1. Introduction
For the front-end hidden elements, it has always been the invisible killer of selenium's automatic positioning elements. When the script runs to the hidden elements, it will report all kinds of errors, but there is no way to avoid this hidden drop-down menu, so it's a headache. This article is only to exchange the automatic positioning processing methods of hidden elements and brother Hong's own shallow opinions.
2. What are hidden elements
Hidden elements must be familiar to friends or children's shoes who are familiar with the front end or HTML. The attributes of elements are hidden and displayed, mainly type="hidden" and style="display: none;" Attribute to control. Of course, there are other methods to control. Brother Hong will not explain it in detail here. Interested friends or children's shoes can check the data by themselves. What are hidden elements? Hidden elements are hidden through the attribute value hidden="hidden". If this appears in the front-end code, it means that the element has been hidden. As we all know, if the element is hidden, there is no way to operate. The so-called operations are input, click and empty these basic elements. If the element is operated by clicking in selenium, an error will be reported and the element information is not found. As described earlier, hidden elements can only be located, but there is no way to operate.
1. There are two input boxes and a login button, which are originally displayed, as shown in the following figure:
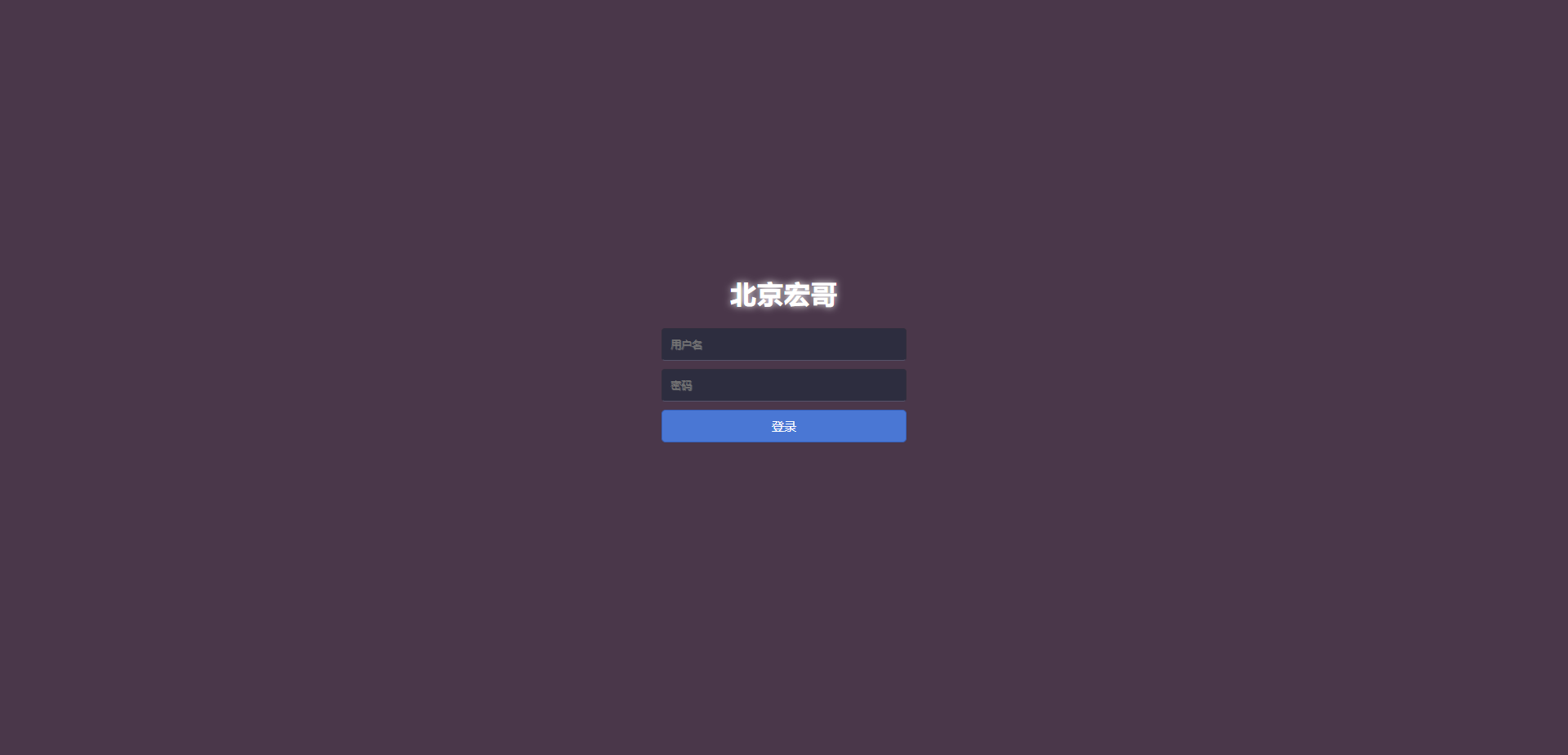
2. Next, hide it in the login element attribute. The code is as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Login</title> <link rel="stylesheet" type="text/css" href="Login.css"/> </head> <body> <div id="login"> <h1>Beijing Hongge</h1> <form method="post"> <input type="text" required="required" placeholder="user name" name="u"></input> <input type="password" required="required" placeholder="password" name="p"></input> <button id="bjhg" class="but" type="submit" style="display: none;" onclick="display_alert()">Sign in</button> </form> </div> </body> <script type="text/javascript"> function display_alert(){ alert("Please pay attention to the official account: Beijing, Hong brother.") } </script> </html>
In this way, the login button will not be displayed, as shown in the following figure:
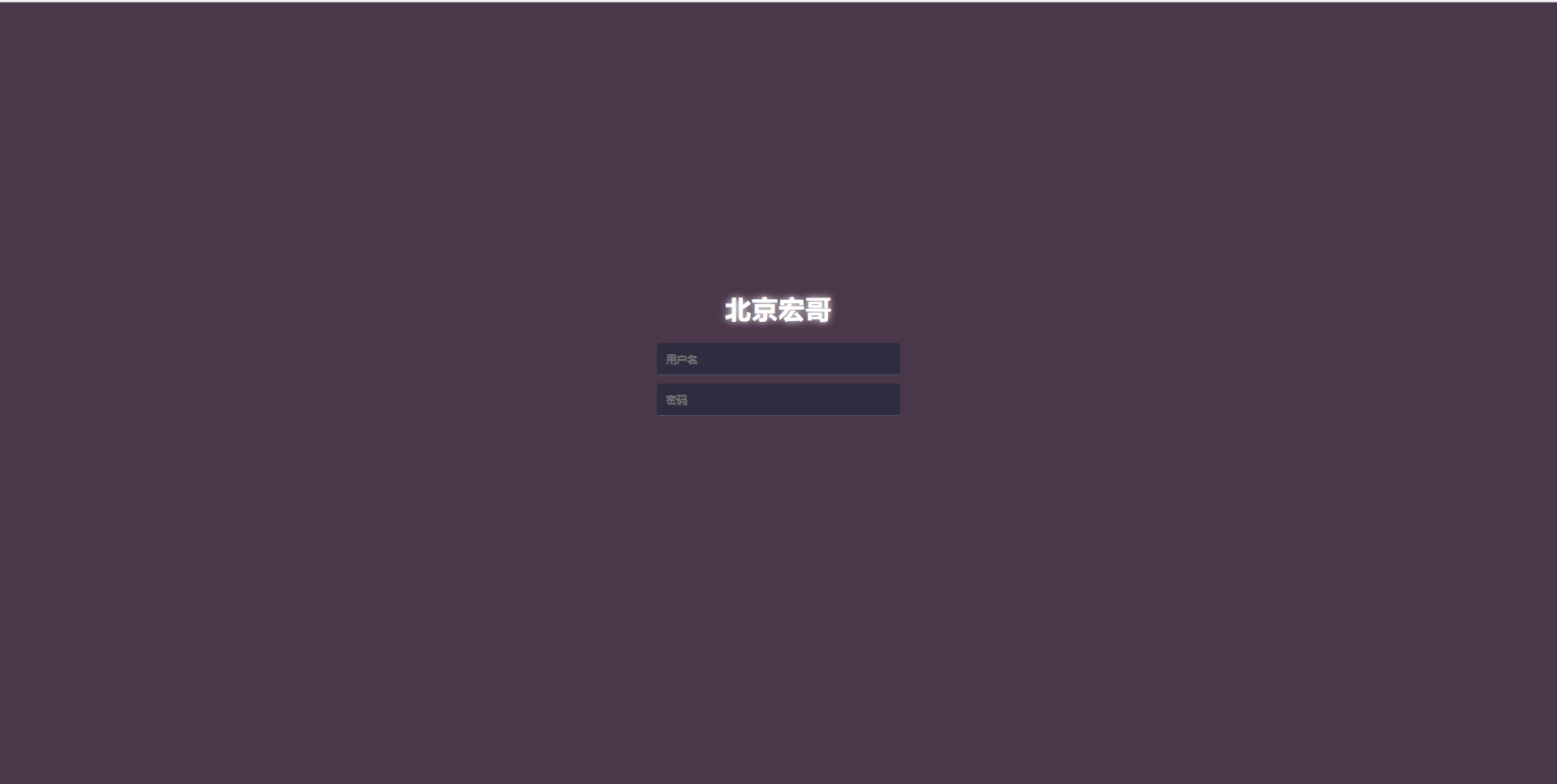
3. Locate hidden elements
Brother Hong said earlier that there is no difference between locating hidden elements and ordinary elements. Next, let's verify whether we can locate them? In fact, it has been verified in the previous article. Maybe the little friends or children's shoes didn't notice or notice, so brother Hong will verify it again here.
