Implementation of data structure and algorithm with single chain table C language
Posted by John Rowe on Sat, 04 Apr 2020 04:44:45 +0200
1. Difference between head node and head pointer
- Head pointer: pointer to the first node of the list. If the list has a head node, it refers to the head node. No matter whether the list is empty or not, the head pointer is not empty. The head pointer is a necessary element of the list
- Head node: it is set up for the unification and convenience of operation. Before the first node, the data field is generally meaningless. With the head node, the operation of inserting and deleting the first node before the first node is unified with that of other nodes
2. Structure pointer description single chain table
#include <stdio.h>
#include <stdlib.h>
typedef int ElemType;
typedef struct Node{
ElemType data;
struct Node *next;
} Node;
typedef struct Node *LinkList;
3. Get the i-th element
//p is a pointer to a node, p - >nextPointer to the next node:So the data field of the next node is p->next->data,The pointer field is p->next->next
//Pointers and structs access member variables. Structs use. Pointers use - >
int GetElem(LinkList L, int i, ElemType *e){
int j;
LinkList p;
p = L->next;//Here L Represents the pointer,L->nextIt may be the head node,It could be the first node
j = 1;
while (p && j < i) {//Iterative traversal
p = p->next;
j++;
}
if (!p || j > i) { //p=null Or more than i,Can't find
return 0;
}
*e = p->data;
return 1;
}
4. Insert element at position i
int ListInsert(LinkList *L,int i, ElemType e){
int j = 1;
LinkList p,s;
p = *L;//L->nextThe difference between the two?
while (p && j < i) {
p = p->next;
j++;
}
if (!p || j > i) {
return 0;
}
s = (LinkList)malloc(sizeof(Node));
s->data = e;
s->next = p->next;
p->next = s;
return 0;
}
5. Delete the i-th element
int ListDelete(LinkList *L, int i, ElemType *e){
int j = 1;
LinkList p,s;
p = *L;
while (p && j < i) {
p = p->next;
j++;
}
if (!p || j > i) {
return 0;
}
s = p->next;//First of all i Nodes saved,In order to release
p->next = s->next;//s->nextNamely p->next->next
*e = s->data;
free(s);
return 1;
}
6. Creating single chain table by head insertion
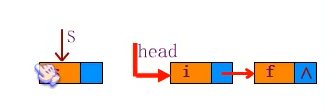
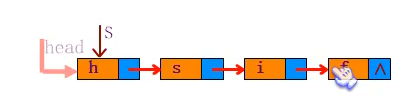
void CreateListHead(LinkList *L, int n){
LinkList p;
int i;
*L = (LinkList)malloc(sizeof(Node));//Head node-->Header
(*L)->next = NULL;//Empty linked list
for (i = 0; i < n; i++) {
p = (LinkList)malloc(sizeof(Node));//Generate new node
p->data = rand() %100 + 1;
p->next = (*L)->next;
(*L)->next = p;
}
}
7. Create single chain table by tail insertion
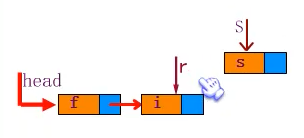
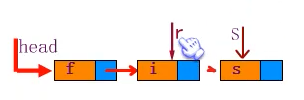
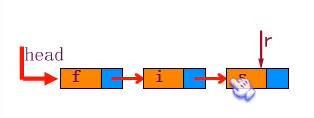
void CreateListTail(LinkList *L,int n){
LinkList p,r;
int i;
*L = (LinkList)malloc(sizeof(Node));
r = *L;
for (i = 0; i < n; i++) {
p = (LinkList)malloc(sizeof(Node));
p->data = rand() % 100 + 1;
r->next = p;
r = p;
}
r->next = NULL;
}