It has been more than two years since I unknowingly used Go to develop. The experience summary of the preparation point is convenient for later students to get started quickly.
outline
1. Go installation
2. Go ide building
3. Go modules module management
4. Go unit test
5. Go debug
6. Go pprof flame diagram
7. Go online debugging
8. Go future thinking
1. Go installation
Official website: https://golang.org/
Download page https://golang.org/dl/
Page https://golang.org/doc/install
Log in to the official website of Golang. If there is a problem with the visit, please inspire the greatest potential in your life to break through the magic barrier in depth.
Don't ask
Ask
It's just not appropriate
Don't think about it
Think
No chance
Then click [2019 / 10 / 21] to the download page, where the download is go1.12.12.linux-amd64.tar.gz
Then follow the instructions on the installation page. Here is a brief overview of the following script
sudo rm -rf /usr/local/go
sudo tar -C /usr/local -xzf go1.12.12.linux-amd64.tar.gz
sudo vi /etc/profile
Shift + G
i
export PATH=$PATH:/usr/local/go/bin
~
wq!
source /etc/profile
View the installed version through go version

I originally wanted to show you the contents of the outline through the window platform. But I often stayed next to ubuntu.
You can only use linux environment to demonstrate it to your classmates. If you want to do it, you can choose your own environment and practice. The ideas are almost the same.
2. Go ide building
Download VSCode https://code.visualstudio.com/
Here, it is recommended that VSCode build, Golang develop and replace, download and install the deb package.
sudo dpkg -i code_1.39.2-1571154070_amd64.deb
Prepare the following environment
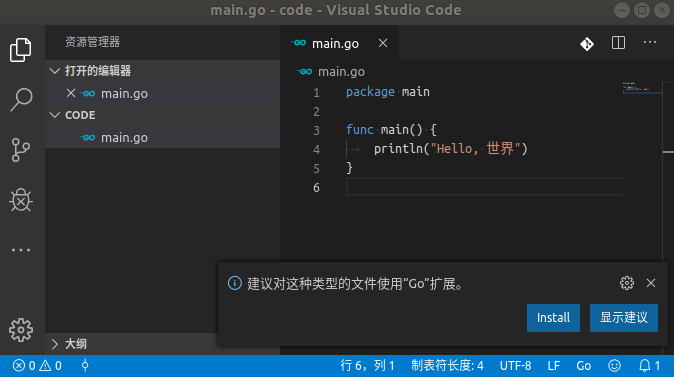
Click [install] - end - > [F1] - > [Go: Install/Update Tools] - > [Select all] - > [OK]
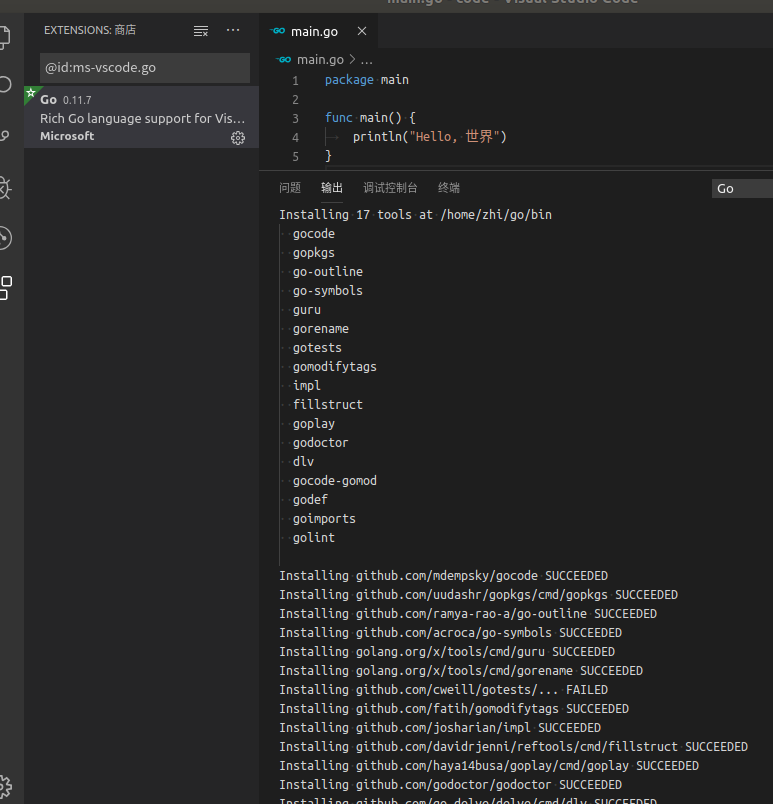
After all of them are successful, run towards the project [F5]
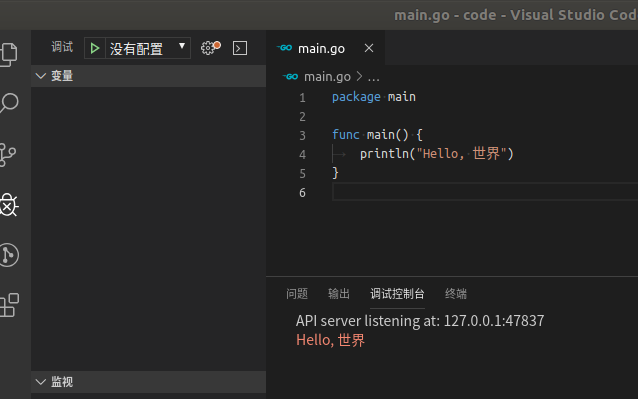
Don't be surprised, our IDE is OK!
3. Go modules module management
Go modules is a great attempt to solve the problem of package dependency management.