3.1 reference code
package lessons; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; /** * @author Beijing - Hongge * * @Official account: Beijing, Hong brother * * <Teach you by hand series tips (49) - java+ selenium automated testing - hidden element positioning and operation (detailed tutorial) * * 2021 November 24 */ public class testHidden { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", ".\\Tools\\chromedriver.exe"); WebDriver driver =null; try { driver = new ChromeDriver(); driver.get("file:///C:/Users/DELL/Desktop/test/hidden/login.html"); driver.manage().window().maximize(); //Locate hidden elements by id WebElement loginButton = driver.findElement(By.id("bjhg")); System.out.println("Print element information:"+loginButton); //Get element properties System.out.println(loginButton.getAttribute("name")); //Determine whether the element is hidden System.out.println(loginButton.isDisplayed()); } catch (Exception e) { e.printStackTrace(); } finally{ System.out.println("end of execution,Close browser"); driver.quit(); } } }
3.2 operation results
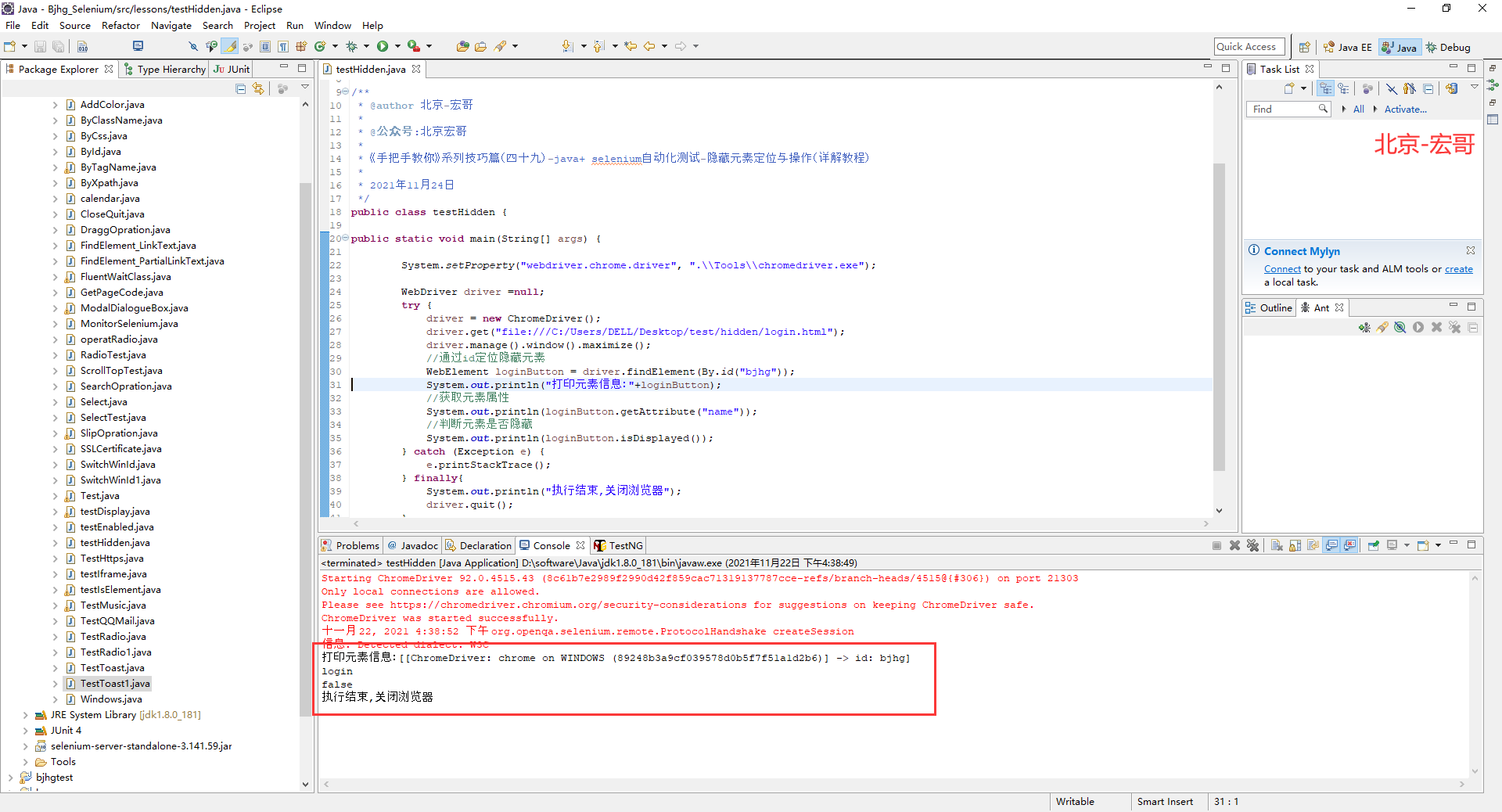
It can be seen from the running results that the hidden elements are actually located by the ordinary positioning method, which is no different from the positioning of ordinary elements! Don't think it has anything special. It needs special positioning methods.
4. Operate hidden elements
