Let's take a look at an example:
Optional<Integer> max = List.of(1, 2, 3).stream() .max((a, b) -> { if (a > b) { return 1; } else { return -1; } }); Copy code
Yes, this is the usage of the max method, which makes me feel that I am not using a functional interface. Of course, you can also use the Integer method for simplification:
Optional<Integer> max = List.of(1, 2, 3).stream() .max(Integer::compare); Copy code
Even so, this method still makes me feel very cumbersome. Although I can understand that passing parameters in the max method is to let us customize the sorting rules, I don't understand why there is no default sorting method according to natural sorting, but to let me pass parameters.
Later, I thought of the basic type Stream. Sure enough, they can get the maximum value directly without passing parameters:
OptionalLong max = LongStream.of(1, 2, 3).max(); Copy code
Sure enough, all the class library designers I could think of ~
Note: Optional long is Optional's encapsulation of the basic type long.
2.3 min: minimum by reduction
min let's just look at the example:
Optional<Integer> max = List.of(1, 2, 3).stream() .min(Integer::compare); Copy code
The difference between it and max is that the bottom layer replaces > with <, which is too simple and will not be repeated.
3. Collector
In Section 3, let's take a look at the collector. Its function is to collect the elements in the Stream to form a new collection.
Although I gave a mind map at the beginning of this article, I drew another one because there are many API s for collectors, which is a supplement to the one at the beginning:
The method name of the collector is collect, and its method definition is as follows:
<R, A> R collect(Collector<? super T, A, R> collector); Copy code
As the name suggests, the Collector is used to collect the elements of the Stream. We can customize the final collection, but we generally don't need to write it ourselves, because the JDK has a built-in implementation class of Collector - Collectors.
3.1 collection method
Through Collectors, we can easily collect data by using its built-in method:
For example, if you want to integrate elements into a collection, you can use the toCollection or toList method, but we generally don't use toCollection because it needs to pass parameters, and no one likes to pass parameters.
You can also use toUnmodifiableList. The difference between toUnmodifiableList and toList is that the returned collection cannot change elements, such as delete or add.
For another example, if you want to collect elements after de duplication, you can use toSet or toUnmodifiableSet.
Next, let's take a simple example:
// toList List.of(1, 2, 3).stream().collect(Collectors.toList()); // toUnmodifiableList List.of(1, 2, 3).stream().collect(Collectors.toUnmodifiableList()); // toSet List.of(1, 2, 3).stream().collect(Collectors.toSet()); // toUnmodifiableSet List.of(1, 2, 3).stream().collect(Collectors.toUnmodifiableSet()); Copy code
The above methods have no parameters and are ready to use. The bottom layer of toList is also a classic ArrayList, and the bottom layer of toSet is a classic HashSet.
Sometimes you may want to collect one order into a Map. For example, by converting the order data into an order number corresponding to an order, you can use toMap():
List<Order> orders = List.of(new Order(), new Order()); Map<String, Order> map = orders.stream() .collect(Collectors.toMap(Order::getOrderNo, order -> order)); Copy code
toMap() has two parameters:
-
The first parameter represents the key, which indicates that you want to set the key of a Map. What I specify here is the orderNo in the element.
-
The second parameter represents value, which means you want to set the value of a Map. Here, I directly regard the element itself as the value, so the result is a Map < string, order >.
You can also treat the attributes of the element as values:
List<Order> orders = List.of(new Order(), new Order()); Map<String, List<Item>> map = orders.stream() .collect(Collectors.toMap(Order::getOrderNo, Order::getItemList)); Copy code
This returns a Map of order number + commodity list.
toMap() also has two companion methods:
-
toUnmodifiableMap(): returns a non modifiable Map.
-
Tocurrentmap(): returns a thread safe Map.
The two methods are as like as two peas (toMap()) parameters. The only difference is that the Map characteristics of the underlying generation are not the same. We usually use simple toMap(), which is the most commonly used HashMap() implementation.
Although toMap() is powerful and commonly used, it has a fatal disadvantage.
We know that HahsMap will overwrite when it encounters the same key, but if the key you specify is repeated when the toMap() method generates the Map, it will directly throw an exception.
For example, in the above order example, we assume that the order numbers of two orders are the same, but you specify the order number as key, then this method will directly throw an IllegalStateException because it does not allow the keys in the element to be the same.
3.2 grouping method
If you want to classify data, but the key you specify can be repeated, you should use groupingBy instead of toMap.
For a simple example, I want to group an order set by order type, which can be as follows:
List<Order> orders = List.of(new Order(), new Order()); Map<Integer, List<Order>> collect = orders.stream() .collect(Collectors.groupingBy(Order::getOrderType)); Copy code
If you directly specify the element attribute for grouping, it will automatically group according to this attribute and collect the grouping results as a List.
List<Order> orders = List.of(new Order(), new Order()); Map<Integer, Set<Order>> collect = orders.stream() .collect(Collectors.groupingBy(Order::getOrderType, toSet())); Copy code
groupingBy also provides an overload that allows you to customize the Collector type, so its second parameter is a Collector collector object.
For the Collector type, we usually use the Collectors class. Here, because we have used Collectors earlier, we do not need to declare that we directly pass in a toSet() method, which means that we collect the grouped elements as sets.
groupingBy also has a similar method called groupingByConcurrent(). This method can improve the grouping efficiency in parallel, but it does not guarantee the order, so it will not be expanded here.
3.3 zoning method
Next, I will introduce another case of grouping - partition. The name is a little windy, but the meaning is very simple:
Grouping data according to TRUE or FALSE is called partitioning.
For example, we group an order set according to whether to pay or not, which is the partition:
List<Order> orders = List.of(new Order(), new Order()); Map<Boolean, List<Order>> collect = orders.stream() .collect(Collectors.partitioningBy(Order::getIsPaid)); Copy code
Because there are only two statuses: paid and unpaid, this grouping method is called partition.
Like groupingBy, it also has an overloaded method to customize the collector type:
List<Order> orders = List.of(new Order(), new Order()); Map<Boolean, Set<Order>> collect = orders.stream() .collect(Collectors.partitioningBy(Order::getIsPaid, toSet())); Copy code
3.4 classical re engraving method
Finally, I come to the last section. Please forgive me for giving this part of the method such a earthy name, but these methods are really like what I said: Classic reproduction.
In other words, Collectors implement the original method of Stream again, including:
-
map → mapping
-
filter → filtering
-
flatMap → flatMapping
-
count → counting
-
reduce → reducing
-
max → maxBy
-
**min ** → minBy
I won't list the functions of these methods one by one. The previous articles have been very detailed. The only difference is that some methods have an additional parameter, which is the collection parameter we talked about in grouping and partition. You can specify what container to collect.
I took them out and mainly wanted to say why there are so many ways to deal with them. Here I'll talk about my personal opinions, not the official opinions.
I think it's mainly for the combination of functions.
What do you mean? For example, I have another requirement: use the order type to group the orders and find out how many orders there are in each group.
We have already talked about order grouping. To find out how many orders there are in each group, we just need to get the size of the corresponding list. However, we can do it in one step without so much trouble. When outputting the results, the key value pairs are order type and order quantity:
Map<Integer, Long> collect = orders.stream() .collect(Collectors.groupingBy(Order::getOrderType, counting())); Copy code
That's it. That's it. That's it. It means that we count the grouped data again.
The above example may not be obvious. When we need to operate on the last collected data, we generally need to convert it into a Stream and then operate, but using these methods of Collectors can make it convenient for you to process data in Collectors.
For another example, the orders are grouped by order type. However, if we want to get the order with the largest amount of each type, we can do this:
List<Order> orders = List.of(new Order(), new Order()); # Final content At the beginning of sharing with you, I said that I was not prepared for the interview. It all depends on my usual accumulation. I really crammed for it temporarily, so that I was very upset myself. (when you're ready, maybe you can get a 40 k,No preparation, only 30 k+,Do you understand that feeling **How to prepare for an interview?