Links to the original text: http://www.cnblogs.com/riasky/p/3371884.html
1. Generic Interface
1.1 Basic concepts of generic interfaces
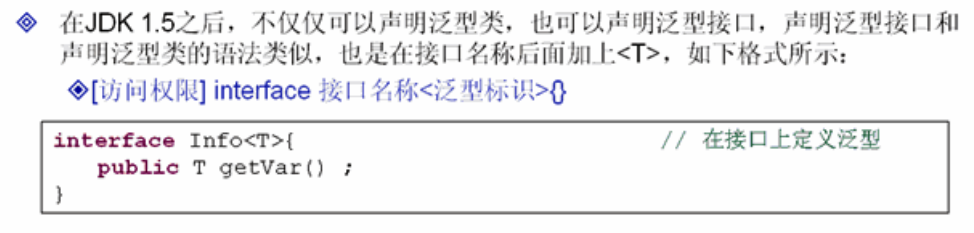
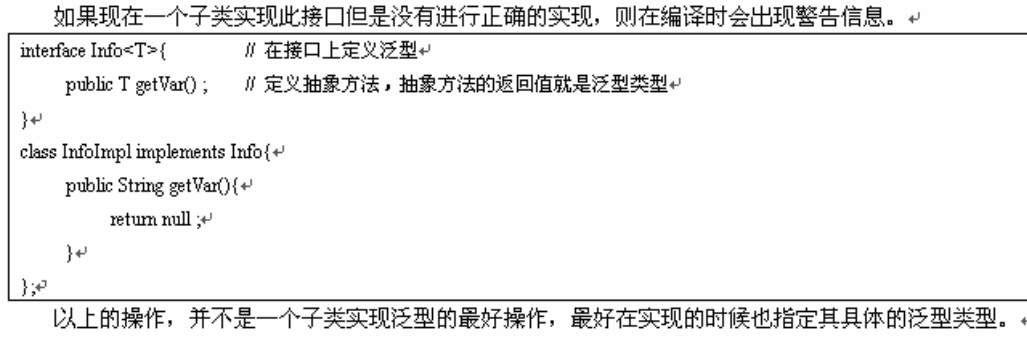
Two Ways of Implementing 1.2 Generic Interface
Define subclasses: Generic types are also declared in the definition of subclasses
If the subclass that implements the interface does not want to use generic declaration now, specify its specific operation type directly when implementing the interface:interface Info<T>{ // Define generics on interfaces public T getVar() ; // Define an abstract method whose return value is a generic type } class InfoImpl<T> implements Info<T>{ // Define subclasses of generic interfaces private T var ; // Define attributes public InfoImpl(T var){ // Setting attribute content by constructing method this.setVar(var) ; } public void setVar(T var){ this.var = var ; } public T getVar(){ return this.var ; } }; public class GenericsDemo24{ public static void main(String arsg[]){ Info<String> i = null; // Declare interface objects i = new InfoImpl<String>("Li Xinghua") ; // Instantiating objects through subclasses System.out.println("Contents:" + i.getVar()) ; } };
interface Info<T>{ // Define generics on interfaces public T getVar() ; // Define an abstract method whose return value is a generic type } class InfoImpl implements Info<String>{ // Define subclasses of generic interfaces private String var ; // Define attributes public InfoImpl(String var){ // Setting attribute content by constructing method this.setVar(var) ; } public void setVar(String var){ this.var = var ; } public String getVar(){ return this.var ; } }; public class GenericsDemo25{ public static void main(String arsg[]){ Info i = null; // Declare interface objects i = new InfoImpl("Li Xinghua") ; // Instantiating objects through subclasses System.out.println("Contents:" + i.getVar()) ; } };
2. Generic Method
2.1 Defining generic methods
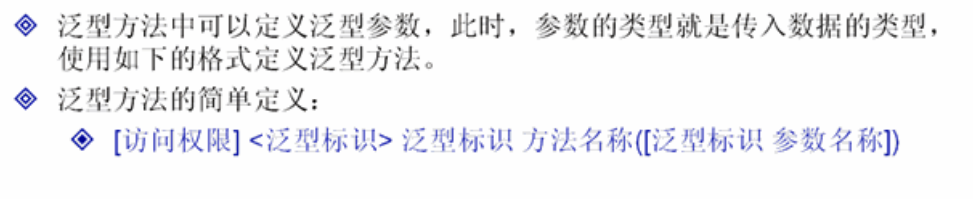
class Demo{ public <T> T fun(T t){ // Can receive any type of data return t ; // Return parameters directly } }; public class GenericsDemo26{ public static void main(String args[]){ Demo d = new Demo() ; // Instantiating Demo Objects String str = d.fun("Li Xinghua") ; // Delivery string int i = d.fun(30) ; // Transfer numbers, automatic packing System.out.println(str) ; // Output content System.out.println(i) ; // Output content } };
2.2 Return instances of generic classes through generic methods
class Info<T extends Number>{ // Specify an upper limit, which can only be a number type private T var ; // This type is determined externally. public T getVar(){ return this.var ; } public void setVar(T var){ this.var = var ; } public String toString(){ // Override the toString() method in the Object class return this.var.toString() ; } }; public class GenericsDemo27{ public static void main(String args[]){ Info<Integer> i = fun(30) ; System.out.println(i.getVar()) ; } public static <T extends Number> Info<T> fun(T param){ Info<T> temp = new Info<T>() ; // Instantiate Info based on the incoming data type temp.setVar(param) ; // Set the passed content to the var attribute of the Info object return temp ; // Returns the instantiated object } };
