<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-hystrix</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-config</artifactId> </dependency> </dependencies> <dependencyManagement> <dependencies> <dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId> <exclusions> <exclusion> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-ribbon</artifactId> </exclusion> </exclusions> </dependency> </dependencies> </dependencyManagement> <build> <finalName>gateway-${system.version}</finalName> </build>
### 2. Profiles
spring:
application:
name: gateway
cloud:
nacos: discovery: server-addr: 127.0.0.1:8848 config: server-addr: 127.0.0.1:8848 file-extension: yml ext-config: - data-id: datasource-share-config.yml group: SHARE_GROUP refresh: true - data-id: log-share-config.yml group: SHARE_GROUP refresh: true
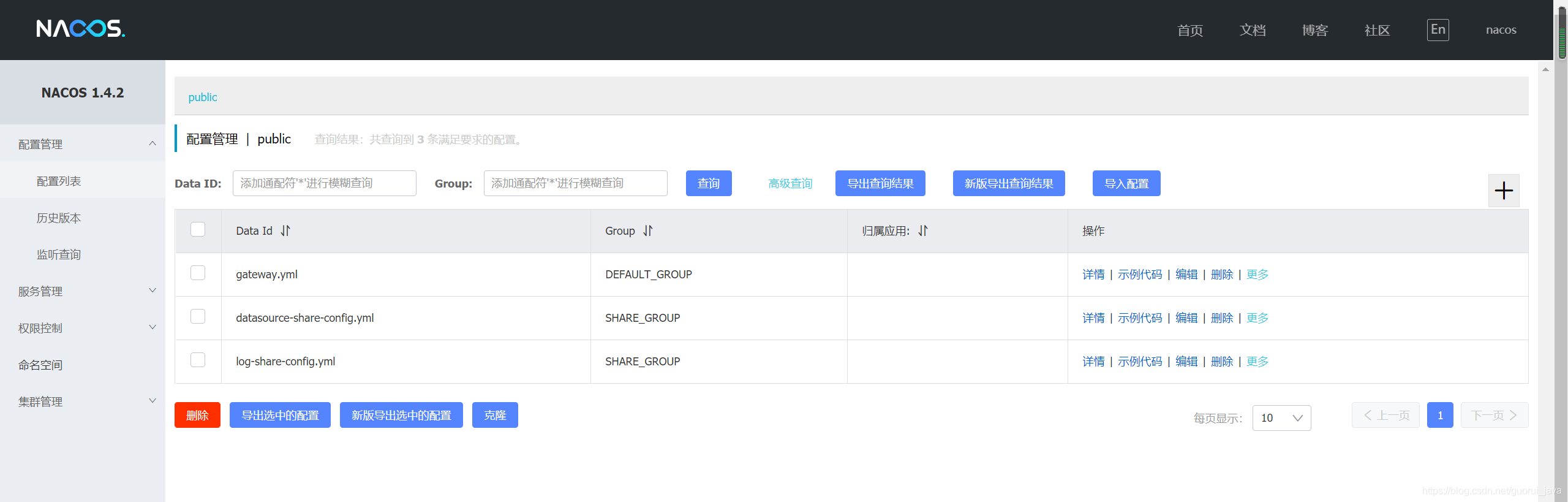 (1)gateway.ymlÂ
server:
port: 8080
spring:
application:
name: gateway version: 1.0.0
cloud:
gateway: discovery: locator: enabled: true lowerCaseServiceId: true filters: - StripPrefix=1 routes: - id: management uri: lb:management # Server-side service_id predicates: - Path=/management/** filters: - name: Hystrix args: name: fallbackcmd fallbackUri: forward:/defaultFallback - id: demo uri: lb://demo predicates: - Path=/demo/**
hystrix:
command:
default: execution: isolation: strategy: SEMAPHORE thread: timeoutInMilliseconds: 1500
shareSecurityContext: true
config:
scheduleThreadPool: 5
restTemplateTimeout: 3000
setting:
loginAccessPath: /permission
login:
tokenCheckFrequency: 600000
(2)datasource-share-config.yml
spring:
datasource:
url: jdbc:mysql://localhost:3306/blue?serverTimezone=UTC driverClassName: com.mysql.cj.jdbc.Driver username: root password: root
mybatis:
typeAliasesPackage: com.guor.*.bean.**
mapperLocations: classpath*:/com/guor//dao/mapping/*Mapper.xml
configuration:
map-underscore-to-camel-case: true
(3)log-share-config.yml
logging:
path: logs
level:
root: info com.panasonic.mes: debug com.panasonic.mes.editor: debug com.alibaba.nacos.client.naming: warn
file:
max-size: 20MB max-history: 30
pattern:
