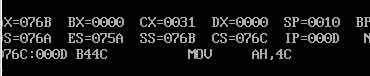



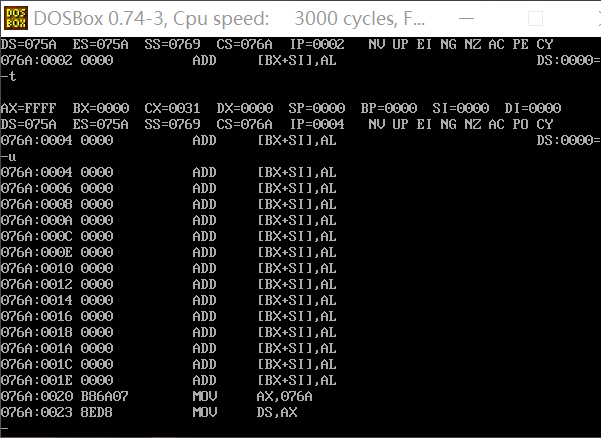
task1_2. It cannot be executed correctly
task1_3. It cannot be executed correctly
task1_4 can be executed correctly
Because after end start is changed to end, the first three start with the data segment, cs points to an error, and the fourth start is the program segment, so it can still be executed
Experimental code task2.asm
assume cs:code code segment start: mov ax,0b800h mov ds,ax mov bx,0f00h mov ax,0403h mov cx,80 s: mov ds:[bx],ax add bx,2 loop s mov ah, 4ch int 21h code ends end start
Experimental task 3
Supplementary task3.asm source code
assume cs:code data1 segment db 50, 48, 50, 50, 0, 48, 49, 0, 48, 49 ; ten numbers data1 ends data2 segment db 0, 0, 0, 0, 47, 0, 0, 47, 0, 0 ; ten numbers data2 ends data3 segment db 16 dup(0) data3 ends code segment start: mov ax, data1 ; mov ax, data2 ; mov ax, data3 mov ds,ax mov bx,0 mov cx,10h s: mov ax,ds:[bx] add ax,ds:[bx+10h] mov ds:[bx+20h],ax inc bx loop s mov ah, 4ch int 21h code ends end start
Code run results
Disassembly result:
Memory condition before operation
The memory after running the code successfully is shown in the figure below
Experimental task 4
task4.asm source code
assume cs:code data1 segment dw 2, 0, 4, 9, 2, 0, 1, 9 data1 ends data2 segment dw 8 dup(?) data2 ends code segment start: mov ax, data1 mov ds, ax mov ax, data2 mov es, ax mov ss, ax mov sp, 32 mov bx, 0 mov cx, 8 s1: push [bx] add bx, 2 loop s1 mov bx,16 mov cx, 8 s2: pop [bx] add bx, 2 loop s2 mov ah, 4ch int 21h code ends end start
Disassembly result:
Memory before running
Memory after code running
Experimental task 5
Source code:
assume cs:code, ds:data data segment db 'Nuist' db 2, 3, 4, 5, 6 data ends code segment start: mov ax, data mov ds, ax mov ax, 0b800H mov es, ax mov cx, 5 mov si, 0 mov di, 0f00h s: mov al, [si] and al, 0dfh mov es:[di], al mov al, [5+si] mov es:[di+1], al inc si add di, 2 loop s mov ah, 4ch int 21h code ends end start
Program running results:
After line 25 and before line 27
The function of the source program is to print NUIST in uppercase color
Change line 4:
The values here are used to set the color of the displayed characters.
Experimental task 6
Program source code:
assume cs:code, ds:data data segment db 'Pink Floyd ' db 'JOAN Baez ' db 'NEIL Young ' db 'Joan Lennon ' data ends code segment start: mov ax, data mov ds, ax mov cx, 64 mov bx, 0 s: or [bx], byte ptr 20h inc bx loop s mov ah, 4ch int 21h code ends end start
After program execution:
Experimental task 7
Source code:
assume cs:code, ds:data, es:table data segment db '1975', '1976', '1977', '1978', '1979' dw 16, 22, 382, 1356, 2390 dw 3, 7, 9, 13, 28 data ends table segment db 5 dup( 16 dup(' ') ) ; table ends code segment start: mov ax, data mov ds, ax mov ax, table mov es, ax mov cx, 5 mov bx, 0 mov si, 0 s1: mov ax, [si] mov es:[bx], ax mov ax, [si+2] mov es:[bx+2], ax add bx, 16 add si, 4 loop s1 mov cx, 5 mov bx, 5 mov si, 20 s2: mov ax, [si] mov es:[bx], ax mov ax, 0000h mov es:[bx+2], ax add bx, 16 add si, 2 loop s2 mov cx, 5 mov bx, 10 mov si, 30 s3: mov ax, [si] mov es:[bx], ax add bx, 16 add si, 2 loop s3 mov cx, 5 mov si, 5 s4: mov ax, es:[si] mov bl, es:[si+5] div bl mov es:[si+8], al add si, 16 loop s4 mov ah, 4ch int 21h code ends end start
End of execution:
4, Experimental summary
Through this experiment, I have a better understanding of the form of data stored in memory and the differences between db and dw. At the same time, I reviewed and consolidated the usage of div, the difference between div when 32 bits are divided by 16 bits and 16 bits are divided by 8 bits. Moreover, through the experiment of removing the start of end start, I know the function of start and the possible impact after removal