3.1 template syntax overview
3.1.1 how to understand front-end rendering?
Fill data into HTML tags
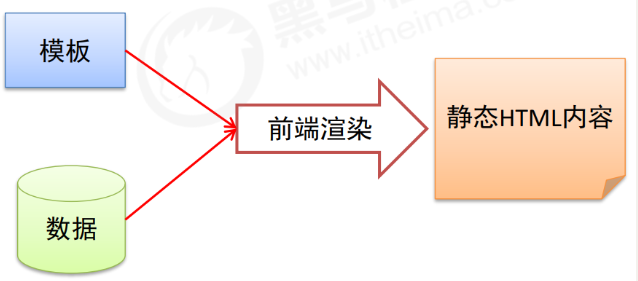
3.1.2 front end rendering mode
- Native js splice string
- Using the front-end template engine
- Use vue specific template syntax
3.1.3 native js splice string
Basically, the data is spliced into HTML tags in the form of strings. The front-end code style is generally shown in the figure.
var d = data.weather; var info = document.getElementById('info'); info.innerHTML = ''; for(var i=0;i<d.length;i++){ var date = d[i].date; var day = d[i].info.day; var night = d[i].info.night; var tag = ''; tag += '<span>Date:'+date+'</sapn><ul>'; tag += '<li>Daytime weather:'+day[1]+'</li>' tag += '<li>Daytime temperature:'+day[2]+'</li>' tag += '<li>Daytime wind direction:'+day[3]+'</li>' tag += '<li>Daytime wind speed:'+day[4]+'</li>' tag += '</ul>'; var div = document.createElement('div'); div.innerHTML = tag; info.appendChild(div); }
Disadvantages: the code styles of different developers vary greatly. With the complexity of the business, the later maintenance becomes more and more difficult.
3.1.4 using front-end template engine
The code on the right is a piece of code based on the template engine art template. Compared with splicing strings, the code is obviously much more standardized. It has its own set of template syntax rules.
<script id="abc" type="text/html"> {{if isAdmin}} <h1>{{title}}</h1> <ul> {{each list as value i}} <li>Indexes {{i + 1}} : {{value}}</li> {{/each}} </ul> {{/if}} </script>
Advantages: everyone follows the same rules to write code, which significantly improves the readability of the code and facilitates later maintenance.
Disadvantages: no special event mechanism is provided.
3.1.5 template syntax overview
- Difference expression
- instructions
- Event binding
- Property binding
- Style binding
- Branch loop structure
3.2 instructions
3.2.1 what is an instruction?
- What are custom attributes
- The essence of an instruction is a custom attribute
- Instruction format: start with V - (for example: v-cloak)
3.2.2 k instruction usage
v-cloak
- Problem with interpolation expression: "flashing"
- How to solve this problem: use the v-cloak instruction
- The principle to solve this problem is to hide and replace the value before displaying the final value
- Prevent flicker problems when loading pages < style type = "text / CSS" > / * 1. Select the tag with attribute v-cloak through the attribute selector and let it hide * / [v-cloak] {/ * element hide * / display: none;}</ style> <body> <div id="app"> <!-- 2. Let the v-cloak attribute added with interpolation syntax be automatically removed after data rendering, Once v-cloak is removed, that is, there is no attribute. The attribute selector cannot select the tag, that is, the corresponding tag will become visible -- > < div v-cloak > {{msg}} < / div > < / div > < script type = "text / JavaScript" SRC = "JS / Vue. JS" > < / script > < script type = "text / JavaScript" > var VM = new Vue( {/ / El specifies that the element ID is the element of APP El: '#app', / / data is stored in data (data: {MSG: 'hello Vue'}})</ script> </body> </html>
v-text
- The v-text instruction is used to fill the label with data. It is similar to the interpolation expression, but there is no flicker problem
- If there are HTML tags in the data, the HTML tags will be output together
- Note: This is one-way binding. When the value on the data object changes, the interpolation will change; However, when the interpolation changes, it will not affect the value of the data object
<div id="app"> <!-- be careful:Do not write interpolation syntax in the instruction. Write the corresponding variable name directly stay v-text Do not write interpolation syntax when assigning values in General attributes are not added {{}} Write the corresponding data name directly --> <p v-text="msg"></p> <p> <!-- Vue Interpolation syntax is used only in the content of the label --> {{msg}} </p> </div> <script> new Vue({ el: '#app', data: { msg: 'Hello Vue.js' } }); </script>
v-html
- The usage is similar to v-text, but it can fill HTML fragments into tags
- There may be security problems. Generally, v-html is only used on trusted content and never on content submitted by users
- The difference between v-text and v-text is that v-text outputs plain text, which will not be parsed by the browser, but v-html will output it when the HTML tag is parsed. <div id="app"> <p v-html="html"></p> <!-- Output: HTML tags are parsed during rendering -- > < p > {message}} < / P > <-- Output: < span > bind through double brackets < / span > -- > < p v-text = "text" > < / P > <-- Output: < span > HTML tag is output by the source code during rendering < / span > -- > < / div > < script > let app = new Vue({el: "#app", data: {message: "< span > bind < / span >" through double brackets, HTML: "< span > HTML tag is parsed during rendering < / span >", text: "< span > HTML tags are output by the source code when rendering < / span >",}})</ script>
v-pre
- Show raw information skip compilation
- Skip the compilation of this element and its child elements.
