import java.lang.FunctionalInterface; // this is functional interface @FunctionalInterface interface MyInterface{ // abstract method double getPiValue(); } public class Main { public static void main( String[] args ) { // declare a reference to MyInterface MyInterface ref; // lambda expression ref = () -> 3.1415; System.out.println("Value of Pi = " + ref.getPiValue()); } }
Output:
Value of Pi = 3.1415
In the above example,
We have created a functional interface called MyInterface. It contains an abstract method called getPiValue()
In the main class, we declare a reference to MyInterface. Note that we can declare a reference to an interface, but we cannot instantiate an interface. namely,
// it will throw an error MyInterface ref = new myInterface(); // it is valid MyInterface ref;
Then, we specify a lambda expression for the reference.
ref = () -> 3.1415;
Finally, we call the method getPiValue () using the reference interface
System.out.println("Value of Pi = " + ref.getPiValue());
Lambda expression with parameters
So far, we have created a lambda expression without any parameters. However, like methods, lambda expressions can have parameters. for example Written examination of job interview
(n) -> (n%2)==0
Here, the variable n in parentheses is the parameter passed to the lambda expression. The lambda body accepts the parameter and checks whether it is even or odd.
Example 4: using lambda expressions with parameters
@FunctionalInterface interface MyInterface { // abstract method String reverse(String n); } public class Main { public static void main( String[] args ) { // declare a reference to MyInterface // assign a lambda expression to the reference MyInterface ref = (str) -> { String result = ""; for (int i = str.length()-1; i >= 0 ; i--) result += str.charAt(i); return result; }; // call the method of the interface System.out.println("Lambda reversed = " + ref.reverse("Lambda")); } }
Output:
Lambda reversed = adbmaL
##General function interface
So far, the function interface we have used accepts only one type of value. for example
@FunctionalInterface interface MyInterface { String reverseString(String n); }
The above function interface only accepts strings and returns strings. However, we can make the function interface generic to accept any data type. If you are not sure about generics, visit Java generics.
Example 5: generic function interfaces and Lambda expressions
// GenericInterface.java @FunctionalInterface interface GenericInterface<T> { // generic method T func(T t); } // GenericLambda.java public class Main { public static void main( String[] args ) { // declare a reference to GenericInterface // the GenericInterface operates on String data // assign a lambda expression to it GenericInterface<String> reverse = (str) -> { String result = ""; for (int i = str.length()-1; i >= 0 ; i--) result += str.charAt(i); return result; }; System.out.println("Lambda reversed = " + reverse.func("Lambda")); // declare another reference to GenericInterface // the GenericInterface operates on Integer data // assign a lambda expression to it GenericInterface<Integer> factorial = (n) -> { int result = 1; for (int i = 1; i <= n; i++) result = i * result; return result; }; System.out.println("factorial of 5 = " + factorial.func(5)); } }
Output:
Lambda reversed = adbmaL factorial of 5 = 120
In the above example, we created a generic function interface called GenericInterface. It contains a generic method called func ().
Here, in the main class,
-
Genericinterface < string > reverse - creates a reference to the interface. The interface now operates on string type data.
-
Genericinterface < integer > factorial - creates a reference to the interface. In this example, the interface operates on integer data.
Lambda expressions and streampapi
New java util. The stream package has been added to JDK8, which allows java developers to perform collections such as search, filter, map, reduce, or action lists.
For example, we have a data stream (in this case, a list of strings), where each string is a combination of country name and country location. Now we can process these data streams and retrieve places only from Nepal.
For this purpose, we can perform batch operations in stream through the combination of stream API and Lambda expression.
Example 6: demonstrates using lambdas with the stream API
import java.util.ArrayList; import java.util.List; public class StreamMain { // create an object of list using ArrayList static List<String> places = new ArrayList<>(); // preparing our data public static List getPlaces(){ // add places and country to the list places.add("Nepal, Kathmandu"); places.add("Nepal, Pokhara"); places.add("India, Delhi"); places.add("USA, New York"); places.add("Africa, Nigeria"); return places; } public static void main( String[] args ) { List<String> myPlaces = getPlaces(); System.out.println("Places from Nepal:"); // Filter places from Nepal myPlaces.stream() .filter((p) -> p.startsWith("Nepal")) .map((p) -> p.toUpperCase()) .sorted() .forEach((p) -> System.out.println(p)); } }
Output:
Write at the end
There is also a collection of JAVA core knowledge points (PDF): JVM, JAVA collection, JAVA multithreading concurrency, JAVA foundation, Spring principle, microservice, Netty and RPC, network, log, Zookeeper, Kafka, RabbitMQ, Hbase, MongoDB, Cassandra, design pattern, load balancing, database, consistency hash, JAVA algorithm, data structure, encryption algorithm, distributed cache, Hadoop, Spark, Storm, YARN, machine science Xi, Cloud Computing
§ -> p.toUpperCase())
.sorted() .forEach((p) -> System.out.println(p)); }
}
Output: # Write at the end **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** There's another one JAVA Sorting of core knowledge points( PDF): **JVM,JAVA Gather, JAVA Multithreading concurrency, JAVA Basics, Spring Principles, microservices, Netty And RPC**,Network, log, Zookeeper,Kafka,RabbitMQ,Hbase,**MongoDB,Cassandra,Design pattern, load balancing, database, consistency hash, JAVA Algorithm, data structure, encryption algorithm, distributed cache**,Hadoop,Spark,Storm,YARN,Machine learning, cloud computing... 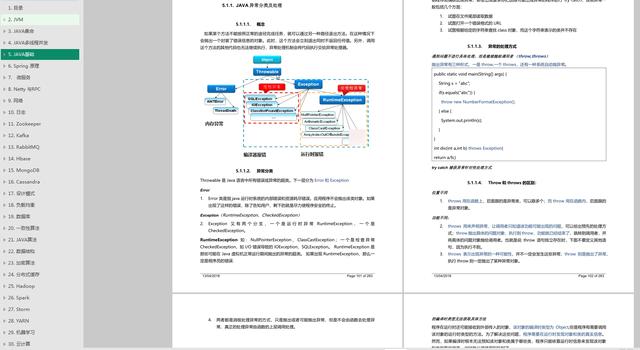