Characteristics of collection class: the type and size can be uncertain
Usage scenario: the stored data should change frequently
===================================================================
Collections are mainly divided into two categories: Collection and Map:
Collection: It is the top-level parent class of a single column set and a sequence of independent elements. These elements obey one or more rules. List The elements must be saved in the order of insertion, and Set Cannot have duplicate elements. Namely Collection Divided into List and Set Two branches.
[key points]
Collection: unordered, repeatable
List Series Collection: ordered, repeatable and indexed.
– ArrayList: the added elements are ordered, repeatable and indexed
– LinekdList: the added elements are ordered, repeatable and indexed
Set series collection: the added elements are unordered, non repeatable, and non indexed
– HashSet: the added elements are unordered, non repeatable, and non indexed
– LinkedHashSet: the added elements are ordered, non repeatable, and non indexed
– TreeSet: default ascending / descending order according to size, non repeatable, no index
Map: storage method key:value key value pair
============================================================================
What is a confliction set?
The confliction collection is the ancestor class of all collections. Its function is that all collections can inherit and use
Common methods of confliction set
|Method name | description|
| — | — |
|Public Boolean add (E) | add an element at the end of the collection|
|public void clear clears all elements in the collection|
|Public Boolean remove (E) deletes the specified element and returns whether the deletion was successful|
|public boolean isEmpty() | judge whether the current collection is empty|
|public boolean contains(Object obj) | judge whether the current collection contains the specified object|
|public Object[] toArray() | store the elements in the collection into the array|
|public int size() | returns the number of elements in the collection|
=====================================================================
ArrayList implements variable length arrays and allocates continuous space in memory. Traversal elements and random access elements are more efficient. The underlying data structure is an array, and the thread is not safe. (it is a collection we use very, very much)
Implementation method:
ArrayList list = new ArrayList();//Create an ArrayList collection, and initialize the underlying Object[] elementData to {} list.add(new Person("Zhang San",1));//When you call add() for the first time, you create an array with a length of 10 at the bottom, and add data sheet 3 to elementDate
Define storage type
ArrayList<Person> list = new ArrayList<>(); //object type list.add("Zhang San",1); //Jiaqun 1025684353 blowing water and chatting together ArrayList<String> list = new ArrayList<>(); //String type list.add("Zhang San"); ArrayList<Integer> list = new ArrayList<>(); //Packing type Boolean Byte Character Short Integer Long Float Double list.add(1);
Common methods of List collection:
|Method name | description|
| — | — |
|Public Boolean add (E) | add an element at the end of the collection|
|public boolean add(int index, E element) | add an element at the specified location|
|public boolean remove(Object o) | deletes the specified element and returns whether the deletion was successful|
|public E remove(int index) | deletes the element at the specified index and returns the deleted element|
|public E set(int index,E element) | modify the element at the specified index and return the modified element|
|public E get(int index) | returns the element at the specified index|
|public int size() | returns the number of elements in the collection|
|indexOf(Object o) | query the position of the specified element. lastIndexOf is the same, just traversing from the tail|
Use of common methods of List collection:
ArrayList<String> list = new ArrayList<>(); list.add("Zhang San"); //Add an element list.add(5,"Zhang San"); //Adds an element at the specified index list.remove("Zhang San"); //Delete an element System.out.println(list.remove("Zhang San")); //Delete an element and output true|false list.remove(1); //Deletes the element at the specified index list.set(0,"Li Si"); //Modify the element of the specified index and return the modified element //Jiaqun 1025684353 blowing water and chatting together list.get(0); //Gets the element of the specified index list.size(); //Gets the number of elements in the collection list.lidexOf("Li Si"); //Gets the location of the specified element
List traversal method:
// The first traversal method: ordinary for loop for (int i = 0; i < arrayList.size(); i++) { System.out.println(arrayList.get(i)); } // The second traversal method: enhance the for loop for (String string : arrayList) { System.out.println(string); } //Jiaqun 1025684353 blowing water and chatting together // The third traversal method: iterator Iterator<String> iterator = arrayList.iterator(); while (iterator.hasNext()) { System.out.println(iterator.next()); }
====================================================================
Map set architecture
What is a Map collection?
