The Notepad development of C # requires us to define two forms, that is, a parent form carrying all notepads and a child form with the basic functions of Notepad.
.
=========================================================================
So let's talk about the development of subform with the basic function of Notepad.
In the form application of C #, we can directly layout the controls on the interface without using code to define the controls. At this point, the form application development of C # is very good.
Then in the notepad application, the controls we should add to the form should include the creation, opening and saving of files, as well as the font style, font size and whether to bold and tilt these basic Notepad controls. At the same time, of course, we also need the edit text box of Notepad to facilitate our modification and input of content.
After understanding these basic controls, we can write the functions bound to each control,
First, we need to get all the font styles of the system when setting the font,
This is achieved by the following functions:
//Get system font private void FormSon_Load(object sender, EventArgs e) { //Font to load when the form is loaded InstalledFontCollection MyFontStaly = new InstalledFontCollection(); //Gets the data of the InstalledFontCollection object FontFamily[] ff = MyFontStaly.Families; //Define a new ArrayList array list ArrayList list = new ArrayList(); //Gets the length of the list to the font array int count = ff.Length; for (int i = 0; i < count; i++) { //Gets the name of the font in the font array string FontName = ff[i].Name; //Save font in font control toolStripComboBox_fonyStaly.Items.Add(FontName); } }
After obtaining all the font styles that can be set by the system, it is the control that changes the font when we click the font. Here, we need to set it in the control that changes the font index,
The functions are as follows:
//Change the index of the font private void toolStripComboBox_fonyStaly_SelectedIndexChanged(object sender, EventArgs e) { //Receives the text of the current font box string fontname = toolStripComboBox_fonyStaly.Text; //Receives the value of the current font size box float fontsize = float.Parse(toolStripComboBox_fontSize.Text); textBox_Text.Font = new Font(fontname, fontsize); }
After setting the properties of the font control, the font size property is set. Similar to the idea of setting the font control, we also need to obtain the index of changing the font size first. The function is as follows:
//Index for changing font size private void toolStripComboBox_fontSize_SelectedIndexChanged(object sender, EventArgs e) { //Receives the text of the current font box string fontname = toolStripComboBox_fonyStaly.Text; //Receives the value of the current font size box float fontsize = float.Parse(toolStripComboBox_fontSize.Text); textBox_Text.Font = new Font(fontname, fontsize); }
When the font size changes, we need to change the font size of the content in the text box. At this time, we need to set it in the corresponding function:
//When the font size changes private void toolStripComboBox_fontSize_TextChanged(object sender, EventArgs e) { //Receives the text of the current font box string fontname = toolStripComboBox_fonyStaly.Text; //Receives the value of the current font size box float fontsize = float.Parse(toolStripComboBox_fontSize.Text); textBox_Text.Font = new Font(fontname, fontsize); }
//Bold button private void toolStripButton_over_Click(object sender, EventArgs e) { //Click the bold button to bold the font. Bold is to click the button to cancel bold //If it is not bold at present, click to bold if (textBox_Text.Font.Bold == false) { textBox_Text.Font = new Font(textBox_Text.Font, FontStyle.Bold); } //If it is currently in bold status, click Cancel bold else { textBox_Text.Font = new Font(textBox_Text.Font, FontStyle.Regular); } }
//Tilt button private void toolStripButton_slanr_Click(object sender, EventArgs e) { if (textBox_Text.Font.Italic == false) { textBox_Text.Font = new Font(textBox_Text.Font, FontStyle.Italic); } else { textBox_Text.Font = new Font(textBox_Text.Font, FontStyle.Regular); } }
When making the save control, we need to consider whether the file information we save is a new text box. If we create a new Notepad, we need to select the save path and file name when saving. If we edit the original file twice, we only need to save when clicking the Save button, There is no need to reselect the save path of the file.
