catalogue
Unidirectional linked list experiment
Creation of bidirectional linked list
Bidirectional linked list experiment
order
On the train of youth, if you want to get off early, please don't wake me up
Hi, this is fox
Today is the weekend. I didn't sleep all night yesterday. I don't know why I lost sleep. Just get up and thank you for your blog. Recently, the anxiety of middle-aged people has slowly spread to my heart and my whole body is uncomfortable. Maybe I can get a little peace by sitting in front of the computer screen. Today, let's share the knowledge of data structure - single linked list and two-way linked list.
Single linked list
Single linked list is a data structure with chain access. A group of storage units with arbitrary address are used to store the data elements in the linear list. The data in the linked list is represented by nodes. The composition of each node is: element (image of data element) + pointer (indicating the storage location of subsequent elements). The element is the storage unit for storing data, and the pointer is the address data connecting each node.
Node of one-way linked list
As shown in the following code, the node of the two-way linked list contains two pointing relationships and a data space. The two points to the previous and next nodes connecting the node respectively. The data type can be a structure type or other types.
typedef struct node { int data; struct node *pre; struct node *next; }Node;
Unidirectional linked list experiment
Experiment content:
1. Randomly generate or input a group of elements (no less than 10 elements) on the keyboard to establish a single linked list of leading nodes.
2. Invert the elements in the single linked list (it is not allowed to apply for new node space).
3. Delete all even element nodes in the single linked list.
4. Write a function to insert an element into the non decreasing ordered linked list so that the elements of the linked list are still ordered, and use this function to establish a
Non decreasing ordered single linked list.
5. Use algorithm 4 to establish two non decreasing ordered single linked lists, and then merge them into a non increasing linked list.
6. Decompose the linked list established by algorithm 1 into two linked lists, one of which is all odd and the other is all even (as far as possible)
Use existing storage space).
7. Design a simple menu in the main function and call the above algorithm.
Experiment Description:
1. Node type definition
typedef int ElemType; // Element type typedef struct LNode { ElemType data ; struct LNode * next ; } LNode, *pLinkList ;
2. For simplicity, the single linked list of leading nodes is adopted.
Source code
#include <iostream> #include <time.h> #include <iomanip> #include <stdlib.h> using namespace std; typedef int ElemType; typedef struct LNode { ElemType data; struct LNode* next; }LNode, *pLinkList; pLinkList LinkList_Init() //Single linked list creation { int n; LNode *phead, *temp, *p; phead = new LNode; if (phead == NULL) { cout << "Failed to create linked list!" << endl; exit(0); } cout << "Enter the number of elements to be generated randomly(No less than 10): "; cin >> n; temp = phead; srand(unsigned(time(NULL))); for (int i = 0; i < n; i++) { p = new LNode; p->data = rand() % 100; temp->next = p; temp = p; } temp->next = NULL; return phead; } void LinkList_Display(pLinkList phead) //Output linked list { phead = phead->next; while (phead) { cout << setw(4) << phead->data; phead = phead->next; } cout << endl; } void LinkList_Free(pLinkList phead) //Linked list release { LNode *p; while (phead) { p = phead; phead = phead->next; delete p; } } void LinkList_Convert(pLinkList phead) //Element inversion in single linked list { LNode *p1, *p2, *p3; //p3 is the first node, p2 is the second node and p1 is the third node if (phead->next == NULL) { cout << "The linked list is empty!" << endl; return; } p3 = phead->next; p2 = p3->next; if (p2 == NULL) { return; } p1 = p2->next; p3->next = NULL; while (p1) { p2->next = p3; p3 = p2; p2 = p1; p1 = p1->next; } p2->next = p3; phead->next = p2; } void LinkLIst_Del_oushu(pLinkList phead) //Delete all even element nodes in the single linked list { LNode *p1, *p2; p2 = phead; phead = phead->next; while (phead) { if (phead->data % 2 == 0) { p1 = phead; phead = phead->next; delete p1; p2->next = phead; continue; } p2 = phead; phead = phead->next; } } void LinkList_Sort(pLinkList phead) //sort { ElemType *num, temp; LNode *p; int count = 0, i = 0; if (phead->next == NULL) { cout << "The linked list is empty!" << endl; return; } p = phead->next; while (p) { count++; p = p->next; } num = new ElemType[count]; p = phead->next; while (p) { num[i++] = p->data; p = p->next; } for (int j = 0; j < count - 1; j++) for (int k = 0; k < count - j - 1; k++) if (num[k] > num[k + 1]) { temp = num[k]; num[k] = num[k + 1]; num[k + 1] = temp; } p = phead->next; i = 0; while (p) { p->data = num[i++]; p = p->next; } delete[] num; } void LinkList_Insert(pLinkList phead, ElemType elem) //Insert an element { LNode *p1, *p2, *tmp = NULL; p1 = phead->next; p2 = phead; while (p1) { if (elem < p1->data) break; p2 = p1; p1 = p1->next; } tmp = new LNode; tmp->data = elem; p2->next = tmp; tmp->next = p1; } void LinkList_Divide(pLinkList L1, pLinkList L2) //The linked list is decomposed into odd and even linked lists { LNode *p1, *p2; p2 = L1; p1 = L1->next; while (p1) { if ((p1->data) % 2 == 0) { L2->next = p1; L2 = p1; p1 = p1->next; p2->next = p1; } else { p2 = p1; p1 = p1->next; } } L2->next = NULL; } void LinkList_Cat(pLinkList L1, pLinkList L2) //List merging, { pLinkList p1, p2; p2 = L1; p1 = L1->next; while (p1) { p2 = p1; p1 = p1->next; } p2->next = L2->next; LinkList_Sort(L1); LinkList_Convert(L1); } int main() { LNode *L = NULL, *L1 = NULL, *L2 = NULL; int num; cout << "1:Random generation or keyboard input of a group of elements (no less than 10 elements),Establish a single linked list of leading nodes" << endl; cout << "2:Element inversion in single linked list" << endl; cout << "3:Single linked list sort output" << endl; cout << "4:Delete all even element nodes in the single linked list" << endl; cout << "5:Insert an element and use this function to establish a non decreasing ordered single linked list (insert)" << endl; cout << "6:Create two non decreasing ordered single linked lists, and then merge them into a non increasing linked list (merge)" << endl; cout << "7:The linked list is decomposed into two linked lists, one of which is all odd and the other is all even" << endl; cout << "8:sign out" << endl; cout << endl; while (true) { cout << "Please enter a numeric option:"; cin >> num; switch (num) { case 1: { L = LinkList_Init(); cout << "L The linked list is:"; LinkList_Display(L); cout << endl; }break; case 2: { LinkList_Convert(L); cout << "The linked list is:"; LinkList_Display(L); cout << endl; }break; case 3: { LinkList_Sort(L); cout << "The linked list is:"; LinkList_Display(L); cout << endl; }break; case 4: { LinkLIst_Del_oushu(L); cout << "The linked list is:"; LinkList_Display(L); cout << endl; }break; case 5: { ElemType elem; cout << "Enter the element to insert:"; cin >> elem; LinkList_Sort(L); LinkList_Insert(L, elem); cout << "The linked list is:"; LinkList_Display(L); cout << endl; }break; case 6: { L1 = LinkList_Init(); cout << "L1 The linked list is:"; LinkList_Display(L1); L2 = LinkList_Init(); cout << "L2 The linked list is:"; LinkList_Display(L2); LinkList_Cat(L1, L2); cout << "The merged linked list is:"; LinkList_Display(L1); cout << endl; }break; case 7: { L2 = new LNode; LinkList_Divide(L1, L2); cout << "L1 The linked list is:"; LinkList_Display(L1); cout << "L2 The linked list is:"; LinkList_Display(L2); LinkList_Free(L2); cout << endl; }break; case 8: { LinkList_Free(L); delete L1; cout << endl; cout << "The linked list has been released and deleted"<<endl; cout << endl; }break; default: cout << "Input error! Please re-enter!" << endl; cout << endl; } } return 0; }
Operation screenshot:
Bidirectional linked list
Bidirectional linked list, also known as double linked list, is a kind of linked list. There are two pointers in each data node, pointing to the direct successor and direct precursor respectively. Therefore, starting from any node in the two-way linked list, you can easily access its predecessor node and successor node. Generally, we construct a two-way circular linked list.
Creation of bidirectional linked list
Head interpolation and tail interpolation only look at the code, which is difficult to understand. It is suggested that drawing the change diagram of the linked list when inserting nodes will be very easy to understand. In addition, I think head interpolation is better because its insertion logic is in place in one step. Unlike tail interpolation, it also needs to complete the ring connection of the two-way linked list later.
