Scala variables and data types
First of all, the basic data type of Scala is the same as that of Java
notes
Scala annotations are used exactly like Java
Annotation is a good programming habit that a programmer must have. First sort out your thoughts through comments, and then reflect them in code.
Basic language method
- Single line note://
- Multiline comment:/**/
- Document comments: / * * **/
/** * Document notes: * * Program entry method * @param args Externally passed in parameters * @author laingzai * @version 1.0 * //Single-Line Comments * /* * multiline comment * */ */ object Test01_Comment { def main(args: Array[String]): Unit = { //Single line note printout //println("hello Scala") /*Multiline annotation multiline output println("hello Scala") println("hello Scala") println("hello Scala") println("hello Scala") println("hello Scala") println("hello Scala") */ println("hello Scala") } }
Code specification
- Use a tab operation to indent. By default, the whole moves to the right and shift + tab moves to the left
- Or use ctrl + alt + L to format
- Operator is habitually added with a space on both sides. For example: 2 + 4 * 5
- The maximum length of a line shall not exceed 80 characters. If it exceeds, please use newline display to keep the format elegant as far as possible
variable and constant
Constant: a variable whose value will not be changed during program execution
- Java variable and constant syntax
- Variable type variable name = initial value int a = 10
- Final constant type constant name = initial value final int b = 20
- Scala basic syntax
- Var variable name [: variable type] = initial value var i:Int = 10
- val constant name [: constant type] = initial value val j:Int = 20
Note: variables are not needed where constants can be used Java Type before name Scala Write the name first, then the type, and use it in the middle:separate Scala If you can use constants, use constants as much as possible and variables as little as possible (facing object language and functional language). What a value is equal to is what it is
- case
1,When declaring variables, the type can be omitted and automatically derived after compilation, that is, type derivation 2,After the type is determined, it cannot be modified. Description Scala Strong data type language 3,Variable declaration must have an initial value 4,In the statement/When defining a variable, you can use var perhaps val To decorate, var The modified variable can be changed, val Modified variables cannot be modified
package day02 import day01.Student object Test02_Variable { def main(args: Array[String]): Unit = { //Syntax for declaring a variable var a: Int = 10 // 1. When declaring variables, the type can be omitted and automatically derived after compilation, that is, type derivation var a1 = 10 val b1 = 24 // 2. After the type is determined, it cannot be modified, which indicates that Scala is a strong data type language var a2 = 15 //a2 type is Int // a2 = "hello" // 3. Variable declaration must have an initial value // var a3: Int // 4. When declaring / defining a variable, you can use var or val to modify it. The variable modified by var can be changed, and the variable modified by val cannot be changed a1 = 18 // b1=24 var liangzai = new Student("liangzai", 24) liangzai = new Student("Liangzai", 25) liangzai = null val diaomao = new Student("diaomao", 21) diaomao.age = 29 diaomao.printerInfo() } }
Operation results: diaomao 29 zhongguo
If you want to modify the value, you can add val or var before the declaration
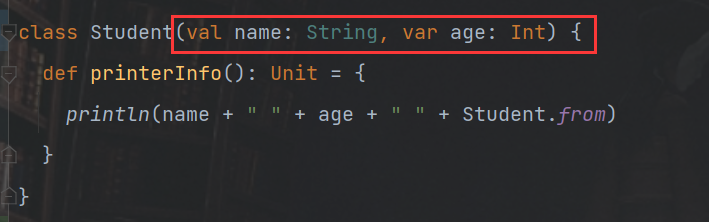
Naming conventions for identifiers
The character sequence used by Scala in naming various variables, methods and functions is called identifier. That is: all places where you can name yourself are called identifiers.
