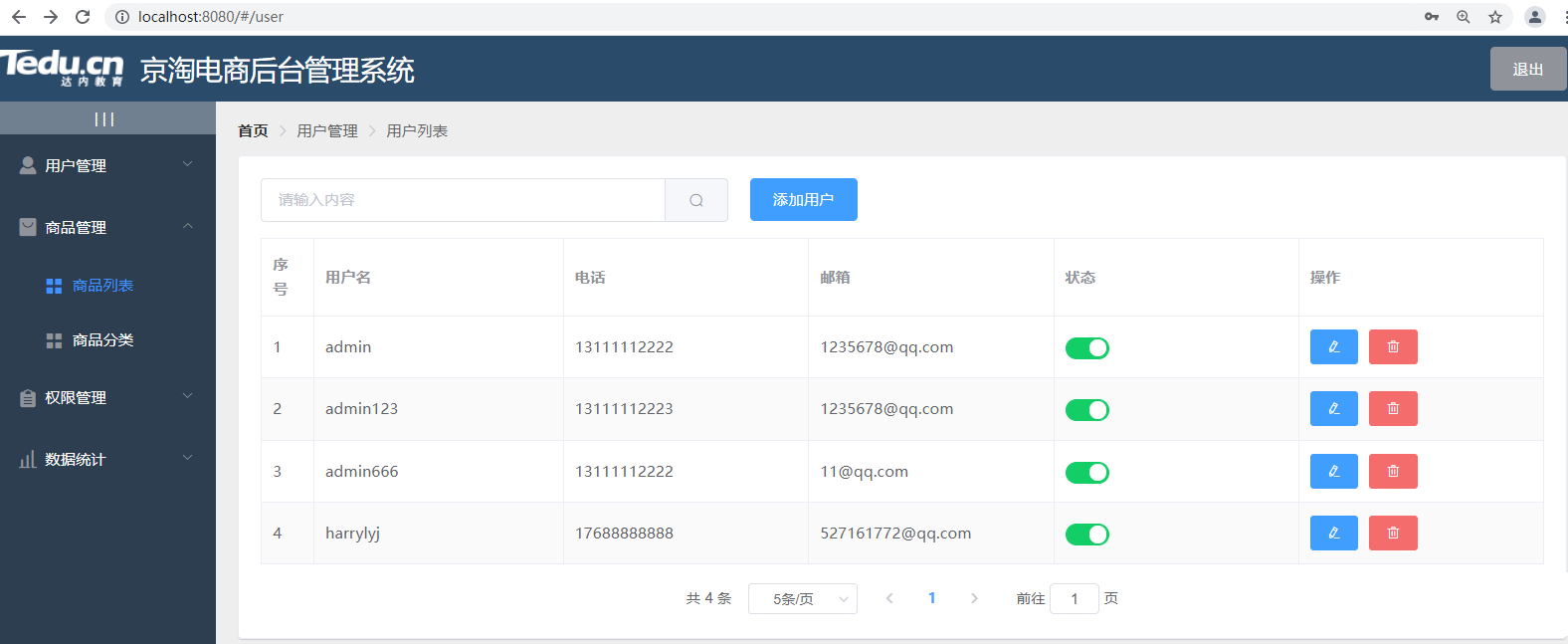
2 Use subqueries to implement left menu list
2.1 Edit Mapping File
2.1.1 Subquery Sql Statement Writing
/*Query Level 1 Menu Information*/
SELECT * FROM rights WHERE parent_id = 0
/* Query Slave Table Data */
SELECT * FROM rights WHERE parent_id = 3
2.1.2 xml Mapping File Writing
<!--Data acquisition using subqueries
1.Query main table information
-->
<select id="getRightsList" resultMap="rightsRM">
select * from rights where parent_id = 0
</select>
<resultMap id="rightsRM" type="Rights" autoMapping="true">
<!--Primary Key Information-->
<id property="id" column="id"></id>
<collection property="children" ofType="Rights"
select="findChildren" column="id"/>
</resultMap>
<select id="findChildren" resultType="Rights">
select * from rights where parent_id = #{id}
</select>
3 User Module Management
3.1 Paging Query Supplement
3.1.1 User Requirements Description
Note: The user's text input box may or may not have data. The data should be queried using dynamic Sql in the back-end server.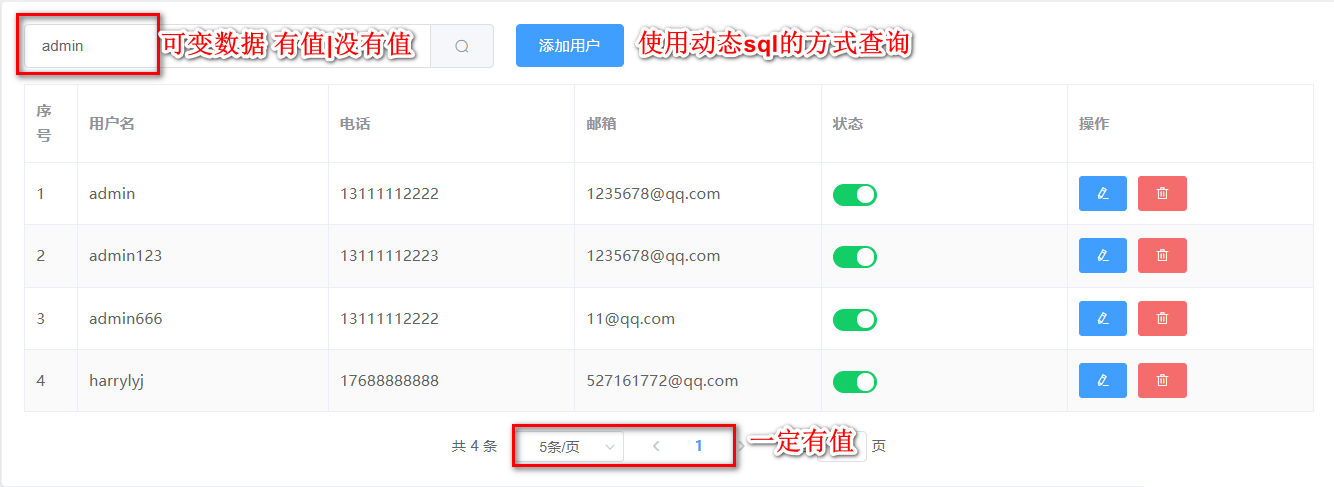
3.1.2 Edit UserController
/**
* Business description:
* 1. /user/list
* 2.Request type: GET
* 3.Parameter Receive: Background Receive with PageResult Object
* 3.Return value: SysResult<PageResult>
*/
@GetMapping("/list")
public SysResult getUserList(PageResult pageResult){//Parameter 3
//Total number of business queries. Number of page breaks.
pageResult = userService.getUserList(pageResult);
