Your problem lies mainly in not reading much but thinking too much.- Yang Jiang
That's right. I must read more!!!
Head->1->2>1-->3->3->5->7->6>7->8, delete the duplicates and make them head->1->2->5->7->6->8.
Today's title is the same as yesterday's. Yesterday we successfully completed this task using the sequential deletion method.However, it uses a double loop to traverse the chain table with a time complexity of O(N^2).Usually, in order to reduce the time complexity, auxiliary space is used when conditions allow it.Specifically as follows:
The Title requires the removal of duplicates, and it is easy to think of Python's own data type, the collection set.Sets are out of order, unique, and deterministic.Disorderliness: Elements in a collection are in the same position and have no order.Uniqueness: Also called anisotropy, set elements are different from each other and each element can only appear once.Certainty: Given a set, assign an element that either belongs to or does not belong to the set. Both must be in one and ambiguity is not allowed.
We can solve this problem by taking advantage of the uniqueness of the set -- whether the current node has recurred or not.Let's first set up a HashSet collection that stores the contents of nodes that have been traversed.Initialize it to null first.Then iterate through the list from scratch and determine if the content of the current node is in the HashSet.If it is, the current node is deleted; if it is not, the current node is retained, and the contents of the current node are added to the HashSet, traversing the next node until the list is empty.
The time complexity of this method is O(n), since only one iteration of the list is required.The following is implemented in code:
def RemoveDup(head): """ //Space Change Time //Headless Node """ #First determine if the list is empty or not if head.next is None: return head Hashset=set()#Save traversed content Hashset.clear()#Initialize Collection pre=head#A precursor node to the current node, used to delete the current node cur=head.next#Used to traverse the list of chains, pointing to the current node #Traversing a list of chains while cur is not None: #If the value of the current node is not in the HashSet, save it in the HashSet if cur.data not in Hashset: Hashset.add(cur.data) #Traverse Next Node cur=cur.next pre=pre.next else: #If the value of the current node is in the HashSet, delete the node pre.next=cur.next cur=cur.next #Returns the list of chains processed return head
As for how to delete the current node, I made it clear in my article yesterday that I would not go into any further details here: python algorithm-005 removes duplicates from an unordered list (sequential deletion).
As before, we construct an unordered list (as we did yesterday, you can see the previous links) to test our algorithm:
#Introducing random library first import random #Still Define Node Class First class LNode(object): def __init__(self, arg): self.data = arg self.next = None #Here! I made a mistake in the first few create s, with a missing e. #What this means by using construct instead def construstLink(x): i = 1 head = LNode(None) tmp = None cur = head while i <= x: #Unlike before, Mr. A generates a random number as the node value n = random.randint(0, 9) tmp = LNode(n) cur.next = tmp cur = tmp i += 1 return head
Main program:
if __name__ == '__main__': #Construct a list of chains head=construstLink(10) #Chain list before printing print("BeforeReverse:") cur = head.next while cur != None: print(cur.data) cur = cur.next #Call the algorithm to process the list of chains head = RemoveDup(head) # Chain list after printing print("\nAfterReverse:") cur = head.next while cur != None: print(cur.data) cur = cur.next
Here are the results:
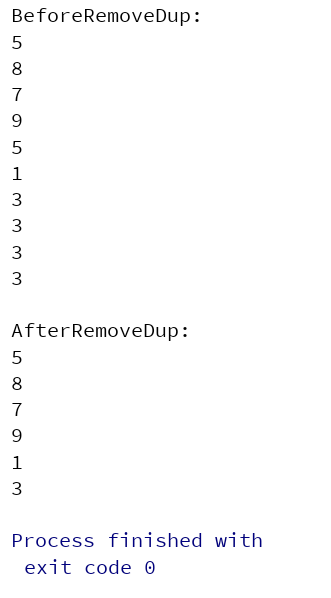
You can try it a few more times too, and I think the algorithm is correct.
This is all the code:
import random class LNode(object): """docstring for LNode""" def __init__(self, arg): self.data = arg self.next = None #Here! I made a mistake in the first few create s, with a missing e. #What this means by using construct instead def construstLink(x): i = 1 head = LNode(None) tmp = None cur = head while i <= x: #Unlike before, Mr. A generates a random number as the node value n = random.randint(0, 9) tmp = LNode(n) cur.next = tmp cur = tmp i += 1 return head """ //Title Description: //Change Head->1->1->3->3->5->7->7->8 //For head->1->2->5->7->8 //Method: Change time by space, utilizing the disorder and uniqueness of sets """ def RemoveDup(head): """ //Space Change Time //Headless Node """ #First determine if the list is empty or not if head.next is None: return head Hashset=set()#Save traversed content Hashset.clear()#Initialize Collection pre=head#A precursor node to the current node, used to delete the current node cur=head.next#Used to traverse the list of chains, pointing to the current node #Traversing a list of chains while cur is not None: #If the value of the current node is not in the HashSet, save it in the HashSet if cur.data not in Hashset: Hashset.add(cur.data) #Traverse Next Node cur=cur.next pre=pre.next else: #If the value of the current node is in the HashSet, delete the node pre.next=cur.next cur=cur.next #Returns the list of chains processed return head if __name__ == '__main__': #Construct a list of chains head=construstLink(10) #Chain list before printing print("BeforeRemoveDup:") cur = head.next while cur != None: print(cur.data) cur = cur.next #Call the algorithm to process the list of chains head = RemoveDup(head) # Chain list after printing print("\nAfterRemoveDup:") cur = head.next while cur != None: print(cur.data) cur = cur.next
Today's algorithm is like that. Tomorrow we will talk about how to use recursion to do this. You can try it first.I am also in the learning stage, there are still many problems, I hope you can give us your advice.I write this kind of articles, one is to better understand what I am learning, after all I can tell it, which is also very good; the other is to share my learning results with you, to learn and progress together, to reach the peak of life together (Ha-ha, of course optical, this is not even possible to find work); the other is: there is one thing you can persist inAlso very happy!
Of course, you can come to me if you need anything. Short Book Number, WeChat Public Number: Dkider Private Trust, Simple Trust, you can find me.
See more questions and article sources github.
It is human vanity to let people know that they have a secret, but not what it is.Qian Zhongshu's Besieged City