(1) Foreword
C + + is the inheritance of C language and is compatible with most C syntax. Some syntax is added on the basis of C + + in order to solve the problems that C language can't do and the modifications are not good enough
- What is C++
C language is a structured and modular language, which is suitable for dealing with small-scale programs. C language is not suitable for complex problems and large-scale programs that require a high degree of abstraction and modeling. In order to solve the software crisis, OOP (object-oriented programming) was proposed in the computer industry in the 1980s, and the object-oriented programming language came into being.
In 1982, Dr. Bjarne Stroustrup introduced and extended the concept of object-oriented on the basis of C language and invented a new programming language. In order to express the origin relationship between the language and C language, it is named C + +. Therefore, C + + is based on C language. It can not only carry out procedural programming of C language, object-based programming characterized by abstract data types, but also object-oriented programming.
- Development history of C + +
The two most important versions | content |
---|---|
C++98 | The first version of C + + standard is supported by most compilers. It has been recognized by the international organization for Standardization (ISO) and the American Institute for standardization. It rewrites the C + + standard library in the form of template and introduces STL (Standard Template Library) |
C++11 | Many features have been added to make C + + more like a new language, such as regular expression, range based for loop, auto keyword, new container, list initialization, standard thread library, etc |
- Application fields of C + +
- Operating system and large system software development
- Server side development
- artificial intelligence
- Network tools
- Game development
- Embedded domain
- digital image processing
- distributed application
- Mobile device
- Learning C + +: it is recommended not to take "Mastering C + +" as a one-year goal, but to take language learning as a continuous process, and use the language in specific applications.
(2) C + + keyword (C++98)
- There are 63 keywords in C + +, 32 of which we have seen when learning C language
- In the following learning process, when you learn a keyword, you can start to talk in detail
asm | do | if | return | try | continue |
auto | double | inline | short | typedef | for |
bool | dynamic_cast | int | signed | typeid | public |
break | else | long | sizeof | typename | throw |
case | enum | mutable | static | union | wchar_t |
catch | explicit | namespace | static_cast | unsigned | default |
char | export | new | struct | using | friend |
class | extern | operator | switch | virtual | register |
const | false | private | template | void | true |
const_cast | float | protected | this | volatile | while |
delete | goto | reinterpret_cast |
(3) Namespace
- A problem in C language
In different scopes, we can define variables with the same name; A variable with the same name cannot be defined in the same scope.
Therefore, in a large project, multiple people write code according to their division of labor. Finally, when they are combined, the names in the code you write and the code I write may conflict, and the names in the code we write may conflict with those in the standard library.
If Zhang San wrote a function named Add, I also wrote a function named Add. When the code is combined, it may cause naming conflicts and so on.
- Examples
If the stdlib.h header file is not included, the code can run normally;
But after it is included, because there is a function called rand in the stdlib.h library, the global variable rand conflicts with it.
#include<stdio.h> #include<stdlib.h> /*rand*/ int rand = 10; //Global variable rand int main() { printf("%d\n", rand); //error: "rand" redefined. The previous definition was "function" return 0; }
- C + + proposes namespace to solve the problem of naming conflict
In C/C + +, there are a large number of variables, functions and classes. The names of these variables, functions and classes will exist in the global scope, which may lead to many conflicts. The purpose of using namespace is to localize the name of identifier to avoid naming conflict or name pollution. The emergence of namespace keyword is aimed at this problem.
A namespace is equivalent to a domain, separating what they write.
1) Definition of namespace
-
To define a namespace, you need to use the namespace keyword, followed by the name of the namespace, followed by a pair of {}, which are the members of the namespace.
-
Note: a namespace represents the definition of a new scope, and all contents in the namespace are limited to the namespace.