Interested students can query more relevant information, or promote themselves through go mod help. Start to prepare materials.
code/arithmetic/div.go
package arithmetic
import "errors"
// Div division operation
func Div(a, b int) (c int, err error) {
if b == 0 {
err = errors.New("divisor is 0")
return
}
c = a / b
return
}
code/main.go
package main
import (
"fmt"
"code/arithmetic"
)
func main() {
println("Hello, world")
c, err := arithmetic.Div(1, 0)
fmt.Printf("c = %d, err = %+v\n", c, err)
}
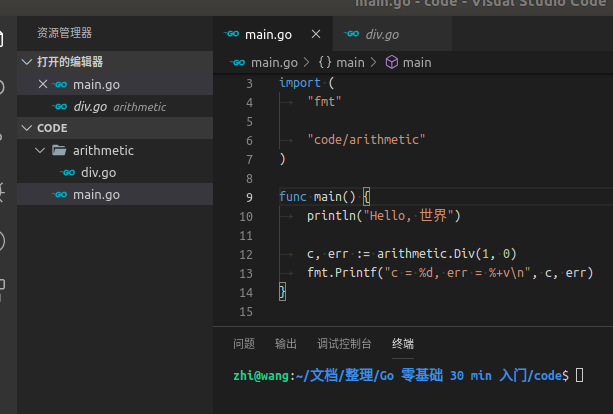
With these, start building
go mod init code
go build
./code
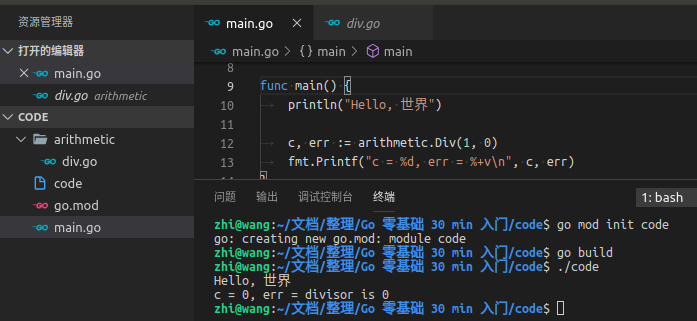
The purer, the better
4. Go unit test
code/arithmetic/div_test.go
package arithmetic
import (
"testing"
"math/rand"
)
func TestDiv(t *testing.T) {
var (
a, b, c int
err error
)
a, b = 1, 2
c, err = Div(a, b)
t.Logf("a = %d, b = %d, c = %d, err = %+v", a, b, c, err)
a, b = 1, 0
c, err = Div(a, b)
t.Logf("a = %d, b = %d, c = %d, err = %+v", a, b, c, err)
}
func BenchmarkDiv(b *testing.B) {
for i := 0; i < b.N; i++ {
Div(rand.Int(), rand.Int())
}
}
The unit test in Go is very simple. The special requirement is naming. In summary, it has the following points
