Basic concepts of inner classes
Concept and classification of internal classes-------------------------------
When the definition of a class appears in the class body of another class, the class is called an Inner class
The class in which the inner class is located is called the outer class
Content in class: member variable, member method, constructor, static member, constructor and static code block, internal class
Practical role-------------------------------
When the value of a class is only to serve a class alone, this class can be defined as an internal class in the served class
In this way, the implementation details of the class can be hidden, and the private members of the external class can be easily accessed without providing public get and set methods
Ordinary inner class: directly put the definition of one class in the class body of another class
Static internal class: an internal class modified with the static keyword, which belongs to the class level
Local inner class: when the definition of a class is directly placed inside the method body
Anonymous inner class: an inner class without a name
Format of normal (member) inner class-------------------------------
Access modifier class Class name of external class{
Access modifier class The class name of the inner class{
Class body of inner class;
}
}
Like ordinary classes, ordinary inner classes can define member variables, member methods and construction methods
Common inner classes, like common classes, can be decorated with the final or abstract keywords
Ordinary inner classes can also be decorated with the private or protected keywords
Ordinary inner classes need to use outer class objects to create objects
If the internal class accesses a member variable or method with the same name in the external class, you need to use the this keyword
package com.lagou.task10;
/**
*Programming the definition and use of common internal classes - Document comments
*/
public class NormalOuter {
private int cnt = 1;
//Defines a common internal class, which is a member of an external class and is an object level
/*private*/ public /*final*/ class NormalInner{
//final prevents inheritance from other inner classes
private int ia = 2;
private int cnt = 3;
public NormalInner(){
System.out.println("The constructor body of the ordinary inner class has been executed");
}
public void show(){
System.out.println("Variables in external classes cnt The values are:" + cnt); //1
System.out.println("ia = " + ia); //2
}
public void show2(int cnt){
System.out.println("Formal parameter variable cnt = " + cnt); //Local priority principle
System.out.println("Inner class cnt = " + this.cnt); //3
System.out.println("External class cnt = " + NormalOuter.this.cnt); //1
//NormalOuter.this: this of the parent reference type, no.new NormalInner();, That is, the type of No
}
}
}
package com.lagou.task10;
/**
*
*/
public class NormalOuterTest {
public static void main(String[] args) {
//A reference declaring a NormalOuter type points to an object of that type
NormalOuter no = new NormalOuter();
//Declare that the reference to the inner class in the NormalOuter class points to the object of the inner class
NormalOuter.NormalInner ni = no.new NormalInner();
//NormalOuter.NormalInner ni = new NormalOuter.NormalInner();
//An error is reported because the internal class needs to access things of the external class
//If so, then ni is a separate reference, that is, only things of this class can be accessed
//That is, you can't access the external class, so it is stipulated that there must be a reference to the external class to create the object of the internal class
//Something that makes the object an external class
//Call the show method in the inner class
ni.show();
System.out.println("------------------");
ni.show2(4);
}
}
Format of static inner class-------------------------------