1. Head insertion method
Node * creatList() { Node * head = (Node*)malloc(sizeof(Node)); Node * cur = NULL; head->next = head; head->pre = head; int data; scanf("%d",&data); while(data) { cur = (Node*)malloc(sizeof(Node)); cur->data = data; cur->next = head->next; cur->pre = head; head->next = cur; cur->next->pre = cur; scanf("%d",&data); } return head; }
2. Tail interpolation
Node * creatList() { Node * head = (Node*)malloc(sizeof(Node)); Node * phead = head; Node * cur = NULL; phead->next = NULL; phead->pre = NULL; int data; scanf("%d",&data); while(data) { cur = (Node*)malloc(sizeof(Node)); cur->data = data; phead->next = cur; cur->pre = phead; phead = cur; scanf("%d",&data); } //Complete loopback cur->next = head; head->pre = cur; return head; }
Bidirectional linked list experiment
Experiment content:
1. Establish a two-way linked list by tail interpolation.
2. Traverse the two-way linked list.
3. Delete a specified element from the bidirectional linked list.
4. Implement the algorithm of inserting element e in non decreasing ordered bidirectional linked list.
5. Judge whether the elements in the two-way linked list are symmetrical. If they are symmetrical, return 1, otherwise return 0.
6. Set the element as positive integer, and implement the algorithm to arrange all odd numbers before even numbers.
7. Design a simple menu in the main function and call the above algorithm.
Experiment Description:
Type definition of bidirectional linked list
typedef int ElemType; // Element type typedef struct DuLNode { ElemType data; DuLNode *prior, *next; } DuLNode, *pDuLinkList;
source code
#include <iostream> #include <time.h> #include <stdlib.h> #include <iomanip> using namespace std; typedef int ElemType; typedef struct DuLNode { ElemType data; struct DuLNode *prior, *next; } DuLNode, *pDuLinkList; pDuLinkList DuLinkLinst_Init() //Establish a two-way linked list { int n; DuLNode *phead, *tmp, *p; srand(unsigned(time(NULL))); cout << "Enter the number of elements to create the linked list:"; cin >> n; phead = new DuLNode; phead->next = phead; phead->prior = phead; p = phead; for (int i = 0; i < n; i++) { tmp = new DuLNode; tmp->data = rand() % 100; tmp->prior = p; p->next = tmp; p = tmp; } p->next = phead; phead->prior = p; return phead; } void DuLinkList_Display(pDuLinkList phead) //Output bidirectional linked list { DuLNode *p; p = phead->next; while (p != phead) { cout << setw(4) << p->data; p = p->next; } cout << endl; } void DuLinkList_Free(pDuLinkList phead) //Release double linked list { DuLNode *p, *t; p = phead->next; while (p != phead) { t = p; p = p->next; delete t; } delete p; } void DuLinkList_Del(pDuLinkList phead, ElemType x) //Deletes the specified element { DuLNode *p1, *p2; p1 = phead->next; p2 = phead; while (p1) { if (x == p1->data) { p2->next = p1->next; p1->next->prior = p2; delete p1; return; } p2 = p1; p1 = p1->next; } cout << "Specified element not found!" << endl; } void DuLinkList_Sort(pDuLinkList phead) //sort { DuLNode *p; int count = 0, i = 0; ElemType *num, tmp; p = phead->next; if (p == phead) { cout << "The two-way linked list is empty!" << endl; return; } while (p != phead) { count++; p = p->next; } num = new ElemType[count]; p = phead->next; while (p != phead) { num[i++] = p->data; p = p->next; } for (int k = 0; k < count - 1; k++) for (int j = 0; j < count - k - 1; j++) if (num[j] > num[j + 1]) { tmp = num[j]; num[j] = num[j + 1]; num[j + 1] = tmp; } p = phead->next; i = 0; while (p != phead) { p->data = num[i++]; p = p->next; } delete[] num; } void DuLinkList_Insert(pDuLinkList phead, ElemType elem) //Insert an element { DuLNode *p, *tmp; p = phead->next; while (p != phead) { if (elem <= p->data) break; p = p->next; } tmp = new DuLNode; tmp->data = elem; tmp->next = p; tmp->prior = p->prior; p->prior->next = tmp; p->prior = tmp; } int DuLinkList_symmetry(pDuLinkList phead) //Are the elements in the linked list symmetrical { DuLNode *p1, *p2; p1 = phead->prior; p2 = phead->next; while (p1 != p2) { if (p1->data != p2->data) { return 0; } p1 = p1->prior; p2 = p2->next; } return 1; } void DuLinkList_jiousort(pDuLinkList phead) //Odd numbers precede even numbers { DuLNode *p1, *p2; p1 = phead->next; p2 = p1->next; if (p1 == phead) { cout << "The two-way linked list is empty!" << endl; return; } if (p2 == phead) { return; } while (p1 != phead) { if ((p1->data) % 2 != 0) { p2 = p1; p1 = p1->next; p1->prior = p2->prior; p2->prior->next = p1; p2->next = phead->next; phead->next->prior = p2; phead->next = p2; p2->prior = phead; } else p1 = p1->next; } } int main() { DuLNode *L = NULL; ElemType t; int num; cout << "1:Using tail interpolation method to establish a two-way linked list" << endl; cout << "2:Traversing bidirectional linked list" << endl; cout << "3:Deletes a specified element from a two-way linked list" << endl; cout << "4:Insert elements in non decreasing ordered bidirectional linked list e Still ordered algorithm." << endl; cout << "5:Judge whether the elements in the two-way linked list are symmetrical. If they are symmetrical, return 1; otherwise, return 0" << endl; cout << "6:Set the element as positive integer, and the implementation algorithm arranges all odd numbers before even numbers" << endl; cout << "7:sign out" << endl; while (true) { cout << "Please enter a numeric option:"; cin >> num; switch (num) { case 1: { L = DuLinkLinst_Init(); cout << "L The two-way linked list is:"; DuLinkList_Display(L); cout << endl; }break; case 2: { cout << "The two-way linked list is:"; DuLinkList_Display(L); cout << endl; }break; case 3: { cout << "Enter the element to delete:"; cin >> t; DuLinkList_Del(L, t); cout << "The two-way linked list is:"; DuLinkList_Display(L); cout << endl; }break; case 4: { cout << "Enter the element to insert:"; cin >> t; DuLinkList_Sort(L); DuLinkList_Insert(L, t); cout << "The two-way linked list is:"; DuLinkList_Display(L); cout << endl; }break; case 5: { if(DuLinkList_symmetry(L)==1) cout << "Element symmetry in bidirectional linked list" << endl; else cout << "Asymmetric elements in a two-way linked list" << endl; cout << endl; }break; case 6: { DuLinkList_jiousort(L); cout << "The two-way linked list is:"; DuLinkList_Display(L); cout << endl; }break; case 7: { DuLinkList_Free(L); cout << endl; return 0; }break; default: cout << "Input error! Please re-enter!" << endl; cout << endl; } } return 0; }
Operation screenshot:
Summary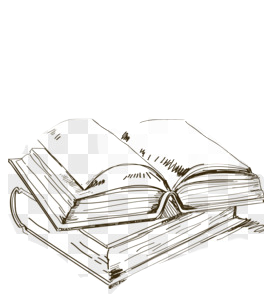
If the programming language is compared to a sentence, the data structure is like an article. Write a good sentence with clear meaning. If you write an article, you pay more attention to the idea it conveys. Mark Twain once said that when you hold a hammer in your hand, you see that many things are nails. I don't want to delve into the original meaning of this sentence. After all, it's not a technical problem. After reading a classic article by the famous hacker Raymond< asHe Cheng is a hacker >, I have a new understanding of this statement. He wrote in his article: "being a hacker will have a lot of fun, but it} is fun that takes a lot of effort. These efforts need motivation. Successful athletes get motivation from the pleasure of exercising and surpassing their own limits. Similarly, being a hacker, you have to get basic fun from solving problems, honing skills and exercising intelligence." Hammer is a tool, nail is a problem. When tools are used to solve your problems, it is difficult to describe the feeling of satisfaction. Learning C language is not just to learn C language. You want to use it to solve problems. Unfortunately, in reality, many students left this tool aside after finishing the C language course in their freshman and sophomore years, and even got a good score. It's a pity that they didn't enjoy it. Are you a man with a hammer? Hold on!
All right. That's all for today. It's time for me to go to bed. In the future, I will release more project sources or learning materials. I hope you can continue to pay attention and reply to any questions. If you want to master in advance, you can add groups to get C/C + + learning materials and the source code of other projects, you can add groups [1083227756] to understand. Interested in the future development of programmers, you can focus on WeChat official account: Fox encoding time, hope to learn progress with everyone!