Time complexity mainly measures the running speed of an algorithm, while space complexity mainly measures the additional space required by an algorithm.
In the early days of computer development, the storage capacity of computer was very small. So I care about the complexity of space.
However, with the rapid development of computer industry, the storage capacity of computer has reached a high level. Therefore, we no longer need to pay special attention to the spatial complexity of an algorithm.
2. Time complexity
=======
2.1 concept of time complexity
Definition of time complexity:
In computer science, the time complexity of an algorithm is a function, which quantitatively describes the running time of the algorithm.
In theory, the time spent in the execution of an algorithm cannot be calculated. You can only know when you put your program on the machine and run it.
But do we need every algorithm to be tested on the computer? Yes, it can be tested on the computer, but it is very troublesome, so there is the analysis method of time complexity.
The time spent by an algorithm is directly proportional to the execution times of the statements in it. The execution times of the basic operations in the algorithm is the time complexity of the algorithm.
2.2 progressive representation of large O
// Please calculate how many times func1 basic operations have been performed? void func1(int N){ int count = 0; for (int i = 0; i < N ; i++) { for (int j = 0; j < N ; j++) { count++; } } for (int k = 0; k < 2 * N ; k++) { count++; } int M = 10; while ((M--) > 0) { count++; } System.out.println(count); }