A Map set is a two column set, where each element contains two values.
Format of each element of the Map set: key = value (key value pair element).
Characteristics of Map set
1.Map sets are determined by keys.
2. The keys of the map set are unordered, non repetitive and non indexed.
– the element corresponding to the repeated key behind the Map set will overwrite the whole previous element!
3. The value of the map set is not required.
4. Key value pairs of map collection can be null.
Differences between HashMap and LinkedHashMap
HashMap: elements are unordered, non repetitive and non indexed according to the key, and the value is not required.
LinkedHashMap: elements are ordered by key, without repetition, without index, and without value requirements.
The Map collection uses:
1.Map The information stored in the collection is more specific and rich.
//Conllection: {"Zhang San", "male", 23, "China", "Shenzhen", "programmer"}
//Map:{name = "Zhang San", sex = "male", age=23,country = "China", city = "Shenzhen", occupation = "programmer"}
Map<Person,String> maps = new HashMap<>
maps.put(new Person("Zhang San", "male", "23"), "China");
2.Map Collections are suitable for storing object data ``` ### []( https://gitee.com/vip204888/java-p7 )**Common methods of map collection:** | Method name | explain | | --- | --- | | public v put(K key, V value) | Adds the specified key and the specified value to the Map In collection | | public v remove(Object key) | Deletes the element corresponding to the specified value | | public boolean isEmpty() | Determine whether the collection is empty | | abstract int size() | Get collection size | | public v get(Object key) | Gets the specified element according to the key | | public set keySet() | obtain Map All keys in the collection, stored in Set In collection | | public Conllection values() | obtain Map All values in the collection, stored in Conllection In collection | | public set<Map.Entry<K,V>> entrySet() | Get Map A collection of all key value pair objects in the