Today, I am still a fashion, a salted fish programmer with a dream
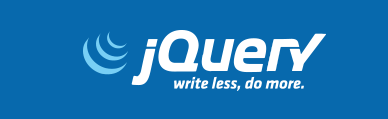
Today, we'll get close to jQuery
But today, there's a big play
-------Ajax-------
What is Ajax?
Baidu entry: Ajax is Asynchronous Javascript And XML (Asynchronous and JavaScript) XML )A new term proposed by Jesse James Garrett in 2005 to describe a 'new' method using a collection of existing technologies, including: HTML or XHTML, CSS, JavaScript, DOM, XML, XSLT And most importantly XMLHttpRequest , using Ajax technology, web applications can quickly render incremental updates in user interface Instead of reloading (refreshing) the entire page, which makes the program respond to user operations faster.
My summary: asynchronous JavaScript and XML
ajax principle:
- Asynchronous exchange using XMLHttpRequest
- The data on the server is usually JSON Save text data in format;
- Run javascript to manipulate the rendered dom
About the advantages of Ajax
Because Ajax contains many features, its advantages and disadvantages are also very obvious.
- No plug-in support is required (general browser and JavaScript can be turned on by default);
- Excellent user experience (updatable data can be obtained without refreshing the page);
- Improve the performance of Web programs (load on demand in terms of data transmission without overall submission);
- Reduce the burden of server and bandwidth (transfer some operations of the server to the client);
-
It is not a single technology of JavaScript, but a combination of a series of technologies related to interactive web applications. Using Ajax, we can update the page without refresh status, and realize asynchronous submission, which improves the user experience
Everything has two sides: Ajax's shortcomings:
- The function of forward and backward is destroyed (because Ajax is always on the current page and will not be on the front and back pages);
- The support of the search engine is not enough (because the search engine crawler cannot understand the content of the changed data caused by JS);
Definition and Usage
The ajax() method loads remote data through an HTTP request.
This method is the underlying AJAX implementation of jQuery. For easy-to-use high-level implementation, see $. get, $.post, etc ajax() returns the XMLHttpRequest object it created. In most cases, you don't need to operate the function directly, unless you need to operate infrequent options for more flexibility.
In the simplest case, $. ajax() can be used directly without any parameters.
What is asynchronous?
The key to using Ajax is to implement asynchronous requests, accept responses, and execute callbacks. So what's the difference between asynchronous and synchronous?
Our ordinary Web program development is basically synchronous, which means that one program can be executed before the next one. Similar to a call on the phone, one call can be answered before the next call is answered; Asynchronous can perform multiple tasks at the same time. It feels like there are multiple lines, which is similar to SMS. It won't stop accepting another SMS because of reading one SMS.
Ajax can also be executed in synchronous mode, but the synchronous mode belongs to blocking mode, which will lead to the execution of multiple lines one by one, which will make the Web page appear pseudo dead state. Therefore, most Ajax adopt asynchronous mode.
Using Ajax
Create an XMLHttpRequest
1. Create xhr
var xhr=new XMLHttpRequest();
2. Open the link open (method,url,asny);
xhr.open("get","url?params1=some¶ms2=somm2",true);
3. send ("params1 = some & params2 = somm2");
xhr.send("name="+uname.value);//send data // The post request needs to send a header xhr.setRequestHeader('Content-Type','application/x-www-form-urlencoded');
4. Listen for readyState status
xhr.onreadystatechange=function(){//When XHR status mode changes if(xhr.status==200&&xhr.readyState==4){ //If the status code of http is 200 (request successful), the status of xhr is 4 (successful status) } }
readyState
0,'uninitialized....' 1,'The request parameters have been prepared and the request has not been sent...' 2,'Request sent,No response has been received' 3,'Accepting partial response.....' 4,'All responses accepted'
response
status Response code 200 successful statusText Response status ok success responseText Text form of response questions
XMLHTTPRequest case
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>ajax</title> </head> <body> <button>get files</button> <script> var btn = document.querySelector("button"); btn.onclick=function(){ //01 declare an xhr object var xhr =new XMLHttpRequest(); //02 open link address xhr.open("GET", "./text.txt",true); //03 send xhr.send(); //04 listening events // readyState is opened when the status is 4 // When the response code of the status service is 200, the response is successful // Return data of responseText service xhr.onreadystatechange=function(){ if(xhr.readyState==4&&xhr.status==200){ console.log(xhr.responseText,xhr); document.querySelector("body") .append(xhr.responseText) } } } </script> </body> </html>
post mode
The url code of xhr request header needs to be set for post
xhr.setRequestHeader('Content-Type','application/x-www-form-urlencoded');
var xhr = new XMLHttpRequest(); xhr.open("post","http://520mg.com/ajax/echo.php"); xhr.setRequestHeader('Content-Type','application/x-www-form-urlencoded'); xhr.send("name=mumu&age=18");
File upload
get files var fileObj = document.getElementById("file").files[0];
To upload text, you need to create a form data object
var form = new FormData()
form.append("file",fileObj);
onload finished loading callback function. Note xhr.send(form) to call last.
function UploadFile(){ var fileObj = document.getElementById("file").files[0]; var url ="https://www.520mg.com/ajax/file.php"; var form = new FormData(); form.append("file",fileObj); var xhr = new XMLHttpRequest(); xhr.open("post",url,true); xhr.onload = function(){ console.log(xhr); } xhr.send(form); }
Upload progress tracking
XMLHttpRequest object. When transmitting data, there is a progress event to return progress information.
It is divided into upload and download
1) The downloaded progress event belongs to the XMLHttpRequest object
2) The uploaded progress event belongs to the XMLHttpRequest.upload object.
xhr.upload.onprogress =function(e){ console.log(e); console.log(e.loaded,e.total,Math.round(e.loaded/e.total*100)+"%") }
jq upload progress
$.ajax({ type: "POST", // Data submission type url: "https://www.520mg.com/ajax/file.php ", / / sending address data: formData, //send data async: true, // Asynchronous processData: false, //processData defaults to false. When it is set to true, jquery ajax will not serialize data when submitting, but directly use data contentType: false, //It needs to be set to false because it is a FormData object and the attribute enctype = "multipart / form data" has been declared xhr:function(){ var xhr = new XMLHttpRequest(); if(xhr.upload){ xhr.upload.addEventListener("progress",function(e){ console.log(e); }) } return xhr; } });
This is the end of today's sharing
I'm still a fashion, a salted fish programmer with a dream! Let's work together to create tomorrow's brilliance! If you like, give me a little attention,
Pay attention to fashion and don't get lost!
Remember to click three times and prohibit white whoring