1. Single instance Bean declaration
**By default, Spring only creates a unique instance for each bean declared in the IOC container, which can be shared across the IOC container: all subsequent getBean() calls and bean references will return this unique bean instance. This scope is called singleton, * * it is the default scope for all beans. That is, single instance.
To verify this statement, we create a single instance bean in the IOC and obtain the bean object for comparison:
<!-- singleton Single instance bean 1,Created when the container is created 2,There is only one instance --> <bean id="book02" class="com.spring.beans.Book" scope="singleton"></bean>
Test whether the obtained single instance bean is the same:
@Test public void test09() { // When a single instance is created, the two bean s created are equal Book book03 = (Book)iocContext3.getBean("book02"); Book book04 = (Book)iocContext3.getBean("book02"); System.out.println(book03==book04); }
The result is true;
2. Multi instance Bean declaration
Since there is a single instance, there must be multiple instances. We can set the prototype parameter for the scope attribute of the bean object to indicate that the instance is multi instance. At the same time, we can obtain the multi instance beans in the IOC container, and then compare the obtained multi instance beans,
<!-- prototype Multiple instances bean 1,It will not be created when the container is created, 2,It is created only when called 3,Multiple instances can exist --> <bean id="book01" class="com.spring.beans.Book" scope="prototype"></bean>
Test whether the obtained multi instance bean is the same:
@Test public void test09() { // When creating multiple instances, the two bean objects created are not equal Book book01 = (Book)iocContext3.getBean("book01"); Book book02 = (Book)iocContext3.getBean("book01"); System.out.println(book01==book02); }
The result is false
This shows that bean objects created through multiple instances are different.
Note here:
At the same time, the creation of single instance and multi instance beans is also different. When the scope of the bean is a single instance, Spring will create the object instance of the bean when the IOC container object is created. When the scope of the bean is prototype, the IOC container creates the instance object of the bean when obtaining the instance of the bean.
2, Bean life cycle
===============
1. bean initialization and destruction
In fact, each bean object we create in IOC has its specific life cycle. The bean life cycle can be managed in the IOC container of spring. Spring allows the specified tasks to be executed at a specific time point in the bean life cycle. Such as the method executed when the bean is initialized and the method executed when the bean is destroyed.
The process of managing the bean's life cycle by the Spring IOC container can be divided into six steps:
-
Create a bean instance through a constructor or factory method
-
Set values for bean properties and references to other beans
-
Call the initialization method of the bean
-
Beans can be used normally
-
When the container is closed, the bean's destruction method is called
So how to declare the methods executed at the beginning and destruction of the bean?
First, we should add methods that execute on initialization and destruction inside the bean class. As shown in the following Java Bean:
package com.spring.beans; public class Book { private String bookName; private String author; /** * Initialization method * */ public void myInit() { System.out.println("book bean Created"); } /** * Method of destruction * */ public void myDestory() { System.out.println("book bean Destroyed"); } public String getBookName() { return bookName; } public void setBookName(String bookName) { this.bookName = bookName; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } @Override public String toString() { return "Book [bookName=" + bookName + ", author=" + author + "]"; } }
At this time, when configuring beans, we can specify initialization and destruction methods for beans through init method and destroy method attributes,
<!-- set up bean Life cycle of destory-method: End called method init-method: Method called at start --> <bean id="book01" class="com.spring.beans.Book" destroy-method="myDestory" init-method="myInit"></bean>
In this way, when we create and destroy bean objects through the IOC container, we will execute the corresponding methods,
However, there is one thing to note here:
As mentioned above, the creation time of single instance beans and multi instance beans is different, so the execution time of their initial method and destruction method is slightly different.
Life cycle of bean under single instance
Container startup - > initialization method - > destruction method (container closing)
Life cycle of bean in multi instance
Container start - > call bean - > initialize method - > container close (the destroy method is not executed)
2. Post processor of bean
What is the post processor of a bean? The bean postprocessor allows additional processing of the bean before and after calling the initialization method
The bean post processor processes all bean instances in the IOC container one by one, rather than a single instance.
Its typical application is to check the correctness of bean properties or change bean properties according to specific standards.
When the bean post processor is used, it needs to implement the interface:
org.springframework.beans.factory.config.BeanPostProcessor.
Before and after the initialization method is called, Spring will pass each bean instance to the following two methods of the above interface:
Before postProcessBeforeInitialization(Object, String) call
After postProcessAfterInitialization(Object, String) call
The following is a post processor implemented in the interface:
package com.spring.beans; import org.springframework.beans.BeansException; import org.springframework.beans.factory.config.BeanPostProcessor; # last Gold, silver and four will arrive soon. I hope you can learn these technical points well. Please welcome friends who need to receive these learning materials and interview notes**[Click here for free!](https://gitee.com/vip204888/java-p7)** Learning video: 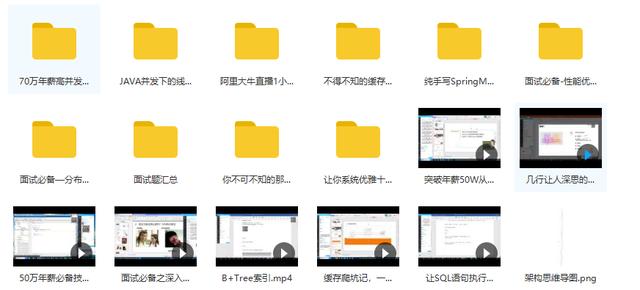 Real interview questions for large factories: The following is a post processor implemented in the interface:
package com.spring.beans;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.BeanPostProcessor;
last
Gold, silver and four will arrive soon. I hope you can learn these technical points well. Please welcome friends who need to receive these learning materials and interview notes** Click here for free!**
Learning video:
[external chain picture transferring... (img-9ju3KUu2-1628296202262)]
Real interview questions for large factories: