Before using mobile phone login do not verify code, now login always verify code, boring people to death! So why do you have annoying verification codes every time you log in? In fact, this involves the complete network problem!
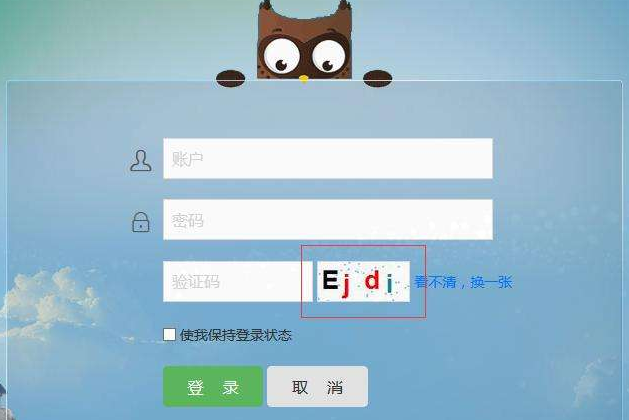
1, Application scenario
Many partners should know:
Prevent hackers from calling the attack system through the interface, and prevent the machine from accessing each time they log in the system and input the verification code.
Do the current limiting processing to prevent the influx of a large number of users at the same time and prevent the system from crashing.
Types of verification codes
Traditional input verification code: users input letters, numbers and Chinese characters in the picture for verification. It is easy to operate and has good human-computer interaction. But the safety factor is low and easy to be attacked.
Input type graphic verification code: there are exquisite patterns, the recognition text is clear and recognizable, focusing on advertising. A display form of advertising space.
Pure behavior verification code: slide the candidate fragment straight to the correct position as required. Simple operation, good experience. Single dimension, easy to be reverse simulated, incompatible with mobile page switching.
Icon selection and behavior assistance: give a group of pictures and click one or more of them as required. Use the difficulty of everything identification to block the machine. Strong security. High requirements for pictures, library and technology.
Click to text verification and behavior assistance: users are reminded to click the same word position in the diagram to verify. The operation is simple, the experience is good, the single picture area is large, and it is difficult to be attacked.
Smart verification code: normal users are exempt from verification and abnormal users are forced to verify through behavior characteristics, device fingerprint, data risk control and other technologies. It is simple and convenient to distinguish people and machines, people and people, equipment and equipment.
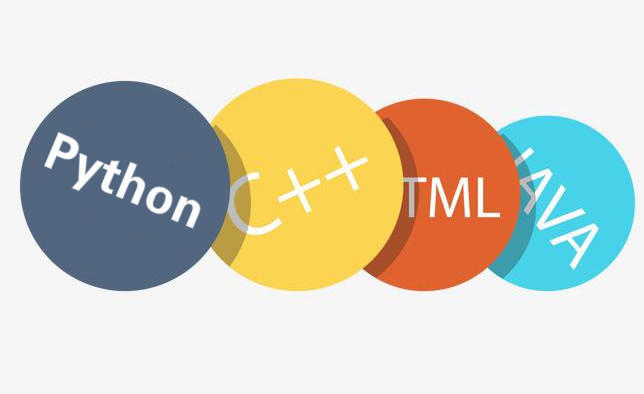
2, Different implementations
The following three different programming languages, through the code generation verification code.
2.1 Java language implementation
Let's see how Java code generates verification code. Create the following class manually to generate the verification code. The code is as follows:
public class GenVerifyCodeUtils { private static char mapTable[] = { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9'}; public static void main(String[] args) { OutputStream outputStream = new BufferedOutputStream(new ByteArrayOutputStream()); System.out.println(getImageCode(100,80,outputStream )); } public static Map<String, Object> getImageCode(int width, int height, OutputStream os) { Map<String,Object> returnMap = new HashMap<String, Object>(); if (width <= 0) width = 60; if (height <= 0) height = 20; BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB); // Get drawing context Graphics g = image.getGraphics(); //Generate random class Random random = new Random(); // Set background color g.setColor(getRandColor(200, 250)); g.fillRect(0, 0, width, height); //set font g.setFont(new Font("Times New Roman", Font.PLAIN, 18)); // 168 interference lines are randomly generated, which makes the authentication code in the image hard to be detected by other programs g.setColor(getRandColor(160, 200)); for (int i = 0; i < 168; i++) { int x = random.nextInt(width); int y = random.nextInt(height); int xl = random.nextInt(12); int yl = random.nextInt(12); g.drawLine(x, y, x + xl, y + yl); } //Take random code String strEnsure = ""; //4 4-digit verification code,If you want to generate more authentication codes,Then increase the value for (int i = 0; i < 4; ++i) { strEnsure += mapTable[(int) (mapTable.length * Math.random())]; // Display