6, Prototype mode
- 1, Instances generated by copying
- 2, Sample program
- 3, Roles in Prototype mode-
- 4, Supplement
- 5, Source code and original file
Author: Gorit Date: 2021/1/13 Refer: graphic design mode Blog post published in 2021: 15 / 50
1, Instances generated by copying
In java, we can generate instances through the new keyword. We usually specify the class name for the instance generated in this way.
But sometimes, in the process of opening, we also need to generate instances without specifying the class name.
1.1 the following situations do not generate instances based on classes
In the following cases, we cannot generate instances based on classes, but generate new instances based on existing instances.
- There are so many kinds of objects that they cannot be integrated into one class.
- It is difficult to generate instances from classes.
- When decoupling the framework from the generated instance.
1.2 how to generate instances independent of classes?
The design pattern of generating instances based on instances instead of classes is called Prototype pattern
Prototype means prototype and * * model * *. In design pattern, it generates new instances according to instance prototype and instance model.
Now let's answer the questions raised by the title:
We can create a copy of the instance through clone. We will also use the knife to beat the use of the clone method and clonable interface.
2, Sample program
2.1 achieving objectives
The program function we want to achieve is to put the string into a box and display it or underline it
2.2 class and interface list
package | name | explain |
---|---|---|
framework | Product | The abstract method use and createClone interface are declared |
framework | Manager | Call the createClone method to copy the instance class |
nothing | MessageBox | Put the string into the box and display it, and implement the use method and createClone method |
nothing | UnderlinePen | The class that underlines the string and displays it implements the use and createClone methods |
nothing | Main | Class for testing program behavior |
The Product interface and Message class belong to the framework package and are responsible for copying instances. Although the Manager class will call the createClone method, for which to copy. I don't know. However, as long as the class that implements the Product interface calls its createClone method, a new instance can be copied.
MessageBox class and UnderlinePen class are two classes that implement the Product interface. Only by "registering" these two classes into the Manager class, you can copy new instances at any time.
2.3 code implementation
Product
package Prototype.framework; // Inheriting the copied interface, clonable calls the object by calling clone public interface Product extends Cloneable{ public abstract void use(String s); // How to use it and give it to subclasses to implement public abstract Product createClone(); }
Manager
package Prototype.framework; import java.util.*; /** * Copy instances using the product interface */ public class Manager { // Save the correspondence between the name and the instance private HashMap showcase = new HashMap(); public void register(String name, Product proto) { showcase.put(name,proto); } public Product create(String protoname) { Product p = (Product) showcase.get(protoname); return p.createClone(); } }
MessageBox
package Prototype; import Prototype.framework.Product; public class MessageBox implements Product { private char decochar; public MessageBox(char decochar) { this.decochar = decochar; } public void use(String s) { int length = s.getBytes().length; for (int i=0;i<length+4;i++) { System.out.print(decochar); } System.out.println(""); System.out.println(decochar + " " + s + " " + decochar); for (int i=0;i<length+4;i++) { System.out.print(decochar); } System.out.println(""); } public Product createClone() { Product p = null; try { p = (Product) clone(); } catch (CloneNotSupportedException e) { e.printStackTrace(); } return p; } }
UnderlinePen
package Prototype; import Prototype.framework.Product; public class UnderlinePen implements Product { private char ulchar; public UnderlinePen(char ulchar) { this.ulchar = ulchar; } public void use(String s) { int length = s.getBytes().length; System.out.println("\"" + s + "\""); System.out.println(" "); for (int i=0;i< length;i++) { System.out.print(ulchar); } System.out.println(""); } public Product createClone() { Product p = null; try { p = (Product) clone(); } catch (CloneNotSupportedException e) { e.printStackTrace(); } return p; } }
Main
package Prototype; import Prototype.framework.Manager; import Prototype.framework.Product; public class Main { public static void main(String[] args) { // prepare Manager manager = new Manager(); UnderlinePen upen = new UnderlinePen('~'); MessageBox mbox = new MessageBox('*'); MessageBox sbox = new MessageBox('/'); manager.register("strong msg",upen); manager.register("warning box",mbox); manager.register("slash box",sbox); // generate Product p1 = manager.create("strong msg"); p1.use("Hello World"); Product p2 = manager.create("warning box"); p2.use("Hello World"); Product p3 = manager.create("slash box"); p3.use("Hello World"); } }
Execution effect
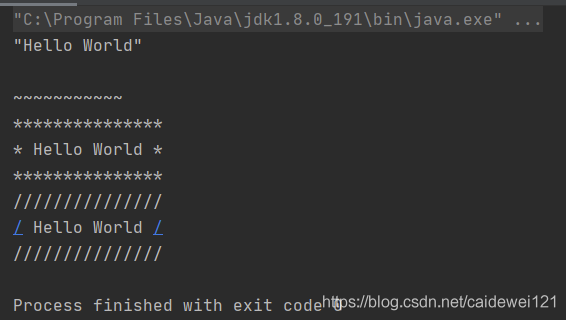
3, Roles in Prototype mode-
- Prototype
The Product role is responsible for defining the method used to copy an existing instance to generate a new instance. The Product interface plays this role
- Concrete prototype
The ConcretePrototype role is responsible for implementing the method of copying an existing instance and generating a new instance. In the above program, the MessageBox class and the UnderlinePen class play this role
- Client (user)
The Client role is responsible for generating new instances using the method of copying instances. In the example, the Message class plays this role.
4, Supplement
4.1 relevant design modes
- Flyweight mode (the same instance can be used in different places)
- Memento mode (you can save the current instance state to realize snapshot and Undo functions)
- Composite pattern and Decorator pattern (dynamically create instances of complex structures. Here, Prototype pattern can be used to quickly generate instances)
- Command mode (Prototype can be used when the commands in this mode need to be copied)
4.2 clone method and java.lang.Cloneable interface
To use the clone method to copy instances, you must implement the clonable interface, otherwise clonnotsupportedexception will appear
The java.lang package is introduced by default. Therefore, it is not necessary to introduce the Java. Lang package to call the clone() method
4.3 where is the clone () method defined?
The clone() method is defined in java.lang.Object because the Object class is the parent class of all Java classes. Therefore, all classes inherit the Object class by default.
5, Source code and original file
Links to the original text - and integration of other series of articles source code