- js runs inside the js engine in the browser's kernel
Node.js is a JavaScript program that runs out of the browser environment and is based on the V8 engine (Chrome's JavaScript engine)
3. Server side application development (understand)
Create 02 server app js
const http = require('http'); http.createServer(function (request, response) { // Send HTTP header // HTTP status value: 200: OK // Content type: text/plain response.writeHead(200, {'Content-Type': 'text/plain'}); // Send response data "Hello World" response.end('Hello Server'); }).listen(8888); // The terminal prints the following information console.log('Server running at http://127.0.0.1:8888/');
Run the server program
node 02-server-app.js
After the server starts successfully, enter in the browser: http://localhost:8888/ View the successful operation of the web server and output the html page
Stop service: ctrl + c
NPM
=======
1, Introduction to npm
The full name of NPM is Node Package Manager JS package management tool is the world's largest module ecosystem, in which all modules are open source and free; It's also a node JS package management tool, which is equivalent to Maven on the front end.
1. Installation location of NPM tools
Through npm, we can easily download js library and manage front-end projects.
Node.js the location of the npm package and tools installed by default: node JS directory \ node_modules
- In this directory, you can see the npm directory. npm itself is a tool managed by the npm package manager to explain node JS has integrated npm tools
#Enter npm -v at the command prompt to view the current npm version npm -v
2, Managing projects with npm
1. Create folder npm
2. Project initialization
#Create an empty folder and enter the folder at the command prompt to execute command initialization npm init #Enter relevant information according to the prompt. If the default value is used, press enter directly. #Name: project name #Version: project version number #Description: item description #keywords: {Array} keyword, which is convenient for users to search our project #Finally, a package. XML file is generated JSON file, which is the package configuration file, is equivalent to maven's POM xml #We can also modify it as needed later.
#If you want to directly generate package JSON file, you can use the command
npm init -y
### 3. Modify npm image NPM The official management packages are from [http://npmjs. com]( https://gitee.com/vip204888/java-p7 )Download, but this website is very slow in China. Taobao is recommended here NPM image [http://npm. taobao. org/]( https://gitee.com/vip204888/java-p7 ), Taobao NPM image is a complete npmjs Com image. The synchronization frequency is once every 10 minutes to ensure that it is synchronized with the official service as much as possible. **Set mirror address:**
#After the following configuration, all NPM installations will be downloaded through the image address of Taobao in the future
npm config set registry https://registry.npm.taobao.org
#View npm configuration information
npm config list
### 4. * * use of npm install command**
#Use npm install to install the latest version of the dependent package,
#Module installation location: project directory \ node_modules
#The installation will automatically add package lock. In the project directory JSON file, which helps lock the version of the installation package
#Also package In the JSON file, the dependency package will be added to the dependencies node, similar to that in maven
npm install jquery
#npm managed projects generally do not carry nodes during backup and transmission_ Modules folder
npm install # according to package The configuration download dependency in JSON initializes the project
#If you want to specify a specific version during installation
npm install jquery@2.1.x
#devDependencies node: the dependency package during development, which is not included when the project is packaged into the production environment
#Use the - D parameter to add dependencies to the devDependencies node
npm install --save-dev eslint
#Or
npm install -D eslint
#Global installation
#Node.js location of npm packages and tools installed globally: user directory \ AppData\Roaming\npm\node_modules
#Some command-line tools often use global installation
npm install -g webpack
### 5. Other commands
#Update package (update to the latest version)
npm update package name
#Global Update
npm update -g package name
#Uninstall package
npm uninstall package name
#Global uninstall
npm uninstall -g package name
* * * **Babel** ========= I Babel brief introduction --------- Babel Is a widely used transcoder, which can ES6 Code to ES5 Code to execute in an existing environment. That means you can use it now ES6 Write programs without worrying about whether the existing environment supports them. 2, Installation ----
npm install --global babel-cli
#Check whether the installation is successful
babel --version
III Babel Use of ---------- ### 1. Initialize project
npm init -y
### 2. Create file src/example.js Here is a paragraph ES6 code:
//Before transcoding
//Define data
let input = [1, 2, 3]
//Add + 1 to each element of the array
input = input.map(item => item + 1)
console.log(input)
### 3. Configuration babelrc Babel Your profile is.babelrc,It is stored in the root directory of the project. This file is used to set transcoding rules and plug-ins. The basic format is as follows.