Access modifier class Class name of external class{
Access modifier static class The class name of the inner class{
Class body of inner class;
}
}
Static inner classes cannot directly access non static members of external classes
Static inner classes can create objects directly
If a static inner class accesses a member variable or method with the same name in the outer class, it needs to use the class name Access by
package com.lagou.task10;
/**
* Implement the definition and use of static internal classes
*/
public class StaticOuter {
private int cnt = 1; // Belongs to the object level
private static int snt = 2; // Belonging to class level
public /*static*/ void show() {
System.out.println("External class show The way is here!");
}
/**
* Static keyword is used to define static internal classes, which belong to class level
*/
public static class StaticInner {
private int ia = 3;
public static int snt = 4;
public StaticInner() {
System.out.println("Static internal class construction method Oh!");
}
public void show() {
System.out.println("ia = " + ia); // 3
System.out.println("In an external class snt = " + snt); // 2
//System.out.println("CNT of external class =" + CNT);
//Error: non static members cannot be accessed in a static context, so the object may not have been created at this time
}
public void show2(int snt) { // Proximity principle
System.out.println("snt = " + snt); // 5
System.out.println("Members in Inner Classes snt = " + /*StaticOuter.*/StaticInner.snt); // 4
//There can be static in static, that is, there are two ways to access
//If the names of other classes are StaticInner, the static variables of your own class will be used first due to the proximity principle
System.out.println("Members in external classes snt = " + StaticOuter.snt); // 2
//StaticOuter.show();
new StaticOuter().show();
}
}
}
package com.lagou.task10;
public class StaticOuterTest {
public static void main(String[] args) {
// 1. Declare that the reference of StaticInner type points to the object of this type
StaticOuter.StaticInner si = new StaticOuter.StaticInner();
//When the inner class is written, its name is staticouter StaticInner
//Instead of StaticInner, because when access is required, it must pass through the external class of the internal class
// 2. Call the show method to test
si.show();
System.out.println("---------------------------------------------");
si.show2(5);
}
}
Format of local (method) inner classes-------------------------------
Access modifier class Class name of external class{
Access modifier return value type member method name(parameter list ){
class The class name of the inner class{ //There is no modifier because it is released after the method is called
//That is, it is only related to the method
Class body of inner class;
}
}
}
How local inner classes are used-------------------------------
Local inner classes can only be used inside the method
Local inner classes can create objects directly inside methods
Local inner classes cannot use access control characters and static keyword modifiers
Local internal classes can use local variables of external methods, but they must be final, which is caused by the different declaration cycles of local internal classes and local variables
Local variables cannot use private and static modifiers
package com.lagou.task10;
/**
*Programming to achieve the definition and use of local internal classes
*/
public class AreaOuter {
private int cnt = 1;
public void show(){
//Define a local variable to test
//Starting from Java 8, it is understood by default as the content modified by the final keyword
//Although the final keyword can be omitted, it is recommended to add it
final int ic = 4;
//Define a local inner class, which is easy to use only inside the current method body