return SysResult.success(pageResult);//Parameter 5
}
3.1.3 Edit UserService
@Override
public PageResult getUserList(PageResult pageResult) {
//1. total number of records
long total = userMapper.getTotal();
//2. Paged data
//2.1 Get the number of bars per page
int size = pageResult.getPageSize();
//2.2 Get Start Location
int start = (pageResult.getPageNum() - 1) * size;
//2.3 Get data from user queries
String query = pageResult.getQuery();
List<User> rows = userMapper.findUserListByPage(start,size,query);
return pageResult.setTotal(total).setRows(rows);
}
3.1.4 Edit UserMapper
- Edit Mapper Interface
List<User> findUserListByPage(@Param("start") int start,
@Param("size") int size,
@Param("query") String query);
- Edit UserMapper.xml mapping file
<mapper namespace="com.jt.mapper.UserMapper">
<!--
resultType: Suitable for form queries
resultMap: 1.Multi-table Association Query 2.Use when field names and attributes are inconsistent
if Judgment Conditions
test="query !=null and query !='' Indicates that the condition is valid when the condition is not satisfied at the same time
-->
<select id="findUserListByPage" resultType="User">
select * from user
<where>
<if test="query !=null and query !='' ">username like "%"#{query}"%"</if>
</where>
limit #{start},#{size}
</select>
</mapper>
3.2 Completion status modification
3.2.1 Business Description
Description: status=true/false database display 1/0 1/0 and true/false object mapping in switch control database can be converted to each other.