Number of basic operations performed by Func1:
N = 10 F(N) = 130
N = 100 F(N) = 10210
N = 1000 F(N) = 1002010
In fact, when we calculate the time complexity, we do not have to calculate the exact execution times, but only the approximate execution times. Here, we use the asymptotic representation of large O.
Big O notation:
Is a mathematical symbol used to describe the asymptotic behavior of a function.
Derivation of large O-order method:
1. Replace all addition constants in the run time with constant 1.
2. In the modified run times function, only the highest order term is retained.
3. If the highest order term exists and is not 1, the constant multiplied by this item is removed. The result is large O-order.
After using the asymptotic representation of large O, the time complexity of Func1 is
N = 10 F(N) = 100
N = 100 F(N) = 10000
N = 1000 F(N) = 1000000
From the above, we can find that the progressive representation of big O removes those items that have little impact on the results, and succinctly represents the execution times.
In addition, the time complexity of some algorithms has the best, average and worst cases:
Worst case: maximum number of runs of any input scale (upper bound)
Average case: expected number of runs of any input scale
Best case: minimum number of runs of any input scale (lower bound)
For example:
Search for a data x in an array of length N
Best case: 1 time
Worst case: found N times
Average: N/2 times
In practice, we usually focus on the worst-case operation of the algorithm, so the time complexity of searching data in the array is O(N)
2.3 common time complexity calculation examples
Example 1:
// Calculate the time complexity of func2? void func2(int N) { int count = 0; for (int k = 0; k < 2 * N ; k++) { count++; } int M = 10; while ((M--) > 0) { count++; } System.out.println(count); }
The basic operation of example 1 was performed 2N+10 times. It is known that the time complexity is O(N) by deriving the large O-order method
Example 2:
// Calculate the time complexity of func3? void func3(int N, int M) { int count = 0; for (int k = 0; k < M; k++) { count++; } for (int k = 0; k < N ; k++) { count++; } System.out.println(count); }
The basic operation of example 2 is executed M+N times, with two unknowns M and N, and the time complexity is O(N+M)
Example 3:
// Calculate the time complexity of func4? void func4(int N) { int count = 0; for (int k = 0; k < 100; k++) { count++; } System.out.println(count); }
The basic operation of example 3 is performed 100 times. By deriving the large O-order method, the time complexity is O(1)
Example 4:
// Calculate the time complexity of bubbleSort? void bubbleSort(int[] array) { for (int end = array.length; end > 0; end--) { boolean sorted = true; for (int i = 1; i < end; i++) { if (array[i -1] > array[i]) { Swap(array, i - 1, i); sorted = false; } } if (sorted == true) { break; } } }
