It mainly acts on the DAO interface and can automatically generate the implementation class of the interface.
8: @MapperScan
It is mainly used on the startup class to generate the implementation class of DAO interface. If there are many DAO interfaces, it is recommended to use @ MapperScan annotation, such as @ MapperScan("com.example.demo.dao")
9: @Controller
It is mainly used on the control layer class to process http requests, etc.
10: @PathVariable
It is mainly used to get the value of the variable in the url, such as @ RequestMapping("/student/{studentName}"), which can be written as: (@ PathVariable String studentName) in the corresponding method input parameter
11: @RequestParam
Bind the request parameter to the Controller method, @ RequestParam(value = "parameter name").
How to use mybaits plus
=================
Add dependency
- Add code generator dependency
<dependency> <groupId>com.baomidou</groupId> <artifactId>mybatis-plus-generator</artifactId> <version>3.4.1</version> </dependency>
- Add template engine dependency. Mybatis plus supports Velocity (default), Freemarker and Beetl. Users can choose their familiar template engine. If none of them meet your requirements, they can use a custom template engine.
Velocity (default):
<dependency> <groupId>org.apache.velocity</groupId> <artifactId>velocity-engine-core</artifactId> <version>latest-velocity-version</version> </dependency>
Freemarker:
<dependency> <groupId>org.freemarker</groupId> <artifactId>freemarker</artifactId> <version>latest-freemarker-version</version> </dependency>
Beetl:
<dependency> <groupId>com.ibeetl</groupId> <artifactId>beetl</artifactId> <version>latest-beetl-version</version> </dependency>
be careful! If you select a non default engine, you need to set the template engine in AutoGenerator.
AutoGenerator generator = new AutoGenerator(); // set freemarker engine generator.setTemplateEngine(new FreemarkerTemplateEngine()); // set beetl engine generator.setTemplateEngine(new BeetlTemplateEngine()); // set custom engine (reference class is your custom engine class) generator.setTemplateEngine(new CustomTemplateEngine()); // other config
Write configuration
- Configure GlobalConfig
GlobalConfig globalConfig = new GlobalConfig(); globalConfig.setOutputDir(System.getProperty("user.dir") + "/src/main/java"); globalConfig.setAuthor("jobob"); globalConfig.setOpen(false);
- Configure DataSourceConfig
DataSourceConfig dataSourceConfig = new DataSourceConfig(); dataSourceConfig.setUrl("jdbc:mysql://localhost:3306/ant?useUnicode=true&useSSL=false&characterEncoding=utf8"); dataSourceConfig.setDriverName("com.mysql.jdbc.Driver"); dataSourceConfig.setUsername("root"); dataSourceConfig.setPassword("password");
Custom template engine
Please inherit class com baomidou. mybatisplus. generator. engine. AbstractTemplateEngine
Custom code template
//Specify the path of custom template at: / resources / templates / entity2 java. FTL (or. vm) //Be careful not to take it with you FTL (or. vm) will be automatically recognized according to the template engine used TemplateConfig templateConfig = new TemplateConfig() .setEntity("templates/entity2.java"); AutoGenerator mpg = new AutoGenerator(); //Configure custom templates mpg.setTemplate(templateConfig);
Custom attribute injection
InjectionConfig injectionConfig = new InjectionConfig() { //Custom attribute injection: abc //Yes In the FTL (or. vm) template, get the attributes through ${cfg.abc} @Override public void initMap() { Map<String, Object> map = new HashMap<>(); map.put("abc", this.getConfig().getGlobalConfig().getAuthor() + "-mp"); this.setMap(map); } }; AutoGenerator mpg = new AutoGenerator(); //Configure custom attribute injection mpg.setCfg(injectionConfig);
entity2.java.ftl
Custom attribute injection abc=${cfg.abc}
entity2.java.vm
Custom attribute injection abc=$!{cfg.abc}
Field other information query injection ----------- 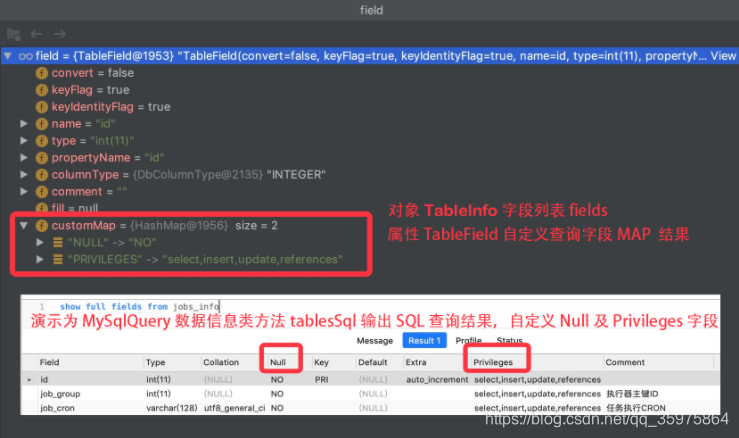
new DataSourceConfig().setDbQuery(new MySqlQuery() {