file: "%d{${LOG_DATEFORMAT_PATTERN:yyyy-MM-dd HH:mm:ss.SSS}} ${LOG_LEVEL_PATTERN:%5p} ${PID:- } --- [%15.15t] %-40.40logger{39} [%5.5line] : %m%n${LOG_EXCEPTION_CONVERSION_WORD:%wEx}" console: "%d{${LOG_DATEFORMAT_PATTERN:yyyy-MM-dd HH:mm:ss.SSS}} %clr(${LOG_LEVEL_PATTERN:%5p}) %clr(${PID:- }){magenta} --- %clr([%15.15t]){faint} %clr(%-40.40logger{39}){cyan} %clr([%5.5line]){cyan} : %m%n${LOG_EXCEPTION_CONVERSION_WORD:%wEx}"
### 3. Startup Class
package com.guor;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.context.config.annotation.RefreshScope;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication(exclude = DataSourceAutoConfiguration.class)
@EnableDiscoveryClient
@EnableScheduling
@RefreshScope
public class GatewayApplication {
public static void main(String[] args) { SpringApplication.run(GatewayApplication.class, args); System.out.println("hello world"); }
}
<?xml version="1.0" encoding="UTF-8"?>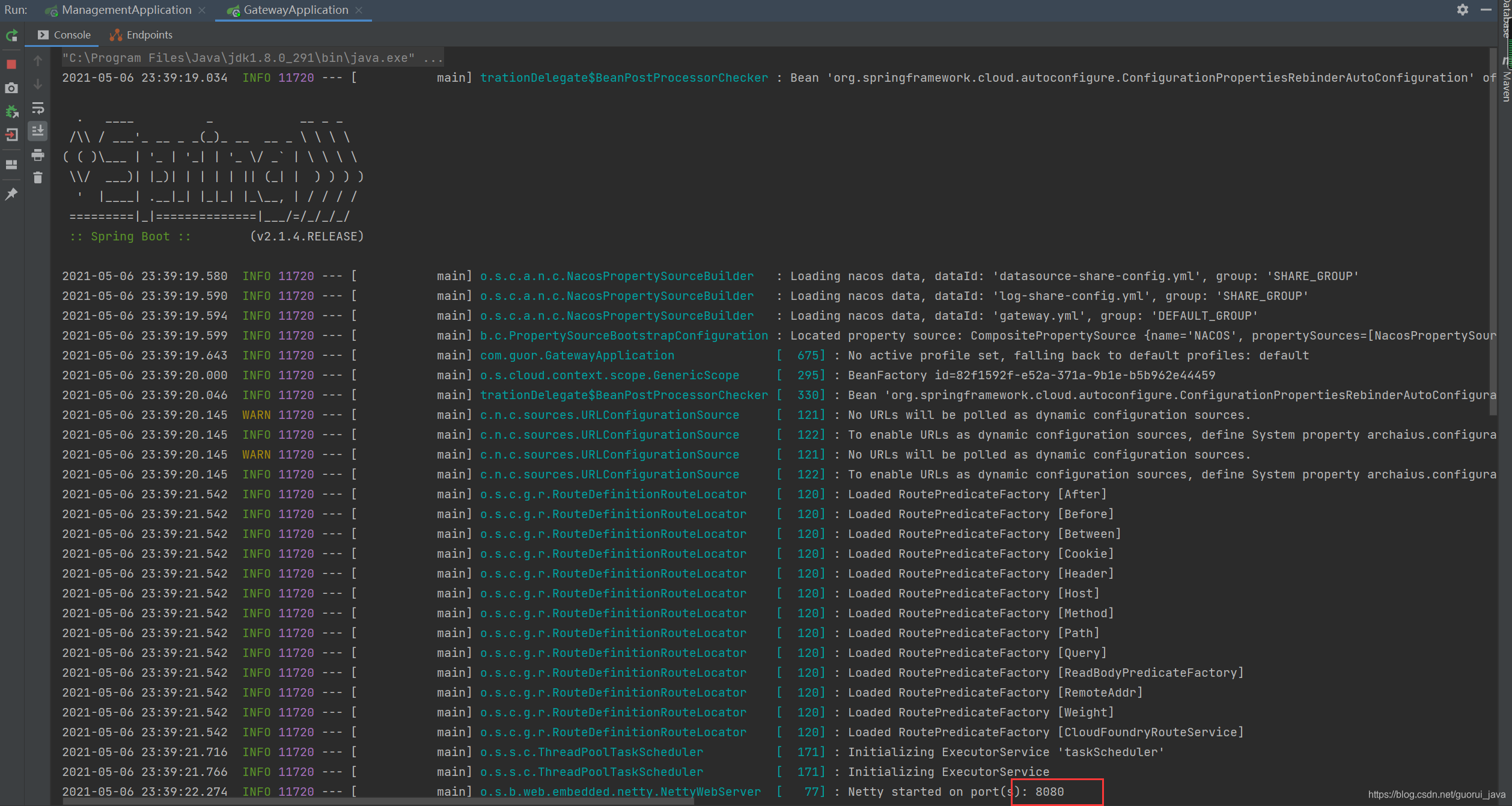 4. Creation management Management module ------------------ ### 1. pom files
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <parent> <artifactId>GooReeyProject</artifactId> <groupId>com.guor</groupId> <version>1.0-SNAPSHOT</version> </parent> <modelVersion>4.0.0</modelVersion> <artifactId>03management</artifactId> <properties> <maven.compiler.source>8</maven.compiler.source> <maven.compiler.target>8</maven.compiler.target> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.0.1</version> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-config</artifactId> </dependency> <!--MySQL rely on --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-jdbc</artifactId> </dependency> </dependencies> <build> <finalName>management-${system.version}</finalName> </build>
### 2. Profiles
spring:
application:
name: management
cloud:
nacos: discovery: server-addr: 127.0.0.1:8848 config: server-addr: 127.0.0.1:8848 file-extension: yml ext-config: - data-id: datasource-share-config.yml group: SHARE_GROUP refresh: true - data-id: log-share-config.yml group: SHARE_GROUP refresh: true
