Hello, I'm Charlie. Today I'll share some Python basics. Let's have a look~
1, Function introduction
The so-called function means that the code of some specific functions is composed into a whole, which is called a function.
2, Function definition and call
What is the definition of function: it is equivalent to defining a function that can complete some events; It's like building a tool yourself.
Define function format:
def test(): print('----Hee hee----') print('----This is my first function----')
What is a function call: if only a function is defined, it cannot be executed automatically. You must call it.
Generally speaking, defining a function is equivalent to creating a tool, and calling a function is equivalent to using this tool to complete what you want to do.
\# Define a function def test(): print('----Hee hee----') print('----This is my first function----') \# Call function test()
Operation results:
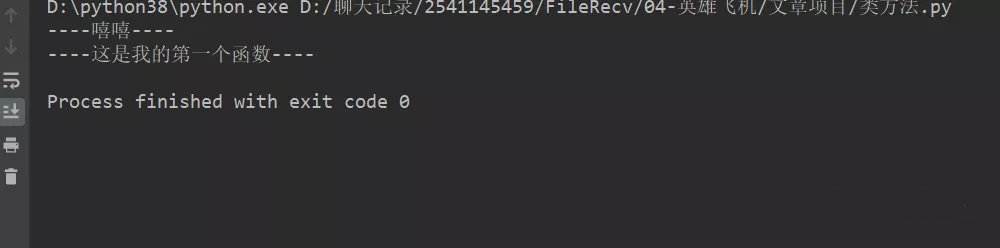
One of the reasons why Python is more and more popular with developers is that Python has a wealth of functions and basically all the functions it needs.
Time function
In development, it is often necessary to print some debugging information. At this time, it is necessary to output time, which requires some time functions.
1. Get the current date: time time()
import time \# Introducing time module currentTime \= time.time() print("The current timestamp is:", currentTime)
Operation results:
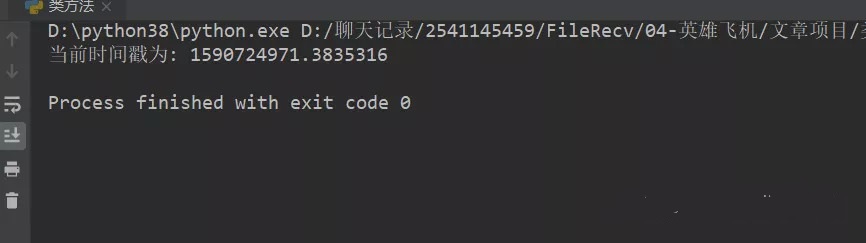
2. Get timestamp in tuple form: time local(time.time())
import time localtime \= time.localtime(time.time()) print ( "Local time is :", localtime)
Operation results:

import time localtime \= time.asctime( time.localtime(time.time()) ) print ( "Local time is :", localtime)
Operation results:
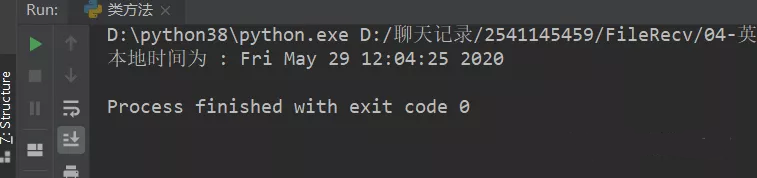
Extension (datetime module):
1. Date output format datetime = > string
import datetime now \= datetime.datetime.now() now.strftime('%Y-%m-%d %H:%M:%S')
2. Date output format string = > datetime
import datetime t\_str \= '2019-04-07 16:11:21' d \= datetime.datetime.strptime(t\_str, '%Y-%m-%d %H:%M:%S') print(d)
Operation results:
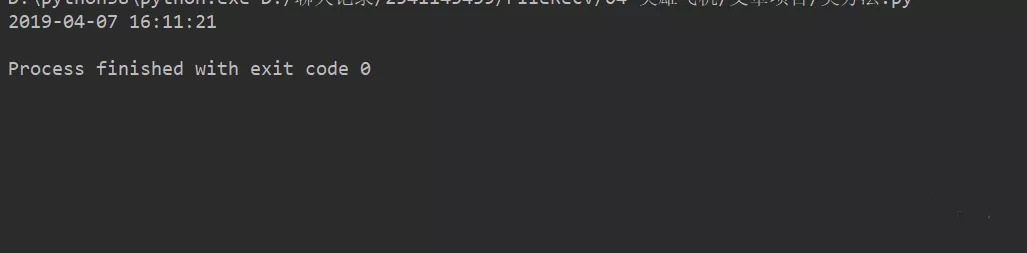
strptime is a static method of the datetime class.
