- π¬ Turntable lottery applet production
- π³οΈπ Step 1: open UnityHub and create a new Unity project
- π³οΈπ Step 2: build a simple turntable lottery UI
- π³οΈπ Step 3: write the script code of the turntable lottery
- π³οΈπ Step 4: improve the lottery mechanism and increase the controllable lottery probability
- π Source code project Download
- π₯ summary
π’ preface
- Today, I'll bring you a small project of turntable lottery
- In the daily game development, the demand for lottery is super common
- Let's do this small project today. By the way, let's know what's inside about the lottery!
π¬ Turntable lottery applet production
First, let's talk about the composition of the turntable lottery applet.
The turntable lottery is mainly composed of two parts, one is the turntable and the other is the pointer.
There are also two kinds of rotation logic. One is that the pointer does not move and the turntable rotates; The other is that the turntable does not move and the pointer rotates.
In either case, the principle is the same. We just need to change the rotating object to the one we want.
Therefore, the idea of using a fixed pointer and rotating the turntable is adopted here!
Let's introduce the production process of this turntable lottery applet step by step!
π³οΈπ Step 1: open UnityHub and create a new Unity project
Don't explain too much about the new project. You have to cook and buy vegetables first!
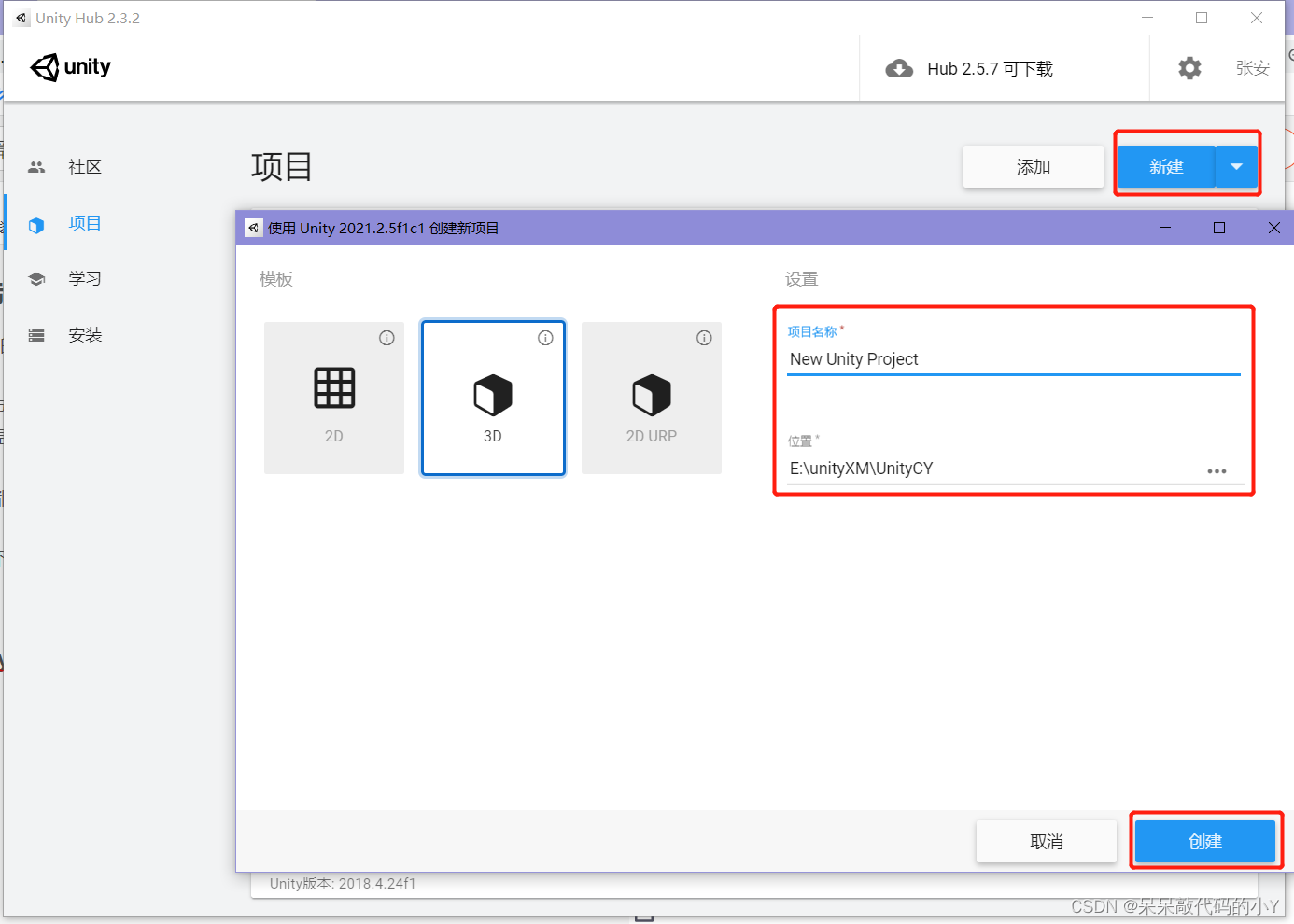
Open UnityHub to create a new project. The Unity version is optional, and then wait until the project is loaded.
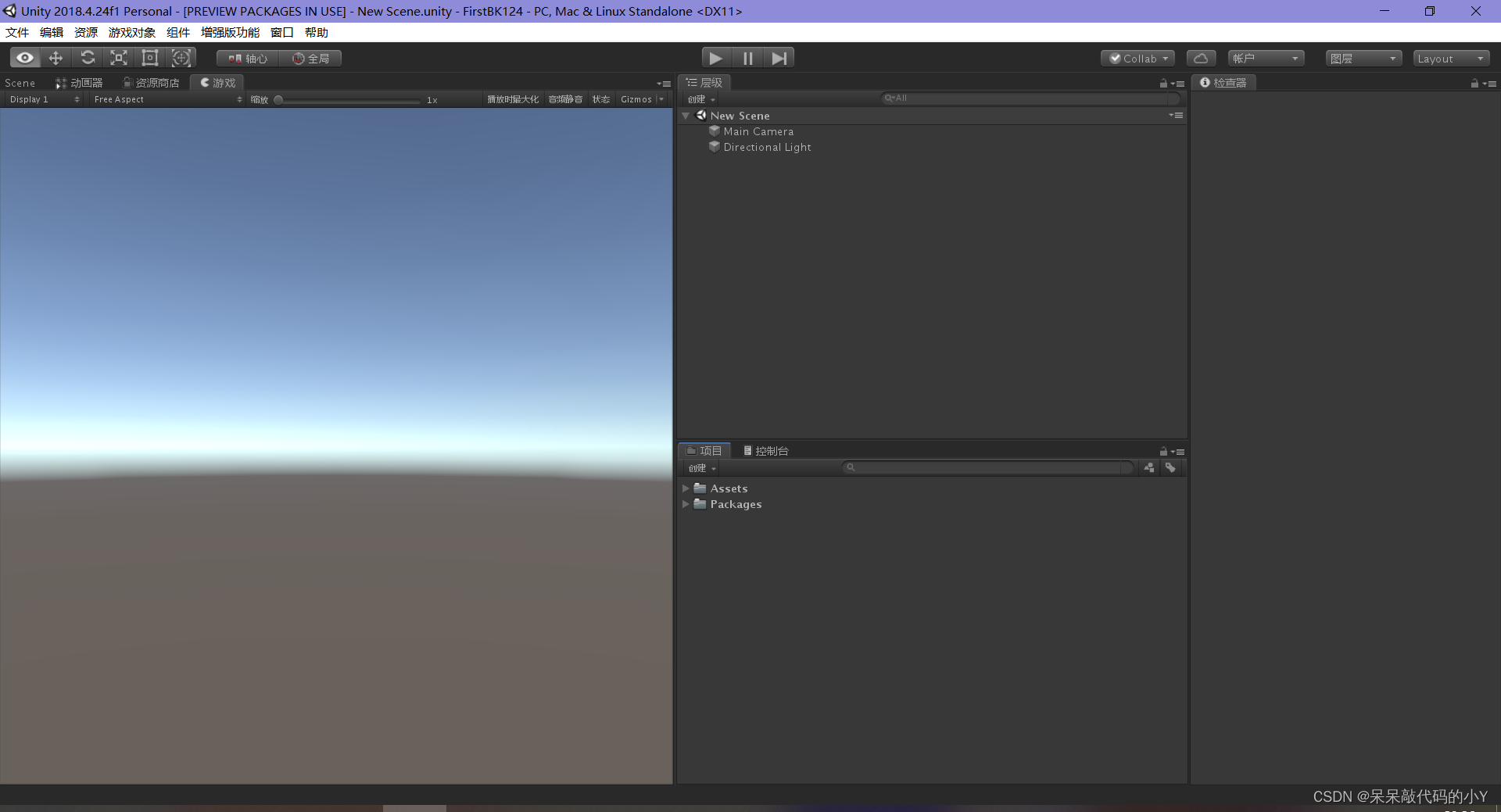
Then create a new Scene scene and a Scripts script under Assets.
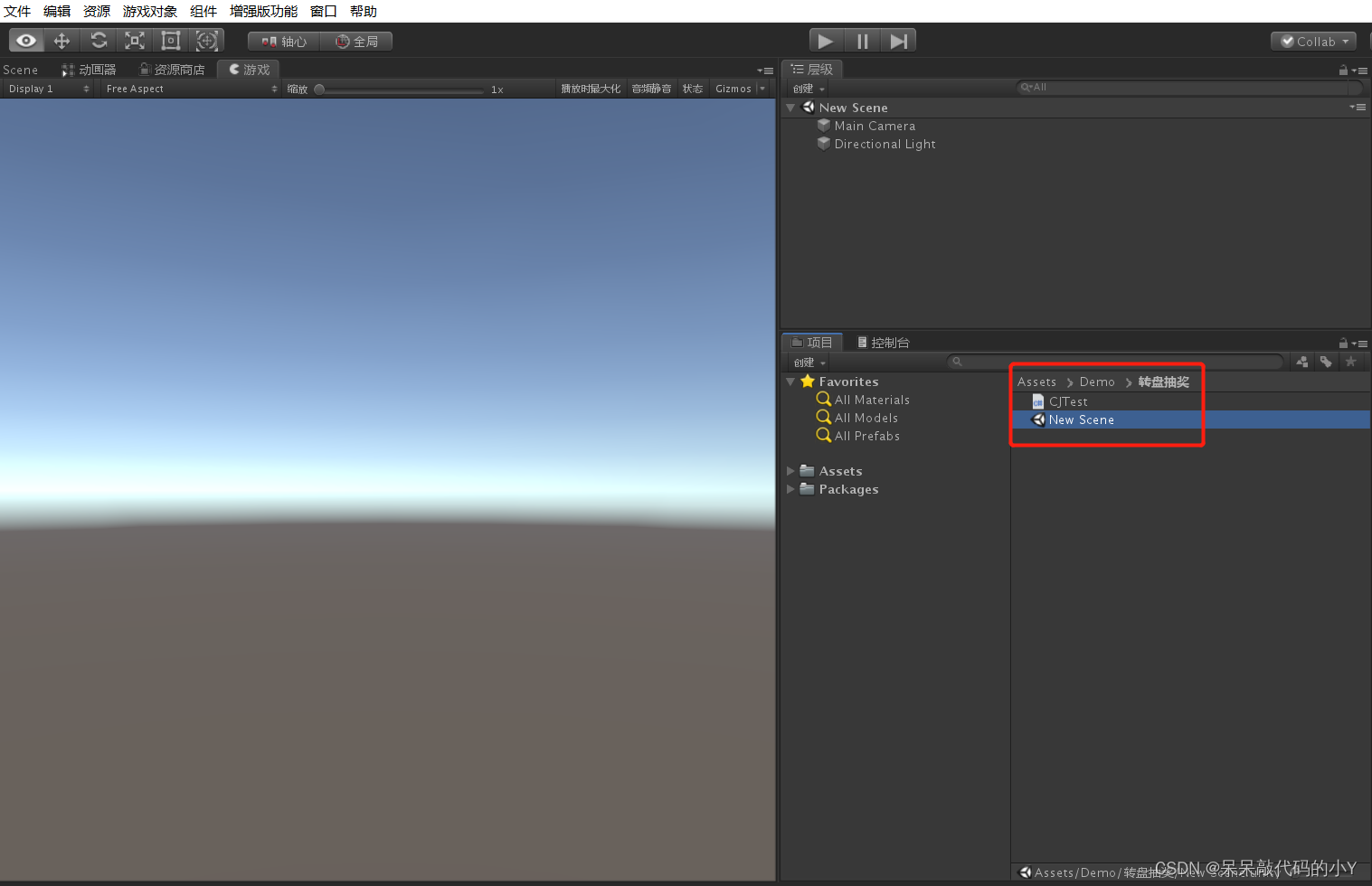
After the preparation process, let's start making this small project of the turntable lottery!
π³οΈπ Step 2: build a simple turntable lottery UI