server: port: 8081 spring: application: name: management version: 1.0.0 mvc: static-path-pattern: /management/** resources: static-locations: - file:../../web/management - file:../../web/common ``` ### 3. Startup Class ``` package com.guor; import org.mybatis.spring.annotation.MapperScan; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.client.discovery.EnableDiscoveryClient; import org.springframework.cloud.context.config.annotation.RefreshScope; @EnableDiscoveryClient @SpringBootApplication(scanBasePackages = "com.guor") @MapperScan("com.guor.management.dao") @RefreshScope public class ManagementApplication { public static void main(String[] args) { SpringApplication.run(ManagementApplication.class, args); } } ``` 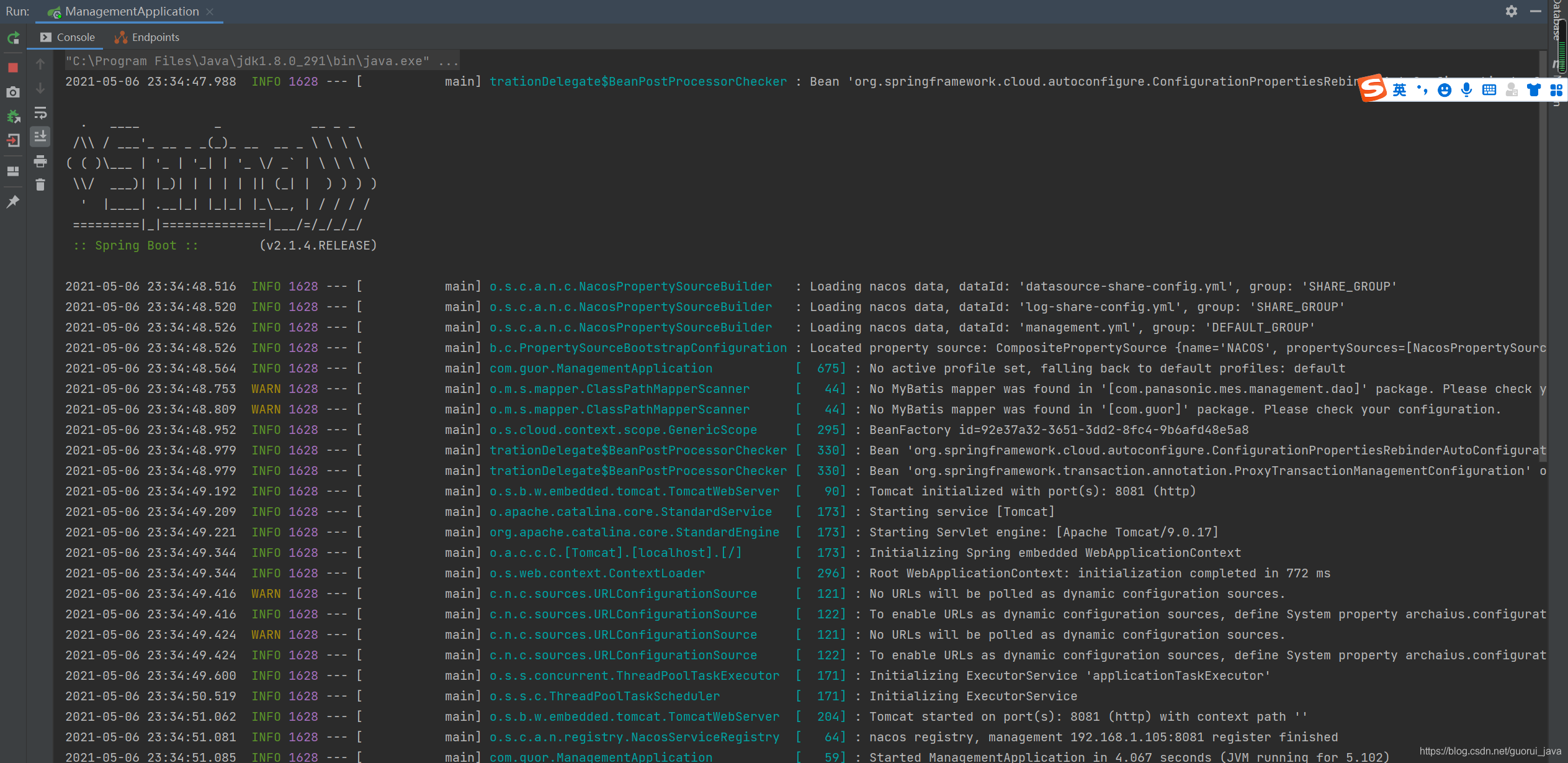 5. Integration mybatis ----------- ### 1. user table design Database selection is the most common MySQL ``` CREATE TABLE `user` ( `user_id` int(10) unsigned NOT NULL AUTO_INCREMENT, `username` varchar(100) NOT NULL, `password` varchar(40) NOT NULL, `age` int(11) DEFAULT NULL, `sex` int(11) DEFAULT NULL, `telephone` varchar(100) DEFAULT NULL, `address` varchar(100) DEFAULT NULL, `create_date` date DEFAULT NULL, `update_date` date DEFAULT NULL, `deleted` int(11) DEFAULT NULL, `version` int(11) DEFAULT NULL, PRIMARY KEY (`user_id`) ) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8; ``` ### 2,UserController ``` package com.guor.management.controller; import com.guor.management.bean.User; import com.guor.management.service.UserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PutMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RestController; import java.util.List; @RestController public class UserController { @Autowired private UserService userService; @GetMapping("/getUserList") public List<User> getUserList(){ return userService.getUserList(); } @PutMapping("/insertUser") public void insertUser(@RequestBody User user){ userService.insertUser(user); } } ``` ### 3,UserService ``` package com.guor.management.service; import com.guor.management.bean.User; import java.util.List; public interface UserService { List<User> getUserList(); void insertUser(User user); }
package com.guor.management.service.impl;
