1. Distributed delivery objects, or network transmission, need to be serialized
2. I call the method of your jvm, and the result is returned to my jvm for processing
3. Serialization preserves the state of an object
For example, after tomcat is closed, the session object will be serialized to sessions In the ser file, load these sessions into memory the next time you start.
4. Data transmission and recovery
In j2ee, there are many pages and background. Especially when it is used in the list.
For example, if a person's list is saved, you can serialize the list, transfer it to the background, and then deserialize it into a person object to save the object directly.
5. For example, EJB remote call, distributed storage, cache storage, etc
6. Fields such as bank card and password cannot be serialized
4, Considerations for serialization and deserialization
===============
1. Java serialization
Implement the Serializable interface: you can customize the writeObject, readObject, writeReplace and readResolve methods, which will be called through reflection.
Implement the Externalizable interface: you need to implement the writeExternal and readExternal methods.
2. Serialization ID problem
Whether the virtual machine allows deserialization depends not only on whether the class path and function code are consistent, but also on whether the serialization IDs of the two classes are consistent (that is, private static final long serialVersionUID = 1L).
3. Static fields are not serialized
Static variables are not saved during serialization because serialization saves the state of the object and static variables belong to the state of the class. Therefore, serialization does not save static variables.
4,transient
transient represents the temporary data of the object.
If you don't want a member of an object to be serialized, you can modify it by adding the transient keyword when defining it, so that it won't be serialized when the object is serialized.
After deserialization, the member modified by transient will be given a default value, that is, 0 or null.
Sometimes fields such as bank card number do not want to be transmitted on the network. The function of transient is to save the life cycle of this field only in the caller's memory and will not be written to disk for persistence.
5. Serialization of parent class
When a parent class implements serialization, the child class automatically implements serialization; The subclass implements the Serializable interface, and the parent class also needs to implement the Serializable interface.
6. When the instance variable of an object references other objects, the reference object is also serialized when the object is serialized
7. Not all objects can be serialized
(1) For security reasons, for example, an object has private, public and other fields. For an object to be transmitted, such as writing to a file or RMI transmission, the private and other fields of the object are not protected during serialization and transmission;
(2) For resource allocation reasons, such as socket and thread classes, if they can be serialized, transmitted or saved, they cannot be reallocated, and it is not necessary to implement them in this way;
8. Serialization solves deep copy problems
If the member variable of an object is an object, the data members of the object will also be saved, which is an important reason why serialization can be used to solve deep copy.
5, Code example
======
1. Entity class
package javase.transientpackage; import java.io.Serializable; public class User implements Serializable { private static final long serialVersionUID = 1L; private String username; private transient String password; //construct,setter,getter }
2. ObjectOutputStream implements serialization and ObjectInputStream implements deserialization
package javase.transientpackage; import java.io.*; public class TransientTest { public static void main(String[] args) { try { SerializeUser(); DeSerializeUser(); } catch (IOException e) { e.printStackTrace(); }catch (ClassNotFoundException e) { e.printStackTrace(); } } //serialize private static void SerializeUser() throws IOException{ User user = new User(); user.setUsername("Su xiaonuan"); user.setPassword("123456"); ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("D://data.txt")); oos.writeObject(user); oos.close(); System.out.println("Normal field serialization: username= "+user.getUsername()); System.out.println("Added transient Keyword serialization: password= "+user.getPassword()); } //Deserialization private static void DeSerializeUser() throws IOException, ClassNotFoundException { File file = new File("D://data.txt"); ObjectInputStream ois = new ObjectInputStream(new FileInputStream(file)); User user = (User)ois.readObject(); # **Feeling:** In fact, when I submit my resume, I don't dare to send it to Ali. Because Ali has had three interviews in front of him, he didn't get his resume, so he thought he hung up. Special thanks to the interviewer on one side for fishing me, giving me the opportunity, and recognizing my efforts and attitude. Comparing my facial Sutra with those of other big men, I'm really lucky. Others 80% strength, I may 80% luck. So for me, I will continue to redouble my efforts to make up for my lack of technology and the gap with the leaders of Keban. I hope I can continue to maintain my enthusiasm for learning and continue to work hard. **I also wish you all can find your heart offer. ** The preparations I made before the interview (review materials for brushing questions and learning notes and learning routes of some big guys) have been sorted into electronic documents,**[Friends in need can [like]+Follow] Click here to get it for free](https://gitee.com/vip204888/java-p7)** Effort and attitude. Comparing my facial Sutra with those of other big men, I'm really lucky. Others 80% strength, I may 80% luck. So for me, I will continue to redouble my efforts to make up for my lack of technology and the gap with the leaders of Keban. I hope I can continue to maintain my enthusiasm for learning and continue to work hard. **I also wish you all can find your heart offer. ** The preparations I made before the interview (review materials for brushing questions and learning notes and learning routes of some big guys) have been sorted into electronic documents,**[Friends in need can [like]+Follow] Click here to get it for free](https://gitee.com/vip204888/java-p7)** 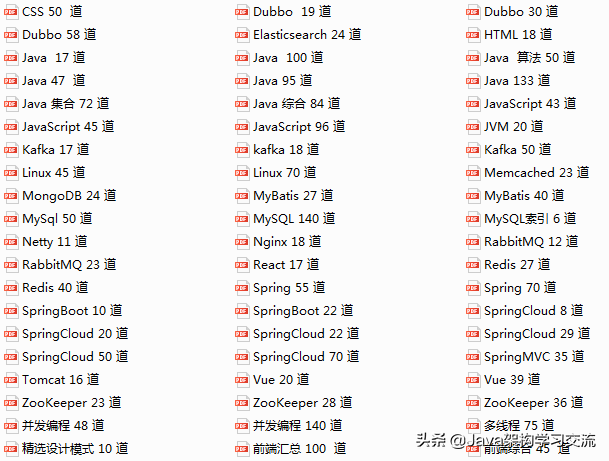