Preface: A path can contain one or more shapes and subpaths. Quatz provides many convenient shapes that can be called directly. For example: point, line, Arc (arc), Curves (curve), Ellipse (ellipse), Rectangle.
These path s can be stroke or fill. They can also be used to intercept an area (clip).
For example, use a truncated circular area
If the basic concept of Quartz is still unclear, I strongly recommend that you read my previous article, otherwise you can't understand it.
Overview of iOS 2D Drawing Details (Quartz 2D)
Points/Lines
In Quartz, you use CGContextMoveToPoint to move the brush to a point to start a new subpath, and CGContextAddLineToPoint to add a line from the current starting point to the end point. Note that after the CGContextAddLineToPoint call, the start point is reset, which knows the end point.
For example,
Code
- (void)drawRect:(CGRect)rect {
CGContextRef context = UIGraphicsGetCurrentContext(); //Get the current context
//Setting colors
CGContextSetFillColorWithColor(context, [UIColor whiteColor].CGColor);
CGContextSetStrokeColorWithColor(context, [UIColor lightGrayColor].CGColor);
//To better differentiate colors, draw rectangular edges
CGContextFillRect(context, rect);
CGContextStrokeRect(context, rect);
//Code for actual line and point
CGContextSetStrokeColorWithColor(context, [UIColor redColor].CGColor);// Setting the edge color
CGContextSetLineWidth(context, 4.0);//Width of line
CGContextSetLineCap(context, kCGLineCapRound);//The top of the line
CGContextSetLineJoin(context, kCGLineJoinRound);//Line intersection mode
CGContextMoveToPoint(context,10,10);
CGContextAddLineToPoint(context, 40, 40);
CGContextAddLineToPoint(context, 10, 80);
CGContextStrokePath(context);
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
The top mode of the line is mentioned here, which is set using CGContextSetLineCap. There are three types.
The intersection mode of lines is set up by CGContextSetLineJoin. There are also three types.
Dashed line
Effectiveness
Code
CGContextSetStrokeColorWithColor(context, [UIColor redColor].CGColor);
CGContextSetLineWidth(context, 1.0);
CGContextSetLineCap(context, kCGLineCapRound);
CGContextSetLineJoin(context, kCGLineJoinRound);
CGFloat lengths[] = {2};
CGContextSetLineDash(context, 1, lengths, 1);
CGContextMoveToPoint(context,10,10);
CGContextAddLineToPoint(context, 40, 40);
CGContextAddLineToPoint(context, 10, 80);
CGContextStrokePath(context);
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
Detailed explanations of the CGContextSetLineDash parameter using the method
void CGContextSetLineDash (
CGContextRef _Nullable c,
CGFloat phase,
const CGFloat * _Nullable lengths,
size_t count
);
- 1
- 2
- 3
- 4
- 5
- 6
- 1
- 2
- 3
- 4
- 5
- 6
- c Drawing context, this needn't be said much.
- phase, where does the first dashed segment start, for example, in the third unit
- lengths, a C array, represents the allocation of drawing and blank parts. For example, in [2,2], two units are drawn and then two units are blank to repeat.
- Number of count lengths
Arc
Quartz provides two ways to draw arcs
- CGContextAddArc, a part of an ordinary arc (an arc with a center, a radius, an arc of a certain arc)
- CGContextAddArcToPoint, which is used to draw rounded corners, is described in detail below.
CGContextAddArc parameter
void CGContextAddArc (
CGContextRef _Nullable c,
CGFloat x,
CGFloat y,
CGFloat radius,
CGFloat startAngle,
CGFloat endAngle,
int clockwise
);
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- c. context s don't need to be chopped
- x,y specifies the origin of coordinates
- Radius, specified radius length
- startAngle/endAngle, specifying a certain radian
- Clockwise, 1 is clockwise, 0 is counterclockwise.
For example
This function is very simple, no graphics.
CGContextAddArc(context,50,50, 25,M_PI_2,M_PI,1);
CGContextStrokePath(context);
- 1
- 2
- 1
- 2
CGContextAddArcToPoint is a complex method, but it's not that difficult to understand.
Function Body
void CGContextAddArcToPoint (
CGContextRef _Nullable c,
CGFloat x1,
CGFloat y1,
CGFloat x2,
CGFloat y2,
CGFloat radius
);
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
parameter
- c context
- x1,y1 and the current point (x0,y0) determine the first tangent (x0,y0) - > (x1,y1)
- x2,y2 and (x1,y1) determine the second tangent
- Radius, the radius of the cut.
That is to say,
Draw an arc radius, tangent to both lines.
Code
CGContextMoveToPoint(context, 100, 50);
CGContextAddArcToPoint(context,100,0,50,0, 50);
CGContextStrokePath(context);
- 1
- 2
- 3
- 1
- 2
- 3
Effectiveness
Explain why.