1. The file name must be * * test.go
Test func tion (t * testing.T) {...}
Performance test function func Benchmark*(b * testing.B) {for i: = 0; < i < b.N; + i + + {...}}}
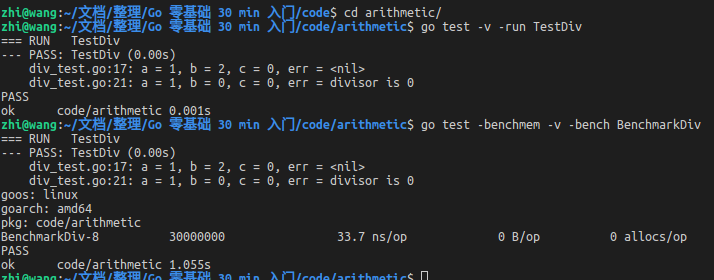
It's much better to have a test than not./~~
5. Go debug
Don't go into it
Deep research
F5 F10 F11 go race
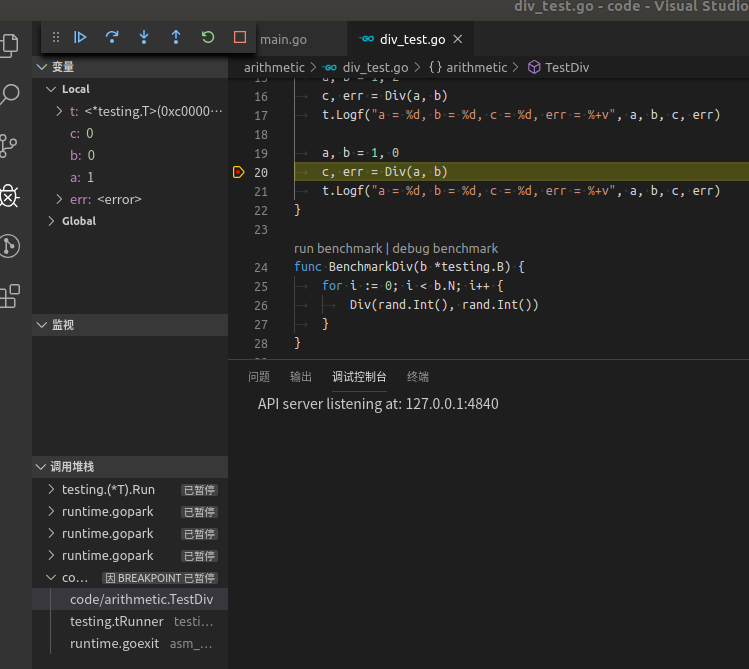
6. Go pprof flame diagram
Preparation material
package main
import (
"fmt"
"log"
"time"
"net/http"
_ "net/http/pprof"
"code/arithmetic"
)
func main() {
println("Hello, world")
c, err := arithmetic.Div(1, 0)
fmt.Printf("c = %d, err = %+v\n", c, err)
for i := 0; i < 1000000; i++ {
go func() {
time.Sleep(time.Second * 10)
}()
}
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {})
err = http.ListenAndServe(":8088", nil)
if err != nil {
log.Fatalf("ListenAndServe: %+v\n", err)
}
}
Start the service, view the running status of the program
go pprof debug http://127.0.0.1:8088/debug/pprof/
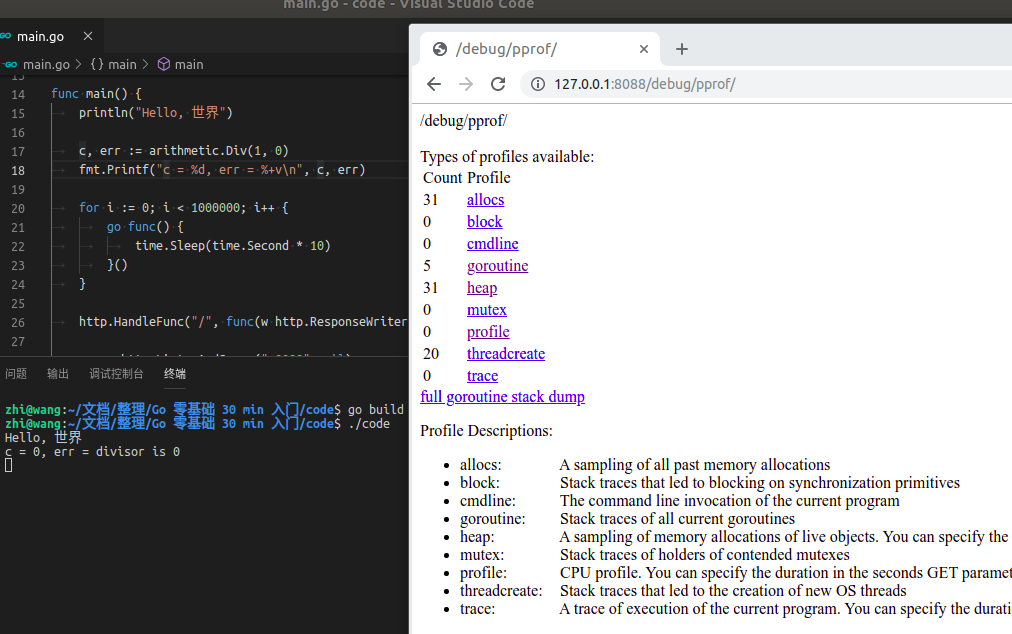
For more details, you can check the cpu running flame chart in one piece.