- Naming rules
Scala Identifier declaration in, basic and Java Yes, but the details will change. There are three rules: 1,Begin with a letter or underscore followed by letters, numbers, and underscores 2,Starts with an operator and contains only operators(+ - * / # ! Etc.) 3,Use reverse quotation marks`....`Including any string, even Scala keyword(39 individual)it's fine too //package,import,class,object,trait,extends,with,type,for //private,protected,abstract,sealed,final,implicit,lazy,override //try,catch,finally,throw //if,else,match,case,do,while,for,return,yield //def,val,var //this,super //new //true,false,null
- case
package day02 object Test03_Identifier { def main(args: Array[String]): Unit = { // 1. Begin with a letter or underscore followed by letters, numbers, and underscores val hello: String = "" var Hello123 = "" val _abc = 123 // val h-b = "" / / syntax error. No error is reported in IDEA // val 123abc = 234 // 2. Starts with an operator and contains only operators (+ - * / #! Etc.) val -+/% = "hello" println(-+/%) // 3. Use back quotation marks` Any string included, even Scala keywords (39) can be used // val if = "" val `if` = "if" println(`if`) } }
Output result: hello if
String output
- Basic grammar
1,String, by + Number connection 2,printf Usage: string, through%Value transmission 3,String template (interpolated string): Pass $Get variable value
- case
package day02 object Test04_String { def main(args: Array[String]): Unit = { // 1. String, connected by + sign val name: String = "liangzai" val age: Int = 24 println(age + "Year old" + name + "Staying up late blogging") // *Used to copy and splice a string multiple times println(name * 3) // 2. printf usage: string, passing value through% printf("%d Year old%s Staying up late blogging\n",age,name) // 3. String template (interpolation string): get variable value through $ println(s"${age}Year old ${name}Staying up late blogging") val num:Float = 3.1415F val num1:Double = 2.3456 println(f"The num is ${num}") println(f"The num1 is ${num1}%2.2f") //Format template string println(raw"The num1 is ${num1}%2.2f") //Output as is // Three quotation marks represent the string and keep the original format output of multi line string val sql = s""" |select * |from | student |where | name = ${name} |and | age > ${age} |""".stripMargin println(sql) } }
Output result: 24 Year old liangzai Staying up late blogging liangzailiangzailiangzai 24 Year old liangzai Staying up late blogging 24 Year old liangzai Staying up late blogging The num is 3.1415 The num1 is 2.35 The num1 is 2.3456%2.2f select * from student where name = liangzai and age > 24
keyboard entry
In programming, if you need to receive the data entered by the user, you can use the keyboard to input statements to obtain it.
