Write in the front: if...else nesting, multiple options (Switch statement), for loop, while loop and do...while, break, continue knowledge points related introduction and demo summary. It's been five or six days since we finished this part of Mucho.com. Many of them are not proficient enough, and then the demo and related introductions are summarized. It is also convenient to check them up later.
Make judgments (if statement)
The if statement is used when executing the corresponding code based on the condition.
Grammar:
If (condition) {Execution code when condition is established}
Note: If lowercase, capital letter (IF) will be wrong! Can not write else!
Choose one of two (if...else statement)
The if...else statement executes the code when the specified condition is established, and the code after the else is executed when the condition is not established.
Grammar:
if(condition)
{ Code executed when conditions are established}
else
{Code executed when conditions are not established}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>if...else</title>
<script type="text/JavaScript">
var ticket =true; //The initial ticket value is true, indicating that there are tickets.
if (ticket="true")
{
document.write("With tickets, you can watch movies.");
}
else
{
document.write("No tickets, no movies.");
}
</script>
</head>
<body>
</body>
</html>
Multiple Judgment (if..else nested statement)
To select a set of statements to execute, use if..else nested statements.
Grammar:
if(condition1)
{ condition1Code executed at setup}
else if(condition2)
{ condition2Code executed at setup}
...
else if(condition n)
{ condition n Code executed at setup}
else
{ condition1,2to n Code executed in case of failure}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>if...else</title>
<script type="text/JavaScript">
var myage =99;//Zhao Hong is 99 years old.
if(myage<=44)
{document.write("Youth");}
else if(myage<=59)
{document.write("Middle-aged person");}
else if (myage<=89)
{document.write("Aged");}
else
{document.write("the longevous");}
</script>
</head>
<body>
</body>
</html>
Multiple Choices (Switch Statement)
When there are many options, switch is more convenient to use than if else.
grammar
Switch (expression) { case value 1: Execution block 1 break; case value 2: Execution code block 2 break; ... case value n: Execution block n break; default: Code executed at different times than case value 1, case value 2...case value n }
Grammatical Explanation
Switch must assign an initial value that matches each case value. Satisfy all statements after executing the case and use the break statement to prevent the next case from running. If all case values do not match, execute the statement after default.
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>switch</title>
<script type="text/JavaScript">
var myweek =3;//myweek represents the week variable
switch (myweek)
{
case 1:
case 2:
document.write("Learning concepts and knowledge");
break;
case 3:
case 4:
document.write("To Enterprise Practice");
break;
case 5:
document.write("Summarize experience");
break;
case 6:
case 7:
document.write("Saturdays, Days Rest and Entertainment");
break;
default:
alert("What do you want?")
}
</script>
</head>
<body>
</body>
</html>
Note: Remember to add a break statement after the statement executed by case. Otherwise, go ahead and execute the following statement in case directly. Look at the following code:
Repeat (for loop)
If you want to run the same code over and over again with different values each time, it's convenient to use loops.
for statement structure:
For (Initializing variables; Cyclic conditions; Cyclic iteration) { Loop statement }
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>if...else</title>
<script type="text/JavaScript">
var mymoney,sum=0;//mymoney variable holds different face values, sum total
for(mymoney=1;mymoney<=10;mymoney++)
{
sum= sum + mymoney;
}
document.write("sum Total:"+sum);
</script>
</head>
<body>
</body>
</html>
Repeated (while loop)
Grammatical structure
While (Judgment Conditions) { Loop statement }
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>while</title>
<script type="text/javascript">
var mynum =1;//mynum initial value is 1
while(mynum<=5)
{
document.write("number:"+mynum);
mynum=mynum;
}
</script>
</head>
<body>
</body>
</html>
Tip: When using a loop, if you forget to add the value of the variable used in the condition, the loop will never end. This may cause the browser to crash or the dead loop to get stuck.