//In the underlying code, the inner class will copy an ic
//That is, final must be added, lest you ic change when I finish copying
class AreaInner{
private int ia = 2;
public AreaInner(){
System.out.println("Construction method of local inner class");
}
public void test(){
System.out.println("ia = " + ia); //2
System.out.println("cnt = " + cnt); //1
//ic = 5; Error
System.out.println("ic = " + ic); //4
}
}
//A reference declaring a local inner class points to an object of a local inner class
AreaInner ai = new AreaInner();
//This is possible because it is in the method, and the method is a separate space
//That is, you can create objects directly
ai.test();
}
}
package com.lagou.task10;
/**
*
*/
public class AreaOuterTest {
public static void main(String[] args) {
//A reference declaring an external class type points to an object of the external class
AreaOuter ao = new AreaOuter();
//The definition and use of local internal classes are realized through the call of show method
ao.show();
}
}
The concept of callback pattern-------------------------------
Callback mode means that if the parameter of a method is an interface type, an object implementing this interface type needs to be created and passed when calling the method
This method will call the method implemented in the parameter object (defined in the interface) at run time
package com.lagou.task10;
/**
*
*/
public interface AnonymousInterface {
//Custom abstract method
public abstract void show();
}
package com.lagou.task10;
/**
*
*/
public class AnonymousInterfaceImpl implements AnonymousInterface{
@Override
public void show() {
System.out.println("Here is the implementation class of the interface");
}
}
package com.lagou.task10;
/**
*
*/
public class AnonymousInterfaceTest {
//Assuming that the following methods exist, how can I call the following methods
//AnonymousInterface ai = new AnonymousInterfaceImpl();
//The reference of interface type points to the object of implementation type, forming polymorphism
public static void test(AnonymousInterface ai){
//The compilation stage calls the parent class version, and the runtime stage calls the version of the implementation class rewriting
ai.show();
}
public static void main(String[] args) {
//AnonymousInterfaceTest.test(new AnonymousInterface()); //Error: the interface cannot be instantiated
AnonymousInterfaceTest.test(new AnonymousInterfaceImpl());
//Callback: I pass my object to you, and then you call my internal method according to my object
System.out.println("--------------------");
//Use the syntax format of anonymous inner class to get the reference of interface type
//Format: Interface / parent type reference variable name = new interface / parent type () {override of method};
AnonymousInterface ait = new AnonymousInterface() {
@Override
public void show() {
System.out.println("That's how anonymous inner classes play");
}
};
//Advantage after the new object is completed, the value of this class does not exist, and its storage space can be destroyed
//Starting with Java 8, Lambda expressions can simplify the above code
//The format is: (parameter list) - > {method body}
AnonymousInterface ait2 = () -> System.out.println("Lamda expression");
//Can only be used to override the interface / parent type of a method
AnonymousInterfaceTest.test(ait2);
}
}
Use of anonymous inner classes-------------------------------
When the reference of interface / class type is used as the formal parameter of method, there are two ways to pass the argument
The custom class implements the interface / inheritance class and overrides the method, and then creates the class object to be passed as an argument
Use the syntax format of the above anonymous inner class to get the reference of the interface / class type
Syntax format of anonymous inner class
