What will you learn
- How to parse json objects in fluent
- How to generate code using the auto generation tool
- How to test your data
Start json deserialization
Step 1: create mock data
In the actual development process, we may modify some of the previous code. When our code is complex and large enough, we need to use unit tests to ensure that the newly added code will not affect the previously written code. The server data often changes, so the first step is to create our mock data.
GitHub / Hacker News data is used here( github.com/HackerNews/...)
abstract class JsonString{ static final String mockdata = ''' { "by" : "dhouston", "descendants" : 71, "id" : 8863, "kids" : [ 8952, 9224, 8917, 8884, 8887, 8943, 8869, 8958, 9005, 9671, 8940, 9067, 8908, 9055, 8865, 8881, 8872, 8873, 8955, 10403, 8903, 8928, 9125, 8998, 8901, 8902, 8907, 8894, 8878, 8870, 8980, 8934, 8876 ], "score" : 111, "time" : 1175714200, "title" : "My YC app: Dropbox - Throw away your USB drive", "type" : "story", "url" : "http://www.getdropbox.com/u/2/screencast.html" }'''; }
Step 2: add dependency
In pubspec Add the following dependencies to yaml
dependencies: # Your other regular dependencies here json_annotation: ^1.2.0 dev_dependencies: # Your other dev_dependencies here build_runner: ^0.10.2 json_serializable: ^1.5.1
Three dependencies need to be added here: json_annotation, build_runner and json_serializable.
Note that the yaml configuration file has strict indentation requirements. The following build_runner and json_serializable should be the same as fluent_ Test level, don't write it in fluent_ After the test is indented, it will think that these two are flutters_ Test subset directory!
Because many friends have encountered problems in this step, the source code is posted here
[external chain picture transfer failed. The source station may have anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-m12sx9my-1630926464012)( https://user-gold-cdn.xitu.io/2018/7/30/164ebe0cfdaa64f9?imageView2/0/w/1280/h/960/ignore -error/1)]
Step 3: create an entity class based on json
We have written a dart entity class based on the json data above
class Data{ final String by; final int descendants; final int id; final List<int> kids; final int score; final int time; final String title; final String type; final String url; Data({this.by, this.descendants, this.id, this.kids, this.score, this.time, this.title, this.type, this.url}); }
Here we use the dart syntax to create the constructor. Please refer to( www.dartlang.org/guides/lang...).
Step 4: Associate entity class files
We need to associate the generated file in our entity class.
import 'package:json_annotation/json_annotation.dart'; part 'data.g.dart'; @JsonSerializable() class Data { final String by; final int descendants; final int id; final List<int> kids; final int score; final int time; final String title; final String type; @JsonKey(nullable: false) final String url; Data({this.by, this.descendants, this.id, this.kids, this.score, this.time, this.title, this.type, this.url});
Just finished writing data g. Dart will report an error, which is normal! Because we haven't generated the parsing file yet
- In order for the entity class file to find the generated file, we need part 'data g.dart’.
Step 5: generate Json parsing file
Dangdang! It's the highlight from here!!
If we want to use JsonSerializable to generate code, we must add the annotation @ JsonSerializable() before the entity class that needs to generate code, and to use this annotation, we must introduce json_annotation/json_annotation.dart, this bag.
import 'package:json_annotation/json_annotation.dart'; @JsonSerializable() class Data{ final String by; final int descendants; final int id; final List<int> kids; final int score; final int time; final String title; final String type; final String url; Data({this.by, this.descendants, this.id, this.kids, this.score, this.time, this.title, this.type, this.url}); }
It should be noted here that the dart file name in the fluent encoding specification is uniformly lowercase, which can avoid many problems. ok, so the entity class is created.
So the question is, how should we generate code?
Remember the build we just added_ Runner's dependence? At this time, we need it to help.
build_runner
build_runner is an external package provided by the dart team to generate dart code files.