The function code is as follows:
//Save button private void toolStripButton_save_Click(object sender, EventArgs e) { //Create a filter saveFileDialog1.Filter = ("Text document(*,txt)|*.txt"); // Judge whether to save if the current text box is not empty if (textBox_Text.Text.Trim() != "") { //If the notepad file is new, select the path to save if (this.Text == "Notepad") { //Save the file to the user's specified directory //Judge whether the user saves or cancels if (saveFileDialog1.ShowDialog() == DialogResult.OK) { //Obtain the file and path selected by the user string path = saveFileDialog1.FileName; //Save the file to the specified path StreamWriter sw = new StreamWriter(path, false); sw.WriteLine(textBox_Text.Text.Trim()); //Show path above form this.Text = path; //wipe cache sw.Flush(); sw.Close(); } } //If it is an open file, save it directly else { //Save the file to the user's specified directory //Obtain the file and path selected by the user string path = this.Text; //Save the file to the specified path StreamWriter sw = new StreamWriter(path, false); sw.WriteLine(textBox_Text.Text.Trim()); sw.Flush(); sw.Close(); } } //If it is blank, a message box prompt will be given else { MessageBox.Show("The current text box is empty!\n Cannot save!", "warning"); } }
When opening files, we need to filter the opened files. We only need to open text files in txt format.
The implementation functions are as follows:
//Open file private void toolStripButton_open_Click(object sender, EventArgs e) { //Filter an open file openFileDialog1.Filter = ("Text document(*,txt)|*.txt"); if (openFileDialog1.ShowDialog() == DialogResult.OK) { string path = openFileDialog1.FileName; StreamReader sr = new StreamReader(path, Encoding.UTF8); string text = sr.ReadToEnd(); //Displays the path above the text box this.Text = path; textBox_Text.Text = text; sr.Close(); } }
After clicking new text, we need to empty the original edited content and re-establish the editing.
The functions are as follows:
//New text private void toolStripButton1_Click(object sender, EventArgs e) { this.Text = "Notepad"; textBox_Text.Text = ""; toolStripTextBox1.Text = ""; # Ending **Tip: Due to the limited length of the article, there are 20 more about MySQL I'll make a copy of all the questions pdf I'll show you the list of the remaining problems,[Click here to unlock all contents!](https://gitee.com/vip204888/java-p7)** **If you find it helpful, you may wish to [forward]+give the thumbs-up+Attention] support me, and more technical articles and learning articles will be brought to you in the future!**(Ali yes MySQL The underlying implementation and index implementation ask a lot) 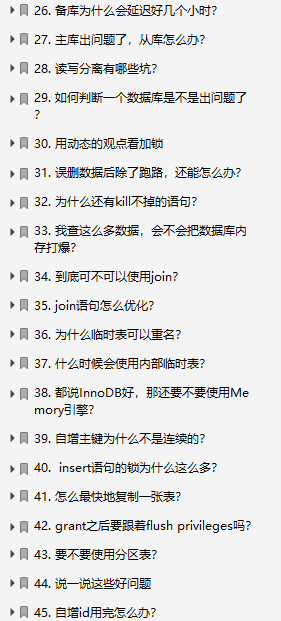 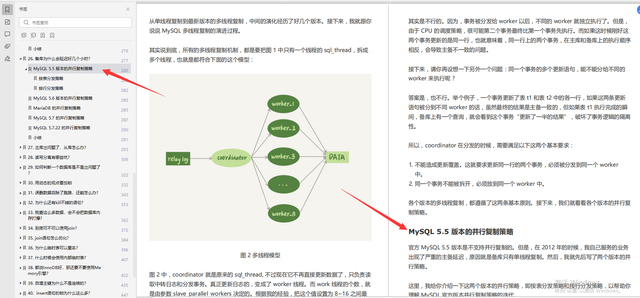 //New text private void toolStripButton1_Click(object sender, EventArgs e) { this.Text = "Notepad"; textBox_Text.Text = ""; toolStripTextBox1.Text = ""; # Ending **Tip: Due to the limited length of the article, there are 20 more about MySQL I'll make a copy of all the questions pdf I'll show you the list of the remaining problems,[Click here to unlock all contents!](https://gitee.com/vip204888/java-p7)** **If you find it helpful, you may wish to [forward]+give the thumbs-up+Attention] support me, and more technical articles and learning articles will be brought to you in the future!**(Ali yes MySQL The underlying implementation and index implementation ask a lot) [External chain picture transfer...(img-cZ31bOjf-1628665602865)] [External chain picture transfer...(img-ld76U9XK-1628665602868)] After eating through this pdf,You can also talk to the interviewer MySQL. Actually, it's like Ali p7 The job demand is not so difficult (but not simple), solid Java Basics+No short board knowledge+Deep learning of some open source technologies+Read the source code+Algorithm brush questions, this set down p7 There's almost no problem with the post. I still hope everyone can get a high salary offer All right.