1, Experimental purpose
- Understand and master the jump principle of transfer instruction
- Master the method of using call and ret instructions to realize subroutine, and understand and master its parameter transmission mode
- Understand and master 80 × 25 color character mode display principle
- Integrated application of addressing mode and assembly instructions to complete simple application programming
2, Experimental preparation
- Jump principle of transfer instruction
- Usage of assembly instructions jmp, loop, jcxz, call, ret, retf
3, Experimental results
Task1
assume cs:code, ds:data data segment x db 1, 9, 3 len1 equ $ - x y dw 1, 9, 3 len2 equ $ - y data ends code segment start: mov ax, data mov ds, ax mov si, offset x mov cx, len1 mov ah, 2 s1:mov dl, [si] or dl, 30h int 21h mov dl, ' ' int 21h inc si loop s1 mov ah, 2 mov dl, 0ah int 21h mov si, offset y mov cx, len2/2 mov ah, 2 s2:mov dx, [si] or dl, 30h int 21h mov dl, ' ' int 21h add si, 2 loop s2 mov ah, 4ch int 21h code ends end start
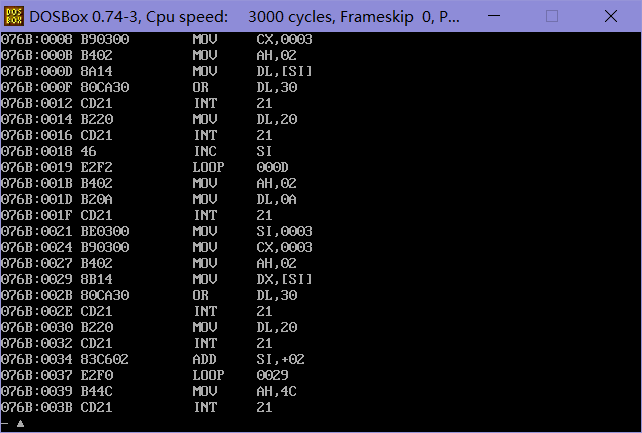
Question 1: line27, when the assembly instruction loop s1 jumps, it jumps according to the displacement. Check the machine code through debug disassembly and analyze the jump displacement? (the displacement value is answered in decimal) from the perspective of the CPU, explain how to calculate the offset address of the instruction after the jump label s1.
Question 2: line44, when the assembly instruction loop s2 jumps, it jumps according to the displacement. Check the machine code through debug disassembly and analyze the jump displacement? (the displacement value is answered in decimal) from the perspective of the CPU, explain how to calculate the offset address of the instruction after the jump label s2.
Task2
assume cs:code, ds:data data segment dw 200h, 0h, 230h, 0h data ends stack segment db 16 dup(0) stack ends code segment start: mov ax, data mov ds, ax mov word ptr ds:[0], offset s1 mov word ptr ds:[2], offset s2 mov ds:[4], cs mov ax, stack mov ss, ax mov sp, 16 call word ptr ds:[0] s1: pop ax call dword ptr ds:[2] s2: pop bx pop cx mov ah, 4ch int 21h code ends end start