collection(Set aggregate) | | public boolean containKey(Object key) | Determine whether the collection contains a key | | public boolean containValue(Object value) | Determine whether the collection contains a value | ### []( https://gitee.com/vip204888/java-p7 )**Map usage * *: ``` Map<String,Integer> maps = new HashMap<>(); maps.put("Zhang San",1); //Add element maps.put(null,null); maps.clear(); //Empty collection maps.isEmpty(); //Judge whether the collection is empty, return true if it is empty, and return false if it is not empty maps.get("Zhang San"); //Obtain the corresponding value according to the key Return 1 maps.size(); //Get collection size maps.remove("Zhang San"); //Delete an element according to the key Return 1 maps.containsKey("Zhang San"); //Judge whether the collection contains Zhang San and return true or false //Jiaqun 1025684353 blowing water and chatting together maps.containsValue(1); //Judge whether the collection contains key 1 and return true or false //The keys of the map Set are unordered and not repeated, so a Set set is returned Set<String> keys = maps.keySet();//Get all the keys in the Map collection and store them in the Set collection //The value of the Map set cannot meet the requirements and may be repeated, so it should be received with the confliction set! Conllection<Integer> values = maps.values();//Store the values in the maps set into the collision set ``` ### []( https://gitee.com/vip204888/java-p7 )**Traversal of map collection * *: map Traversal method in set 3: 1."Traversal by "key finding value": get first Map Set all the keys, and then find the value according to the traversal key. 2."Traversal mode of "key value pair": difficult. # last Dear readers, since the scope of this article is too long, in order to avoid affecting the reading experience, I will roughly summarize and sort it out below. If necessary, please**[Click here to download the article information for free after you like it!](https://gitee.com/vip204888/java-p7)** 