- Basic grammar
StdIn.readLine()
StdIn.readShort()
StdIn.readDouble()
- case
Requirement: user information can be received from the console, [name, age, salary]
package day02 import scala.io.StdIn object Test05_StdIn { def main(args: Array[String]): Unit = { // Input information println("Please enter your name:") val name : String = StdIn.readLine() println("Please enter your age:") val age: Int = StdIn.readInt() // Console printout println(s"young ${age}Year old ${name}Staying up late blogging") } }
Output result: Please enter your name: handsome young man Please enter your age: 24 The pretty 24-year-old is staying up late to write a blog
File operation read and write
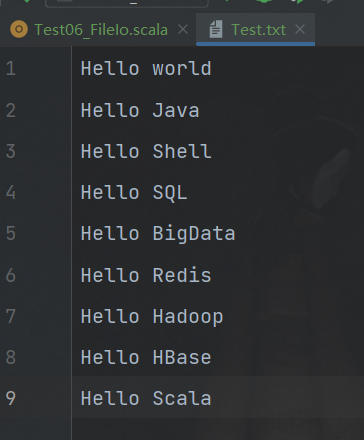
package day02 import java.io.{File, PrintWriter} import scala.io.Source object Test06_FileIo { def main(args: Array[String]): Unit = { //1. Read data from file Source.fromFile("src/main/resources/Test.txt").foreach(print) // 2. Write data to file val writer = new PrintWriter(new File("src/main/resources/Put.txt")) writer.write("Learn Hello Scala day day up!") writer.close() } }
Output result: Hello world Hello Java Hello Shell Hello SQL Hello BigData Hello Redis Hello Hadoop Hello HBase Hello Scala
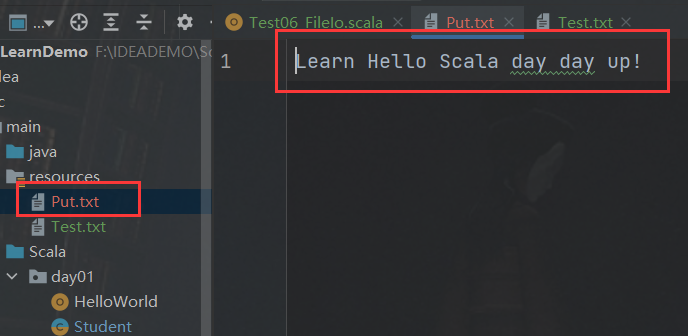
data type
Java data type
Java Basic type: char,byte,short,int,long,float,double,Boolean Java Reference type: (object type) because Java There are basic types, but the basic type is not a real object. Even if the wrapper class of the basic type is generated later, there are still basic data types, so Java Language is not really object-oriented. Java Packing class of basic type: Character,Byte,Short,Integer,Long,Float,Double,Boolean be careful: Java The base type and reference type in do not have a common parent class
Scala data type
1,Scala All data in is an object and is Any Subclass of 2,Scala Data types in are divided into two categories: numeric types( AnyVal),Reference type( AnyRef),Both value type and reference type are objects. 3,Scala Data types are still followed, and low precision value types are automatically converted to high-precision value types (implicit conversion) 4,Scala Medium StringOps Yes Java in String enhance 5,Unit:corresponding Java Medium void,The position used for the return value of the method, indicating that the method has no return value. Unit Is a data type. There is only one object(). Void Not a data type, just a keyword 6,Null Is a type with only one object null. It is all reference types( AnyRef)Subclass of.
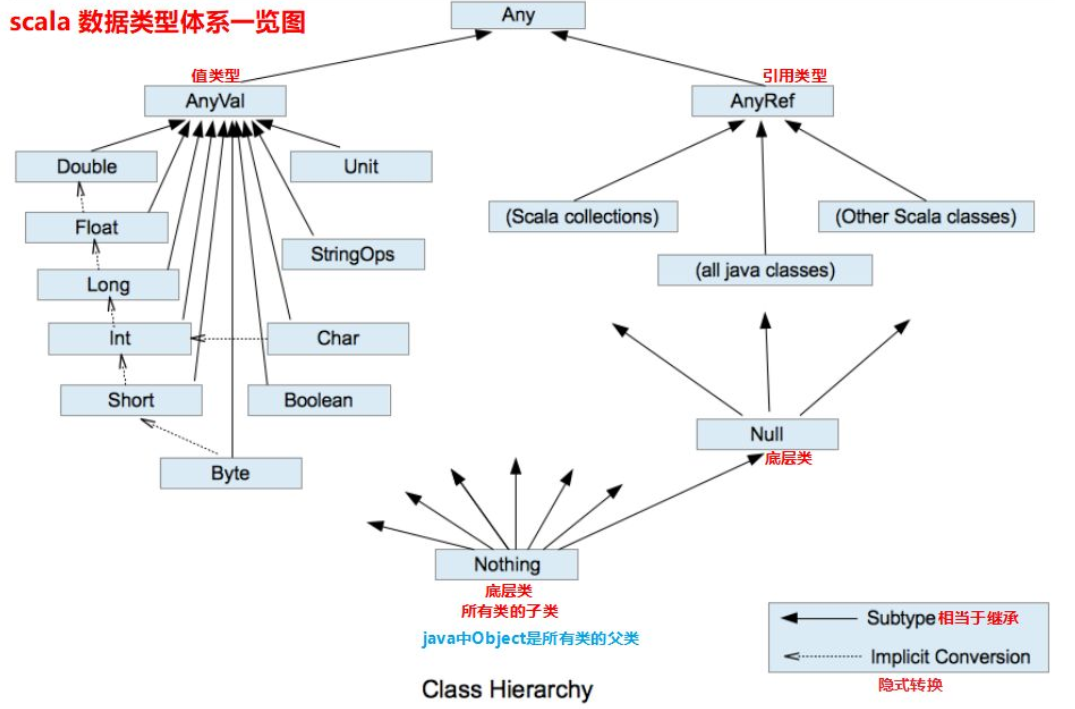
Integer type (Byte, Short, Int, Long)
Scala's integer type is used to store integer values, such as 18, 243456 and so on.