import com.guor.management.bean.User;
import com.guor.management.dao.UserMapper;
import com.guor.management.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class UserServiceImpl implements UserService {
@Autowired private UserMapper userMapper; @Override public List<User> getUserList() { return userMapper.getUserList(); } @Override public void insertUser(User user) { userMapper.insertUser(user); }
}
### 4,UserMapper
summary
Be prepared for an interview, especially if some questions are easy to dig. For example, why did you leave your current company (you should certainly not complain about what's wrong with your current company, more should indicate that you want to find better development opportunities, some of your own practical factors, such as for me, the company you are applying for is closer to your home, or you have reached a confused period of your own work, want to jump out of confusion, etc.)
Java Interview Topics, Architecture Documentation
It's not easy to organize. Friends who feel helpful can help share some favors and support a little edition.
til.List;
@Service
public class UserServiceImpl implements UserService {
@Autowired private UserMapper userMapper; @Override public List<User> getUserList() { return userMapper.getUserList(); } @Override public void insertUser(User user) { userMapper.insertUser(user); }
}
### 4,UserMapper
summary
Be prepared for an interview, especially if some questions are easy to dig. For example, why did you leave your current company (you should certainly not complain about what's wrong with your current company, more should indicate that you want to find better development opportunities, some of your own practical factors, such as for me, the company you are applying for is closer to your home, or you have reached a confused period of your own work, want to jump out of confusion, etc.)
[img-pEnSVRgf-1630407892341)]
Java Interview Topics, Architecture Documentation
It's not easy to organize. Friends who feel helpful can help share some favors and support a little edition.
Your support, my motivation; Wishing you all a bright future and endless offer s!