- Common definition of namespace
//Common definition of namespace namespace L1 //L1 is the name of the namespace { //Variables / functions / types can be defined in the namespace int a = 10; int Add(int x, int y) { return x + y; } struct Node { struct Node* next; int val; }; }
- Nested definition of namespace
//Nested definition of namespace namespace L2 { int a = 10; namespace L3 //Nested defines a namespace named L3 { int b = 20; int sub(int x, int y) { return x - y; } } }
- Multiple namespaces with the same name are allowed in the same project, and the compiler will finally synthesize them into the same namespace (therefore, variables / functions / types with the same name cannot appear in namespaces with the same name, otherwise they will cause conflicts)
2) Use of namespaces
//Define a namespace N1 namespace N1 { int a = 10; int b = 10; int Add(int x, int y) { return x + y; } }
There are three ways to use namespaces:
- Expand all to the global, and you can use it directly (using namespace to introduce namespace names)
// Advantages: easy to use // Disadvantages: expose your own definition, resulting in naming pollution //using namespace std; //std is the namespace that contains the C + + standard library using namespace N1; int main() { Add(1, 2); return 0; }
- When accessing members in a namespace, add the namespace name and scope qualifier
// Advantages: no naming pollution // Disadvantages: it's cumbersome to use. Everyone needs to specify a namespace int main() { printf("%d\n", N1::a); N1::Add(1, 2); return 0; }
- using to bring in commonly used members from a namespace
// Advantages: it will not cause large-area naming pollution, and can expand the commonly used names // This is a compromise solution using N1::Add; //The Add function and variable a in namespace N1 are used very much. Expand them to the global using N1::a; int main() { printf("%d\n", a); Add(1, 2); return 0; }
- One additional point: the standard use of namespace std
C + + puts everything in the standard library into the namespace std, so in actual development, in order to avoid conflicts between variables / functions / types written by yourself and the standard library, it is recommended not to expand the namespace std directly to the global, but to expand the commonly used ones. The general specification is as follows:
#include<iostream> //using namespace std; // Do not expand STD directly to the global //Just expand the common ones using std::cout; using std::endl; int main() { cout << "hello world" << endl; return 0; }
If it's a daily exercise, it doesn't need to be as standardized as above. It can be used directly
(3) Input & output of C + +
C language has its own input and output functions scanf and printf, so c + + also has its own unique input and output mode. cin standard input stream and cout standard output stream must contain header file and std standard library namespace.
Note: in the early standard library, all functions were implemented in the global domain and declared in the header file with. h suffix. When used, it only needs to include the corresponding header file. Later, it is now in the std namespace. In order to distinguish it from the C header file and correctly use the namespace, it is stipulated that the C + + header file does not contain. h; The old compiler (vc 6.0) also supports the < iostream. h > format, which is no longer supported by subsequent compilers. Therefore, the + std method is recommended.
It is more convenient to use c + + input and output. It can automatically identify the type of variables without adding data format control, such as shaping –% d and character –% c
#include<iostream> using namespace std; //C + + standard library int main() { int a; cin >> a; // >>Input operator / stream extraction operator cout << a; // < < output operator / stream insertion operator cout << endl; //Line feed, equivalent to cout < < '\ n'; return 0; }
- Note: for floating-point numbers in C + +, when the number after the decimal point exceeds 5 digits, cout specifies that the output is 5 digits after the decimal point. If you want to realize the formatted output of floating-point numbers, it is recommended to use printf for output. The use must be flexible.
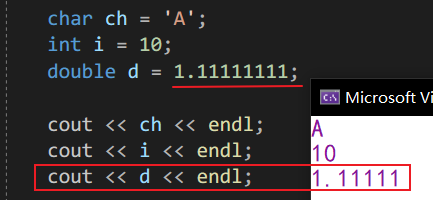

(4) Default parameters
1) Concept of default parameters
**The default parameter is to specify a default value for the parameter of the function when the function is declared or defined** When calling the function, if no argument is specified, the default value is adopted; otherwise, the specified argument is used.
#include<iostream> using namespace std; void Func(int a = 0) { cout << a << endl; } int main() { Func(); //When no parameter is passed, the default value of the parameter is used Func(10); //When passing parameters, use the specified parameters return 0; }
- Use of default parameters
Before entering the stack, you must judge whether the capacity is 0. If it is 0, you can assign an initial value to capacity, but the assigned initial value cannot be controlled flexibly. With default parameters, we can control the initial capacity as needed.