Since it's a turntable lottery, let's first build a simple lottery UI screen!
Let's take a look at a UI I've simply built, with a turntable and pointer, and a button to start and stop the lottery.
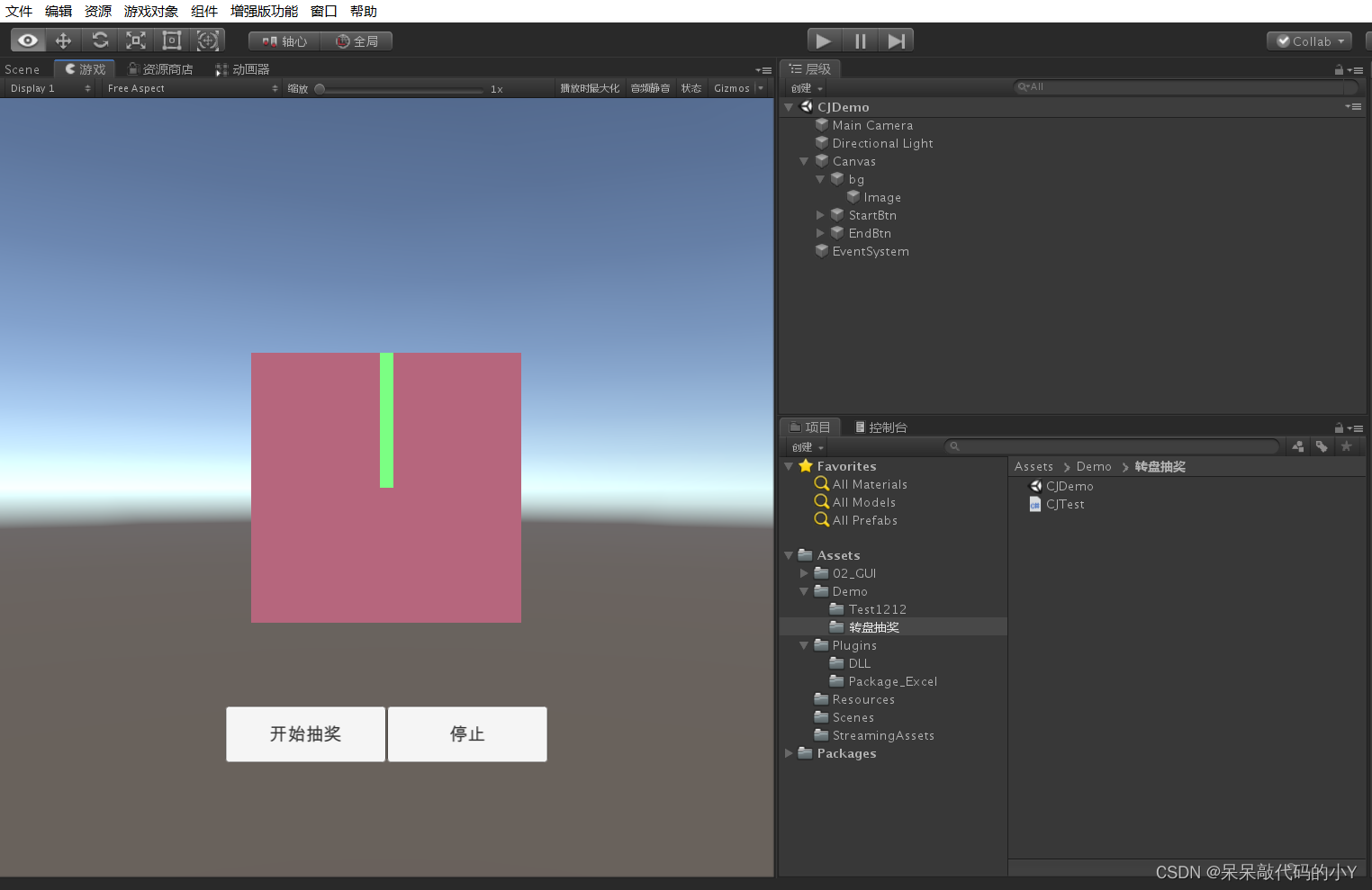
It looks simple. It doesn't matter! We first set up the shelf, and then we can find the material and change it with one click!
Just add the key elements of the turntable lottery. The key elements here refer to the turntable and pointer
π³οΈπ Step 3: write the script code of the turntable lottery
Since it is a small project, natural code is an essential part.
Now let's write the script code of the turntable lottery.
Direct code:
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class zDemo : MonoBehaviour { /// <summary> ///Four states of rotary table rotation /// </summary> public enum RollState { None, SpeedUp, SpeedDown, End } public Transform RollPanel;//Rotating turntable public Button startButton;//Start