Question 1:
I'll give you an ordered array {nums, please In situ Delete the repeated elements so that each element {only appears once, and return the new length of the deleted array.
Do not use additional array space, you must In situ Modify the input array {and do it with O(1) extra space.
explain:
Why is the returned value an integer, but the output answer is an array?
Note that the input array is passed by reference, which means that modifying the input array in the function is visible to the caller.
You can imagine the internal operation as follows:
//nums is passed by reference. That is, no copy of the arguments is made int len = removeDuplicates(nums); //Modifying the input array in the function is visible to the caller. //According to the length returned by your function, it will print all elements within that length range in the array. for (int i = 0; i < len; i++) { print(nums[i]); }
Example 1:
Input: nums = [1,1,2] Output: 2, nums = [1,2] Explanation: the function should return a new length of 2 and the original array nums The first two elements of are modified to 1, 2 . There is no need to consider the elements in the array that exceed the new length.
Example 2:
Input: nums = [0,0,1,1,1,2,2,3,3,4] Output: 5, nums = [0,1,2,3,4] Explanation: the function should return a new length of 5 and the original array nums The first five elements of are modified to 0, 1, 2, 3, 4 . There is no need to consider the elements in the array that exceed the new length.
Tips:
- 0 <= nums.length <= 3 * 104
- -104 <= nums[i] <= 104
- nums # is arranged in ascending order
1 class Solution { 2 public int removeDuplicates(int[] nums) { 3 if (nums.length<2) return nums.length; 4 int l=0,r=1; 5 while (r<=nums.length) { 6 while (r<nums.length&&nums[l]==nums[r]) r++; 7 if (r==nums.length) return l+1; 8 else { 9 l++; 10 nums[l]=nums[r]; 11 } 12 } 13 return l+1; 14 } 15 }
Double pointer, the left pointer represents the current position, and the right pointer searches for the next different number
Question 2:
There is a linked list arranged in ascending order. Give you the head node , head of the linked list. Please delete all duplicate elements so that each element , appears only once.
Returns a linked list of results in the same ascending order.
Example 1:
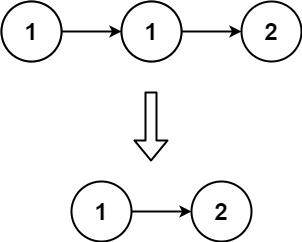
Input: head = [1,1,2] Output: [1,2]
Example 2:
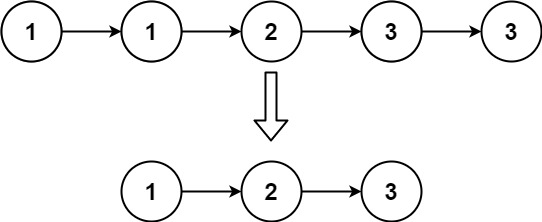
Input: head = [1,1,2,3,3] Output: [1,2,3]
Tips:
- The number of nodes in the linked list is within the range [0, 300]
- -100 <= Node.val <= 100
- The title data ensures that the linked list has been arranged in ascending order
1 /** 2 * Definition for singly-linked list. 3 * public class ListNode { 4 * int val; 5 * ListNode next; 6 * ListNode() {} 7 * ListNode(int val) { this.val = val; } 8 * ListNode(int val, ListNode next) { this.val = val; this.next = next; } 9 * } 10 */ 11 class Solution { 12 public ListNode deleteDuplicates(ListNode head) { 13 if (head==null) return head; 14 ListNode l=head,r=head; 15 while (r!=null){ 16 if (r.val!=l.val) { 17 l.next=r; 18 l=l.next; 19 } 20 r=r.next; 21 } 22 l.next=null; 23 //Pay attention to the last position next Leave blank 24 return head; 25 } 26 }
Give you an array , num , and a value , val, you need In situ Removes all elements with a value equal to {val}, and returns the new length of the array after removal.
Do not use extra array space, you must only use {O(1) extra space and In situ Modify the input array.
The order of elements can be changed. You don't need to consider the elements in the array that exceed the new length.
explain:
Why is the returned value an integer, but the output answer is an array?
Note that the input array is passed by reference, which means that modifying the input array in the function is visible to the caller.
You can imagine the internal operation as follows:
//nums is passed by reference. That is, no copy of the arguments is made int len = removeElement(nums, val); //Modifying the input array in the function is visible to the caller. //According to the length returned by your function, it will print all elements within that length range in the array. for (int i = 0; i < len; i++) { print(nums[i]); }
Example 1:
Input: num = [3,2,2,3], Val = 3 Output: 2, Num = [2,2] Explanation: the function should return a new length of 2, and the first two elements in num are 2. You don't need to consider the elements in the array that exceed the new length. For example, if the new length returned by the function is 2, and num = [2,2,3,3] or num = [2,2,0,0], it will also be regarded as the correct answer.
Example 2:
Input: nums = [0,1,2,2,3,0,4,2], val = 2 Output: 5, nums = [0,1,4,0,3] Explanation: the function should return a new length of 5, also nums The first five elements in are 0, 1, 3, 0, 4. Note that these five elements can be in any order. You don't need to consider the elements in the array that exceed the new length.
Tips:
- 0 <= nums.length <= 100
- 0 <= nums[i] <= 50
- 0 <= val <= 100
1 class Solution { 2 public int removeElement(int[] nums, int val) { 3 if (nums.length==0) return 0; 4 int l=0,r=0; 5 while (r<nums.length) { 6 if (nums[r]!=val){ 7 nums[l]=nums[r]; 8 l++; 9 } 10 r++; 11 } 12 return l; 13 } 14 }
Question 4:
Given an array of {nums, write a function to move all {0} to the end of the array while maintaining the relative order of non-zero elements.
Example:
input: [0,1,0,3,12] output: [1,3,12,0,0]
explain:
- You must operate on the original array and cannot copy additional arrays.
- Minimize the number of operations.
1 class Solution { 2 public void moveZeroes(int[] nums) { 3 int l=0,r=0; 4 if (nums.length==0) return; 5 while (r<nums.length){ 6 if (nums[r]!=0) { 7 int t=nums[r]; 8 nums[r]=nums[l]; 9 nums[l]=t; 10 l++; 11 } 12 r++; 13 } 14 } 15 }