go tool pprof http://127.0.0.1:8088/debug/pprof/profile -seconds 10
A profile related file will be generated, and open pprof.code.samples.cpu.001.pb.gz with the tool.
go tool pprof -http=:8081 /home/zhi/pprof/pprof.code.samples.cpu.001.pb.gz
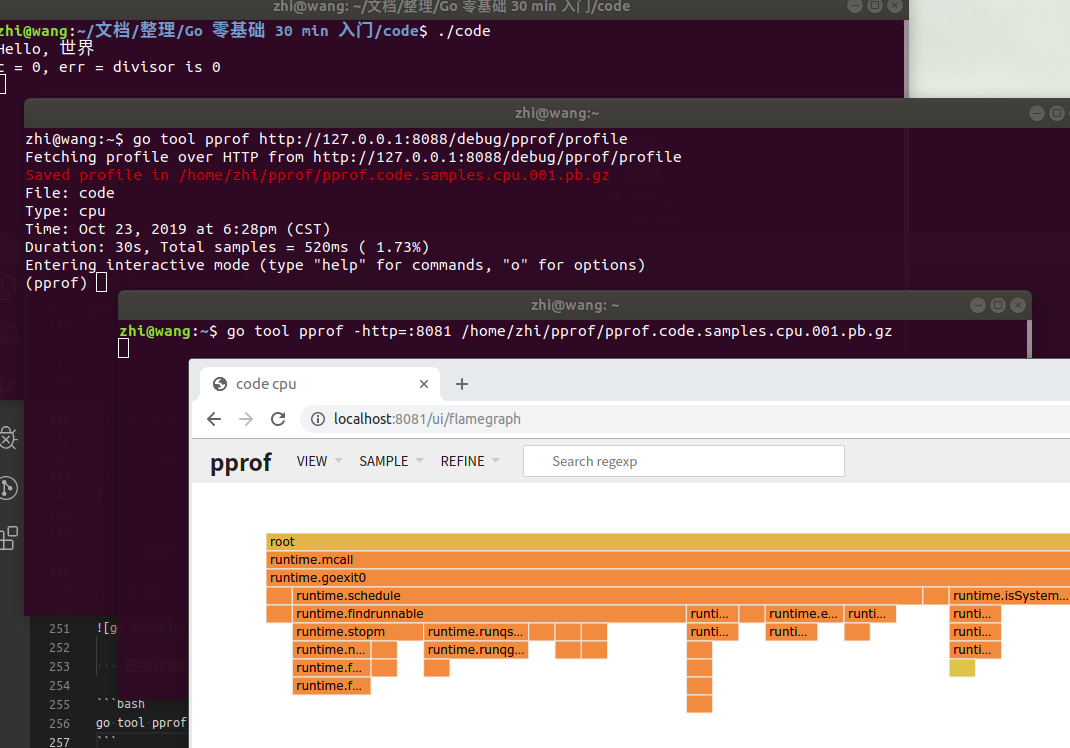
With all of them, I don't know if they add to the tiger's strength, but I know that you have more ways to deal with problems.
7. Go online debugging
delve https://github.com/go-delve/delve
Instead of looking at the log, you can also perform b r n c through delete after the service is removed.
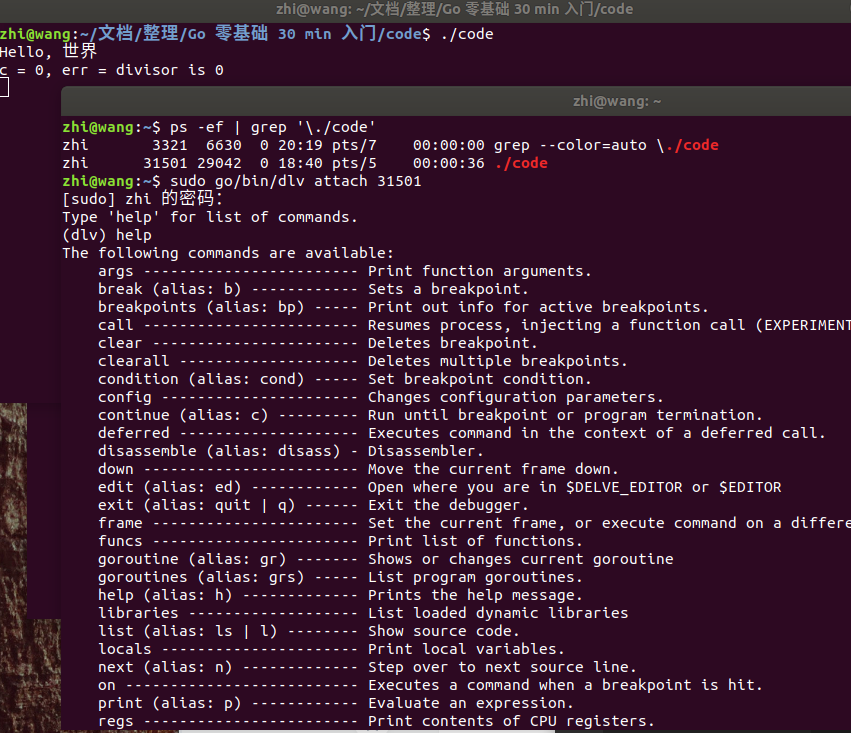
Buddhas and predestined people
8. Go future thinking
Many concepts of Go are very common, and the implementation is also very selective. I didn't expect that in the end, it was so practical to develop engineering leverage..
In my opinion, one of the highlights is to serve the engineers in actual production, and then the machines, so as to reasonably solve their pain points.
Thinking about the future of Go, I'm looking forward to the arrival of generic pattern.