lottery button RollState curState; //Status of current turntable float allTime = 0;//Total rotation time #region transmission section float MaxSpeed = 1000;//Maximum speed float factor;//Velocity factor float accelerateTime = 1;//Time to accelerate to the maximum speed --- tentatively 1 float speedUpTime = 3;//Total time of acceleration period float bufferSpeed=1;//Slow down #endregion void Start() { startButton.onClick.AddListener(StartTurnWheel); } void Update() { if (curState == RollState.None) { return; } allTime += Time.deltaTime; //Enter the acceleration phase if (curState == RollState.SpeedUp) { factor = allTime / accelerateTime; factor = factor > 1 ? 1 : factor; Debug.Log("During acceleration:" + factor); //Turn the turntable RollPanel.Rotate(new Vector3(0, 0, -1) * factor * MaxSpeed * Time.deltaTime, Space.Self); } //When the rotation time is greater than or equal to the acceleration period, the deceleration begins if (allTime >= speedUpTime && curState == RollState.SpeedUp) { bufferSpeed -= Time.deltaTime; bufferSpeed = bufferSpeed > 0 ? bufferSpeed : 0; Debug.Log("During deceleration:"+ bufferSpeed); RollPanel.Rotate(new Vector3(0, 0, -1) * bufferSpeed * 500 * Time.deltaTime, Space.Self); if (bufferSpeed <= 0) { curState = RollState.SpeedDown; } } //After deceleration, the rotary table stops rotating if (curState == RollState.SpeedDown) { Debug.Log("The turntable has stopped!"); curState = RollState.None; bufferSpeed = 1; } } /// <summary> ///Start rotating the turntable /// </summary> public void StartTurnWheel() { if (curState != RollState.None) { return; } allTime = 0; curState = RollState.SpeedUp; } }
Hang the script on the Canvas in the scene, and then assign a value to the Button that starts the lottery.
And the game object of the turntable should also be dragged into the script, and then you can run the program to see the effect!
