Template syntax
Text:
<span>Message: {{ msg }}</span>
v-once
One-time interpolation, when the data changes, the contents of the interpolation will not be updated
<span v-once>This will not change: {{MSG}}</span>
v-html directive
<p>Using mustaches: {{ rawHtml }}</p> <p>Using v-html directive: <span v-html="rawHtml"></span></p>
<div v-bind:id="dynamicId"></div>
{{ number + 1 }} {{ ok ? 'YES' : 'NO' }} {{ message.split('').reverse().join('') }} <div v-bind:id="'list-' + id"></div>
<p v-if="see">Now you see me </p>
The v-if directive inserts/removes elements based on the true or false value of the expression seed
<a v-bind:href="url">...</a>
Deeply Understanding Semanticization
Web semanticization refers to HTML tags, Class class names, etc. that use appropriate semantics
HTML provides context structure and meaning for the content of Web documents
CSS semantics is the semantics of class and ID naming
The Class attribute serves as a link between HTML and CSS and is intended to describe the content of an element
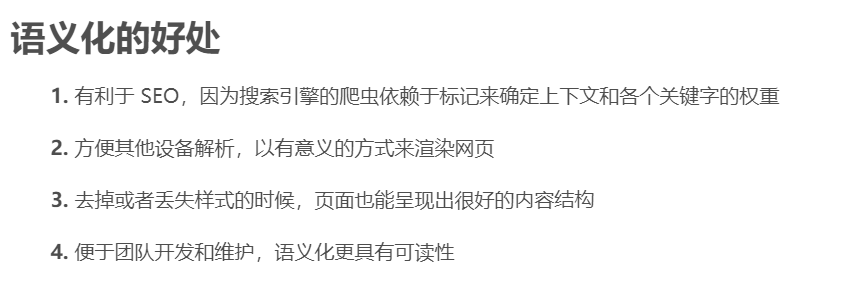
html semantics is about structuring the content of a page
Web semantics refers to the use of appropriate semantics such as html tags, class class names, and so on, so that the page has a good structure and meaning, so that people and machines can quickly understand the content of the page
html semantics and css naming semantics
Favor SEO, Read and Maintain Understanding
Web Standards - Separation of Structure, Style, and Behavior
Structure, Style, and Behavior
The structure part mainly includes XML standard, XHTML standard
Style standards refer primarily to CSS standards
Behavior standards mainly include DOM and ECMAScript standards
HTML: Hypertext Markup Language responsible for the structure of web pages
Typically, semantically HTML makes code smaller and pages load faster
Semantic HTML clarifies the HTML structure and helps maintain code and add styles
Enhance Web page accessibility and interoperability
Improve the effectiveness of search engine optimization (SEO)
v-on directive, which listens for DOM events
<!-- Complete Grammar --> <a v-bind:href="url">...</a> <!-- Abbreviation --> <a :href="url">...</a> <!-- Complete Grammar --> <a v-on:click="doSomething">...</a> <!-- Abbreviation --> <a @click="doSomething">...</a>
Compute properties and listeners
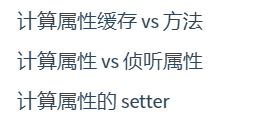
<div id="example"> <p>Original message: "{{ message }}"</p> <p>Computed reversed message: "{{ reversedMessage }}"</p> </div> var vm = new Vue({ el: '#example', data: { message: 'Hello' }, computed: { // getter for calculating attributes reversedMessage: function () { // `this`points to a vm instance return this.message.split('').reverse().join('') } } }) console.log(vm.reversedMessage) // => 'olleH' vm.message = 'Goodbye' console.log(vm.reversedMessage) // => 'eybdooG'
Calculate attribute caches, methods, compute attributes, listen attributes, compute setter s for attributes
<p>Reversed message: "{{ reversedMessage() }}"</p> // In Components methods: { reversedMessage: function () { return this.message.split('').reverse().join('') } }
Calculated properties are cached based on their responsive dependencies
They are reevaluated only when the relevant responsive dependencies change
As long as the message has not changed
Multiple access to a computed property immediately returns the previous computed result without having to execute the function again
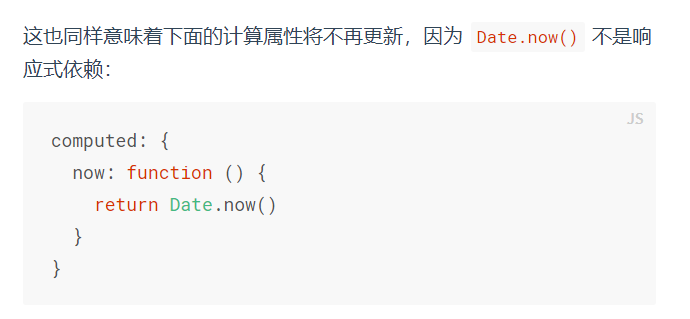
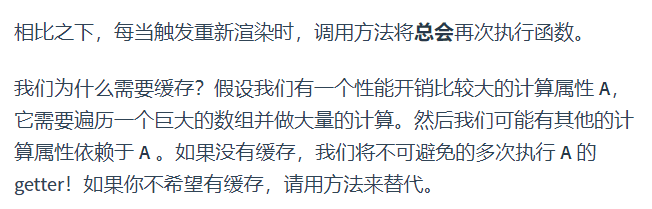
Listening Properties
<div id="demo">{{ fullName }}</div> var vm = new Vue({ el: '#demo', data: { firstName: 'Foo', lastName: 'Bar', fullName: 'Foo Bar' }, watch: { firstName: function (val) { this.fullName = val + ' ' + this.lastName }, lastName: function (val) { this.fullName = this.firstName + ' ' + val } } }) var vm = new Vue({ el: '#demo', data: { firstName: 'Foo', lastName: 'Bar' }, computed: { fullName: function () { return this.firstName + ' ' + this.lastName } } })
// ... computed: { fullName: { // getter get: function () { return this.firstName + ' ' + this.lastName }, // setter set: function (newValue) { var names = newValue.split(' ') this.firstName = names[0] this.lastName = names[names.length - 1] } } } // ...

This is most useful when you need to perform asynchronous or expensive operations when data changes.
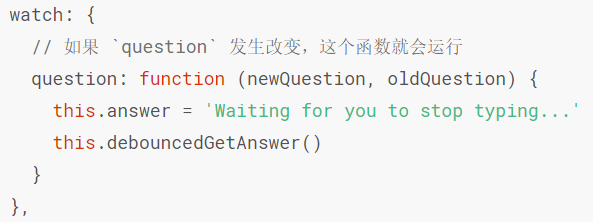
Please compliment!Because your encouragement is my greatest motivation to write!
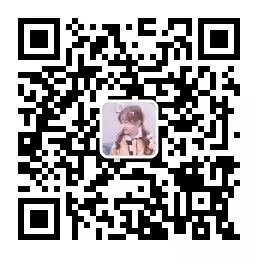
Blow the crowd: 711613774
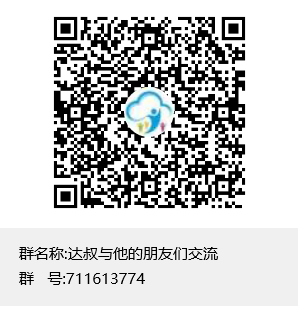