We run fluent packages pub run build in the directory of the current project_ runner build
[external chain picture transfer failed. The source station may have anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-54cn0tet-1630926464014)( https://user-gold-cdn.xitu.io/2018/7/30/164eb50624fba89e?imageView2/0/w/1280/h/960/ignore -error/1)]
After running successfully, we should be able to find a new file under the entity class
[external chain picture transfer failed. The source station may have anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-qd6wwfij-1630926464015)( https://user-gold-cdn.xitu.io/2018/7/30/164eb53d3b4b021c?imageView2/0/w/1280/h/960/ignore -error/1)]
This data g. Dart is build_ The runner parses the file according to the json generated by JsonSerializable. Let's take a look at the generated dart file
// GENERATED CODE - DO NOT MODIFY BY HAND part of 'data.dart'; // ************************************************************************** // JsonSerializableGenerator // ************************************************************************** Data _$DataFromJson(Map<String, dynamic> json) { return Data( by: json['by'] as String, descendants: json['descendants'] as int, id: json['id'] as int, kids: (json['kids'] as List)?.map((e) => e as int)?.toList(), score: json['score'] as int, time: json['time'] as int, title: json['title'] as String, type: json['type'] as String, url: json['url'] as String); } Map<String, dynamic> _$DataToJson(Data instance) => <String, dynamic>{ 'by': instance.by, 'descendants': instance.descendants, 'id': instance.id, 'kids': instance.kids, 'score': instance.score, 'time': instance.time, 'title': instance.title, 'type': instance.type, 'url': instance.url };
Comrades, please pay attention to the comment "/ / GENERATED CODE - DO NOT MODIFY BY HAND" at the top of this code. You must not generate files by handwriting, ha ha ha.
This code generates a part of the entity class library. In the old version of part, there is a mixin of the abstract entity class. In dart, mixin based inheritance is used to solve the limitation of single inheritance.
The new version claims two methods, fromjason and tojason. I believe you can guess what they can do from the name.
[students interested in part can refer to https://juejin.im/post/6844903649617936392#heading-8 Dart | analyze the import and split of database in dart.
Students interested in mixin can( www.dartlang.org/guides/lang... )Learn more about mixin.]
- _$ Datafromjason: it receives a map: Map < string, dynamic >, and maps the values in the map to the entity class objects we need. We can use this method to convert the map containing json data into the entity class object we need.
- _$ DataToJson: Map the object calling this method directly into a Map according to the field.
Both of these are private methods. part allows two files to share the scope and namespace, so we need to expose the generated methods to the outside.
Then we go back to the entity class and add the fromJson and toJson methods.
import 'package:json_annotation/json_annotation.dart'; # Resource sharing * **The latest interview topics for large factories** This question bank has a lot of content, except for some popular technical interview questions, such as Kotlin,database Java Virtual machine interview questions, array, Framework ,Mixed cross platform development, etc 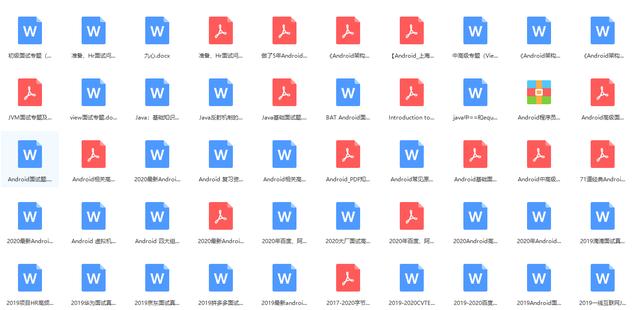 * **Corresponding to the map Android Advanced system learning video for senior engineer** Recently popular, NDK,Thermal repair, MVVM,Source code and a series of system learning videos! 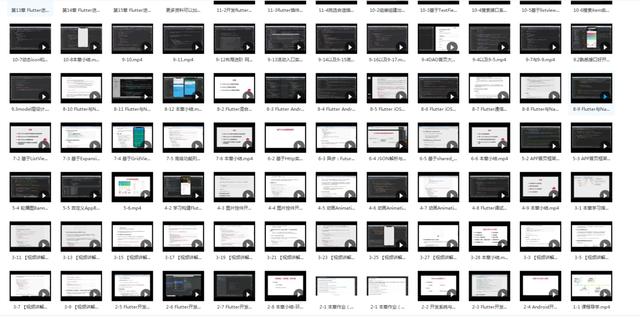 Some popular technical interview questions, such as Kotlin,database Java Virtual machine interview questions, array, Framework ,Mixed cross platform development, etc [External chain picture transfer...(img-xLbsXtpy-1630926464017)] * **Corresponding to the map Android Advanced system learning video for senior engineer** Recently popular, NDK,Thermal repair, MVVM,Source code and a series of system learning videos! [External chain picture transfer...(img-pZfqEqUB-1630926464018)] **[CodeChina Open source projects:< Android Summary of study notes+Mobile architecture video+Real interview questions for large factories+Project practice source code](https://codechina.csdn.net/m0_60958482/android_p7)**