The effect demonstration is as follows:
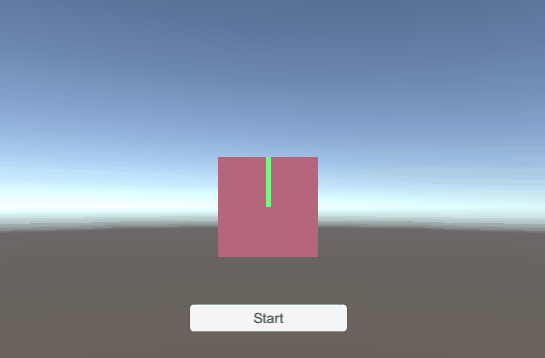
The above script code can rotate the turntable.
But he just makes a random rotation, and we can't control its rotation angle.
That is to say, the probabilities at all angles of the turntable are the same.
But how can this work! It's too fair. Aren't all the awards going to be taken away by others!
So please see the next step to improve the code and increase the lottery probability!
π³οΈπ Step 4: improve the lottery mechanism and increase the controllable lottery probability
We must increase the probability of task control to improve the script code!
By the way, find the material of a turntable and change it for him. Just change the turntable and pointer!
Improved script code:
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class NewLuckyRoll : MonoBehaviour { /// <summary> ///Four states of rotary table rotation /// </summary> public enum RollState { None, SpeedUp, SpeedDown, End } public GameObject ResultUI; public Text resultTxt; public Transform RollPanel;//Rotating turntable public Button startBtn; RollState curState; //Status of current turntable int rollID;//Rotation result ID -- the turntable of this case is divided into 6 copies float allTime = 0;//Total rotation time float endAngle;//Final angle #region transmission section float MaxSpeed = 1500;//Maximum speed float factor;//Velocity factor float accelerateTime = 1;//Time to accelerate to the maximum speed --- tentatively 1 float speedUpTime = 3;//Total time of acceleration period float tempAngle;//The rotation angle of the turntable at this time when starting the deceleration section float k = 2f; //The speed coefficient of deceleration stage - deceleration speed is determined by this #endregion void Start() { rollID = 0; ResultUI.SetActive(false); startBtn.onClick.AddListener(StartTurnWheel); } void Update() { if (curState == RollState.None) { return; } allTime += Time.deltaTime; //Advanced into acceleration phase if (curState == RollState.SpeedUp) { factor = allTime / accelerateTime; factor = factor > 1 ? 1 : factor; RollPanel.Rotate(new Vector3(0, 0, -1) * factor * MaxSpeed * Time.deltaTime, Space.Self); } //When the rotation time is greater than or equal to the acceleration period, the deceleration begins if (allTime >= speedUpTime && curState == RollState.SpeedUp) { curState = RollState.SpeedDown; tempAngle = GetTempAngle(); Debug.Log("tempAngle:" + tempAngle); } if (curState == RollState.SpeedDown) { //Rotate to the specified angle through difference operation (spherical interpolation cannot realize calculation greater than 360 °) tempAngle = Mathf.Lerp(tempAngle, endAngle, Time.deltaTime * k); RollPanel.rotation = Quaternion.Euler(0, 0, tempAngle); //RollPanel.eulerAngles = new Vector3(0, 0, tempAngle); //End of rotation if (Mathf.Abs(tempAngle - endAngle) <= 1) { curState = RollState.None; ResultUI.SetActive(true); } } } /// <summary> ///Start rotating the turntable /// </summary> public void StartTurnWheel() { ResultUI.SetActive(false); if (curState != RollState.None) { return; } allTime = 0; tempAngle = 0; rollID = GetRandomID(); Debug.Log("rollID: " + rollID); endAngle = (-1) * rollID * 55; curState = RollState.SpeedUp; } /// <summary> ///Get the rotation angle of the current turntable /// </summary> /// <returns></returns> private float GetTempAngle() { Debug.Log("RollPanel.eulerAngles.z: " + RollPanel.eulerAngles.z); return (360 - RollPanel.eulerAngles.z) % 360; } //(0,60)(60,120)(120,180)(180,210)(210,270)(270,360) //Get the result of rotation, set the probability, and then get the result randomly. We can customize the probability private int GetRandomID() { //The turntable is inverted, because it rotates clockwise, the rotation angle is negative int id = 0; int a = Random.Range(0, 100); if (a <= 1)//%1 { id = 6; resultTxt.text = "Congratulations on getting 1 G flow"; } else if (1 < a && a <= 3) //%2 { id = 5; resultTxt.text = "Congratulations on getting 500 M flow"; } else if (3 < a && a <= 10) //%7 { id = 4; resultTxt.text = "Congratulations on getting 100 M flow"; } else if (10 < a && a <= 30) // %20 { id = 10; resultTxt.text = "Congratulations on getting 50 M flow"; } else if (30 < a && a <= 60) //%30 { id =9; resultTxt.text = "Congratulations on getting 30 M flow"; } else //%40 { id = 8; resultTxt.text = "Congratulations on getting 10 M flow"; } return id; } }
The effects are as follows:
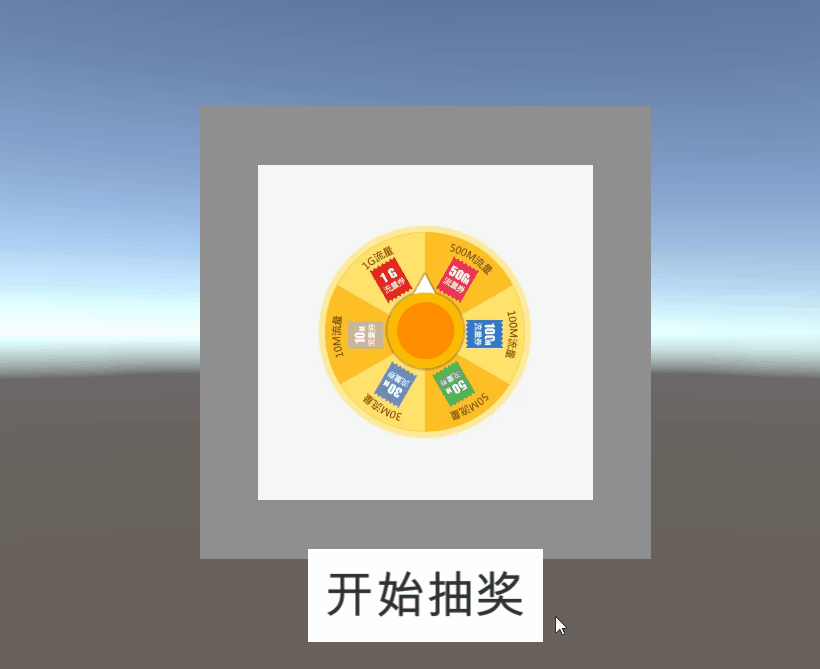
OK, a simple lucky draw rotation is written. After changing to a simple UI, there is still a model!
The code adds a lucky draw probability. The moment we click the lucky draw, in fact, the prize already has the result.
It just adds a circle process and delays our time.
Moreover, from the perspective of lucky draw, the probability of each award should be the same. After all, it is six equal points!
However, we have already added a probability in the code, and the probability of winning the largest award is only 1%
So sometimes the fairness we see on the surface is an illusion!
The probability behind the scenes has already been written, waiting for you to enter the pit.
π Source code project Download
Even if the small program of the turntable lottery is finished, the source code project is here. You can download the experience you need.
Download address of turntable lottery items: https://download.csdn.net/download/zhangay1998/68820401
π₯ summary
- This article makes a simple "big turntable lottery" small program
- Just one script can do it. Isn't it very simple and practical!
- With this small item of the turntable lottery, we can use it during the lottery.
- We can replace the UI with our own prizes, and then use the code to customize the lottery probability.
- Such a simple turntable applet is completed, and the lottery probability can be controlled by yourself.
- That's the end of this article. If you like it, remember to have three waves in a row!