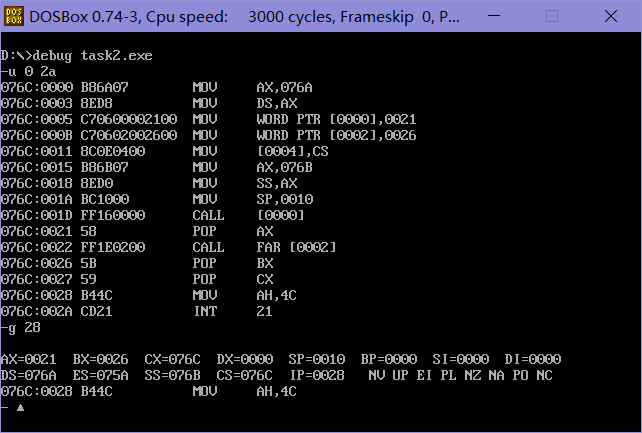
Task3
assume ds:data, cs:code data segment x db 99, 72, 85, 63, 89, 97, 55 len equ $- x data ends data1 segment db 10 data1 ends code segment start: mov ax, data mov ds, ax mov si, offset x mov cx, len s1: mov ah, 0 mov al, ds:[si] div byte ptr ds:[10h] call printNumber call printSpace inc si loop s1 mov ax, 4c00h int 21h printNumber: mov bx, ax or bl, 30h or bh, 30h mov ah, 2 mov dl, bl int 21h mov dl, bh int 21h ret printSpace: mov ah, 2 mov dl, ' ' int 21h ret code ends end start
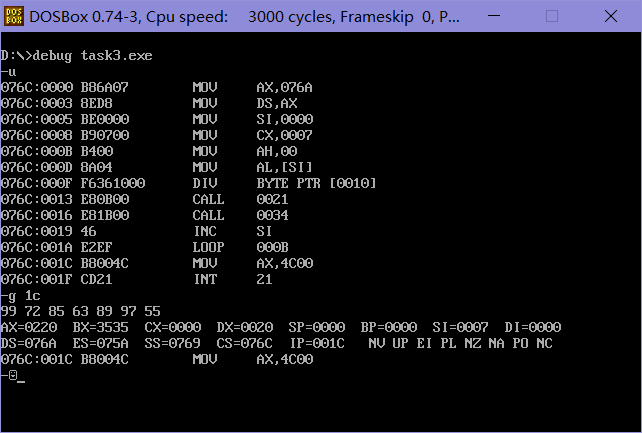
Task4
assume ds:data, cs:code data segment str db 'try' len equ $ - str data ends code segment start: mov ax,data mov ds,ax mov ax,0b800h mov es,ax mov bh,0 mov bl,2 call printStr mov bh,24 mov bl,4 call printStr mov ax,4c00h int 21h printStr: mov si,offset str mov al,0a0h mul bh mov di,ax mov cx,len mov bh,bl s: mov bl,[si] mov es:[di],bx inc si add di,2 loop s ret code ends end start
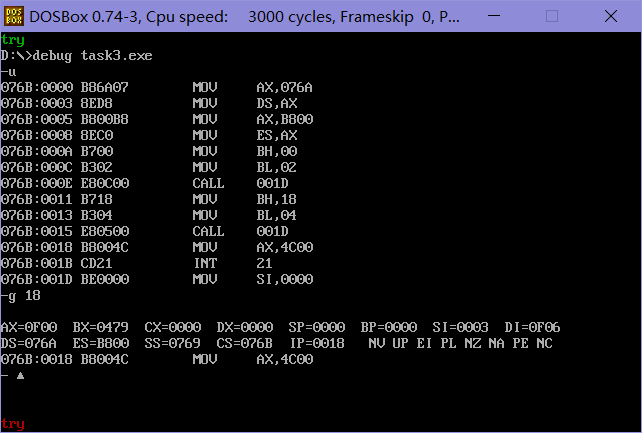
Task5
assume ds:data, cs:code data segment stu_no db '201983290408' len = $ - stu_no data ends code segment start: mov ax, data mov ds, ax mov ax, 0b800h mov es, ax call backgroundCol mov di, 3840 call printLine mov di, 3908 mov si, offset stu_no call printStu_no mov ax, 4c00h int 21h backgroundCol: mov ax,1700h mov di, 0 mov cx, 4000 s: mov es:[di], al mov es:[di+1], ah add di, 2 loop s ret printLine: mov cx, 50h mov ax, 172dh s1: mov es:[di], al mov es:[di+1], ah add di,2 loop s1 ret printStu_no: mov cx, len mov ah, 17h s2: mov al, [si] mov es:[di], al mov es:[di+1], ah inc si add di, 2 loop s2 ret code ends end start
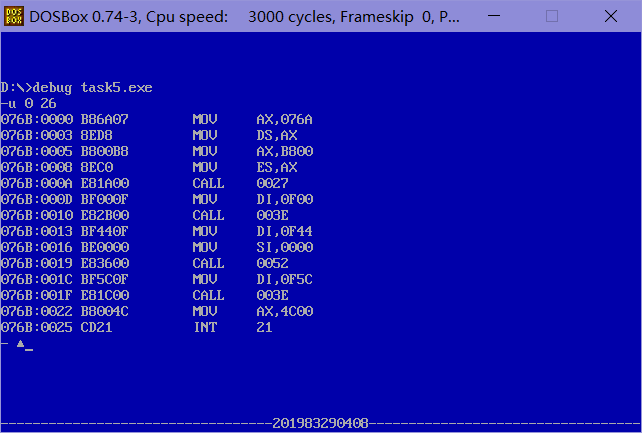
4, Experimental summary
Through this experiment, I understand and master the jump principle of transfer instruction, the method of using call and ret instruction to realize subroutine, the parameter transmission mode, and 80 × 25 color character mode display principle; comprehensive application of addressing mode and assembly instructions to complete simple application programming.