The two red lines in the graph are the two lines mentioned above, namely (x0, y0) - > (x1, y1) and (x1, y1) - > (x2, y2). Then the nature that both lines want to cut is the blue arc in the graph.
Ellipse/rectangle
It's relatively simple. I won't go into any more details here.
Example
CGContextSetStrokeColorWithColor(context, [UIColor blueColor].CGColor);
CGContextAddEllipseInRect(context, CGRectMake(10, 10,40, 20));
CGContextAddRect(context, CGRectMake(10, 10,40, 20));
CGContextStrokePath(context);
- 1
- 2
- 3
- 4
- 1
- 2
- 3
- 4
Effectiveness
curve
Quartz uses polynomials in computer graphics to draw curves, supporting quadratic and cubic curves.
Using the function CGContextAddCurveToPoint, the cubic curve can be drawn.
Function Body
void CGContextAddCurveToPoint (
CGContextRef _Nullable c,
CGFloat cp1x,
CGFloat cp1y,
CGFloat cp2x,
CGFloat cp2y,
CGFloat x,
CGFloat y
);
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
parameter
- c context
- Cp1x, the first control point of cp1y
- Cp2x, the second control point of cp2y
- x,y endpoint
The effect is shown in the figure.
Use CGContextAddQuadCurve ToPoint to draw quadratic curves.
Function body,
void CGContextAddQuadCurveToPoint (
CGContextRef _Nullable c,
CGFloat cpx,
CGFloat cpy,
CGFloat x,
CGFloat y
);
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 1
- 2
- 3
- 4
- 5
- 6
- 7
parameter
- c context
- cpx,cpy control point
- x,y endpoint
- Effectiveness
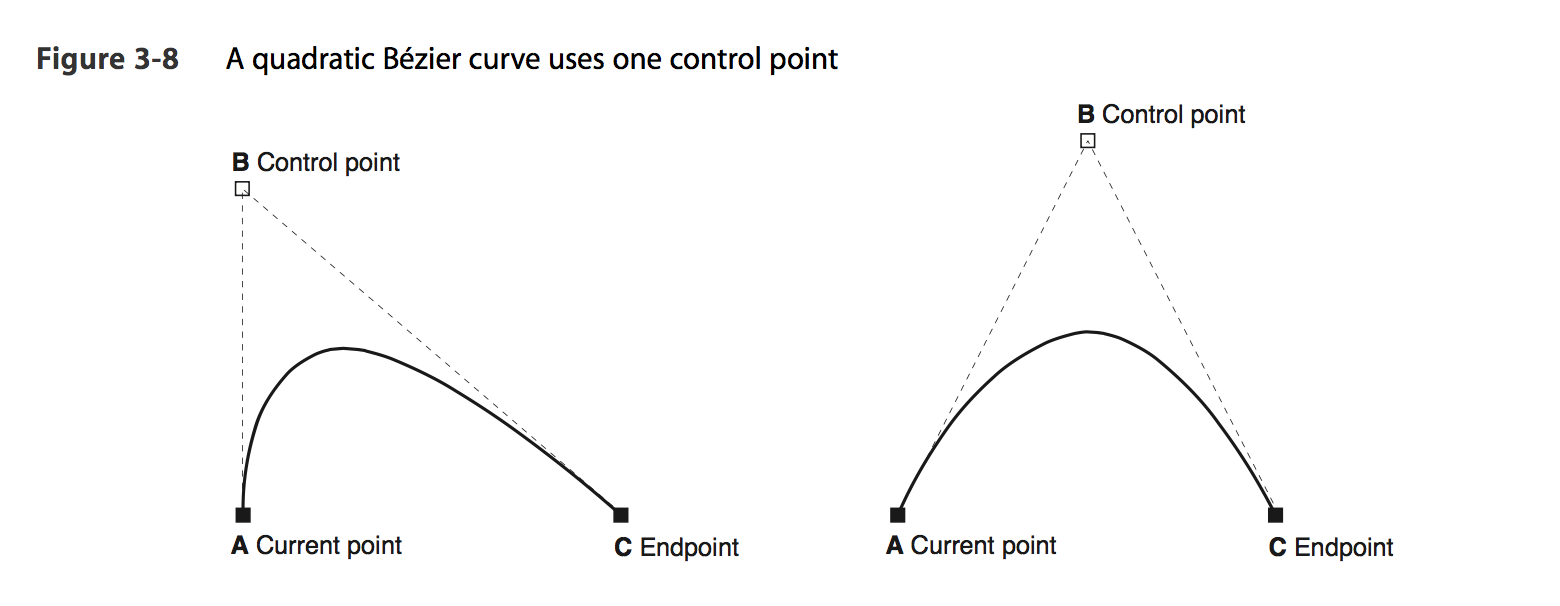