decoupling
Just now we said that the creation of resources was entrusted to sring. We can find spring for what we need. This process is like a factory model. But what objects it needs to create in the spring framework, it needs a configuration file. This configuration file tells spring which resources need to be created.
For example, suppose I need to go to the database to query the data display page
When the program starts, the spring framework looks for the configuration file to create resources, places the resources in another container, starts running, the front end requests data, looks for the controller layer in spring, then looks for the service layer, and then looks for the dao layer to ask for data. Finally, the data returns to the controller in the original way and is displayed on the page. The service is injected into the controller layer by spring, and the dao layer is injected into the service layer by spring. The division of labor in this process is clear. Each floor performs its own duties. In a traditional development, new is directly in the servlet, then the data is checked, and then the data is returned to the interface. In case of operating more than one judgment and querying different tables, the code of this servlet becomes very bloated. Not to mention slow development, it's hard for you to read the code after development. So control inversion can be used to decouple
What is AOP?
concept
-
In the software industry, AOP is the abbreviation of Aspect Oriented Programming, which means Aspect Oriented Programming
-
AOP is a programming paradigm, which belongs to the category of software engineering and guides developers how to organize program structure
-
AOP was first proposed by the organization of AOP alliance and formulated a set of specifications Spring introduces the idea of AOP into the framework, which must comply with the specifications of AOP alliance
-
A technology of unified maintenance of program functions through precompiled mode and runtime dynamic agent
-
AOP is the continuation of OOP, a hot spot in software development, an important content in Spring framework, and a derivative paradigm of functional programming
-
AOP can isolate each part of business logic, reduce the coupling between each part of business logic, improve the reusability of program, and improve the efficiency of development
In fact, AOP can enhance the program without modifying the source code!!
The underlying implementation of AOP in Spring framework
1. The underlying AOP technology of spring framework also adopts proxy technology. There are two proxy methods
①. Dynamic agent based on JDK
It must be interface oriented. Only classes that implement specific interfaces can generate proxy objects
②. Dynamic agent based on CGLIB
For classes that do not implement interfaces, you can also generate proxies and subclasses of this class
2. Spring's traditional AOP uses different proxy methods according to whether the class implements the interface
①. If the class interface is implemented, use JDK dynamic proxy to complete AOP
②. If the interface is not implemented, CGLIB dynamic agent is used to complete AOP
Dynamic agent of JDK
Note: you need to implement the class interface
Example: suppose I have two jobs, job 1 and job 2
//Write an interface public interface Working { void wokingOne(); void WorkingTwo(); }
//Interface implementation class
public class WorkingImpl implements Working {
@Override public void wokingOne() { System.out.println("Do task 1"); } @Override public void WorkingTwo() { System.out.println("Do task 2"); }
}
> OK, now I'm going to do task 1 first, and then task 2:
public static void main(String[] args) {