Interface/The parent type references the variable name = new Interface/Parent class type(){Method override};
Basic concepts of enumeration-------------------------------
All seasons of the year: spring, summer, autumn, winter
All genders: male, female
All direction keys on the keyboard: up, down, left, right
In daily life, the values of these things have only a few definite fixed values. At this time, all the values describing these things can be listed one by one
This enumerated type is called an enumerated type
package com.lagou.task10;
/**
*Programming enumeration of all directions, all directions: up, down, left, right
*/
public class Direction {
private final String desc; //Member variable used to describe the direction string
//A reference declaring this class type points to an object of this class type
public final static Direction UP = new Direction("Up");
public final static Direction DOWN = new Direction("down");
public final static Direction LEFT = new Direction("towards the left");
public final static Direction RIGHT = new Direction("towards the right");
//There are only four objects developed externally, that is, a limited number of objects
//Then such a class is called enumeration
//The initialization of member variables is realized by construction method, which is more flexible
//Privatize the constructor, which can only be used inside this class
private Direction(String desc){
this.desc = desc;
}
//Through the public get method, you can access the value of the member variable of this class outside this class
public String getDesc() {
return desc;
}
}
package com.lagou.task10;
/**
*
*/
public class DirectionTest {
public static void main(String[] args) {
/* //Declares that a reference to a Direction type points to an object of that type and prints the feature
Direction d1= new Direction("Upward ");
System.out.println("The obtained string is: "+ d1.getDesc()); / / up
Direction d2= new Direction("Down "");
System.out.println("The obtained string is: "+ d2.getDesc()); / / down
Direction d3= new Direction("Left "");
System.out.println("The obtained string is: "+ d3.getDesc()); / / left
Direction d4= new Direction("Right "");
System.out.println("The obtained string is: "+ d4.getDesc()); / / right
System.out.println("--------------------------");
Direction d5= new Direction("Forward ");
System.out.println("The obtained string is: "+ d5.getDesc()); / / forward
*/
//Direction.UP = 2; type mismatch
//Direction d2 = null;
//Direction UP = d2; Error:final keyword modification
Direction d1 = Direction.UP;
System.out.println("The obtained direction is:" + d1.getDesc()); //Up
System.out.println("-------------------------");
//Use the enumeration types that start with Java 5
DirectionEnum de = DirectionEnum.DOWN;
System.out.println("The obtained direction is:" + de.getDesc());
}
}
Definition of enumeration-------------------------------
The description of constants represented by public static final is cumbersome. enum keyword is used to define enumeration type instead of constants
Enumeration type is a reference data type added from Java 5
The enumeration value is the type of the current class, that is, the object pointing to this class. By default, it is decorated with public static final, so the enumeration type is adopted Called by
Enumeration classes can customize the constructor, but the modifier of the constructor must be private, which is also private by default
package com.lagou.task10;
/**
*Programming enumeration of all directions, all directions: up, down, left, right
*/
public enum DirectionEnum implements DirectionInterface{
//A reference declaring this class type points to an object of this class type
//enum enumeration types are initialized automatically. There is no need to initialize them
//Enumeration types require that all enumeration values be placed first of the enumeration types