- Integer classification
data type | describe |
---|---|
Byte[1] | 8-bit signed complement integer. The range of values is - 128 to 127 |
Short[2] | 16 bit signed complement integer. The range of values is - 32768 to 32767 |
Int[4] | 32-bit signed complement integer. The range of values is - 2147483648 to 2147483647 |
Long[8] | 64 bit signed complement integer. The value range is - 9223372036854775808 to 9223372036854775807 = 264-1 |
- case
package day02 object Test07_DataType { def main(args: Array[String]): Unit = { // 1. Integer type val a1: Byte = 127 val a2: Byte = -128 // val a2: Byte = 128 //error out of type range val a3 = 18 //The default type of integer is Int // val a4 = 12345678923456 //error out of type range val a4 = 12345678923456L //Long integer value definition val b1: Byte = 10 // Val B2: byte = 10 + 20 / /: int does not report an error when running here, but it cannot be written like this val b2 = 10 + 20 //The default is Int type println(b2) // val b3: Byte = (b1 + 20) / / compilation fails here val b3 = (b1 + 20).toByte println(b3) // 2. Floating point type val f1: Float = 3.14F //Need to add F, not recommended f (Development Specification) val d1 = 3.1415 //Default double type } }
Character type (Char)
- 1. Basic description
The character type can represent a single character, and the character type is Char
- 2. Case
- A character constant is a single character enclosed in single quotation marks' '
- \t: A tab stop to realize the alignment function
- \n: newline character
- \: indicates\
- ": indicates"
Boolean type: Boolean
- Basic description
- Boolean type is also called boolean type. Boolean type can only take values of true and false
- Boolean type takes up 1 byte
package day02 object Test07_DataType { def main(args: Array[String]): Unit = { // 1. Integer type val a1: Byte = 127 val a2: Byte = -128 // val a2: Byte = 128 //error out of type range val a3 = 18 //The default type of integer is Int // val a4 = 12345678923456 //error out of type range val a4 = 12345678923456L //Long integer value definition val b1: Byte = 10 // Val B2: byte = 10 + 20 / /: int does not report an error when running here, but it cannot be written like this val b2 = 10 + 20 //The default is Int type println(b2) // val b3: Byte = (b1 + 20) / / compilation fails here val b3 = (b1 + 20).toByte println(b3) // 2. Floating point type val f1: Float = 3.14F //Need to add F, not recommended f (Development Specification) val d1 = 3.1415 //Default double type // 3. Character type val c1: Char = 'a' println(c1) val c2: Char = '9' //Character 9 println(c2) //Special characters val c3: Char = '\t' //Tab val c4: Char = '\n' //Line feed println("abc" + c3 + "def") println("abc" + c4 + "def") // Escape character val c5 = '\\' //Represents \ itself val c6 = '\"' //Express“ println("abc" + c5 + "def") println("abc" + c6 + "def") //The ASCL code is saved at the bottom of the character variable val i1: Int = c1 println("i1:" + i1) val i2: Int = c2 println("i2:" + i2) val c7: Char = (i1 + 1).toChar println(c7) val c8: Char = (i2 - 1).toChar println(c8) // 4. Boolean type val isTrue: Boolean = true println(isTrue) } }
Output result: 30 30 a 9 abc def abc def abc\def abc"def i1:97 i2:57 b 8 true
Unit type, Null type and Nothing type
- Basic description
data type | describe |
---|---|
Unit | Indicates no value, which is equivalent to void in other languages. The result type used as a method that does not return any results. Unit has only one instance value, written as () |
Null | null,Null type has only one instance value null |