Working working = new WorkingImpl(); working.wokingOne();//Do task 1 working.WorkingTwo();//Do task 2 }
> OK, here comes the wonderful place,I need to rest for 10 minutes before I do task 2, but I can't modify the source code. What shall I do? That's when we need it JDK Dynamic proxy. The code is as follows: > First write a tool class for the agent. Let's take a ten minute break before we do task 2 again
public class MyProxyUtils {
public static Working getProxy(final Working working) { // Generating Proxy objects using Proxy classes Working proxy = (Working) Proxy.newProxyInstance(working.getClass().getClassLoader(), working.getClass().getInterfaces(), new InvocationHandler() { // When the proxy object method is a straight line, the invoke method executes once public Object invoke(Object proxy, Method method, Object[] args) throws Throwable { //I'll take a 10 minute break before I do another job 2 if ("WorkingTwo".equals(method.getName())) { System.out.println("Take a 10 minute break"); } //The work continued return method.invoke(working, args); } }); // Return proxy object return proxy; }
}
public static void main(String[] args) { Working working = new WorkingImpl(); Working proxy = MyProxyUtils.getProxy(working); proxy.wokingOne(); proxy.WorkingTwo(); } ``` The results of the operation can be imagined: ``` Do task 1 Take a 10 minute break Do task 2 ``` ### CGLIB dynamic proxy **Note: class interface is not implemented** > CGLIB Also a java Project, so to use it, you need to introduce CGLIB Developed by jar Package,**Because in Spring Framework core package( core)Has been introduced in CGLIB Your development kit**. So direct introduction Spring Core development package **OK, let's also use the above example as an example** ``` //Work class public class Working { public void wokingOne() { System.out.println("Do task 1"); } public void WorkingTwo() { System.out.println("Do task 2"); } } ``` > new There is no need to say the result of an object calling task 1 and task 2. Let's focus on how to use it CGLIB Let's take a ten minute break before doing the task without changing the source code. ``` public static Working getProxy(){ // Create CGLIB core classes Enhancer enhancer = new Enhancer(); // Set parent class enhancer.setSuperclass(Working.class); // Set callback function enhancer.setCallback(new MethodInterceptor() { @Override public Object intercept(Object obj, Method method, Object[] args, MethodProxy methodProxy) throws Throwable { if("WorkingTwo".equals(method.getName())){ ## Java core interview question bank of first-line Internet manufacturers 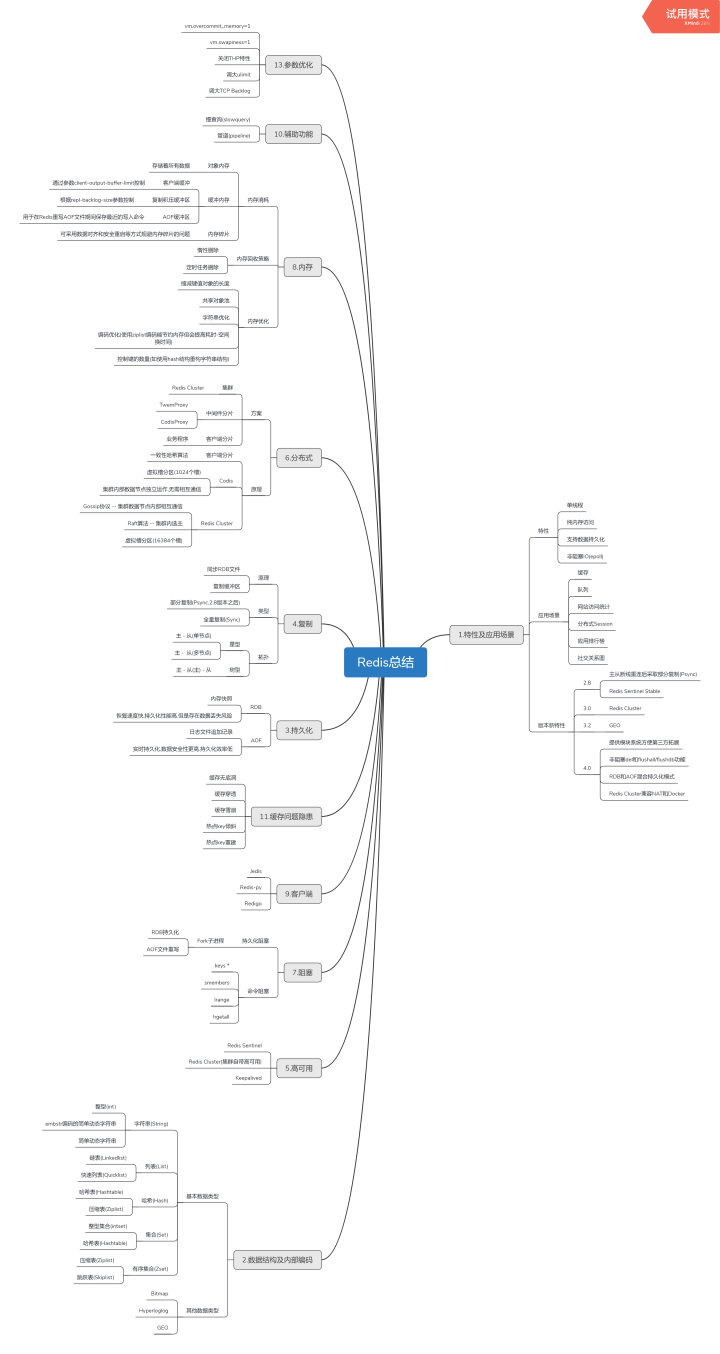 Just in the interview job hopping season, I sorted out some real interview questions asked by the big factory. Due to the length limit of the article, I only showed you some questions and more Java Basic, exception, set, concurrent programming JVM,Spring Family bucket MyBatis,Redis,Database, middleware MQ,Dubbo,Linux,Tomcat,ZooKeeper,Netty wait...It has been sorted and uploaded. Interested friends can see and support a wave! thodProxy) throws Throwable { if("WorkingTwo".equals(method.getName())){ ## Java core interview question bank of first-line Internet manufacturers [External chain picture transfer...(img-rlQ2orRq-1630476096832)] Just in the interview job hopping season, I sorted out some real interview questions asked by the big factory. Due to the length limit of the article, I only showed you some questions and more Java Basic, exception, set, concurrent programming JVM,Spring Family bucket MyBatis,Redis,Database, middleware MQ,Dubbo,Linux,Tomcat,ZooKeeper,Netty wait...It has been sorted and uploaded. Interested friends can see and support a wave! **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)**