//Syntax format of anonymous inner class: Interface / parent type reference variable name = new interface / parent type () {override of method};
//public final static DirectionEnum DOWN = new DirectionEnum("up");
UP("Up"){
@Override
public void show() {
System.out.println("The greedy snake moved upward");
}
},DOWN("down") {
@Override
public void show() {
System.out.println("The greedy snake moved down");
}
},LEFT("towards the left") {
@Override
public void show() {
System.out.println("Moved to the left");
}
},RIGHT("towards the right") {
@Override
public void show() {
System.out.println("Moved to the right");
}
};
private final String desc; //Member variable used to describe the direction string
/* public final static DirectionEnum UP = new DirectionEnum("Upward ");
public final static DirectionEnum DOWN = new DirectionEnum("Down "");
public final static DirectionEnum LEFT = new DirectionEnum("Left "");
public final static DirectionEnum RIGHT = new DirectionEnum("Right "");
*/
//There are only four objects developed externally, that is, a limited number of objects
//Then such a class is called enumeration
//The initialization of member variables is realized by construction method, which is more flexible
//Privatize the constructor, which can only be used inside this class
private DirectionEnum(String desc){
this.desc = desc;
}
//Through the public get method, you can access the value of the member variable of this class outside this class
public String getDesc() {
return desc;
}
//The entire enumeration type is rewritten only once, and all objects call the same
/*@Override
public void show() {
System.out.println("You can now override the abstract method in the interface ");
}*/
}
Use of custom classes and enumeration types in switch structure-------------------------------
package com.lagou.task10;
/**
*
*/
public class DirectionUseTest {
//The custom static method can print the specific direction information according to the string content specified by the parameter
public static void test1(String str){
switch(str){
case "Up":
System.out.println("Look up at the moon"); break;
case "down":
System.out.println("Bow your head and think of your hometown"); break;
case "towards the left":
System.out.println("Zuo Qianhuang"); break;
case "towards the right":
System.out.println("Right Qingcang"); break;
default:
System.out.println("There is no such way");
}
}
public static void test2(DirectionEnum de){
//switch supports enumeration types
//That is, up, down, left and right here can be used without classes Access by
switch(de){
case UP:
System.out.println("Look up at the moon"); break;
case DOWN:
System.out.println("Bow your head and think of your hometown"); break;
case LEFT:
System.out.println("Zuo Qianhuang"); break;
case RIGHT:
System.out.println("Right Qingcang"); break;
default:
System.out.println("There is no such way");
}
}
public static void main(String[] args) {
DirectionUseTest.test1(Direction.UP.getDesc());
DirectionUseTest.test1("How are you today");
System.out.println("--------------------------");
DirectionUseTest.test2(DirectionEnum.DOWN);
//DirectionUseTest.test2("how are you today"); Error: type mismatch, reducing the possibility of error
//Because directionenum de = directionenum DOWN;
//Where DOWN is a reference of type DirectionEnum
//For example, public final static DirectionEnum DOWN = new DirectionEnum("down");
}
}
Concept and common methods of Enum class-------------------------------
All enumeration classes inherit from Java Lang. enum class, the common methods are as follows

package com.lagou.task10;
/**
*Program to implement the test of direction enumeration class and call the methods inherited from Enum class
*/
public class DirectionEnumTest {
public static void main(String[] args) {
//Gets all enumeration objects in the DirectionEnum type
DirectionEnum[] arr = DirectionEnum.values();