2.3 Use generics to unify the types of incoming parameters
If the types of the two generics in the add method are not uniform, compilation errors occur.class Info<T>{ // Specify an upper limit, which can only be a number type private T var ; // This type is determined externally. public T getVar(){ return this.var ; } public void setVar(T var){ this.var = var ; } public String toString(){ // Override the toString() method in the Object class return this.var.toString() ; } }; public class GenericsDemo28{ public static void main(String args[]){ Info<String> i1 = new Info<String>() ; Info<String> i2 = new Info<String>() ; i1.setVar("HELLO") ; // Setting content i2.setVar("Li Xinghua") ; // Setting content add(i1,i2) ; } public static <T> void add(Info<T> i1,Info<T> i2){ System.out.println(i1.getVar() + " " + i2.getVar()) ; } };
class Info<T>{ // Specify an upper limit, which can only be a number type private T var ; // This type is determined externally. public T getVar(){ return this.var ; } public void setVar(T var){ this.var = var ; } public String toString(){ // Override the toString() method in the Object class return this.var.toString() ; } }; public class GenericsDemo29{ public static void main(String args[]){ Info<Integer> i1 = new Info<Integer>() ; Info<String> i2 = new Info<String>() ; i1.setVar(30) ; // Setting content i2.setVar("Li Xinghua") ; // Setting content add(i1,i2) ; } public static <T> void add(Info<T> i1,Info<T> i2){ System.out.println(i1.getVar() + " " + i2.getVar()) ; } };

3. Generic Array
public class GenericsDemo30{ public static void main(String args[]){ Integer i[] = fun1(1,2,3,4,5,6) ; // Returns a generic array fun2(i) ; } public static <T> T[] fun1(T...arg){ // Receiving variable parameters return arg ; // Returns a generic array } public static <T> void fun2(T param[]){ // output System.out.print("Receive generic arrays:") ; for(T t:param){ System.out.print(t + ",") ; } } };
4. Nested settings for generics
The info attribute in the Demo class is that of the Info class, which itself requires two generics.
class Info<T,V>{ // Receive two generic types private T var ; private V value ; public Info(T var,V value){ this.setVar(var) ; this.setValue(value) ; } public void setVar(T var){ this.var = var ; } public void setValue(V value){ this.value = value ; } public T getVar(){ return this.var ; } public V getValue(){ return this.value ; } }; class Demo<S>{ private S info ; public Demo(S info){ this.setInfo(info) ; } public void setInfo(S info){ this.info = info ; } public S getInfo(){ return this.info ; } }; public class GenericsDemo31{ public static void main(String args[]){ Demo<Info<String,Integer>> d = null ; // Using Info as a generic type of Demo Info<String,Integer> i = null ; // Info specifies two generic types i = new Info<String,Integer>("Li Xinghua",30) ; // Instantiate Info objects d = new Demo<Info<String,Integer>>(i) ; // Setting Info Class Objects in Demo Class System.out.println("Content 1:" + d.getInfo().getVar()) ; System.out.println("Content 2:" + d.getInfo().getValue()) ; } };
Reprinted at: https://www.cnblogs.com/riasky/p/3371884.html