** **1,Preliminary bedding (technical deposition)** Programmer interview is actually a thorough examination of technology. If your technology is awesome, you are the boss. The requirements of large factories for technology are mainly reflected in five aspects: foundation, principle, in-depth study of source code, breadth and actual combat. Only by combining principle theory with actual combat can we understand the technical points thoroughly. Here are some information notes I will read, hoping to help you learn from shallow to deep and from point to surface Java,Answer the soul questions of interviewers in large factories,**Poke here if necessary:[Blue portal](https://gitee.com/vip204888/java-p7) pack it and take it away** > This part of the content is too much. Xiaobian only posted part of the content to show you. Forgive me! * Java Programmers must see< Java Development core notes (Huashan version) 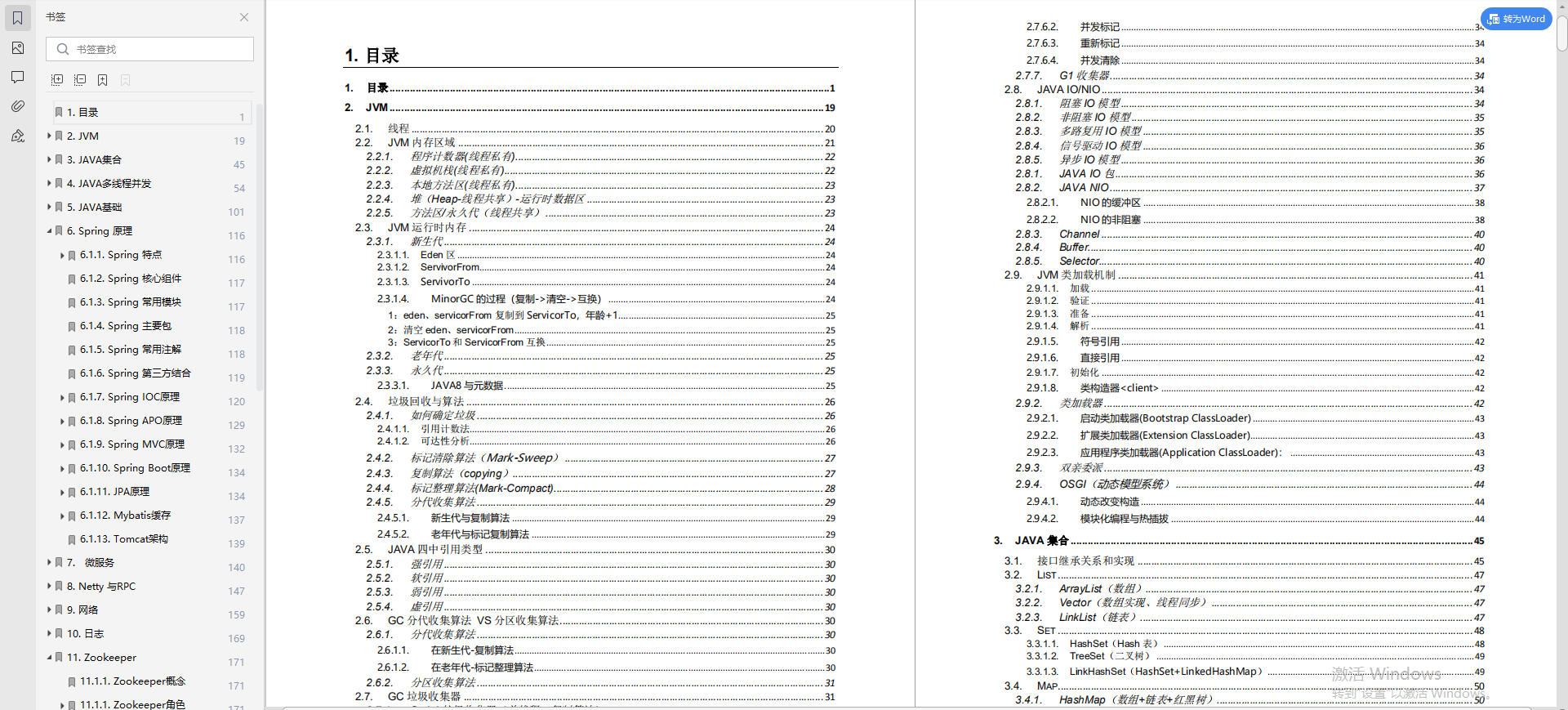 * Redis Study notes 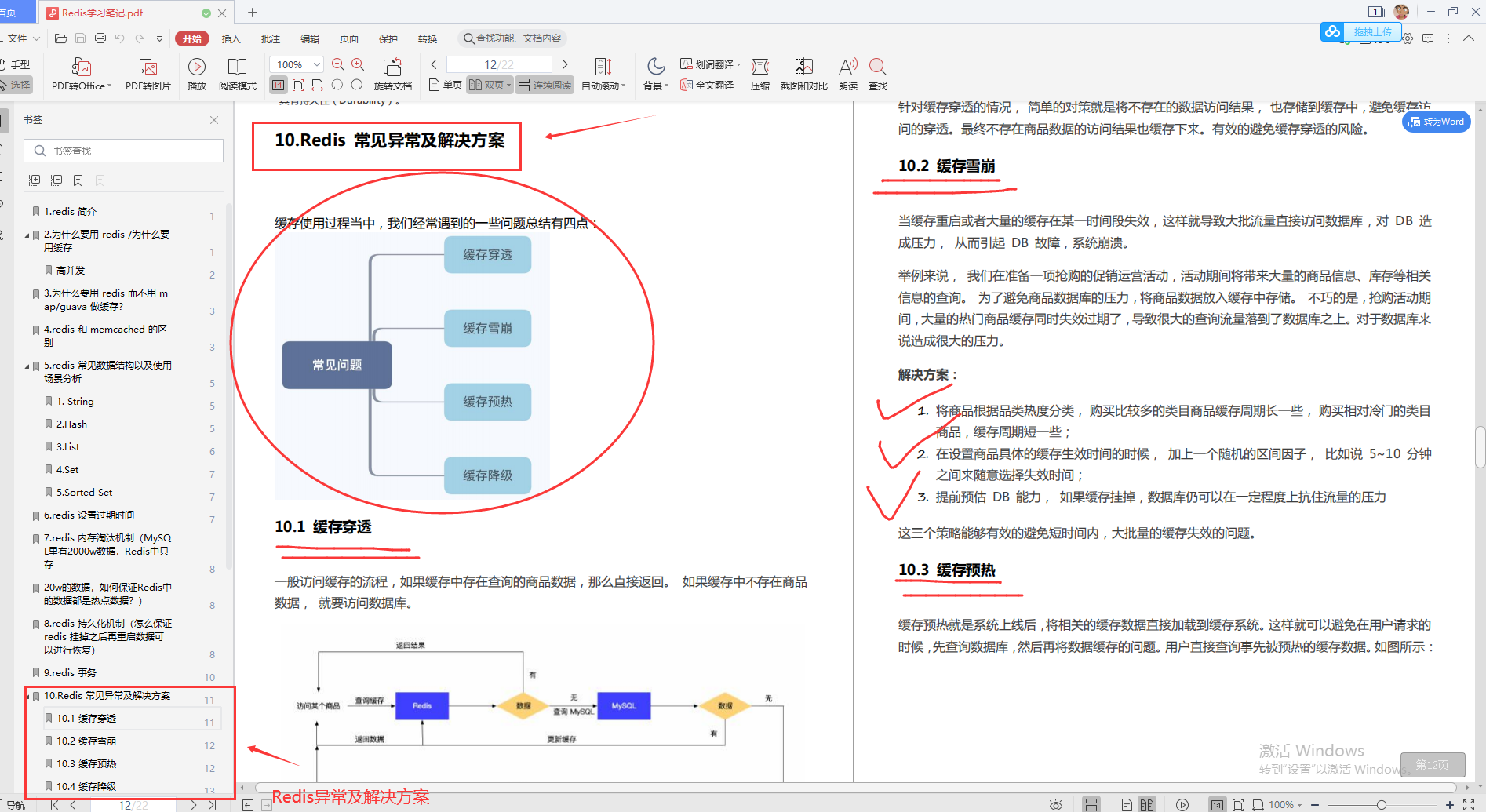 * Java Concurrent programming learning notes The fourth part is to split concurrent programming in detail -- concurrent programming+Model article+Application+Principles 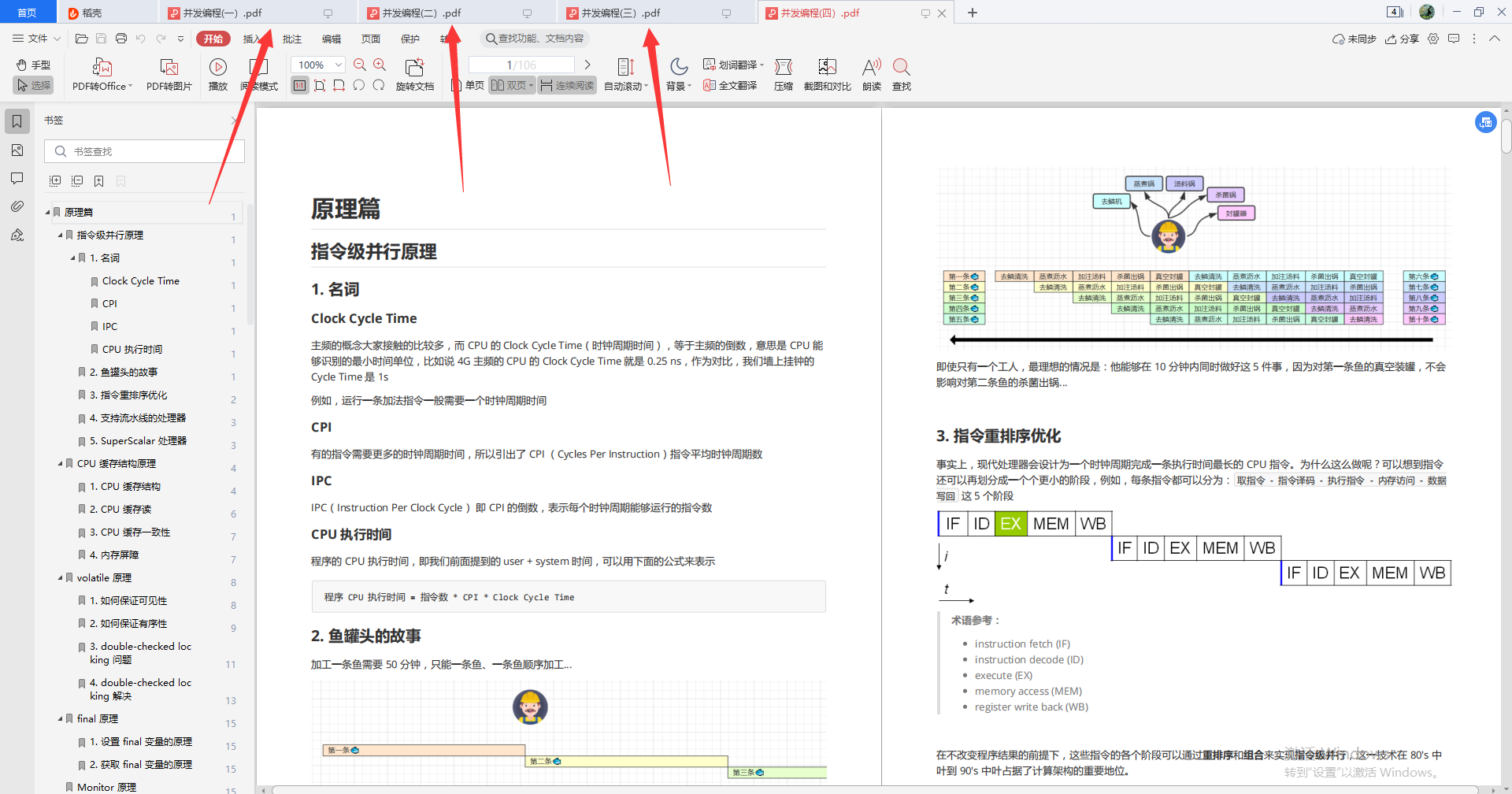 * Java Programmers must read the book in-depth understanding ava Virtual machine version 3( pdf (version) 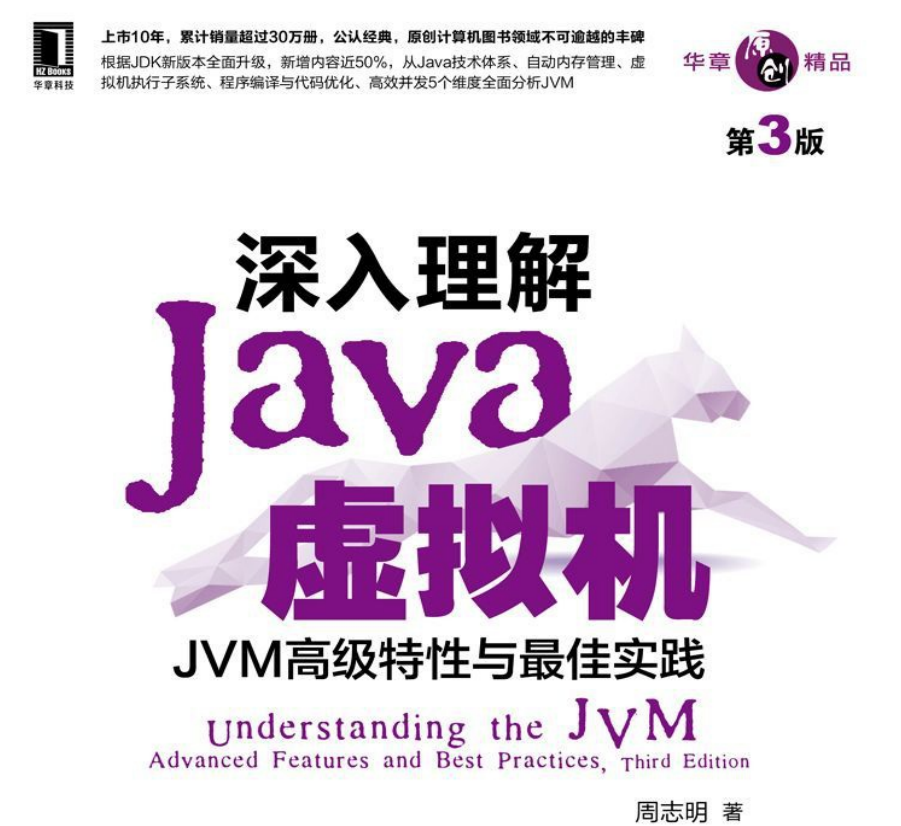 * Interview questions for large factories -- Notes on data structure and algorithm collection 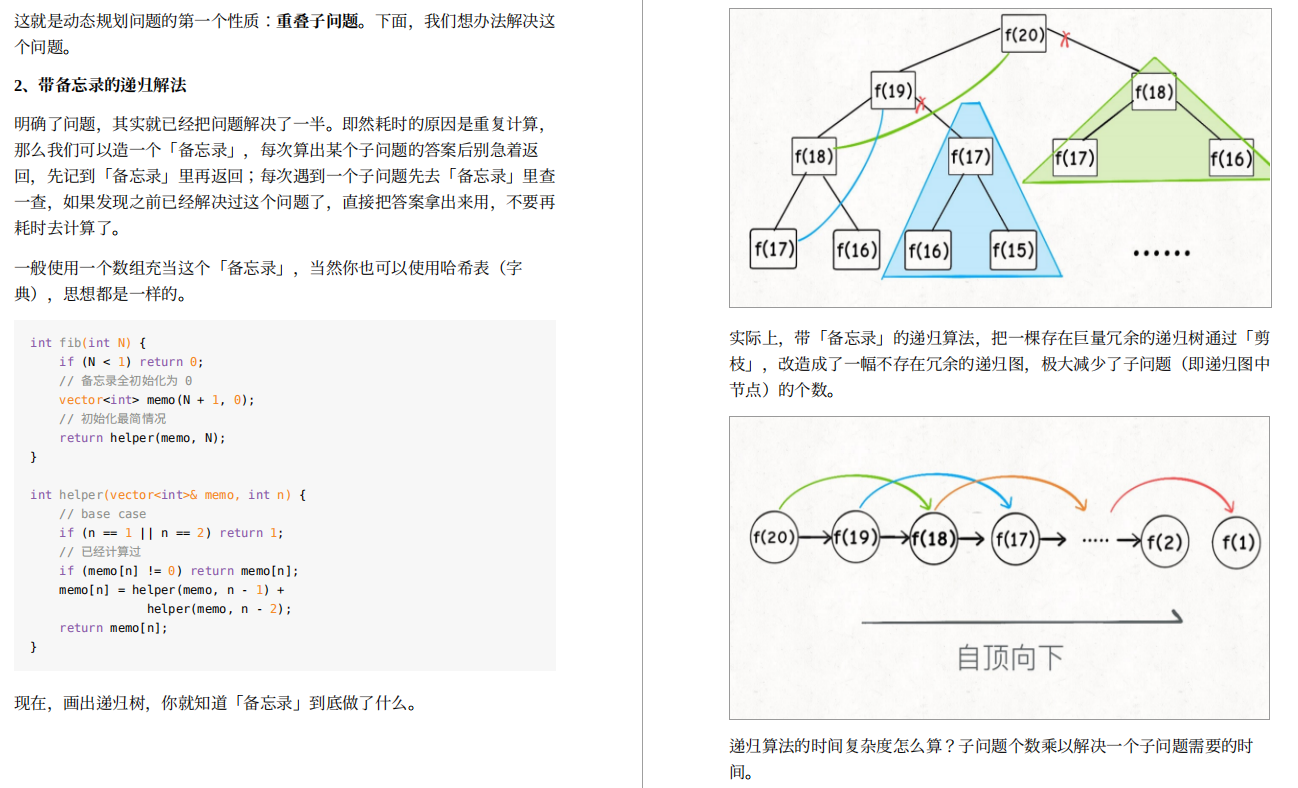 Other like Spring,SpringBoot,SpringCloud,SpringCloudAlibaba,Dubbo,Zookeeper,Kafka,RocketMQ,RabbitMQ,Netty,MySQL,Docker,K8s Wait, I've sorted it out. I won't show it one by one here. 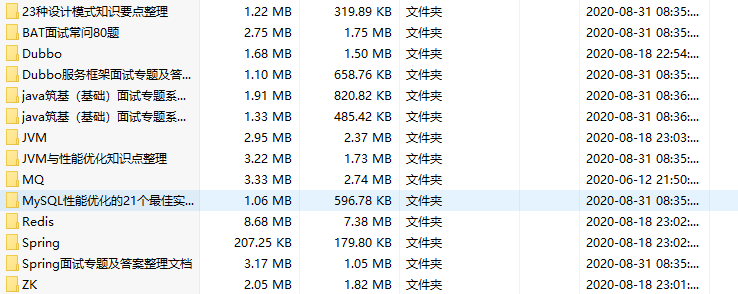 **2,Crazy brush interview questions** The technology is mainly reflected in the accumulation and practicality at ordinary times. You can review it well in two months before the interview, and then you can brush the interview questions. The following interview questions are carefully sorted out by Xiaobian and posted for everyone