//Prints the name and index position of each enumerated object in the enumeration type
for(int i =0; i < arr.length; i++){
System.out.println("The obtained enumeration object name is:" + arr[i].toString());
System.out.println("The index position corresponding to the obtained enumeration object is:" + arr[i].ordinal());
}
System.out.println("--------------------");
//The enumeration type object is obtained according to the string specified by the parameter, that is, the string is converted to an object
//DirectionEnum de = DirectionEnum.valueOf("down");
//DirectionEnum de = DirectionEnum.valueOf("UP LEFT");
//Requires that the string name must exist in the enumeration object
//Compile ok, run IllegalArgumentException, illegal parameter exception
DirectionEnum de = DirectionEnum.valueOf("DOWN");
//System.out.println("the converted enumeration object name is:" + de.toString());
System.out.println("The converted enumeration object name is:" + de);
//The toString() method is automatically called when printing reference variables
System.out.println("--------------------");
//Use the obtained enumeration object to compare the order with the existing objects in the enumeration class
for(int i = 0; i< arr.length; i++){
//When the calling object is behind the parameter object, the comparison result obtained is a positive number
//When the calling object is in the same position as the parameter object, the comparison result obtained is 0
//When the calling object is before the parameter object, the comparison result obtained is negative
//For example, if my position is 1, there are parameter positions 0 and 3, and the result is 1, - 2
System.out.println("The sequence result of comparing the calling object with the object in the array is:" + de.compareTo(arr[i]));
}
System.out.println("--------------------");
//Use each DirectionEnum object in the array to call the show method to test
for(int i = 0; i<arr.length; i++){
arr[i].show();
}
}
}
Enumerating how classes implement interfaces-------------------------------
Enumeration class inherits Java. Net by default Lang. enum class, that is, it can only implement interfaces
After the enumeration class implements the interface, it needs to rewrite the abstract method, and there are two ways to rewrite the method: rewrite one or every object
Basic concepts of annotation-------------------------------
Annotation, also known as annotation, is a reference data type added from Java 5
Enumeration can be regarded as a special class, while annotation can be regarded as a special interface
Annotations are essentially special tags in code that allow you to perform specified processing during compilation, class loading, and runtime
Syntax format of annotations-------------------------------
Access modifier @interface Annotation name{
Annotation member
}
Custom annotation automatically inherits Java lang.annotation. Annotation interface
The @ annotation name can modify the declarations of packages, classes, member methods, member variables, construction methods, parameters, local variables, etc
How annotations are used-------------------------------
In the annotation body, only member variables have no member methods, and the member variables of the annotation are declared in the form of "method without formal parameters"
Its method name defines the name of the member variable, and its return value defines the type of the member variable
If the annotation has only one parameter member, it is recommended to use the parameter name value
The type can only be eight basic data types, String type, Class type (not Class type), enum type and Annotation type
Concept of meta annotation-------------------------------
Meta annotation is an annotation that can be annotated to annotations, or meta annotation is a basic annotation, but it can be applied to other annotations