Back and forth (Do...while loop)
The basic principle of the do while structure is basically the same as that of the while structure, but it guarantees that the loop body is executed at least once. Because it executes the code first, then judges the condition. If the condition is true, continue the loop.
do...while statement structure:
do { Loop statement } While (Judgment Conditions)
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>do...while</title>
<script type="text/javascript">
var mynum =6;//Initialization of mynum to 6
do{
document.write("number:"+mynum+"<br/>");
mynum=mynum-1;
}
while( mynum>=1 )
</script>
</head>
<body>
</body>
</html>
Exit loop break
Use break statements in while, for, do...while, while loops to exit the current loop and execute the following code directly.
The format is as follows and demo:
For (initial condition; judgement condition; condition value update after loop) { If (special case) {break;} Loop code }
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>break</title>
<script type="text/JavaScript">
var mynum =new Array(70,80,66,90,50,100,89);//Define array mynum and assign values
var i=0;
while(i<mynum.length)
{
if(mynum[i]<60)
{
document.write("achievement"+mynum[i]+"Failure, no cycle"+"<br>");
break;
}
document.write("achievement:"+mynum[i]+"Pass, continue the cycle"+"<br>");
i=i+1;
}
</script>
</head>
<body>
</body>
</html>
continue to cycle
The function of continue is to just skip this cycle and the whole cycle continues to execute.
Statement structure and demo:
For (initial condition; judgement condition; condition value update after loop) { If (special case) { continue; } Loop code }
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>continue</title>
<script type="text/JavaScript">
var mynum =new Array(70,80,66,90,50,100,89);//Define array mynum and assign values
var i;
for(i=0;i<mynum.length;i++)
{
if(mynum[i]<60)
{
document.write("Failure in grades, no output!"+"<br>");
continue;
}
document.write("achievement:"+mynum[i]+"Pass, Output!"+"<br>");
}
</script>
</head>
<body>
</body>
</html>
The Final Programming Exercise of Mu Course Net
Links to programming exercises: http://www.imooc.com/code/1484
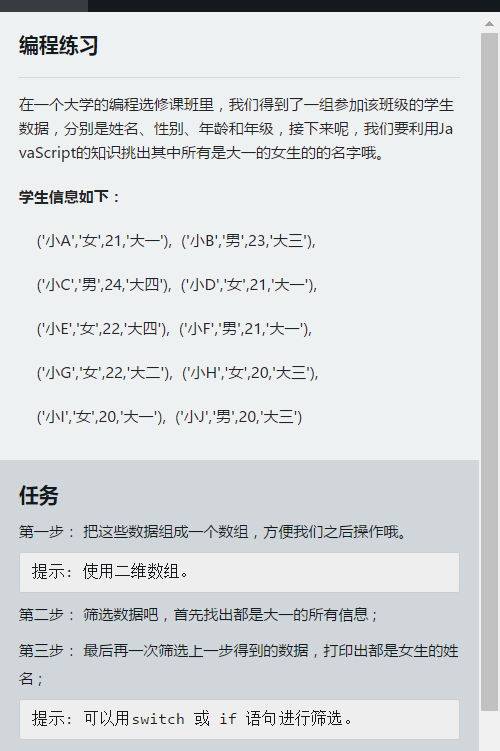
Mu lesson net link: http://www.imooc.com/learn/10
Finally, it comes to the favorite part of the audience's friends to ask for praise and attention: the friends who hope to finish watching will like it. They want to pay attention to how my vegetable chicken grows. They can also pay attention to me. Basically, there will be no fewer than fifteen articles every month (I will share them when I see dry goods). Then? github Also add a star to each other. The code is not easy. Thank you for your support. Thank you very much.
ps: If you want me to comment on what kind of articles I write, or trust me personally, although I don't write well, I think it's a way to record my growth. (Provided I can, if not, I will write it down and come back later.)
Brief Book Home Page Link,csdn blog home page link