In example 4, the basic operation was performed for the best N times and the worst (N*(N-1))/2 times. By deriving the large O-order method + time complexity, it is generally the worst, and the time complexity is O(N^2)
Example 5:
// Calculate the time complexity of binarySearch? int binarySearch(int[] array, int value) { int begin = 0; int end = array.length - 1; while (begin <= end) { int mid = begin + ((end-begin) / 2); if (array[mid] < value) begin = mid + 1; else if (array[mid] > value) end = mid - 1; else return mid; } return -1; }
In example 5, the basic operation is performed for the best time and the worst time O(logN) times. The time complexity is O(logN) ps: logN is expressed as base 2 and logarithm N in algorithm analysis. Some places will be written as lgN. (it is recommended to explain how logN is calculated through origami search) (because half of the unsuitable values are excluded each time for binary search, one score is left: n/2, two scores are left: n/2/2 = n/4)
Example 6:
// The time complexity of computing factorial recursive factorial? long factorial(int N) { return N < 2 ? N : factorial(N-1) * N; }
In example 6, through calculation and analysis, it is found that the basic operation recurses N times and the time complexity is O(N).
Example 7:
// Computing the time complexity of fibonacci recursive fibonacci? int fibonacci(int N) { return N < 2 ? N : fibonacci(N-1)+fibonacci(N-2); }
Example 7 shows that the basic operation recurses 2N times and the time complexity is O(2N).
3. Space complexity
=======