Modify the implementation status based on the user's ID.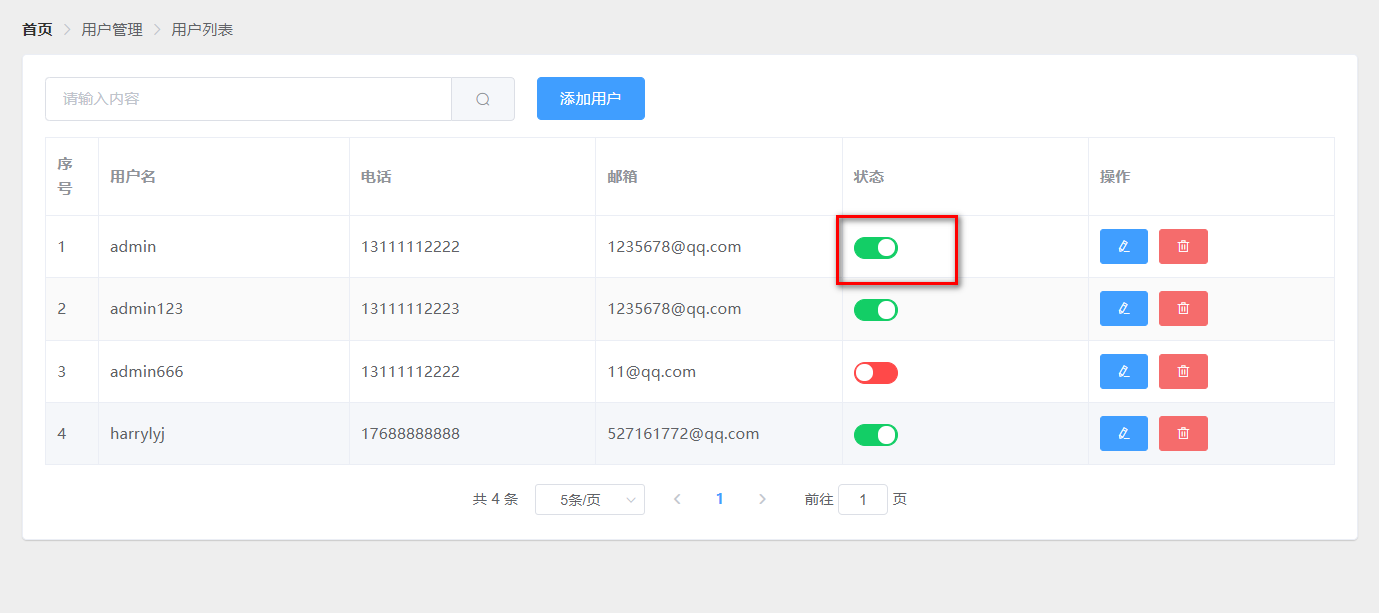
3.2.2 Front End JS Analysis
-
Knowledge Points
Scope slot: Generally, the current row object can be dynamically obtained when the tabular data is presented.
Usage:
1.template
2.slot-scope property="variable"
-
Page JS Analysis
<el-table-column prop="status" label="state">
<!-- <template slot-scope="scope">
{{scope.row.status}}
</template> -->
<template slot-scope="scope">
<el-switch v-model="scope.row.status" @change="updateStatus(scope.row)"
active-color="#13ce66" inactive-color="#ff4949">
</el-switch>
</template>
</el-table-column>
- Page Function Description
async updateStatus(user){
//Implementing user state modification Note the new usage proposed in template string ES6 ${key}
//const {data: result} = await this.$http.put('/user/status/'+user.id+'/'+user.status)
const {data: result} = await this.$http.put(`/user/status/${user.id}/${user.status}`)
if(result.status !== 200) return this.$message.error("User state modification failed!")
this.$message.success("User Status Modified Successfully!")
