Intermediary mode:
Mediator mode, which encapsulates a series of object interactions with a mediation object. Mediators make objects do not need to explicitly refer to each other, so that they are loosely coupled, and can independently change the interaction between them.
The main solution: there are a lot of relationships between objects, which will inevitably lead to the complexity of the system structure. At the same time, if an object changes, we also need to track the objects associated with it, and make corresponding processing.
When to use: multiple classes are coupled to form a mesh structure.
How to solve this problem: the mesh structure is separated into star structure.
For example:
UML structure diagram of mediator pattern:
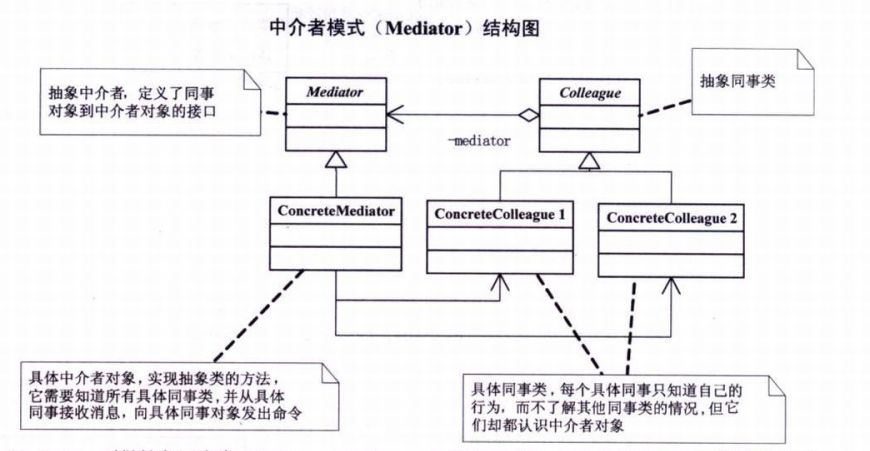
For example:
MVC framework, where C (controller) is the mediator of M (model) and V (view).
The Form object of the Form (the communication between each control depends on the Form object (the communication is carried out through the event mechanism) (the execution of the event mechanism is carried out in the Form object), so that each control does not need to pay attention to the communication with other controls, reducing the coupling degree) or the Web interface aspx, and wants to customize the behavior distributed in multiple classes without Where you want to generate too many subclasses.
Advantages: 1. Reduce the complexity of the class and convert one to many into one to one. 2. Decoupling between classes. 3. In line with the Dimitar principle.
Disadvantages: intermediaries can be large and complex to maintain.
Difference between appearance (facade) mode and intermediary mode:
The facade pattern focuses on how the complex set of objects communicate with the outside world
The mediator pattern focuses on the internal communication between this complex set of objects
The relationship between observer model and intermediary model:
It can be used in combination to inform the mediator object when the colleague object changes, and let the mediator object interact with other related objects.
Example (United Nations and peace):
//United Nations agencies abstract class UnitedNations { /// <summary> ///Declaration /// </summary> ///< param name = "message" > declaration information < / param > ///< param name = "colleague" > declared country < / param > public abstract void Declare(string message, Country colleague); } //UN Security Council class UnitedNationsSecurityCouncil : UnitedNations { private USA colleague1; private Iraq colleague2; public USA Colleague1 { set { colleague1 = value; } } public Iraq Colleague2 { set { colleague2 = value; } } public override void Declare(string message, Country colleague) { if (colleague == colleague1) { colleague2.GetMessage(message); } else { colleague1.GetMessage(message); } } } //country abstract class Country { protected UnitedNations mediator; public Country(UnitedNations mediator) { this.mediator = mediator; } } //U.S.A class USA : Country { public USA(UnitedNations mediator) : base(mediator) { } //statement public void Declare(string message) { mediator.Declare(message, this); } //Get message public void GetMessage(string message) { Console.WriteLine("Us access to each other's information:" + message); } } //Iraq class Iraq : Country { public Iraq(UnitedNations mediator) : base(mediator) { } //statement public void Declare(string message) { mediator.Declare(message, this); } //Get message public void GetMessage(string message) { Console.WriteLine("Iraq obtains information from each other:" + message); } } class Program { static void Main(string[] args) { UnitedNationsSecurityCouncil UNSC = new UnitedNationsSecurityCouncil(); USA c1 = new USA(UNSC); Iraq c2 = new Iraq(UNSC); UNSC.Colleague1 = c1; UNSC.Colleague2 = c2; c1.Declare("No development of nuclear weapons, or war!"); c2.Declare("We have no nuclear weapons and are not afraid of aggression."); Console.Read(); } }