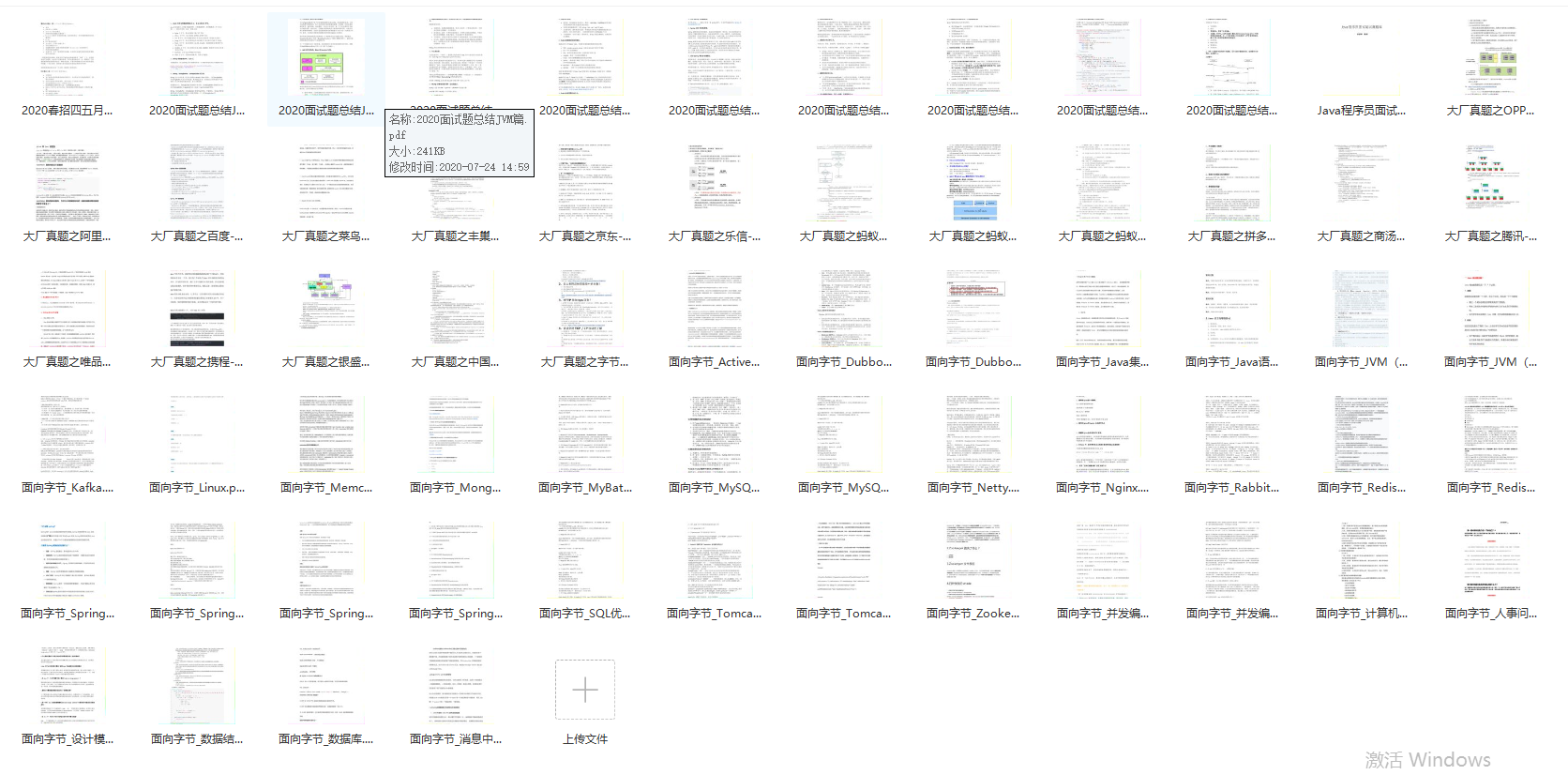 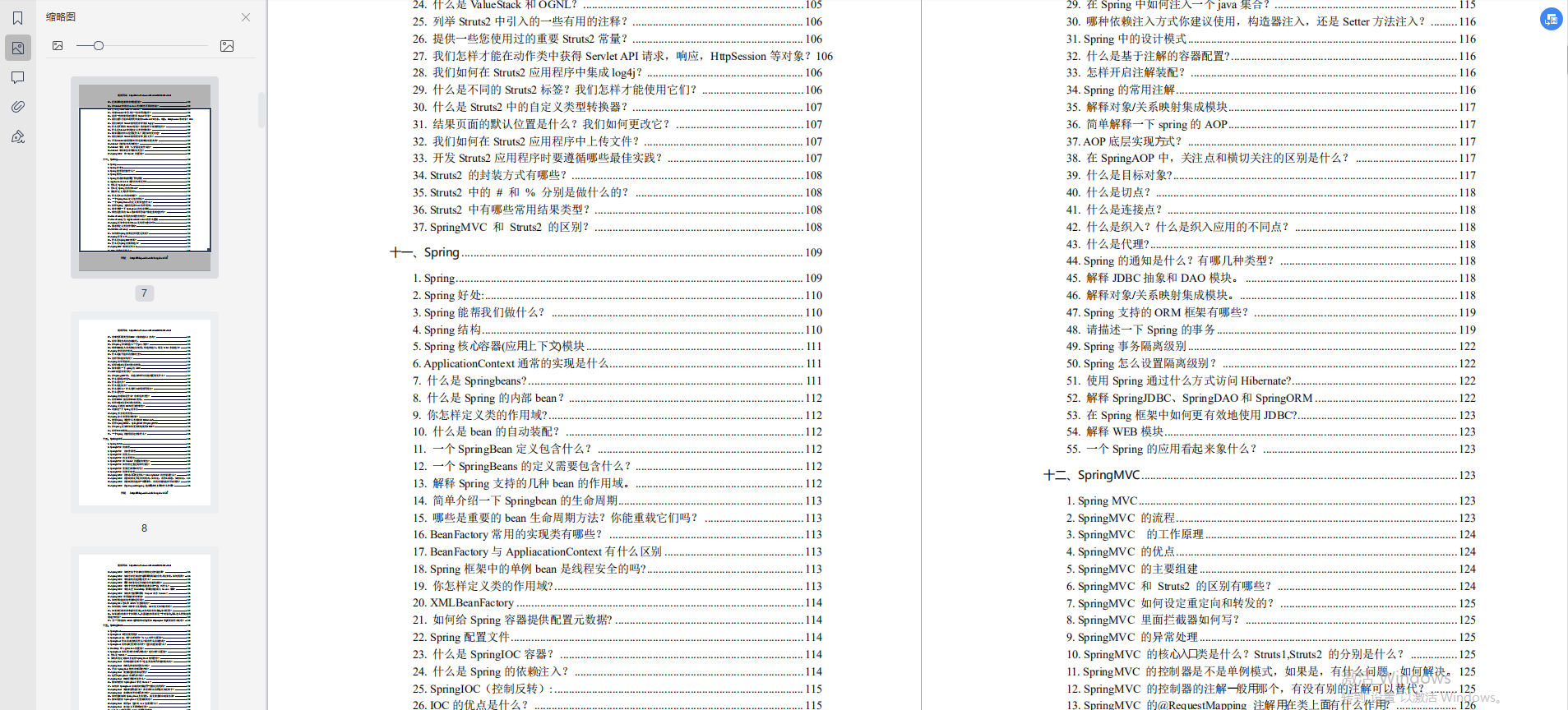 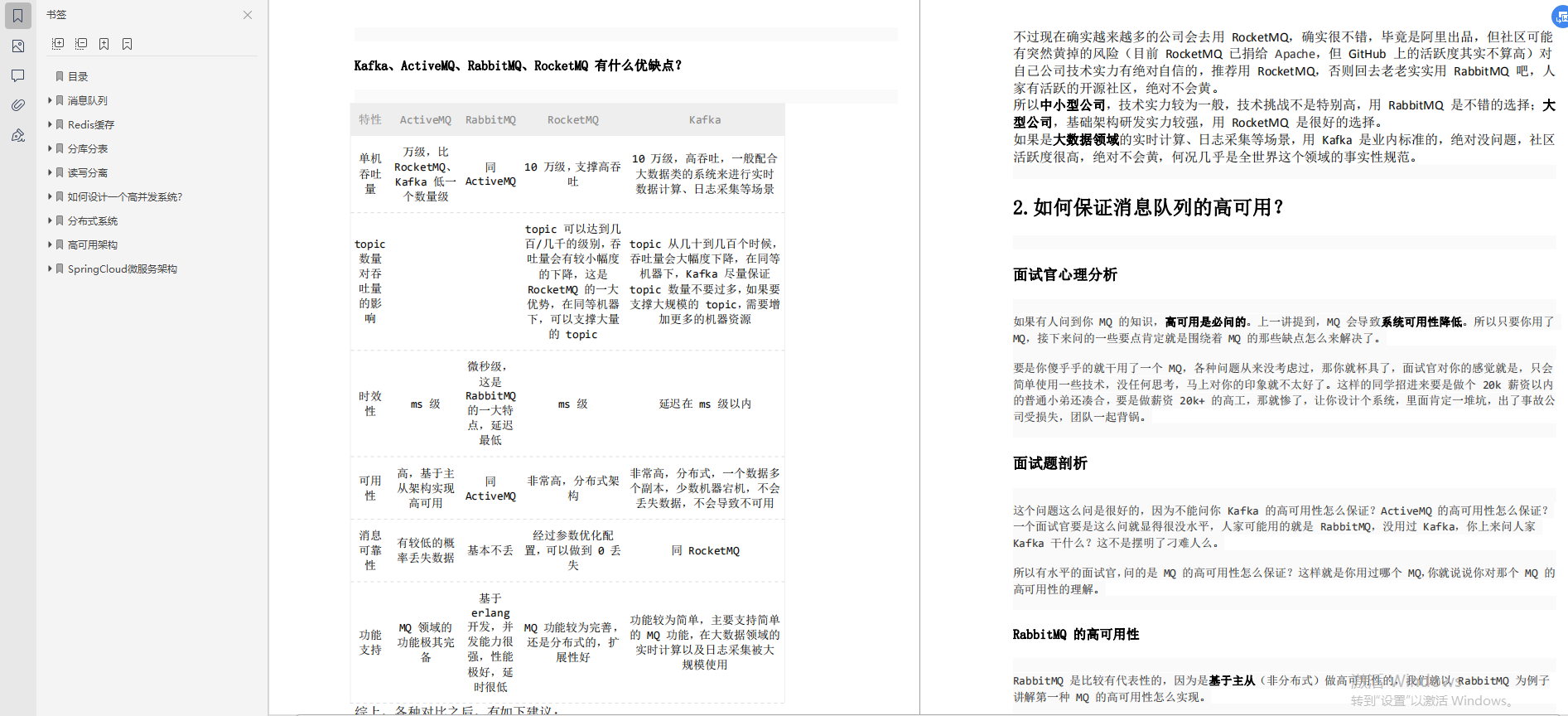 maps The values in the collection are stored in the Conllection In collection ``` ### []( https://gitee.com/vip204888/java-p7 )**Traversal of map collection * *: map Traversal method in set 3: 1."Traversal by "key finding value": get first Map Set all the keys, and then find the value according to the traversal key. 2."Traversal mode of "key value pair": difficult. # last Dear readers, since the scope of this article is too long, in order to avoid affecting the reading experience, I will roughly summarize and sort it out below. If necessary, please**[Click here to download the article information for free after you like it!](https://gitee.com/vip204888/java-p7)** [External chain picture transfer...(img-GrnKfaSw-1628143401140)] [External chain picture transfer...(img-lRu2FibB-1628143401142)] [External chain picture transfer...(img-DNP1rw33-1628143401143)] 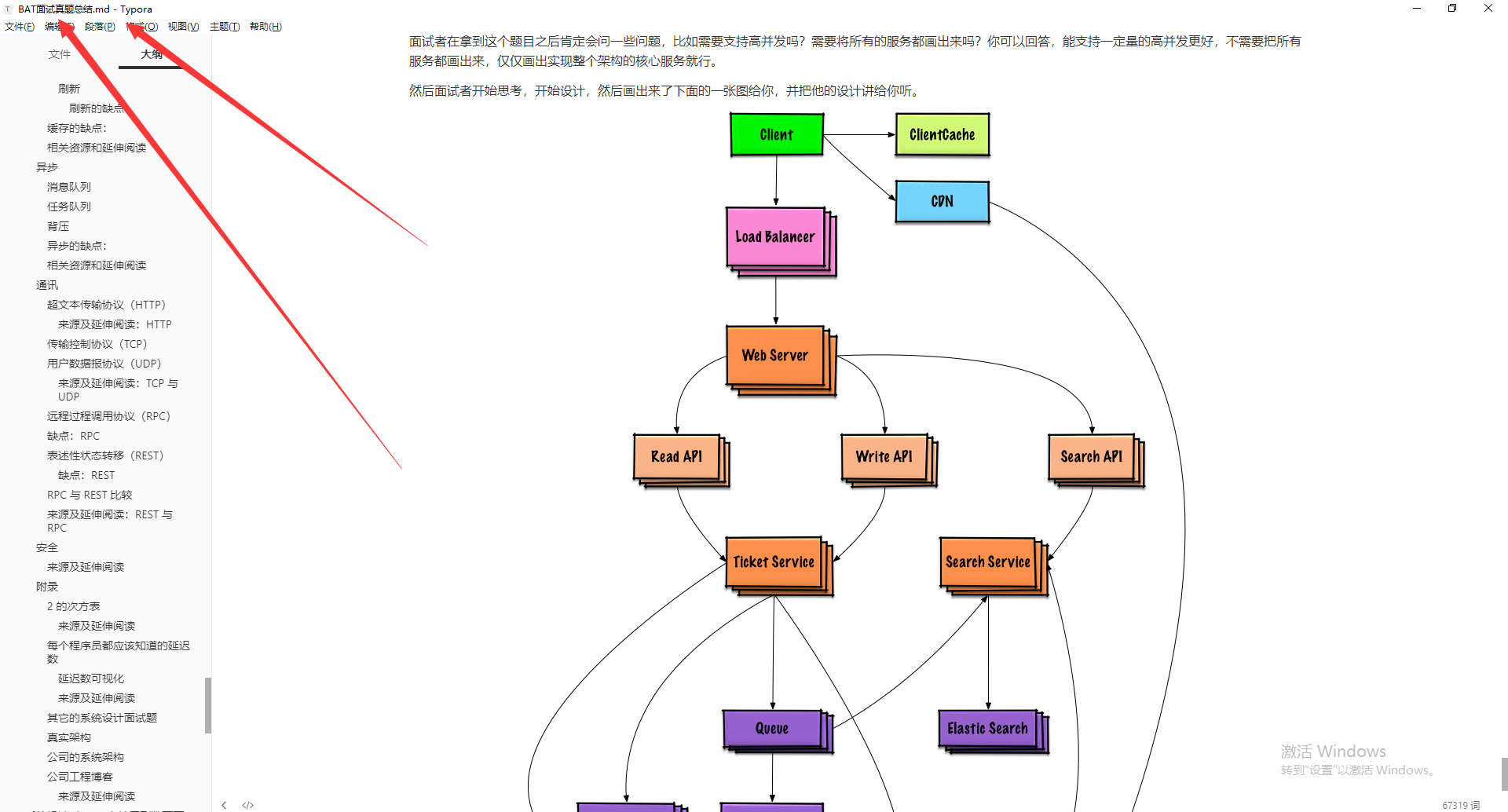