},
3.2.3 Business Interface Document Description
- Request path/user/status/{id}/{status}
- Request Type PUT
- Request Parameters: User ID/Status Value Data
Parameter Name | Parameter type | Parameter Description | Remarks Information |
---|
id | Integer | User ID Number | Cannot be null |
status | boolean | Parameter State Information | Cannot be null |
- Return value result: SysResult object
{"status":200,"msg":"Server call succeeded!","data":null}
3.2.4 Edit UserController
/**
* Business: Implement user state modifications
* Parameters: /user/status/{id}/{status}
* Return value: SysResult object
* Type: put type
*/
@PutMapping("/status/{id}/{status}")
public SysResult updateStatus(User user){
userService.updateStatus(user);
return SysResult.success();
}
3.2.5 Edit UserService
//Modify status/updated update time during update operation
@Override
public void updateStatus(User user) {
user.setUpdated(new Date());
userMapper.updateStatus(user);
}
3.2.6 Edit UserMapper
@Update("update user set status = #{status},updated = #{updated} where id=#{id}")
void updateStatus(User user);
3.3 New User Operations
3.3.1 Page JS Analysis
-
Edit new pages
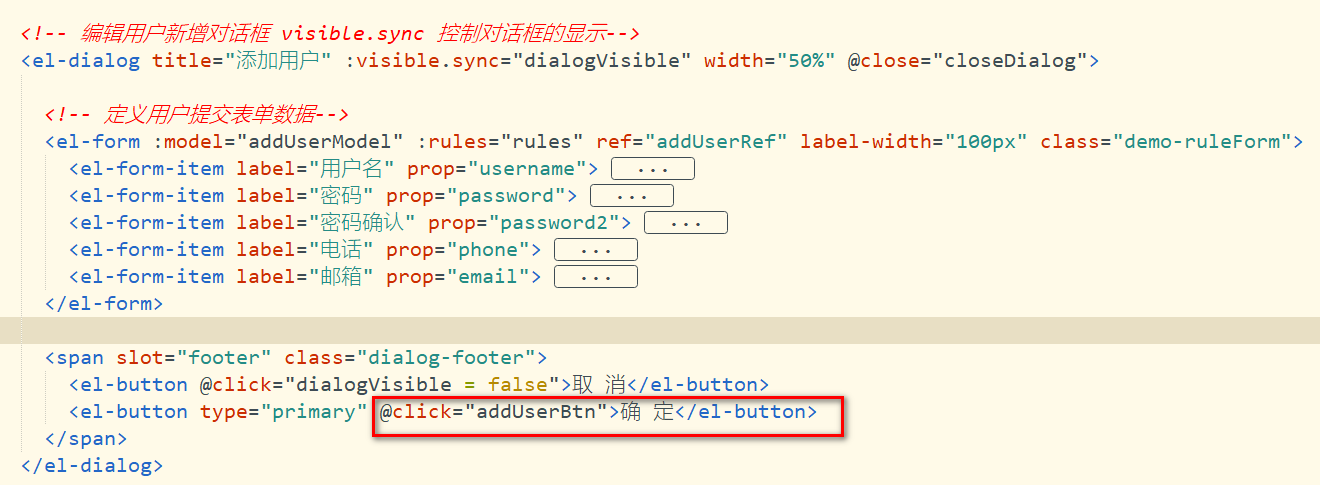
-
JS Analysis for New Pages
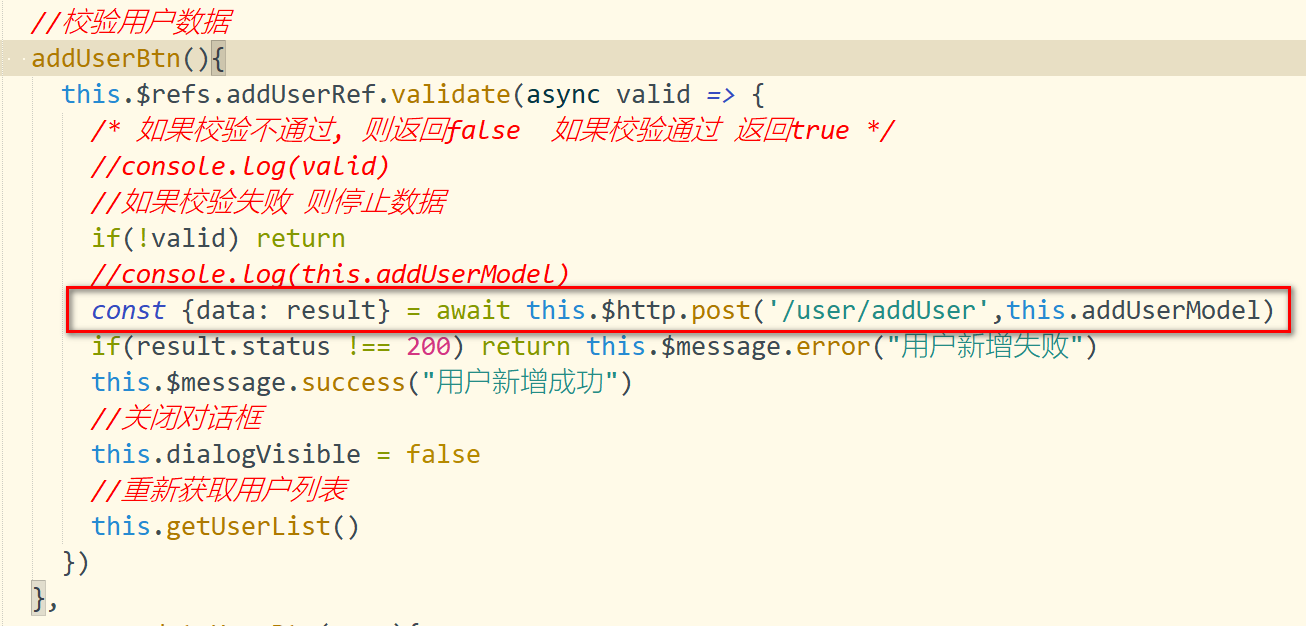
3.3.2 New Business Interface Description
- Request Path/user/addUser
- Request Type POST
- Request parameters: The entire form data is encapsulated as a js object for parameter delivery
Parameter Name | Parameter type | Parameter Description | Remarks |
---|
username | String | User name | Cannot be null |
password | String | Password | Cannot be null |
phone | String | Phone number | Cannot be null |
email | String | Password | Cannot be null |
- Return value result: SysResult object
{"status":200,"msg":"Server call succeeded!","data":null}
3.3.3 Edit UserController
/**
* Business: Implement new user actions
* url: /user/addUser post type
* Parameter: Receive using User object
* Return value: SysResult object
*/
@PostMapping("/addUser")
public SysResult addUser(@RequestBody User user){
userService.addUser(user);
return SysResult.success();
}
3.3.4 Edit UserService
/**
* 1.Password Encryption
* 2.Add status code information
* 3.Add Creation/Modification Time
* 4.How to complete the repository operation xml
* @param user
*/
@Override
public void addUser(User user) {
//1. Password Encryption Processing
Date date = new Date();
String md5Pass = DigestUtils.md5DigestAsHex(user.getPassword().getBytes());
user.setPassword(md5Pass)
.setStatus(true)
.setCreated(date)
.setUpdated(date); //Time uniqueness is best guaranteed.
userMapper.addUser(user);
}
3.3.5 Edit UserMapper/xml mapping file
- Edit mapper interface
void addUser(User user);
- Edit xml Mapping File
<!--Complete user additions-->
<insert id="addUser">
insert into user(id,username,password,phone,email,status,created,updated)
value
(null,#{username},#{password},#{phone},#{email},#{status},#{created},#{updated})
</insert>
3.4 Modify operation data echo
3.4.1 Page JS Analysis
- Button Click Event
<el-button type="primary" icon="el-icon-edit" size="small" @click="updateUserBtn(scope.row)"></el-button>
- Data Echo JS
async updateUserBtn(user){
this.updateDialogVisible = true
const {data: result} = await this.$http.get("/user/"+user.id)
if(result.status !== 200) return this.$message.error("User Query Failed")
this.updateUserModel = result.data
},
3.4.2 Page Interface Documentation
- Request path: /user/{id}
- Request type: GET
- Return value: SysResult object
Parameter Name | Parameter Description | Remarks |
---|
status | status information | 200 indicates successful server request 201 indicates server exception |
msg | Prompt information returned by the server | Can be null |
data | Business data returned by the server | Return user object |
- The JSON format is as follows:
{
"status":200,
"msg":"Server call succeeded!",
"data":{
"created":"2021-02-18T11:17:23.000+00:00",
"updated":"2021-05-17T11:33:46.000+00:00",
"id":1,
"username":"admin",
"password":"a66abb5684c45962d887564f08346e8d",
"phone":"13111112222",
"email":"1235678@qq.com",
"status":true,
"role":null
}
}
3.4.3 Edit UserController
/**
* Query database based on ID
* URL:/user/{id}
* Parameter: id
* Return value: SysResult(user object)
*/
@GetMapping("/{id}")
public SysResult findUserById(@PathVariable Integer id){
User user = userService.findUserById(id);
return SysResult.success(user);
}
3.4.4 Edit UserService
@Override
public User findUserById(Integer id) {
return userMapper.findUserById(id);
}
3.4.5 Edit UserMapper
//Principle: mybatis has an arbitrary name when it takes a value for a single value transfer (basic types such as int/string)
// The underlying data obtained by the subscript [0] is not related to the name.