Annotation only
Annotations will not affect other annotations when they are used for services and classes, because they are only used to mark / identify
Meta annotations mainly include: @ Retention, @ Documented, @ Target, @ Inherited, @ Repeatable
Meta annotation @ Retention-------------------------------
@Retention is applied to an annotation to describe its life cycle. The values are as follows:
RetentionPolicy. The source annotation is reserved only in the source phase and will be discarded and ignored when the compiler compiles (java) = > (class) x
RetentionPolicy. The class annotation is only kept until the compilation, and it will not be loaded into the JVM. The default mode is (class) = > (JVM) x
RetentionPolicy. The runtime annotation can be kept until the program runs, and it will be loaded into the JVM
So you can get them (JVM) when the program is running
Meta annotation @ Documented-------------------------------
Using javadoc tool, you can extract comments such as classes, methods and members from the program source code to form an API help document supporting the source code
The tool does not include annotation content by default
Click Generate JavaDoc in the Tools tool in the IDEA to select the class to generate the document and the directory to store it
You can also write - encoding utf-8 setting code on other command line parameters (the second of the bottom three grids)
When in use, the storage location of this code cannot have a Chinese path, and the storage directory can have a Chinese path
@Documented is used to specify that annotations will be extracted into documents by the javadoc tool
Annotation must be set to the value of @ RUNTIME document
Meta annotation @ Target-------------------------------
@Target is used to specify which elements can be modified by the modified annotation. The values are as follows:
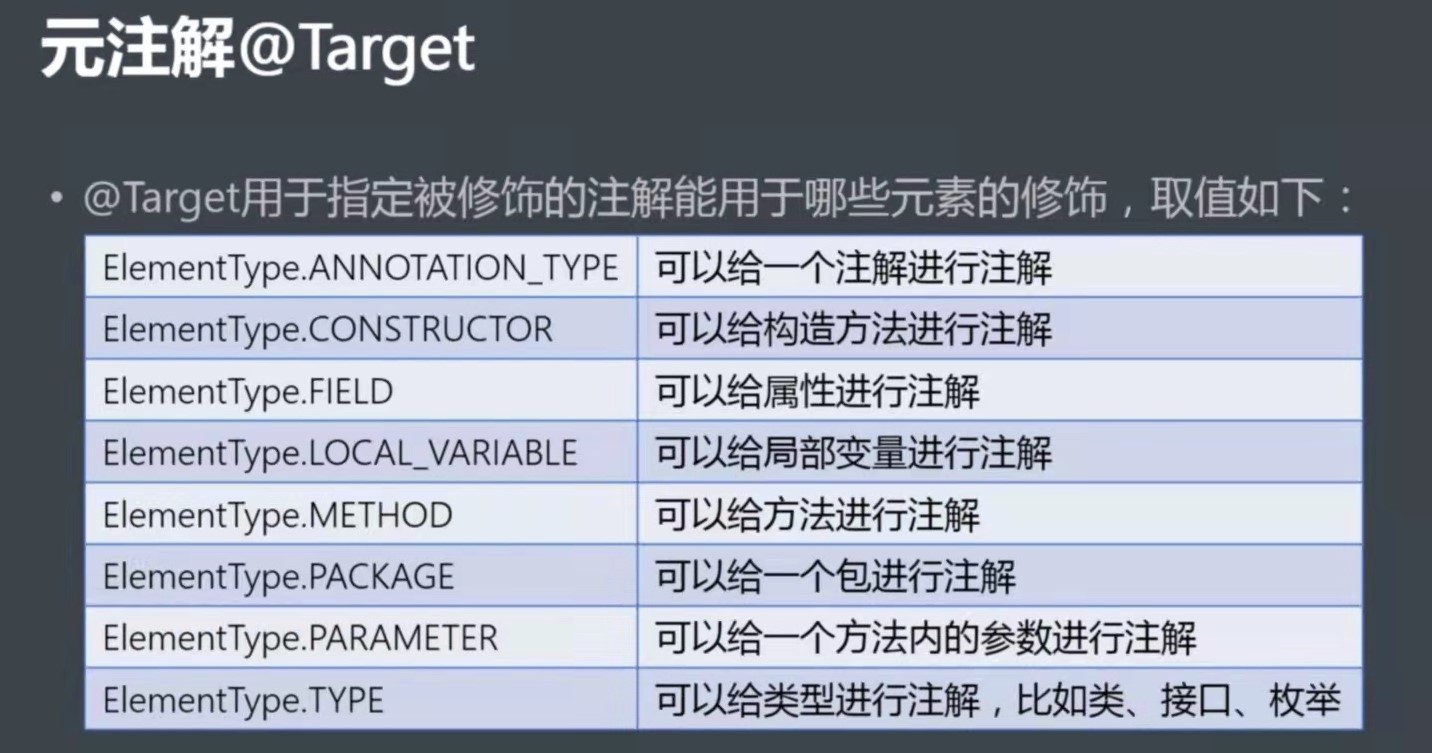
Meta annotation @ Inherited-------------------------------
@Inherited does not mean that the annotation itself can be inherited, but if a superclass is annotated by the annotation marked by the annotation
If no annotation is applied to the subclass, the subclass inherits the annotation of the superclass
Meta annotation @ Repeatable-------------------------------
@Repeatable means naturally repeatable, a new feature added from Java 8
Starting from Java 8, the parameter type ElementType enumeration value of meta annotation @ Target has been increased by two:
Where ElementType TYPE_ Parameter indicates that the annotation can be written in the declaration statement of type variables (such as T,E, etc. in generics), such as generics
Where ElementType TYPE_ Use indicates that the annotation can be written in any statement using the type
package com.lagou.task10;
import java.lang.annotation.*;
/**
*
*/
//@Retention(RetentionPolicy.SOURCE) / / indicates that the following comments are valid in the source code
//@Retention(RetentionPolicy.CLASS) / / indicates that the following annotations are valid in bytecode files. The default method is
@Retention(RetentionPolicy.RUNTIME) //Indicates that the following annotation is valid at run time
//If there are no members in an annotation, such annotation is called tag annotation / identification annotation
@Documented //Indicates that the following annotation information can be extracted by javadoc tool
@Target({ElementType.TYPE,ElementType.CONSTRUCTOR,
ElementType.FIELD,ElementType.METHOD,ElementType.PARAMETER})
//Indicates that the following annotations can be used to modify types, constructor methods, member variables and member methods
//When @ Target has parameters, the default modifications will not be default. If a modification is deleted at this time, an error about this modification will be reported on the other side of the class