to see. ①Dachang high frequency 45 pen test questions (IQ questions) 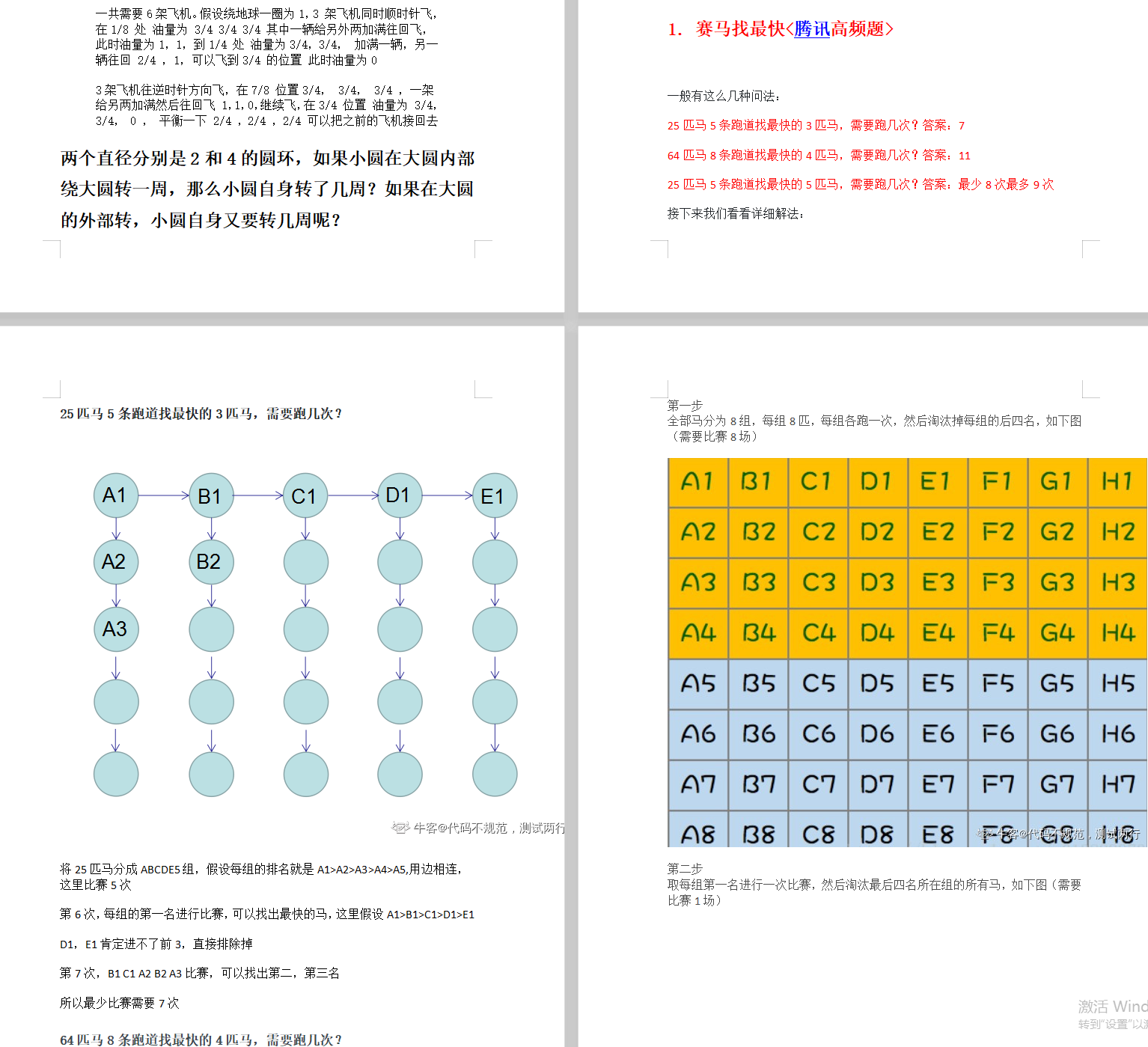 ②BAT Interview summary of large factory (screenshot of some contents) 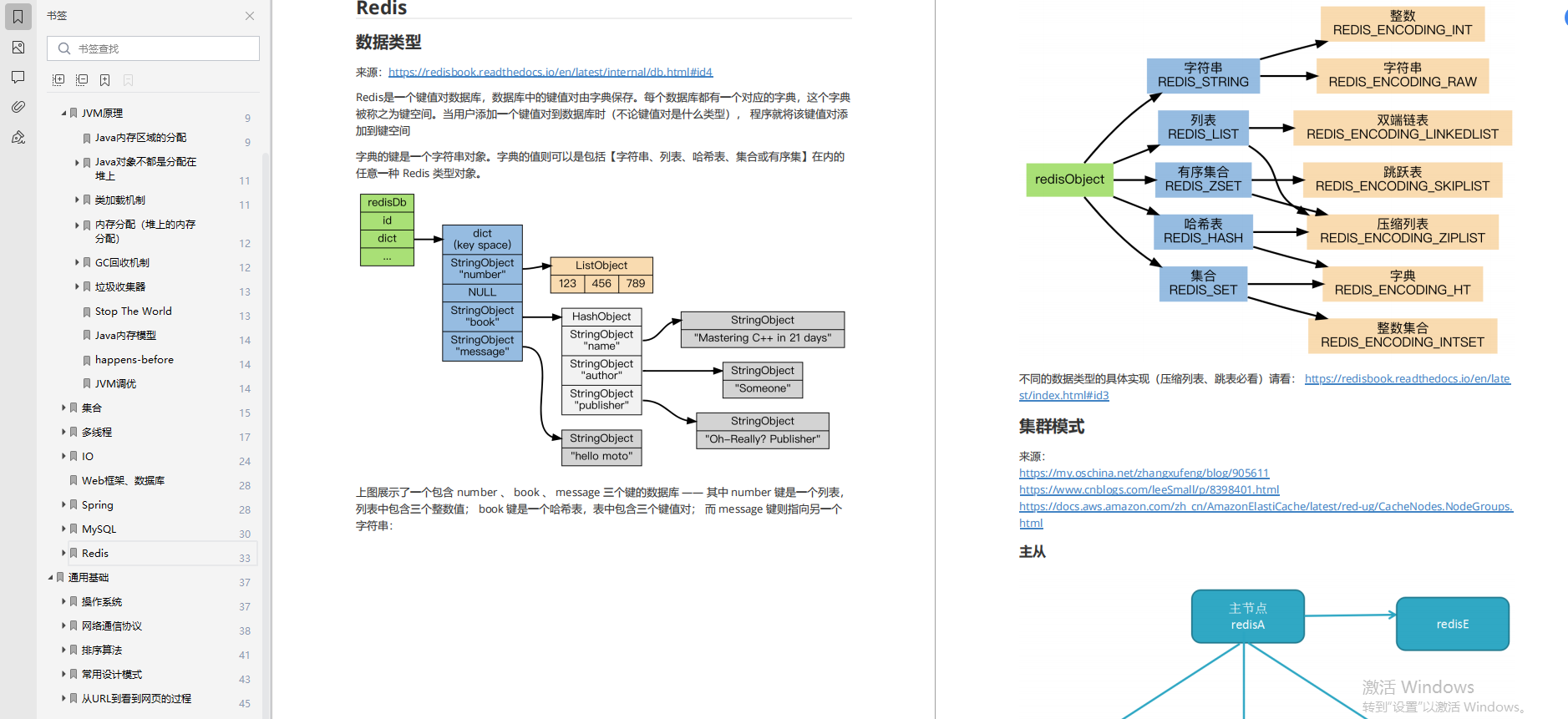 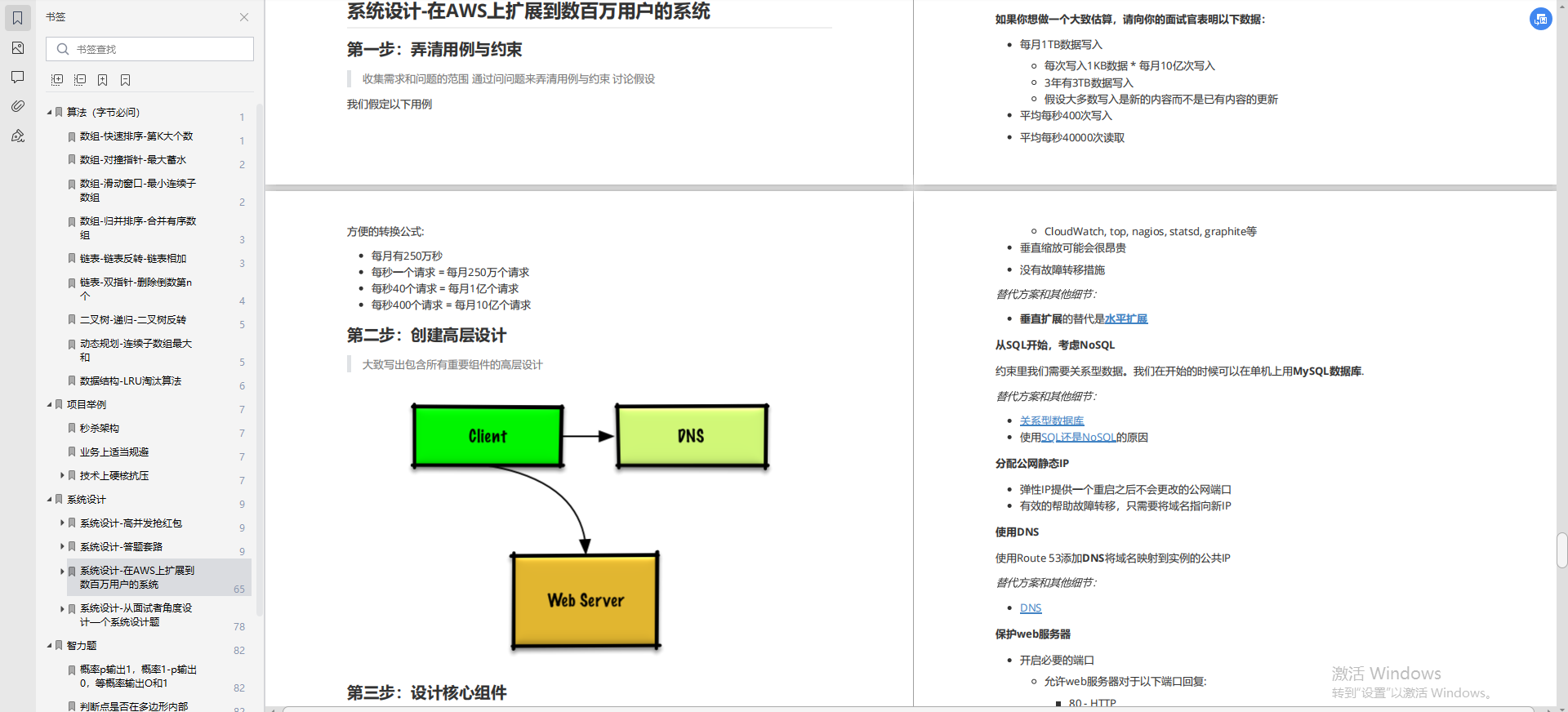 ③Interview summary 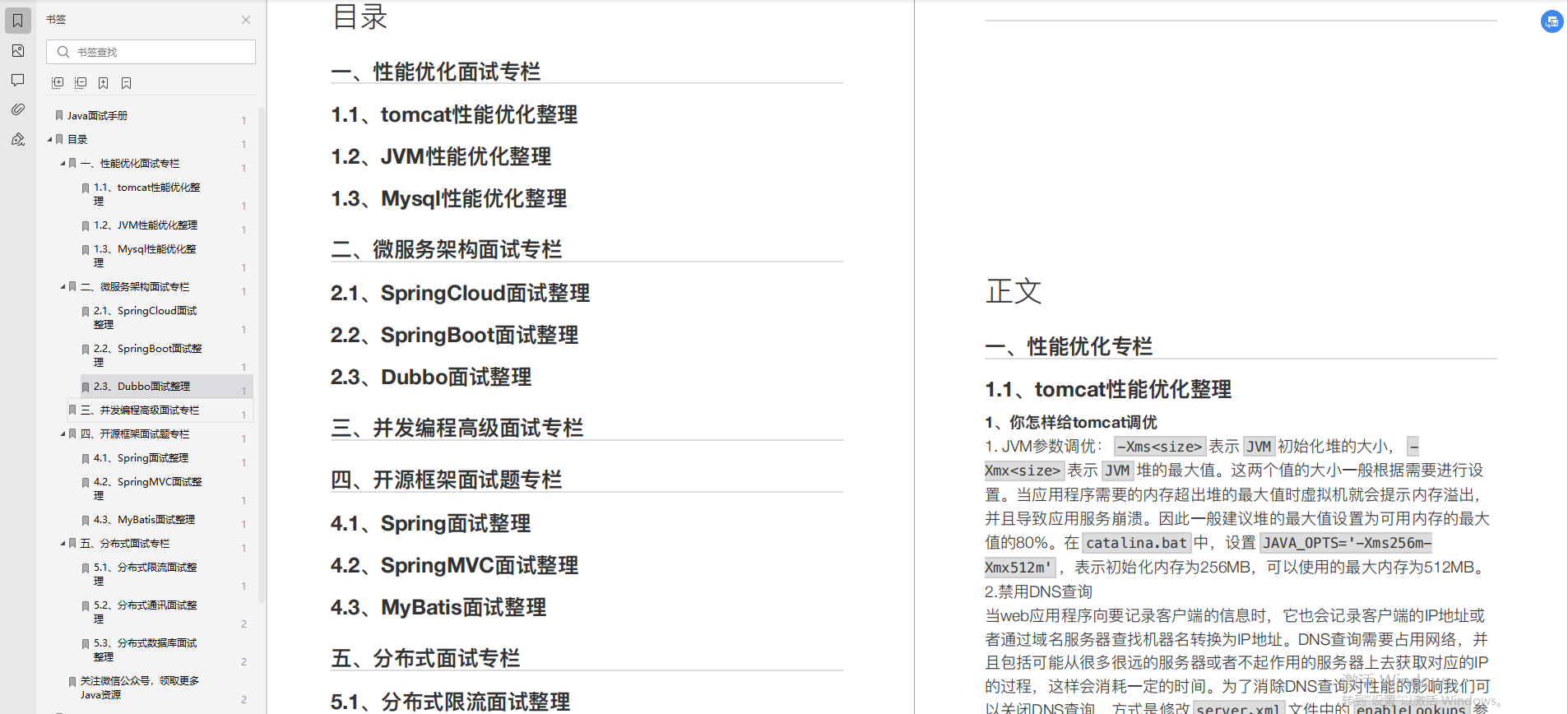 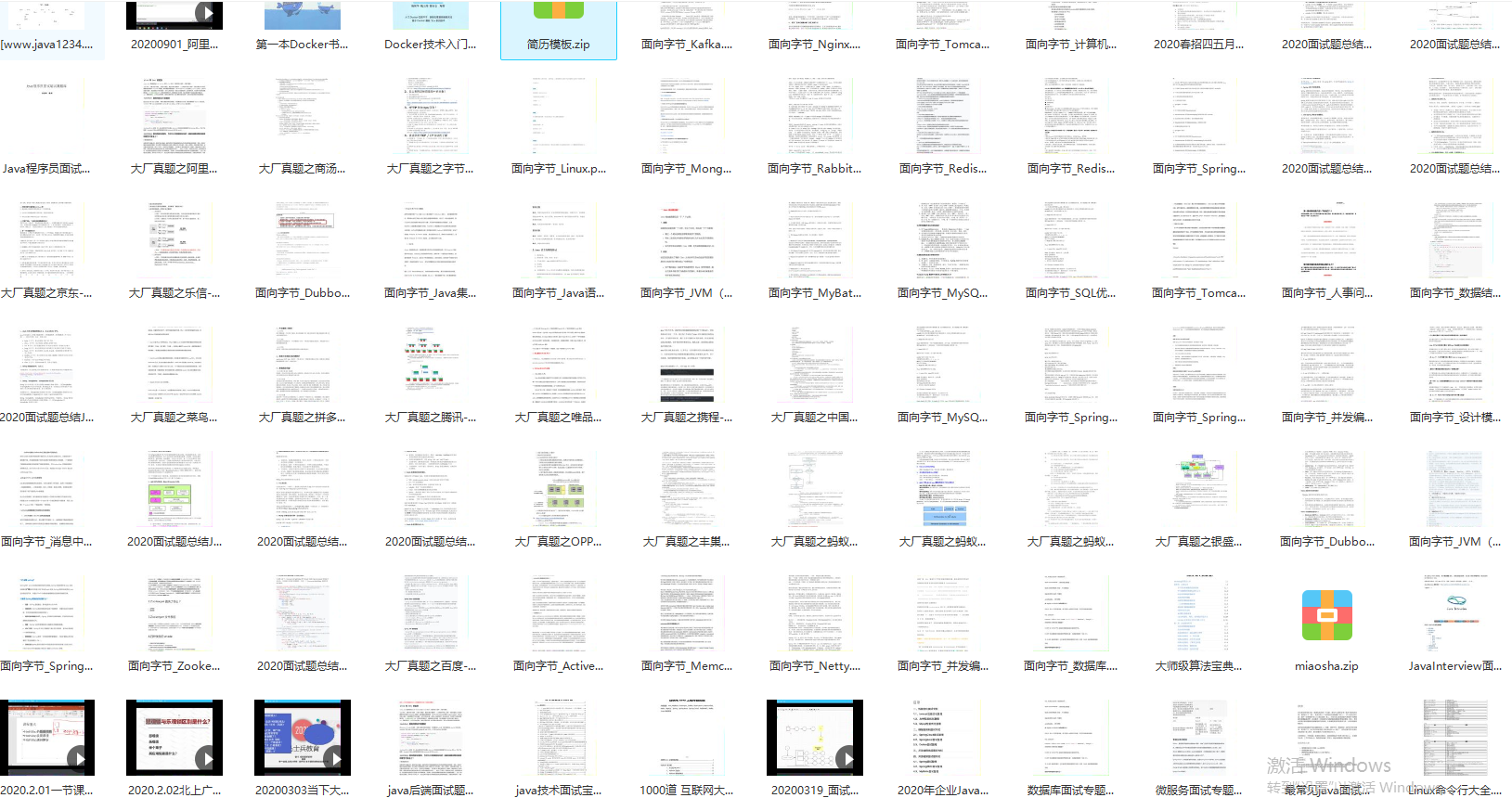 **3,Modify the resume according to the actual situation** Programmers' resumes must make more efforts, especially to think over some words. For example, the differences between "proficient, familiar and understand" must be distinguished clearly, otherwise they are digging a hole for themselves. Of course not. I can give you my resume for reference. If it's not enough, you can choose from the following resume templates: 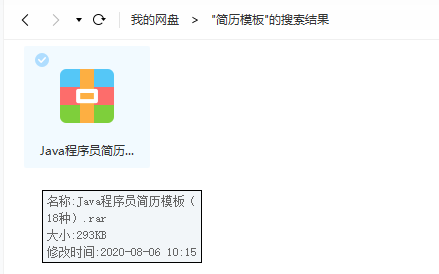 I hope you can find a good job in the golden, silver and four job hopping season, but please remember that the technology must be accumulated through work or self-study (or enrolling in class and learning from teachers) through actual