3. Date comparison
In the datetime module, there is a timedelta class. The object of this class is used to represent a time interval, such as the difference between two dates or times.
Construction method:
import datetime datetime.timedelta(days=0, seconds=0, microseconds=0, milliseconds=0, minutes=0, hours=0, weeks=0)
All parameters have a default value of 0. These parameters can be int or float, positive or negative.
You can use timedelta days,tiemdelta.seconds, etc. obtain the corresponding time value.
The instance of timedelta class supports operations such as addition, subtraction, multiplication and division. The result is also an instance of timedelta class.
import datetime year \= datetime.timedelta(days\=365) t\_years \= year \*10 new\_years \= ten\_years \- year print(t\_years) print(new\_years)
Operation results:
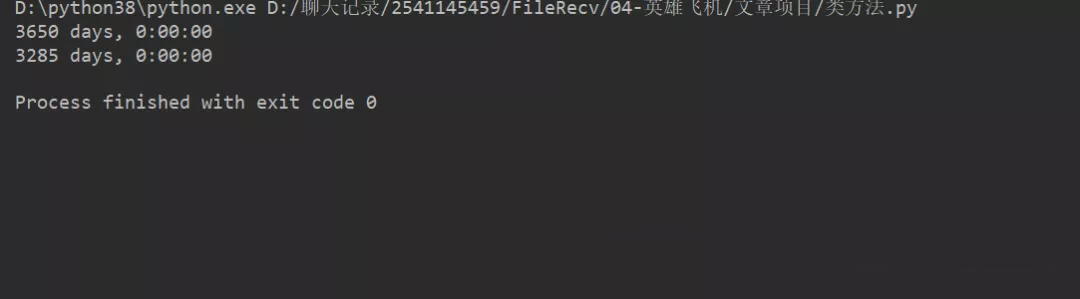
date, time and datetime classes also support addition and subtraction operations with timedelta.
datetime1 \= datetime2 + timedelta timedelta \= datetime1 \- datetime2
In this way, some functions can be easily realized.
Calendar function
datetime1 \= datetime2 + timedelta timedelta \= datetime1 \- datetime2
Operation results:
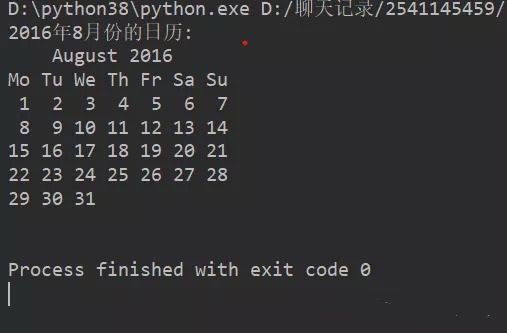
Random number function
import random a \= random.uniform(1, 5) print("a =", a) b \= random.randint(10, 50) print ("b =", b) c \= random.randrange(0, 51, 2) print ("c =", c)
Operation results:
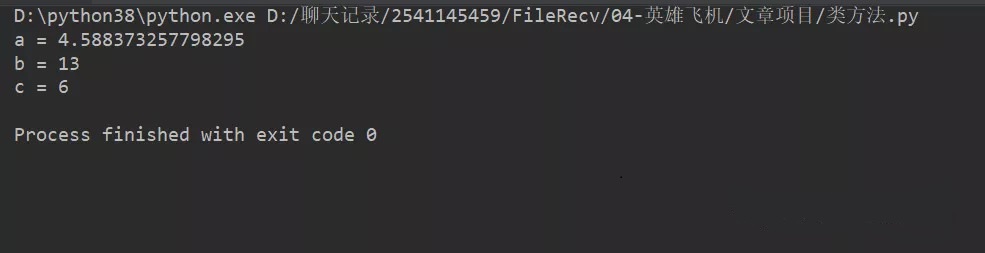
3, Summary
This article explains in detail the definition and calling of Python basic functions. This paper introduces the use methods of three commonly used functions. Through small projects, readers can better understand and use functions, hoping to help you learn Python better.