- Some static content does not need to be compiled. Adding this instruction can speed up rendering
<span v-pre>{{ this will not be compiled }}</span> <!-- It shows{{ this will not be compiled }} --> <span v-pre>{{msg}}</span> <!-- even if data It defines msg It is still shown here{{msg}} --> <script> new Vue({ el: '#app', data: { msg: 'Hello Vue.js' } }); </script>
v-once
- Perform one-time interpolation [when the data changes, the content at the interpolation will not continue to be updated]
<!-- even if data It defines msg Later we modified it and still show it for the first time data The data stored inside is Hello Vue.js --> <span v-once>{{ msg}}</span> <script> new Vue({ el: '#app', data: { msg: 'Hello Vue.js' } }); </script>
3.2.3 data binding instructions
- v-text fill in plain text ① It is more concise than interpolation expression
- v-html fill HTML fragment ① There are security problems ② The internal data of this website can be used, but the data from third parties cannot be used
- v-pre fills in the original information ① Display the original information and skip the compilation process (analyze the compilation process)
3.2.4 data response
- How to understand responsive ① Responsiveness in html5 (the change of screen size leads to the change of style) ② Data response (changes in data lead to changes in page content)
- What is data binding ① Data binding: populating data into labels
- v-once is compiled only once ① No longer responsive after displaying content
3.3 bidirectional data binding instruction
3.3.1 what is bidirectional data binding?

3.3.2 bidirectional data binding analysis
- v-model instruction usage
3.3.3 MVVM design idea
M. What do V and VM stand for?
m model
The data layer in Vue is placed in data
v view view
Vue view is our HTML page
The vm (view model) controller connects the data to the view layer
vm is an instance of Vue
3.4 event binding
v-bind
- The v-bind instruction is used to update HTML attributes in response
- v-bind:href can be abbreviated as: href;
<!-- Bind a property --> <img v-bind:src="imageSrc"> <!-- abbreviation --> <img :src="imageSrc">
Binding object
- We can give v-bind:class an object to dynamically switch classes.
- Note: the v-bind:class instruction can coexist with the normal class feature
1, v-bind Binding an object is supported in If an object is bound, the key is the corresponding class name and the value is the corresponding class name data Data in <!-- HTML Final render as <ul class="box textColor textSize"></ul> be careful: textColor,textSize The corresponding rendering to the page CSS Class name isColor,isSize corresponding vue data If the data in is true The corresponding class name is rendered to the page When isColor and isSize When it changes, class The list will be updated accordingly, For example, the isSize Change to false, class The list becomes <ul class="box textColor"></ul> --> <ul class="box" v-bind:class="{textColor:isColor, textSize:isSize}"> <li>study Vue</li> <li>study Node</li> <li>study React</li> </ul> <div v-bind:style="{color:activeColor,fontSize:activeSize}">Object syntax</div> <sript> var vm= new Vue({ el:'.box', data:{ isColor:true, isSize:true, activeColor:"red", activeSize:"25px", } }) </sript> <style> .box{ border:1px dashed #f0f; } .textColor{ color:#f00; background-color:#eef; } .textSize{ font-size:30px; font-weight:bold; } </style>
Bind class
2, v-bind Binding an array is supported in In array classA and classB Corresponding to data Data in there classA Right use data Medium classA there classB Right use data Medium classB <ul class="box" :class="[classA, classB]"> <li>study Vue</li> <li>study Node</li> <li>study React</li> </ul> <script> var vm= new Vue({ el:'.box', data:{ classA:'textColor', classB:'textSize' } }) </script> <style> .box{ border:1px dashed #f0f; } .textColor{ color:#f00; background-color:#eef; } .textSize{ font-size:30px; font-weight:bold; } </style>
The difference between bound objects and bound arrays
- When binding an object, the attribute of the object is the class name to be rendered, and the attribute value of the object corresponds to the data in data
- When binding an array, the data in data is stored in the array
Binding style
<div v-bind:style="styleObject">Bind style object</div>' <!-- CSS Attribute names can be humped (camelCase) Or dash separation (kebab-case,Remember to enclose it in single quotation marks) --> <div v-bind:style="{ color: activeColor, fontSize: fontSize,background:'red' }">inline style </div> <!--Group syntax can apply multiple style objects to the same element --> <div v-bind:style="[styleObj1, styleObj2]"></div> <script> new Vue({ el: '#app', data: { styleObject: { color: 'green', fontSize: '30px', background:'red' }, activeColor: 'green', fontSize: "30px" }, styleObj1: { color: 'red' }, styleObj2: { fontSize: '30px' } </script>
3.4.1 how does Vue handle events?