{
"presets": [], "plugins": []
}
presets Field to set transcoding rules es2015 Rule join .babelrc:
{
"presets": ["es2015"], "plugins": []
}
### 4. Install transcoder
npm install --save-dev babel-preset-es2015
### 5. Transcoding
Transcoding results are written to a file
mkdir dist1
The - out file or - o parameter specifies the output file
babel src/example.js --out-file dist1/compiled.js
perhaps
babel src/example.js -o dist1/compiled.js
Transcoding the entire directory
mkdir dist2
The - out dir or - d parameter specifies the output directory
babel src --out-dir dist2
perhaps
babel src -d dist2
* * * **Webpack** =========== I Webpack brief introduction ----------- Webpack Is a front-end resource load/Packaging tools. It will conduct static analysis according to the dependencies of modules, and then generate corresponding static resources according to the specified rules. As we can see from the picture, Webpack Multiple static resources can be js,css,less Converting to a static file reduces page requests. 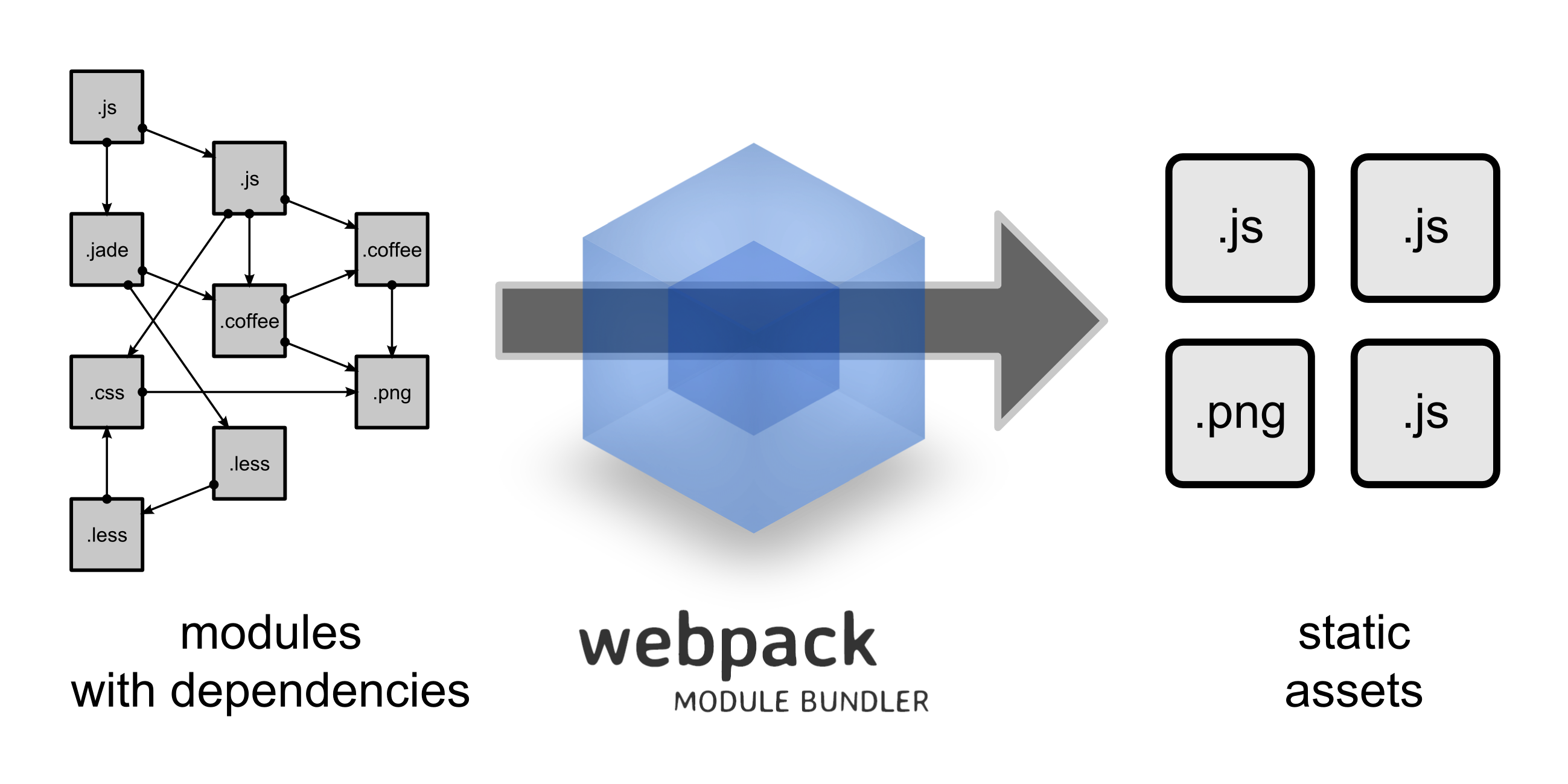 II Webpack install ------------ ### 1. Global installation
npm install -g webpack webpack-cli
### 2. View version number after installation
webpack -v
3, Initialize project ------- ### 1. Create webpack folder get into webpack Directory, executing commands
npm init -y
### 2. Create src folder ### 3. * * create common under * * * * src js**
exports.info = function (str) {
document.write(str);
}
### 4. * * create utils under src js**
exports.add = function (a, b) {
return a + b;
}
### 5. * * create main under src js**
const common = require('./common');
const utils = require('./utils');
common.info('Hello world!' + utils.add(100, 200));
IV JS pack # last In fact, it can be seen from the recruitment needs of large factories that high concurrent experience in recruitment requirements is preferred, including many friends who used to work in traditional industries or outsourcing projects. They have been working in small companies. The technology is relatively simple, and they have not done much distributed systems, but now Internet companies generally do distributed systems. Therefore, if you want to enter a large factory and break away from traditional industries, these technical knowledge are necessary for you. Here's a copy by yourself Java Concurrent system mind map, hope to help you. **[Data acquisition method: free download here](https://gitee.com/vip204888/java-p7)** { document.write(str); }
4. Create utils under src js
exports.add = function (a, b) { return a + b; }
5. Create main under src js
const common = require('./common'); const utils = require('./utils'); common.info('Hello world!' + utils.add(100, 200));
4, JS packaging
last
In fact, it can be seen from the recruitment needs of large factories that high concurrent experience in recruitment requirements is preferred, including many friends who used to work in traditional industries or outsourcing projects. They have been working in small companies. The technology is relatively simple, and they have not done much distributed systems, but now Internet companies generally do distributed systems.
Therefore, if you want to enter a large factory and break away from the traditional industry, these technical knowledge are necessary for you. Now I have made a mind map of Java concurrency system, hoping to help you.
Data acquisition method: free download here