the authentication code in the image g.setColor(new Color(20 + random.nextInt(110), 20 + random.nextInt(110), 20 + random.nextInt(110))); // Direct generation String str = strEnsure.substring(i, i + 1); // Set the position of random code on the background picture g.drawString(str, 13 * i + 20, 25); } // Release drawing context g.dispose(); returnMap.put("image",image); returnMap.put("strEnsure",strEnsure); return returnMap; } static Color getRandColor(int fc, int bc) { Random random = new Random(); if (fc > 255) fc = 255; if (bc > 255) bc = 255; int r = fc + random.nextInt(bc - fc); int g = fc + random.nextInt(bc - fc); int b = fc + random.nextInt(bc - fc); return new Color(r, g, b); } }
The effect is as follows:
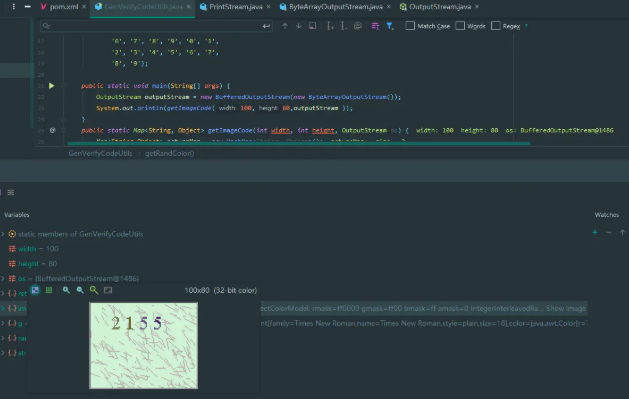
2.2 Javascript implementation
Here I also write a tool to generate verification code with native Js, the code is as follows:
<form action="#"> <input type="text" id="input1" onblur="inputBlur()"/> <input type="text" onclick="createCode()" readonly="readonly" id="checkCode" class="unchanged" /><br /> </form> <script language="javascript" type="text/javascript"> var code; //Define verification code globally var code2; //Define verification code globally function createCode() { code = ""; var checkCode = document.getElementById("checkCode"); function RndNum(n) { var rnd = ""; for (var i = 0; i < n; i++) rnd += Math.floor(Math.random() * 10); return rnd; } var num = RndNum(2); var num2 = RndNum(2); code = num + "+" + num2 + "="; code2 = parseInt(num) + parseInt(num2) if (checkCode) { checkCode.className = "code"; checkCode.value = code; } } function inputBlur(){ var inputCode = document.getElementById("input1").value; if (inputCode.length <= 0) { alert("Please enter the verification code!"); } else if (inputCode != code2) { alert("Verification code input error!"); createCode(); } else { alert("^-^ OK"); } } </script> <style type="text/css"> .code { font-family: Arial; font-style: italic; color: Red; border: 0; padding: 2px 3px; letter-spacing: 3px; font-weight: bolder; } .unchanged { border: 0; } </style>
The effect is as follows:

2.3 python implementation
The code is as follows:
# -*- coding: utf-8 -* from PIL import Image, ImageDraw, ImageFont, ImageFilter import random # Random letters: def rndChar(): return chr(random.randint(65, 90)) # Random color 1: def rndColor(): return (random.randint(64, 255), random.randint(64, 255), random.randint(64, 255)) # Random color 2: def rndColor2(): return (random.randint(32, 127), random.randint(32, 127), random.randint(32, 127)) # 240 x 60: width = 60 * 4 height = 60 image = Image.new('RGB', (width, height), (255, 255, 255)) # establish Font object: font = ImageFont.truetype('C:\Windows\Fonts\Arial.ttf', 36) # establish Draw object: draw = ImageDraw.Draw(image) # Fill each pixel: for x in range(width): for y in range(height): draw.point((x, y), fill=rndColor()) # Output text: for t in range(4): draw.text((60 * t + 10, 10), rndChar(), font=font, fill=rndColor2()) # vague: image = image.filter(ImageFilter.BLUR) image.save('code.jpg', 'jpeg') image.show()
The operation effect is as follows:
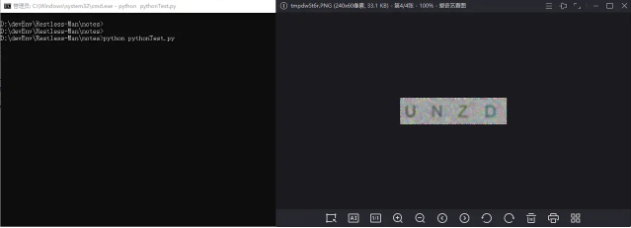
3, Finally
This article talks about why there are verification codes and the types of verification codes on the market. It gives you a brief introduction of science popularization. At last, it shows the process of generating verification codes in different programming languages. Now network security is particularly important. Although the function of verification code is small, it must be done!
In fact, as a programming learner, it's very important to have a learning atmosphere and a communication circle. Here I recommend a C language c + + communication Q group 1108152000. No matter you are Xiaobai or a transfer person, welcome to settle down and grow up together.
WeChat official account: C language programming learning base