Nothing | Nothing type is at the lowest level of Scala's class hierarchy; It is a subtype of any other type. When a function is determined to have no normal return value, we can use nothing to specify the return type. This has the advantage that we can assign the returned value (exception) to other functions or variables (compatibility) |
- case
package day02 import day01.Student object Test07_DataType { def main(args: Array[String]): Unit = { // 5. Empty type // 5.1. Null value Unit def m1(): Unit = { println("m1 Called execution") } val a = m1() println("a:" + a) // 5.2. Null reference // val n:Int = null //error a value cannot receive null references var student = new Student("laingzai", 24) student = null //reference type println(student) //5.3,Nothing // def m2(n: Int): Nothing = { // throw new NullPointerException // } def m2(n: Int): Int = { if (n == 0) throw new NullPointerException else return n } //Exception thrown. The following printing is not executed val b: Int = m2(2) println("b:" + b) } }
Operation results: m1 Called execution a:() null b:2
Original code
final abstract class Unit private extends AnyVal { // Provide a more specific return type for Scaladoc override def getClass(): Class[Unit] = ??? } object Unit extends AnyValCompanion { /** Transform a value type into a boxed reference type. * * @param x the Unit to be boxed * @return a scala.runtime.BoxedUnit offering `x` as its underlying value. */ def box(x: Unit): scala.runtime.BoxedUnit = scala.runtime.BoxedUnit.UNIT /** Transform a boxed type into a value type. Note that this * method is not typesafe: it accepts any Object, but will throw * an exception if the argument is not a scala.runtime.BoxedUnit. * * @param x the scala.runtime.BoxedUnit to be unboxed. * @throws ClassCastException if the argument is not a scala.runtime.BoxedUnit * @return the Unit value () */ def unbox(x: java.lang.Object): Unit = x.asInstanceOf[scala.runtime.BoxedUnit] /** The String representation of the scala.Unit companion object. */ override def toString = "object scala.Unit" }
UNIT original code
package scala.runtime; public final class BoxedUnit implements java.io.Serializable { private static final long serialVersionUID = 8405543498931817370L; public final static BoxedUnit UNIT = new BoxedUnit(); public final static Class<Void> TYPE = java.lang.Void.TYPE; private Object readResolve() { return UNIT; } private BoxedUnit() { } public boolean equals(java.lang.Object other) { return this == other; } public int hashCode() { return 0; } public String toString() { return "()"; } }
Type conversion
Java interview question (implicit type conversion):
public class TestDataTypeConversion { public static void main(String[] args) { //Automatic type conversion principle byte b = 10; test(b); char c = 'a'; test(c); short c2 = (short) c; test(c2); } public static void test(byte b) { System.out.println("bbbb"); } public static void test(short s) { System.out.println("sssss"); } public static void test(char c) { System.out.println("cccc"); } public static void test(int i) { System.out.println("iiii"); } }
Output result: bbbb cccc sssss
Scala value type automatic conversion
When Scala program is performing assignment or operation, the type with low precision is automatically converted to the numerical type with high precision. This is automatic type conversion (implicit conversion). The data types are sorted by precision (capacity):
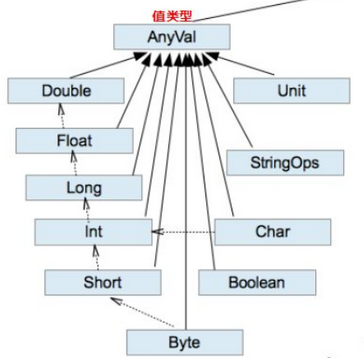
- Basic description
1,Automatic promotion principle: when there are multiple types of data mixed operation, the system will first automatically convert all data into the data type with high precision, and then calculate 2,When assigning a value type with high precision to a value type with low precision, an error will be reported. Otherwise, automatic type conversion will be carried out 3,(byte,short) and char There will be no automatic conversion between them 4,byte,short ,char The three of them can be calculated and converted into int type