@Select("select * from user where id=#{id}")
User findUserById(Integer id);
3.4.6 Page Effect Display
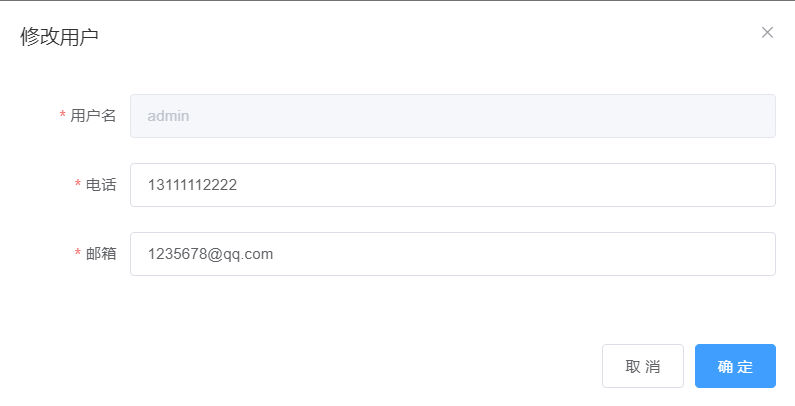
3.5 Implement user updates
3.5.1 Page JS Analysis
-
Page JS
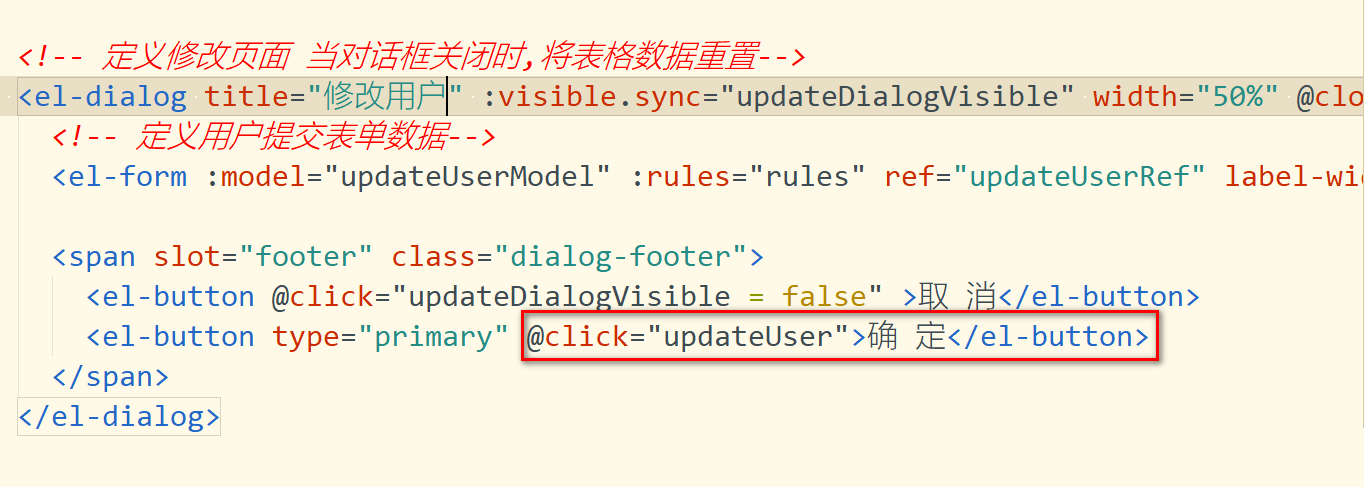
-
Initiate Ajax Request
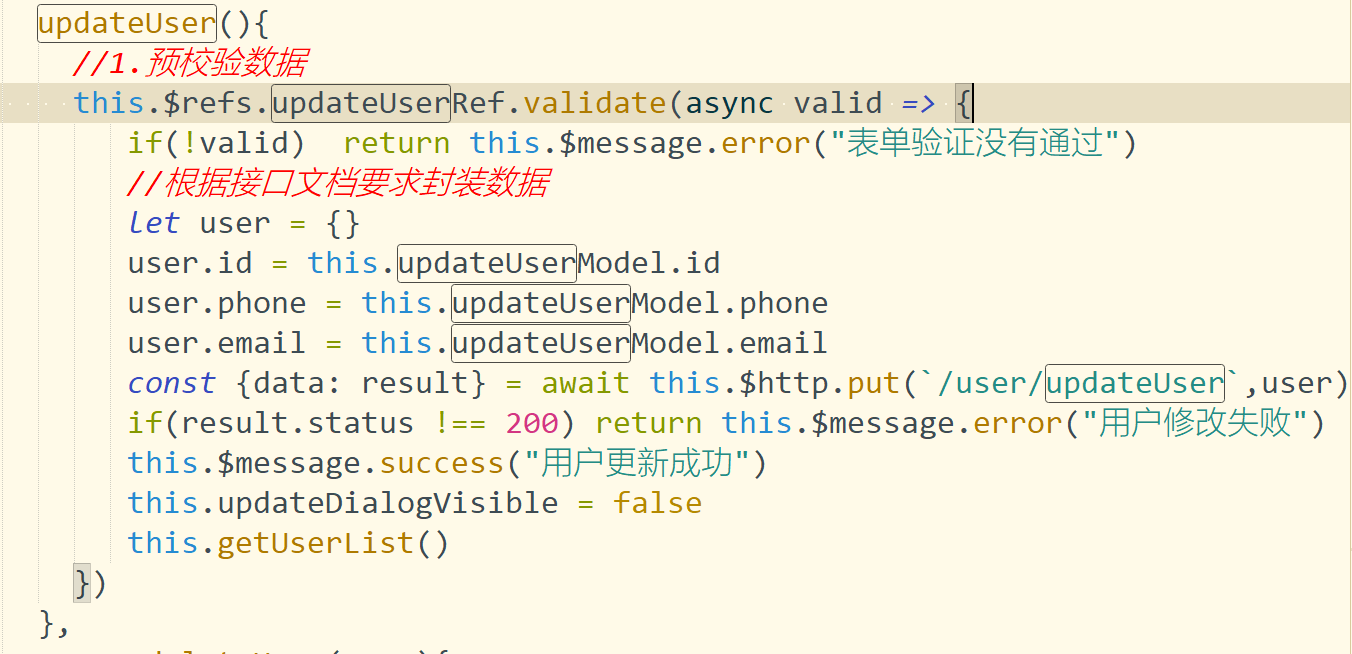
3.5.2 Modified Business Interface
- Request path: /user/updateUser
- Request type: PUT
- Request parameter: User object structure
Parameter Name | Parameter Description | Remarks |
---|
ID | User ID Number | Cannot be null |
phone | Text message | Cannot be null |
email | E-mail address | Cannot be null |
- Return value: SysResult object
Parameter Name | Parameter Description | Remarks |
---|
status | status information | 200 indicates successful server request 201 indicates server exception |
msg | Prompt information returned by the server | Can be null |
data | Business data returned by the server | null |
- The JSON format is as follows:
{
"status":200,
"msg":"Server call succeeded!",
"data":{}
}
3.5.3 Edit UserController
/**
* Business description: Implement data modification operations
* URL: /user/updateUser
* Parameter: user object
* Return value: SysResult object
* Request type: PUT
*/
@PutMapping("/updateUser")
public SysResult updateUser(@RequestBody User user){
userService.updateUser(user);
return SysResult.success();
}
3.5.4 Edit UserService
//id/phone/email
@Override
public void updateUser(User user) {
userMapper.updateUser(user);
}
3.5.5 Edit UserMapper
@Update("update user set phone=#{phone},email=#{email} where id=#{id}")
void updateUser(User user);
3.6 User Delete Action
3.6.1 Page JS Modification
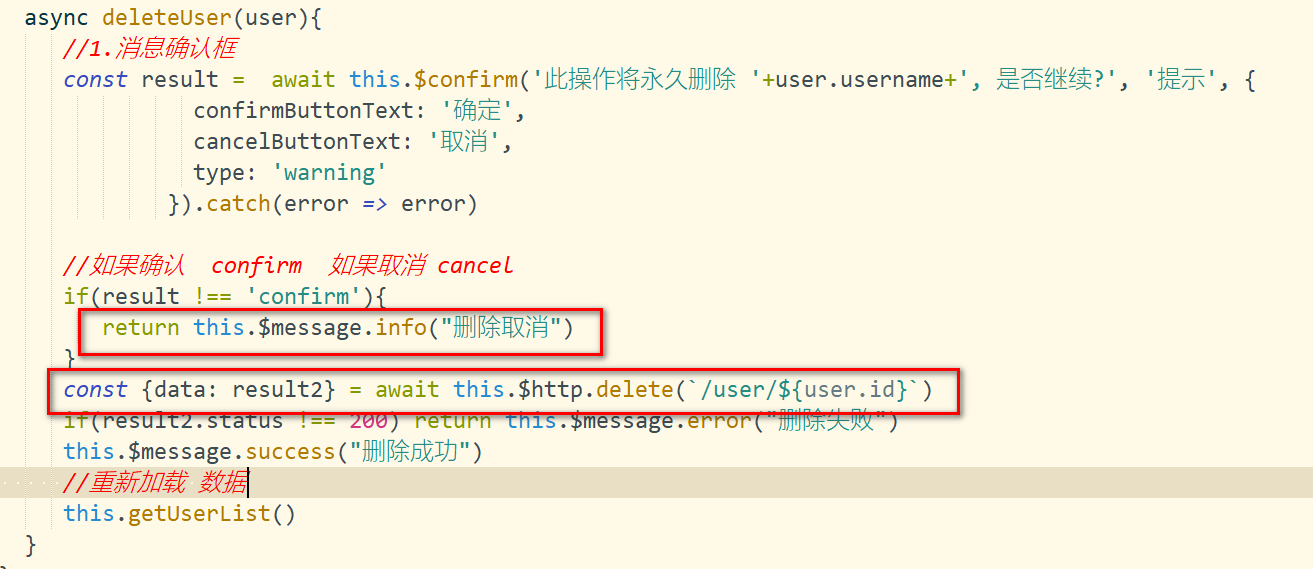
3.6.2 Delete Business Interface Documents
- Request path: /user/{id}
- Request type: delete
- Request parameters:
Parameter Name | Parameter Description | Remarks |
---|
ID | User ID Number | Cannot be null |
- Return value: SysResult object
Parameter Name | Parameter Description | Remarks |
---|
status | status information | 200 indicates successful server request 201 indicates server exception |
msg | Prompt information returned by the server | Can be null |
data | Business data returned by the server | null |
3.6.3 Edit UserController
/**
* Summary of Requests
* 1.General request get/delete? Key=value&key2=value2
* 2.post/put data: JS Object Backend Receive @RequestBody
* 3.restFul Style/url/arg1/arg2/arg3 Receive with Object
* Complete user deletion
* 1.URL Address/user/{id}
* 2.Parameter: id
* 3.Return value: SysResult
*/
@DeleteMapping("/{id}")
public SysResult deleteUserById(@PathVariable Integer id){
userService.deleteUserById(id);
return SysResult.success();
}
3.6.4 Edit UserService
@Override
public void deleteUserById(Integer id) {
userMapper.deleteUserById(id);
}
3.6.5 Edit UserMapper
@Delete("delete from user where id=#{id}")
void deleteUserById(Integer id);