As we all know, the selenium operation method is carried out by simulating the human operation method, so the elements can not be seen, so there is no so-called operation not to operate. If we really want to operate, we can use the JS syntax introduced by brother Hongge, because JS syntax directly operates the front-end code, and hidden elements exist in HTML code, Hidden elements are mainly invisible to front-end pages.
Hidden elements can be located normally, but they cannot be operated (locating elements and operating elements are two different things, which are sometimes unclear to many beginners or interviewers). The operating elements are click, clear and sendkeys.
//Click Hide login box WebElement loginButton = driver.findElement(By.id("bjhg")); loginButton.click();
Hidden elements will throw an exception "org. Openqa. Selenium. ElementNotInteractableException: element not interactive" with the click() method. This error message means that the element is invisible and cannot be operated. Similarly, if the input box on the "login" button is hidden, the operation of "Sendkeys" will also report "ElementNotInteractableException".
5.JS operation hidden elements
Selenium cannot operate hidden elements (but can locate them normally). This is the design of the framework itself. If you have to operate hidden elements, use js methods. Selenium provides an entry to execute JS scripts. JS is different from selenium. Only some elements on the page (in dom) can operate normally. Next, try JS!
Then continue to improve the code that can be located but cannot be operated.
5.1 code design
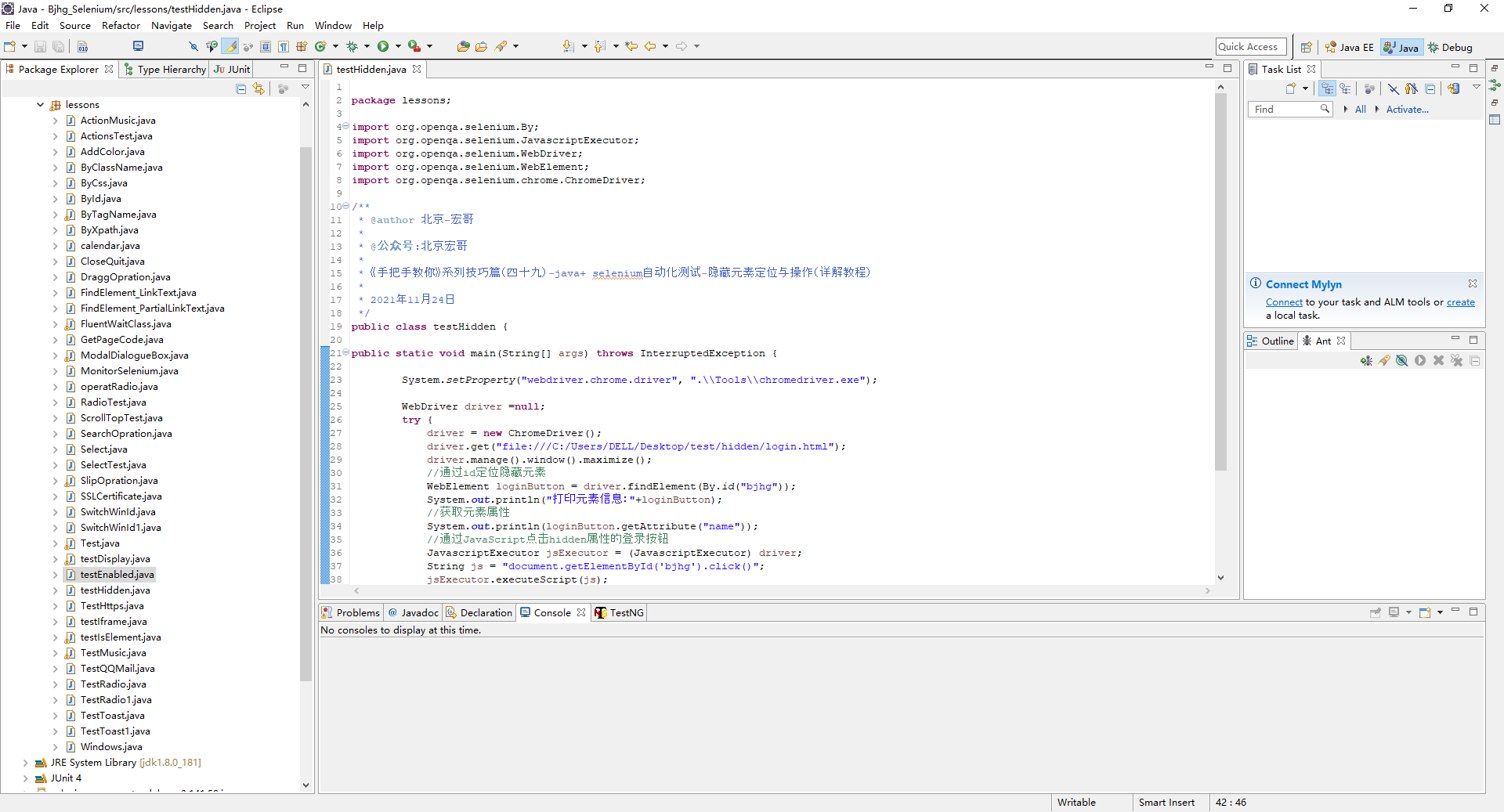
5.2 reference code
package lessons; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; /** * @author Beijing - Hongge * * @Official account: Beijing, Hong brother * * <Teach you by hand series tips (49) - java+ selenium automated testing - hidden element positioning and operation (detailed tutorial) * * 2021 November 24 */ public class testHidden { public static void main(String[] args) throws InterruptedException { System.setProperty("webdriver.chrome.driver", ".\\Tools\\chromedriver.exe"); WebDriver driver =null; try { driver = new ChromeDriver(); driver.get("file:///C:/Users/DELL/Desktop/test/hidden/login.html"); driver.manage().window().maximize(); //Locate hidden elements by id WebElement loginButton = driver.findElement(By.id("bjhg")); System.out.println("Print element information:"+loginButton); //Get element properties System.out.println(loginButton.getAttribute("name")); //Click the login button of the hidden attribute through JavaScript JavascriptExecutor jsExecutor = (JavascriptExecutor) driver; String js = "document.getElementById('bjhg').click()"; jsExecutor.executeScript(js); } catch (Exception e) { e.printStackTrace(); } finally{ System.out.println("end of execution,Close browser"); Thread.sleep(5000); driver.quit(); } } }
5.3 operation code
1. Run the code, right-click run as - > java appliance, and the console will output, as shown in the following figure:
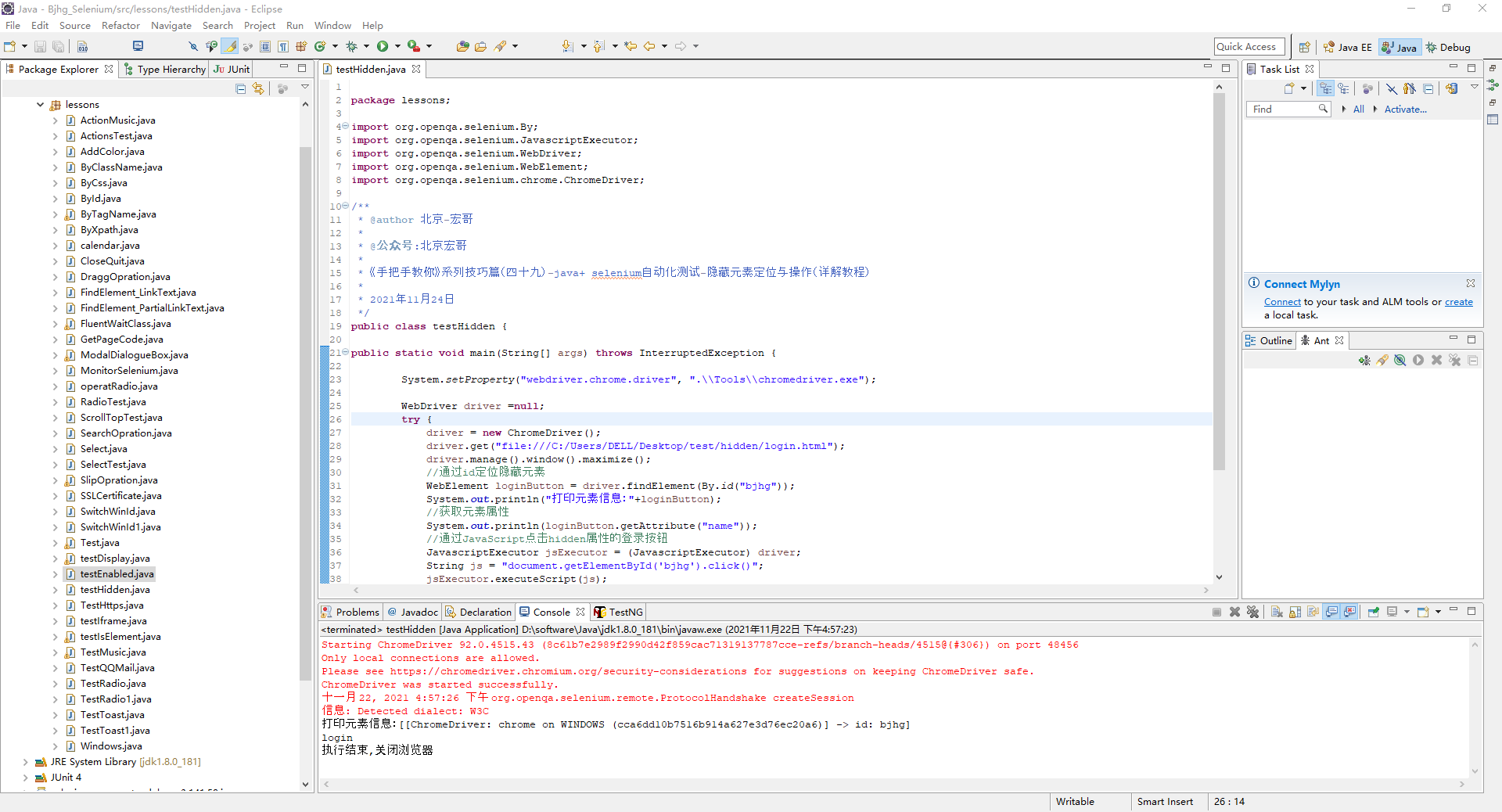
2. After running the code, the action of the browser on the computer side is shown in the following small video:
After running, you will find the normal click of the page and pop up to the official account of the macro brother.
6. Summary
6.1 simplified code
The possible method Baidu found is to remove the hidden attribute with JS first, and then operate selenium. This is a bit superfluous. Since you can already use js, why not click directly at one time? Moreover, brother Hong found that this method was also explained before, which was a little "taking off his pants". Here, brother Hong, correct it and put it in place in one step!
6.2 interview questions
If the interviewer wants to ask is to operate hidden elements after positioning, in essence, this question is meaningless. The purpose of web automation is to simulate people's normal behavior. If you can't see an element on the page, you can't operate it manually, can you? People can't operate, so what's the meaning of automation? So this is just to simply investigate the interviewer's ability to deal with problems. It's not practical! (interview to build a plane, go in and screw in) since the interviewer asked so, find a way to give a good impression! Remember not to answer the interviewer, otherwise your interview will be cold!!!