Space complexity is a measure of the amount of storage space temporarily occupied by an algorithm during operation.
Space complexity is not how many bytes the program occupies, because it doesn't make much sense, so space complexity is the number of variables.
The calculation rules of spatial complexity are basically similar to the practical complexity, and the large O asymptotic representation is also used.
Example 1:
# Finally, how should we learn? **1,Watch videos for systematic learning** In recent years Crud Experience, let me understand that I am really a fighter in the chicken, because Crud,As a result, their technology is fragmented, not deep enough and not systematic enough, so it is necessary to study again. What I lack is systematic knowledge, poor structural framework and ideas, so learning through video is more effective and comprehensive. About video learning, I can recommend it B Stop to learn, B There are many learning videos on the website. The only disadvantage is that it is free and easy to be outdated. In addition, I have also collected several sets of video materials lying on the online disk. I can share them with you if necessary: 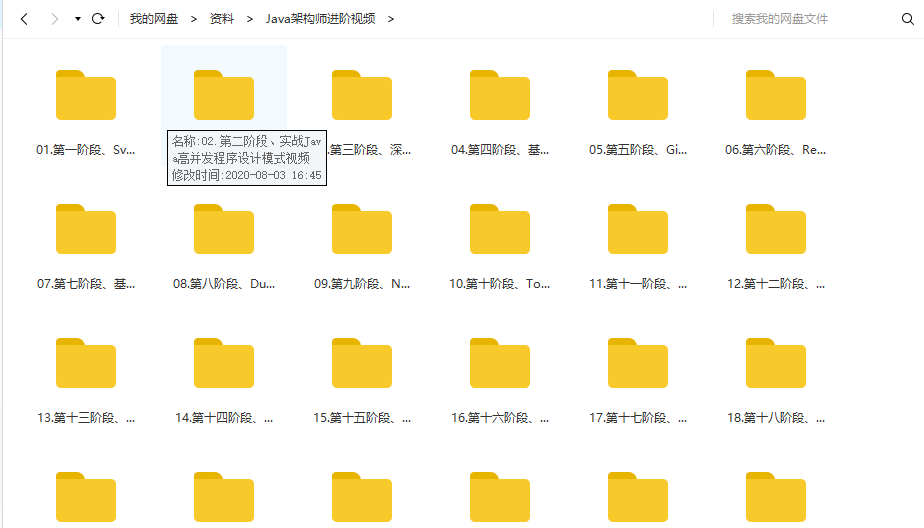 **2,Read the source code, read the actual combat notes, and learn the great God idea** "Programming language is the programmer's way of expression, and architecture is the programmer's cognition of the world ". Therefore, if programmers want to quickly recognize and learn architecture, it is essential to read the source code. Reading the source code is to