- v-on instruction usage <input type='button' v-on:click='num++'/>
- v-on abbreviation
<input type='button' @click='num++'/>
3.4.2 calling method of event function
- Direct binding function name <button v-on:click='say'>Hello</button>
- Call function
<button v-on:click='say()'>Say hi</button>
3.4.3 event function parameter transfer
- Common parameters and event objects <button v-on:click='say("hi",$event)'>Say hi</button>
3.4. 4 event modifiers
- . stop stop bubbling <a v-on:click. Stop = "handle" > jump</a>
- . prevent block default behavior
<a v-on:click.prevent="handle">Jump</a>
3.4.5 key modifier
- . enter <input v-on:keyup.enter='submit'>
- . esc exit key
<input v-on:keyup.delete='handle'>
3.4.6. Custom key modifier
- Global config Keycodes object Vue.config.keyCodes.f1 = 112
Branching structure
v-if usage scenario
- 1 - multiple elements show or hide an element through conditional judgment. Or multiple elements
- 2 - switch between two views
<div id="app"> <!-- Judge whether to load. If it is true, it will be loaded; otherwise, it will not be loaded--> <span v-if="flag"> If flag by true Then display,false Do not display! </span> </div> <script> var vm = new Vue({ el:"#app", data:{ flag:true } }) </script> ---------------------------------------------------------- <div v-if="type === 'A'"> A </div> <!-- v-else-if Follow closely v-if or v-else-if After that v-if Execute when conditions are not satisfied--> <div v-else-if="type === 'B'"> B </div> <div v-else-if="type === 'C'"> C </div> <!-- v-else Follow closely v-if or v-else-if after--> <div v-else> Not A/B/C </div> <script> new Vue({ el: '#app', data: { type: 'C' } }) </script>
The difference between v-show and v-if
- The essence of v-show is to set the label display to none to control hiding
- V-show is compiled only once. In fact, it controls the css, and v-if is constantly destroyed and created. Therefore, the performance of v-show is better.
- v-if dynamically adds or deletes DOM elements to the DOM tree
- v-if switching has a process of local compilation / unloading. During the switching process, the internal event listener and sub components are properly destroyed and rebuilt
Cyclic structure
v-for
- The values in the array used for the loop can be objects or ordinary elements
<ul id="example-1"> <!-- Cyclic structure-Traversal array item It is a name defined by ourselves, which represents each item in the array items Corresponding to data Array in--> <li v-for="item in items"> {{ item.message }} </li> </ul> <script> new Vue({ el: '#example-1', data: { items: [ { message: 'Foo' }, { message: 'Bar' } ], } }) </script>
- Using both v-if and v-for is not recommended
- When v-if is used with v-for, v-for has a higher priority than v-if.
<!-- Cyclic structure-Traversal object v Representing objects value k Key representing the object i Representative index ---> <div v-if='v==13' v-for='(v,k,i) in obj'>{{v + '---' + k + '---' + i}}</div> <script> new Vue({ el: '#example-1', data: { items: [ { message: 'Foo' }, { message: 'Bar' } ], obj: { uname: 'zhangsan', age: 13, gender: 'female' } } }) </script>
- Role of key
- key to make a unique identification for each node
- key is mainly used to update the virtual DOM efficiently
<ul> <li v-for="item in items" :key="item.id">...</li> </ul>