/** * Override the reserved query user-defined fields of the parent class < br > * The SQL queried here corresponds to the query field of the parent class tableFieldsSql. By default, it cannot meet your needs. Please rewrite it < br > * Template: table.fields gets all field information, * Then loop the field to get the field Custommap obtains the injection fields from the MAP as follows: NULL or PRIVILEGES */ @Override public String[] fieldCustom() { return new String[]{"NULL", "PRIVILEGES"}; }
})
mybaits Common notes =========== 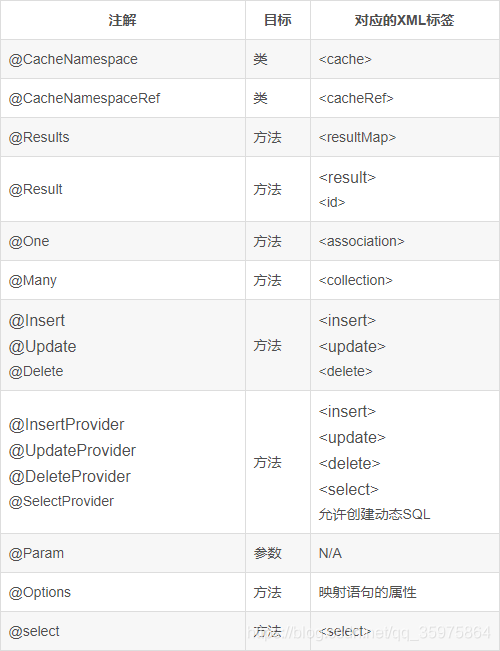 ==================================================================================================================================================================================================== Write a simple login registration function ============== First, establish the database and create User surface 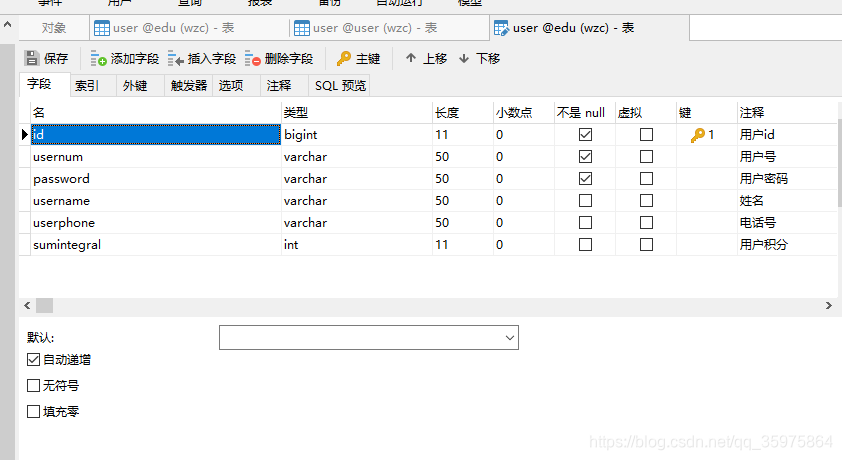 again idea Create a good framework in 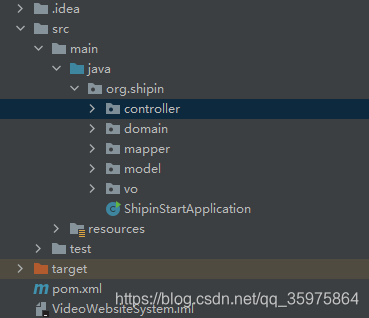 model Inside User.java
package org.shipin.model;
import lombok.Data;
@Data
public class User {
private Integer id; private String usernum; private String password; private String username; private String userphone;
}
mappaer Inside UserMapper.java This is the interface
package org.shipin.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Select;
import org.shipin.model.User;
@Mapper
public interface UserMapper extends BaseMapper {
/** * Query users by phone * @param phone * @return */ @Select("select * from `user` where `usernum` = #{usernum}") User selectUserByUsernum(String phone);
}
controller Inside UserController.java
package org.shipin.controller;
import cn.hutool.crypto.SecureUtil;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import io.swagger.annotations.ApiOperation;
import org.shipin.domain.ResponseMessage;
import org.shipin.mapper.UserMapper;
import org.shipin.model.User;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
Let's share my review interview materials
These interviews are all from the real interview questions and interview collection of large factories, and the small series has been sorted out for you (PDF version)
Data acquisition method: Click here to go to my Tencent document for free download
- Part I: Java Basics - intermediate - Advanced
- Part II: open source framework (SSM: Spring + spring MVC + mybatis)
- Part 3: performance tuning (JVM+MySQL+Tomcat)
- Part IV: distributed (current limit: ZK+Nginx; Cache: Redis+MongoDB+Memcached; communication: MQ+kafka)
- Part V: microservices (SpringBoot+SpringCloud+Dubbo)
- Part VI: others: concurrent programming + design pattern + data structure and algorithm + network
Advanced learning notes pdf
It's all sorted out and needs to be free Download click here
- Architecture foundation for advanced Java architecture (Java foundation + concurrent programming + JVM+MySQL+Tomcat + Network + data structure and algorithm)
- Open source framework for advanced Java architecture (design pattern + Spring + spring MVC + mybatis)
- Advanced Java architecture: distributed architecture (ZK/Nginx) + Redis/MongoDB/Memcached) + communication (MQ/kafka))
- Advanced Java architecture: microservice architecture (RPC+SpringBoot+SpringCloud+Dubbo+K8s)
65)]
- Open source framework for advanced Java architecture (design pattern + Spring + spring MVC + mybatis)
[external chain picture transferring... (img-RvccPxy8-1629242182565)]
[external chain picture transferring... (img-Cf8gKUgE-1629242182566)]
[external chain picture transferring... (img-WGgDcIIf-1629242182566)]
- Advanced Java architecture: distributed architecture (ZK/Nginx) + Redis/MongoDB/Memcached) + communication (MQ/kafka))
[external chain picture transferring... (img-Jt9XUzE9-1629242182566)]
[external chain picture transferring... (img-I75WMEO2-1629242182567)]
[external chain picture transferring... (img-YnUHhTP9-1629242182567)]
- Advanced Java architecture: microservice architecture (RPC+SpringBoot+SpringCloud+Dubbo+K8s)
[external chain picture transferring... (img-i0iUn7OS-1629242182568)]
[external chain picture transferring... (img-e7jwGZDl-1629242182568)]