//As noted here, you can modify the ElementType of the class Type, then you can't modify the class without @ Target annotation
//Then the @ MyAnnotation annotation has a default modification, which is basically the modification of @ Target
//That is, @ Target can limit the modification of annotations
@Inherited //Indicates that when the following annotation modifies a class, the annotation can be inherited by subclasses
public @interface MyAnnotation {
//shift+tab to the front, tab to the back
//ctrl+shift+enter can go to the next line without clicking the last
//public Direction value(); // Declare a member variable of String type with the name value
//Type required
//Declare a member variable of String type with the name value
public String value() default "123";
//Write the default value 123 for this annotation, so you can not write the parameter name when using the annotation
public String value2();
}
package com.lagou.task10;
/**
*
*/
//Indicates that the tag MyAnnotation is pasted in the code of the Person class
//When using annotation, adopt member parameter name = member parameter value
//@There are no members in the MyAnnotation annotation. If there are members, there must be parameters
//However, you can also have members without writing parameters. For example, you can write the default value "default value" for the parameter
//@MyAnnotation(value2 = "world")
@MyAnnotation(value = "hello",value2 = "world")
public class Person {
/**
* name Is a member variable that describes the name
*/
@MyAnnotation(value2 = "1")
private String name;
/**
* age Is a member variable used to describe age
*/
private int age;
/**
* The custom member method implements the parameterless construction method
*/
@MyAnnotation(value2 = "2")
public Person() {
}
/**
* Programming parametric construction method
* @param name
* @param age
*/
public Person(@MyAnnotation(value2 = "3")String name, int age) {
this.name = name;
this.age = age;
}
/**
* The custom member method realizes the acquisition and modification of features
* @return
*/
@MyAnnotation(value2 = "3")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
package com.lagou.task10;
/**
*
*/
//That is, you can inherit the annotation of the Person class
//The premise is that the annotation of the Person is annotated by the meta annotation @ Inherited (identification / tag)
public class Student extends Person {
}
package com.lagou.task10;
import java.lang.annotation.ElementType;
import java.lang.annotation.Repeatable;
import java.lang.annotation.Target;
/**
*Custom annotations are used to describe the character's role
*/
@Repeatable(value = ManTypes.class)
//ManType itself cannot be used more than once
//That is, you need to rely on the ManTypes annotation with ManType array
//@The parameter value of the Repeatable annotation is class
//That is, ManTypes are required class
@Target(ElementType.TYPE_USE)
public @interface ManType {
String value() default "";
}
package com.lagou.task10;
import java.lang.annotation.ElementType;
import java.lang.annotation.Target;
/**
*User defined annotations can describe multiple roles
*/
@Target(ElementType.TYPE_USE)
public @interface ManTypes {
ManType[] value();
}
package com.lagou.task10;
/**
*
*/
@ManType(value = "workers")
@ManType(value = "superman")
//@ManTypes({@ManType(value = "employee"), @ ManType(value = "Superman")})
//How to handle multiple annotations before Java 8
public class Man {
public static void main(String[] args) {
int ia = 97;
char c1 = (@ManType char)ia;
}
}
Common prefabricated notes-------------------------------
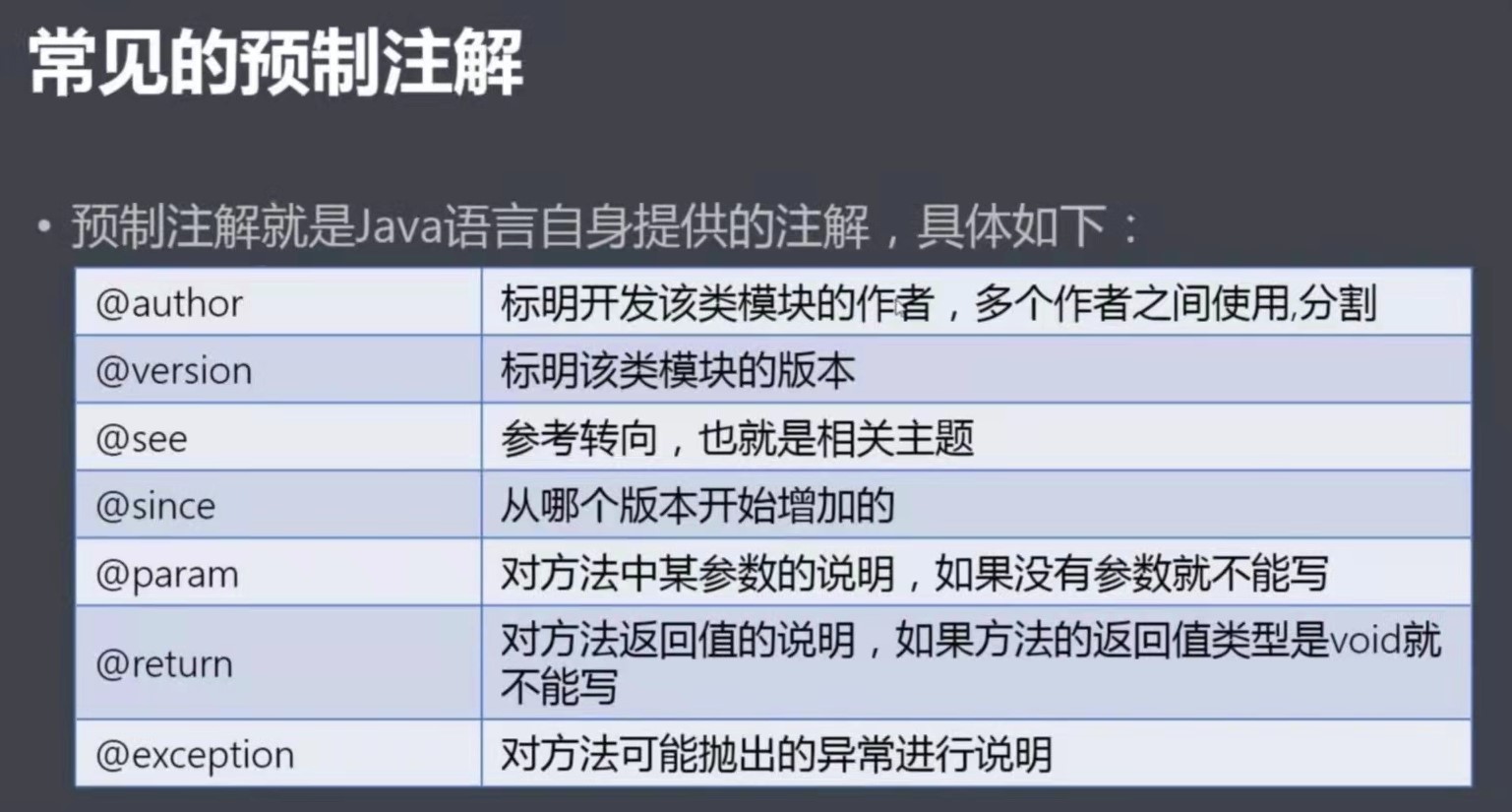
@Override: restrict overriding parent class methods. This annotation can only be used for methods
@Deprecated: used to indicate that the modified element (class, method, etc.) is obsolete
@SuppressWarnings: suppresses compiler warnings