combat. Don't cram temporarily. In addition, you might as well try to talk about your own ideas for the problems you won't encounter in the interview, because some problems don't examine our programming ability, but our logical thinking and expression ability; Finally, we should conduct self-analysis and evaluation, make career planning, constantly explore, and improve our programming ability and abstract thinking ability. AT Interview summary of large factory (screenshot of some contents) [External chain picture transfer...(img-BqbvsrDz-1628624018826)] [External chain picture transfer...(img-MGIzTwiS-1628624018827)] ③Interview summary [External chain picture transfer...(img-824F11hn-1628624018828)] [External chain picture transfer...(img-dV2Ka6Me-1628624018829)] **3,Modify the resume according to the actual situation** Programmers' resumes must make more efforts, especially to think over some words. For example, the differences between "proficient, familiar and understand" must be distinguished clearly, otherwise they are digging a hole for themselves. Of course not. I can give you my resume for reference. If it's not enough, you can choose from the following resume templates: [External chain picture transfer...(img-tnQl0Odn-1628624018830)] I hope you can find a good job in the golden, silver and four job hopping season, but please remember that the technology must be accumulated through work or self-study (or enrolling in class and learning from teachers) through actual combat. Don't cram temporarily. In addition, you might as well try to talk about your own ideas for the problems you won't encounter in the interview, because some problems don't examine our programming ability, but our logical thinking and expression ability; Finally, we should conduct self-analysis and evaluation, make career planning, constantly explore, and improve our programming ability and abstract thinking ability. **All notes, interview questions and other materials mentioned in the above articles can be shared for free. If necessary, you can[Poke here, pack and take away](https://gitee.com/vip204888/java-p7)**