- case
package day02 object Test08_DataTypeConversion { def main(args: Array[String]): Unit = { // 1. Automatic promotion principle: when there are multiple types of data mixed operation, the system will first automatically convert all data into the data type with high precision, and then calculate val a1: Byte = 10 val b1: Long = 2353 val result1: Long = a1 + b1 val result11: Int = (a1+b1).toInt //Cast type // 2. When assigning a value type with high precision to a value type with low precision, an error will be reported. Otherwise, automatic type conversion will be carried out val a2: Byte = 10 val b2: Int = a2 // val c2:Byte = b2 //error val c2: Byte = b2.toByte // 3. (byte,short) and char are not automatically converted to each other val a3: Byte = 10 val b3: Char = 'b' // val c3: Byte = b3 //error val c3: Int = b3 println(3) // 4. Byte, short and char can be calculated. When calculating, they are first converted to int type val a4: Byte = 18 val b4: Short = 24 val c4: Char = 'c' // val result4: Short = (a4 + b4) val result4: Int = a4 + b4 val result44: Int = a4 + b4 + c4 println(result4) println(result44) } }
Operation results: 3 42 141
Cast type
- Basic description
The inverse process of automatic type conversion converts the array type with high precision to the numerical type with low precision. The forced conversion function should be added when using, but it may reduce the accuracy or overflow, so we should pay special attention
Java : int num = (int)2.5
Scala : var num: Int = 2.5.toInt
- case
1,To convert data from high precision to low precision, you need to use forced conversion 2,The strong conversion symbol is only valid for the most recent operand, and parentheses are often used to raise the priority
package day02 object Test08_DataTypeConversion { def main(args: Array[String]): Unit = { // 2. Cast type // 1. To convert data from high precision to low precision, you need to use forced conversion val n1: Int = 2.5.toInt println("n1:" + n1) val n2: Int = -2.8.toInt println("n2:" + n2) // 2. The strong conversion symbol is only valid for the most recent operand, and parentheses are often used to raise the priority val n3: Int = 2.6.toInt + 3.7.toInt //This will result in the loss of numerical accuracy val n4: Int = (2.6 + 3.7).toInt println("n3:" + n3) println("n4:" + n4) // 3. Conversion of numeric type and String type // (1) Value to String val n: Int = 27 // val s:String = n.toString val s: String = n + "" println(s) // (2) String to value val m: Int = "12".toInt val f: Float = "12.3".toFloat val f2: Int = "12.3".toDouble.toInt println(m) println(f) println(f2) } }
Operation results: n1:2 n2:-2 n3:5 n4:6 27 12 12.3 12
Scala interview questions
package day02 /* 128 : Int Type, 4 bytes, 32 bits Original code: 0000 0000 0000 1000 0000 Complement: 0000 0000 0000 1000 0000 Intercept the last Byte, Byte Get complement 10 million Indicates the maximum negative number - 128 130 : Int Type, 4 bytes, 32 bits Original code: 0000 1000 0010 Complement: 0000 1000 0010 Intercept the last Byte, Byte Get complement 1000 0010 Corresponding to original code 1111 1110 -126 */ object Test09_DataTypeConversion { def main(args: Array[String]): Unit = { val n: Int = 128 val b: Byte = n.toByte println(b) val n1: Int = 130 val b1: Byte = n1.toByte println(b1) } }
Operation results: -128 -126
In the end! Give the pretty boy a three company! ༼ つ ◕_ ◕ ༽つ