solve the problem + Understand things, more importantly: see the idea behind the source code; Programmers say: read ten thousand lines of source code and practice ten thousand lines. Spring In depth analysis of source code: 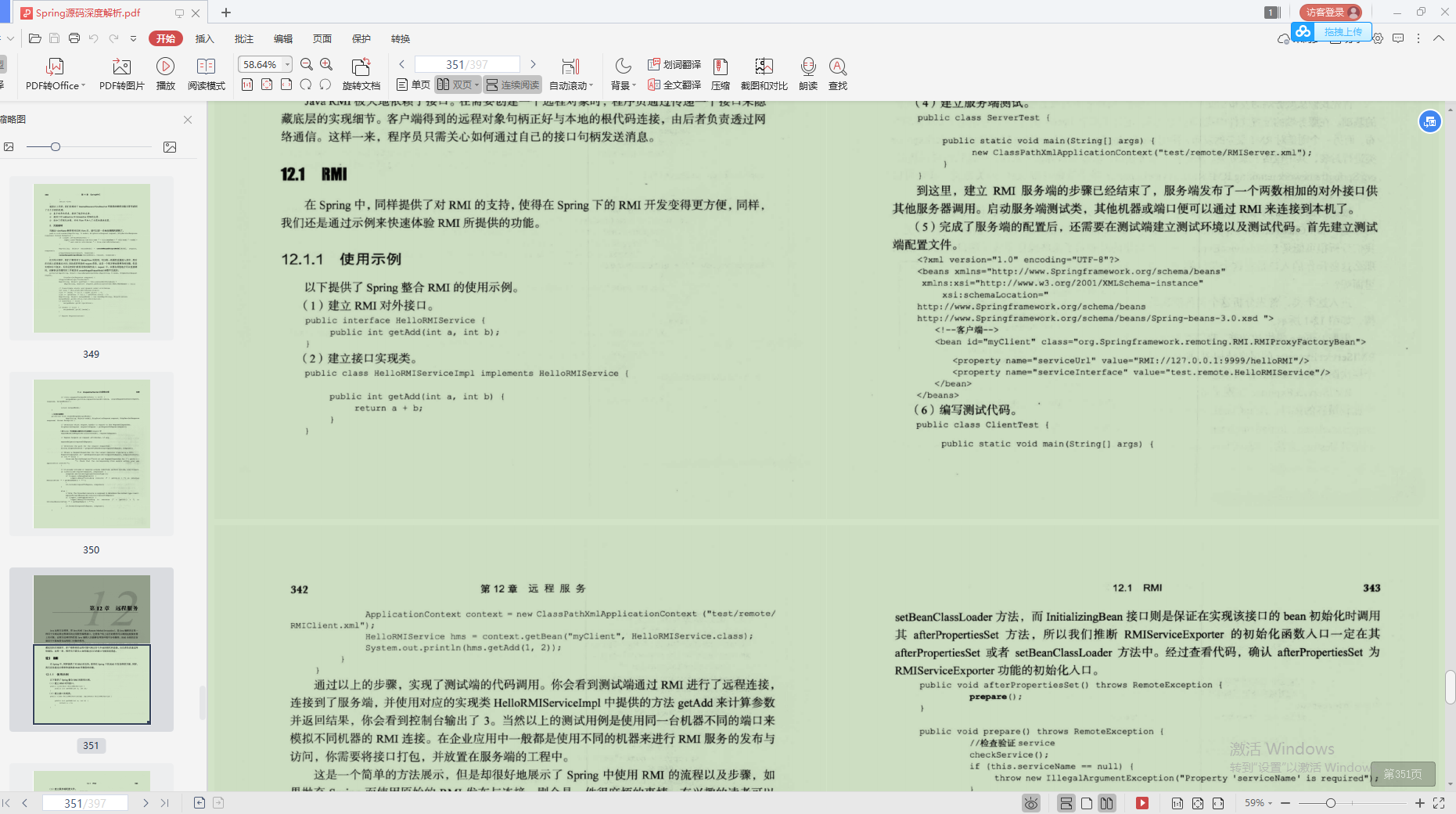 Mybatis 3 In depth analysis of source code: 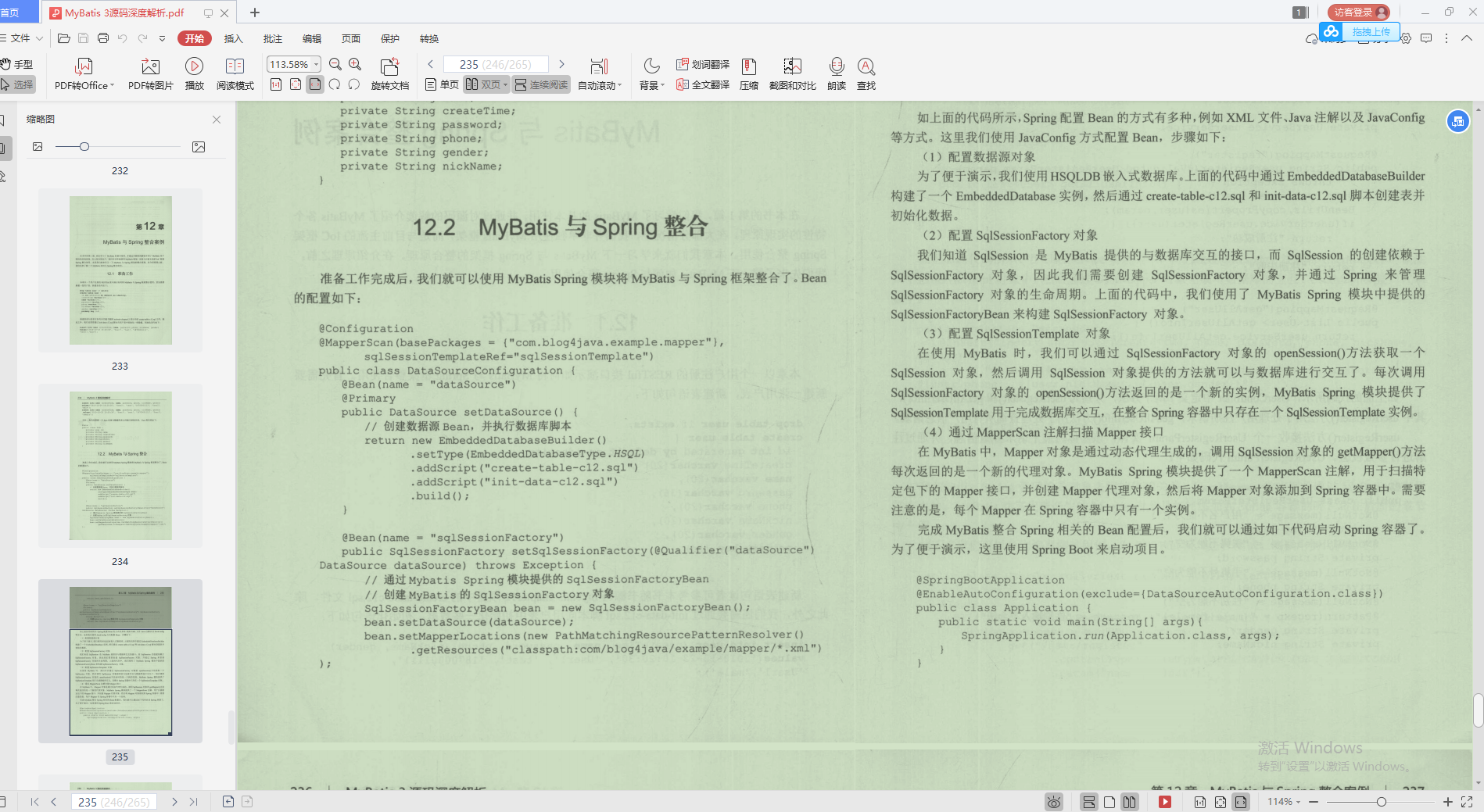 Redis Study notes: 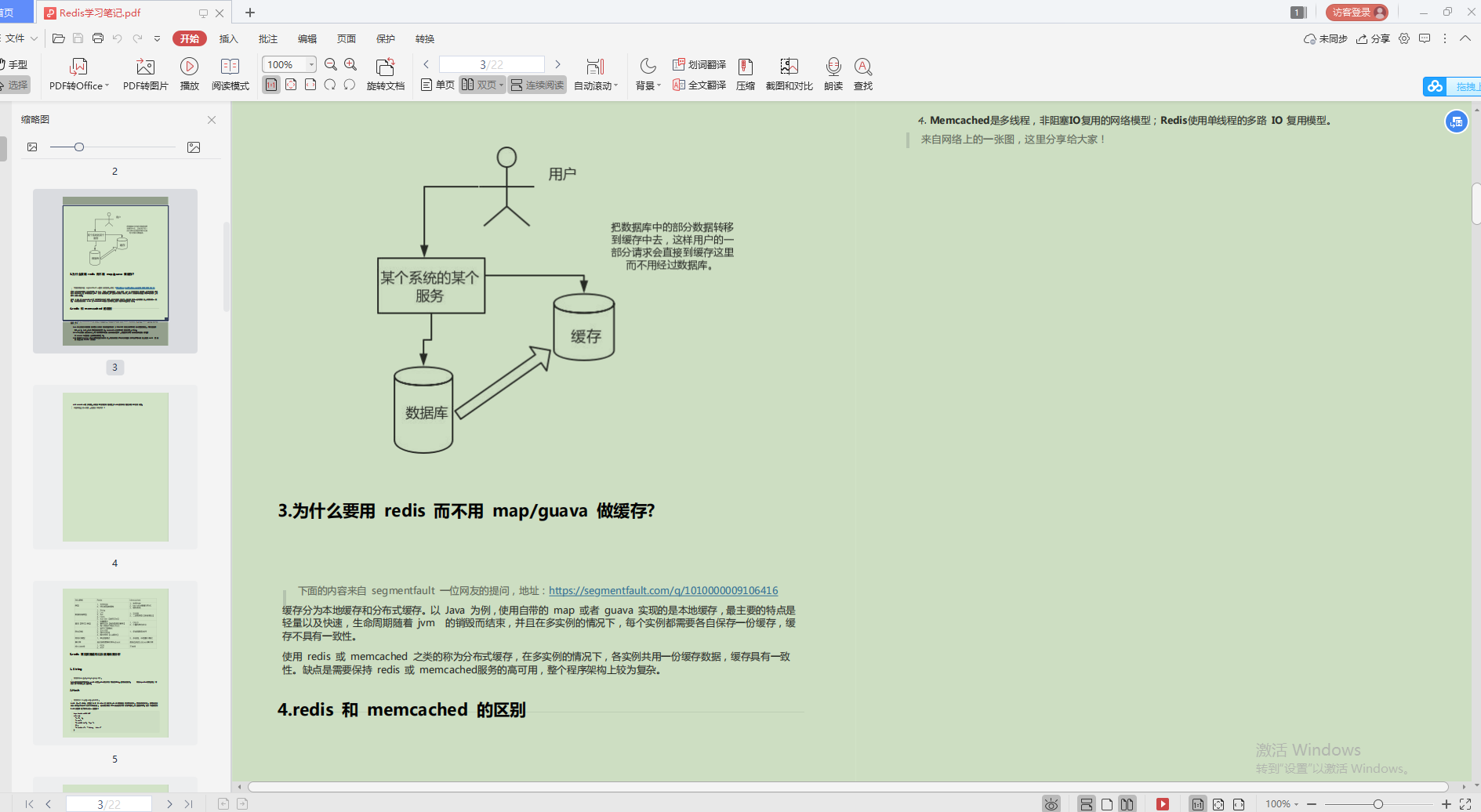 Spring Boot core technology -Notes: 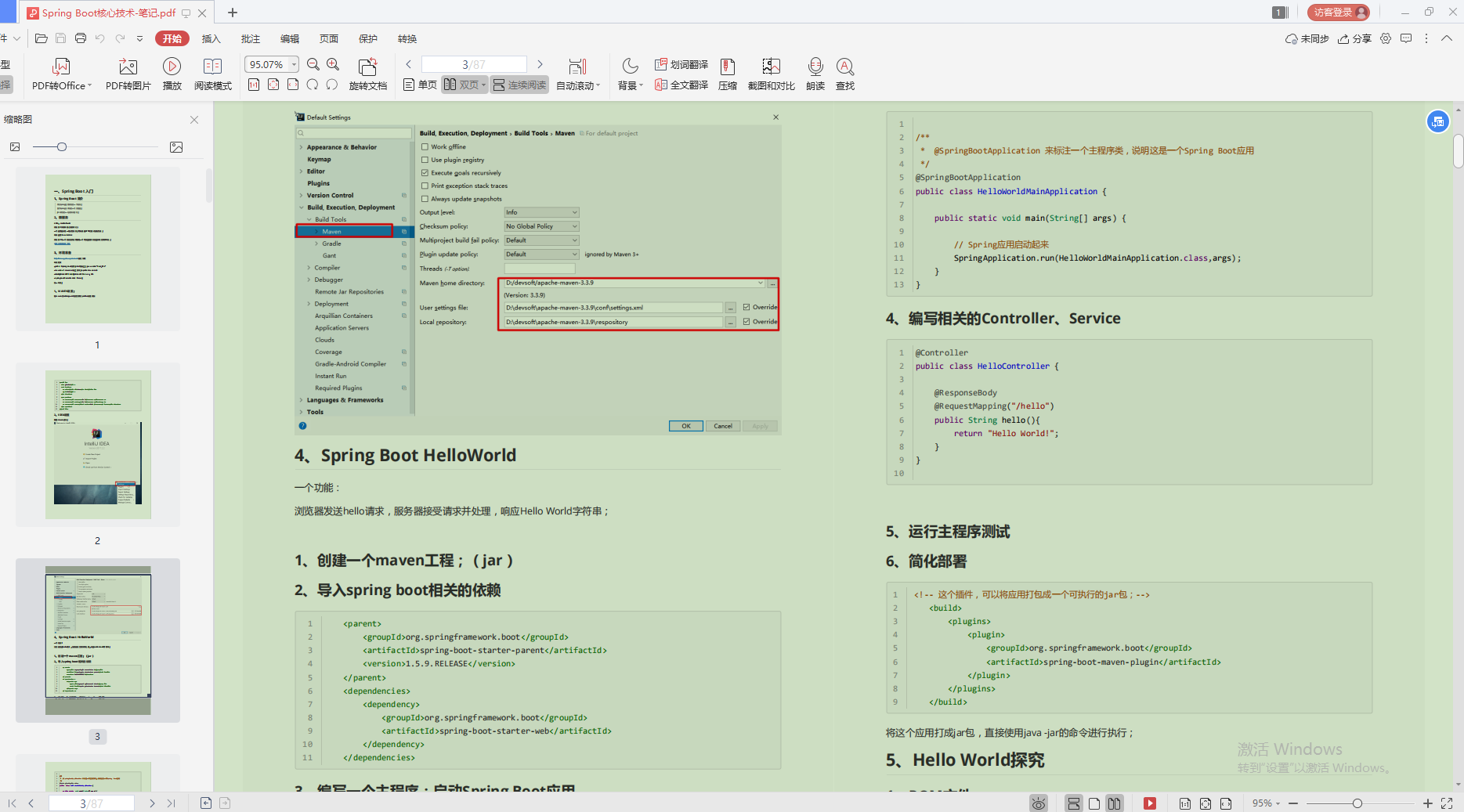 **3,On the eve of the interview, brush questions and sprint** One week before the interview, you can start to brush questions and sprint. Please remember, when you brush questions, technology takes precedence, and the algorithm depends on some basic ones, such as sorting, etc., while intelligence questions are generally not asked unless they are school moves. As for the interview questions, I personally prepared a set of systematic interview questions to help you draw inferences from one example: 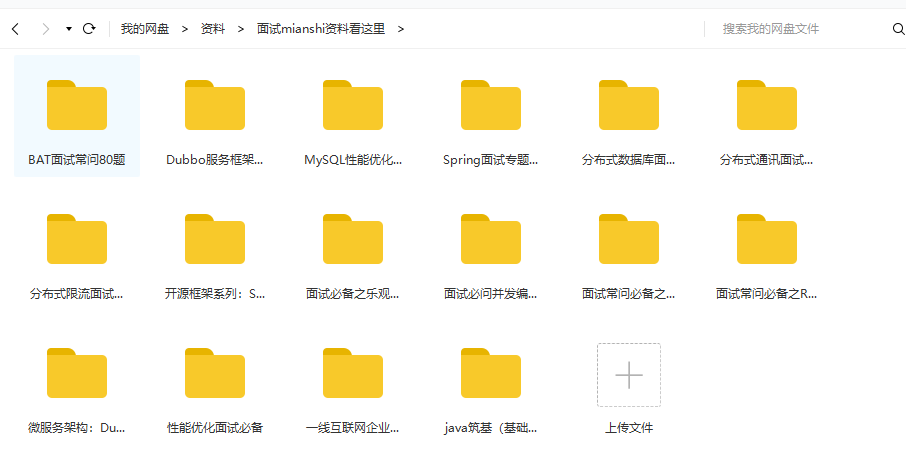 Only with perfect skills, no worries about employment, and "all kinds of things can not be carried away", learning from industry only has the final say in the years of classroom, but is an uninterrupted thing in the journey of life. Life is short, don't muddle through life, don't make do with it. **Data collection method:[Click the blue portal to receive the above information for free](https://codechina.csdn.net/m0_60958482/java-p7)** What is involved in the content of the article Java Interview questions, source code documents, technical notes and other learning materials can be shared to everyone for free. You just need to do it and give more support! mg-8jgXmku9-1629230077888)] Spring Boot core technology -Notes: [External chain picture transfer...(img-ZiiuKKID-1629230077889)] **3,On the eve of the interview, brush questions and sprint** One week before the interview, you can start to brush questions and sprint. Please remember, when you brush questions, technology takes precedence, and the algorithm depends on some basic ones, such as sorting, etc., while intelligence questions are generally not asked unless they are school moves. As for the interview questions, I personally prepared a set of systematic interview questions to help you draw inferences from one example: [External chain picture transfer...(img-IrgPIhdg-1629230077890)] Only with perfect skills, no worries about employment, and "all kinds of things can not be carried away", learning from industry only has the final say in the years of classroom, but is an uninterrupted thing in the journey of life. Life is short, don't muddle through life, don't make do with it. **Data collection method:[Click the blue portal to receive the above information for free](https://codechina.csdn.net/m0_60958482/java-p7)** What is involved in the content of the article Java Interview questions, source code documents, technical notes and other learning materials can be shared to everyone for free. You just need to do it and give more support!