#include<iostream> using namespace std; //Define a sequential stack struct Stack { int* a; int top; int capacity; }; //Initialize the stack and set a default value of 4 for the stack capacity void StackInit(struct Stack* ps, int DefaultCapacity = 4) { ps->a = (int*)malloc(sizeof(int) * DefaultCapacity); ps->top = -1; ps->capacity = 0; } //Push void StackPush(struct Stack* ps) { if (ps->top == ps->capacity + 1) { ps->capacity *= 2; //...... } //...... } int main() { //If I know clearly that at least 100 data should be stored in st1 struct Stack st1; StackInit(&st1, 100); //If I don't know how many data to save to st2 struct Stack st2; StackInit(&st2); return 0; }
2) Classification of default parameters
- All default parameters
#include<iostream> using namespace std; void Func(int a = 10, int b = 20, int c = 30) { cout << a + b + c << endl; } int main() { //It is flexible to call functions with all default parameters Func(); Func(1); Func(1, 2); Func(1, 2, 3); return 0; }
- Semi default parameter
#include<iostream> using namespace std; void Func(int a, int b = 10, int c = 20) { cout << a + b + c << endl; } int main() { //Calling a function with semi default parameters Func(1); //1 to a Func(1, 2); //1 to a, 2 to b Func(1, 2, 3); //1 to a, 2 to b, 3 to c return 0; }
- summary
- Semi default parameters must be given from right to left, and cannot be given at intervals
- Default parameters cannot appear in function declarations and definitions at the same time (it is recommended to give default parameters when declaring, but not when defining)
//Note: if the declaration and definition locations appear at the same time, and the values provided by the two locations happen to be different, the compiler cannot determine which default value to use. //test.h void Func(int a = 10); //test.c void Func(int a = 20) { //...... }
- The default value must be a constant or a global variable
//You cannot give default values like this void Func(int a, int b = x);//error
- The C language does not support default parameters (not supported by the compiler)
(5) Function overloading
In natural language, a word can have multiple meanings. People can judge the true meaning of the word through the context, that is, the word is overloaded.
There used to be a joke that there were two state-owned sports that we didn't have to watch or worry about at all. One is table tennis and the other is men's football. The former is "no one can win!", The latter is "no one can win!"
It can be seen that the same sentence may have multiple meanings.
- Concept of function overloading
Function overloading is a special case of functions. C + + allows to declare several functions with the same name with similar functions in the same scope. The formal parameter list (number of parameters / type / order) of these functions with the same name must be different. It is commonly used to deal with problems with similar functions but different data types.
int Add(int a, int b) { return a + b; } double Add(double a, double b) { return a + b; } long Add(long a, long b) { return a + b; } int main() { Add(10, 20); Add(10.0, 20.0); Add(10L, 20L); //L indicates that the constant is stored as a long integer, long return 0; }
- reflection
- Can the compiler implement function overloading with the same function name, the same parameters and different return values?
Conclusion: it is impossible to implement and overload functions distinguished only by return value type.
int func(); -> _Z3ifunc double func(); -> _Z3dfunc
If the return value is brought into the name modification rule, the compiler level can be distinguished.
However, at the syntax call level, it is impossible to determine which overloaded function to call according to the parameter list, even with serious ambiguity!
For example, this statement func(), When calling, which one is to be called here?
(6)extern "c"
C + + compiler can recognize C + + function name modification rules and C function name modification rules.
-
In the development of C + + projects, we may use some third-party libraries, some of which are implemented in pure C (dynamic library / static library). The function name modification rules in it are C, while C + + is compatible with C. The C + + compiler calls directly according to the modification rules of C.
-
However, if a pure C project uses a library implemented in C + + (dynamic library / static library), for example, tcmalloc is a library implemented by google in C + +. It provides two interfaces tcmallc() and tcfree() (more efficient, instead of malloc and free functions). This library can be used for C + + projects, but not for C projects. There will be link failures, Because the C compiler does not recognize C + + decorating rules.
-
If you want to use it for C, you need to compile some functions in the C + + library in the style of C, and add extern "C" before the function, which means to tell the compiler to compile the function according to the modification rules of C.
extern "C" void Add(int a, int b);
- summary
-
C + + projects can call C + + libraries or C libraries.
-
C projects can call C libraries. If you want to call C + + libraries, you need to add extern "C" before the function.
- Thinking questions
-
Can the following two functions form a function overload? - > No
void Func(int a = 10) { cout << "Func(int)" << endl; } void Func(int a) { cout << "Func(int)" << endl; }
-
Why can't function overloading be supported in C language?
——>The function name modification rules of C and C + + compilers are different. The C compiler directly takes the function name in the target file as the mapping of function name and function address. Therefore, when linking, it takes the function name to the target file. The function name is the same. Although the parameters are different, it does not know who is looking for.
-
How is the underlying function overload handled in C + +?
——>The C + + compiler does not directly find the function name, but the modified function name. The modified function name is different with different parameters, so it can be found.
-
Can a function be compiled in C + + in the style of C?
——>Yes, add extern "C" before the function
To be continued, in the next article, we will learn one of the most important knowledge in C + +, quote... Look forward to it together!