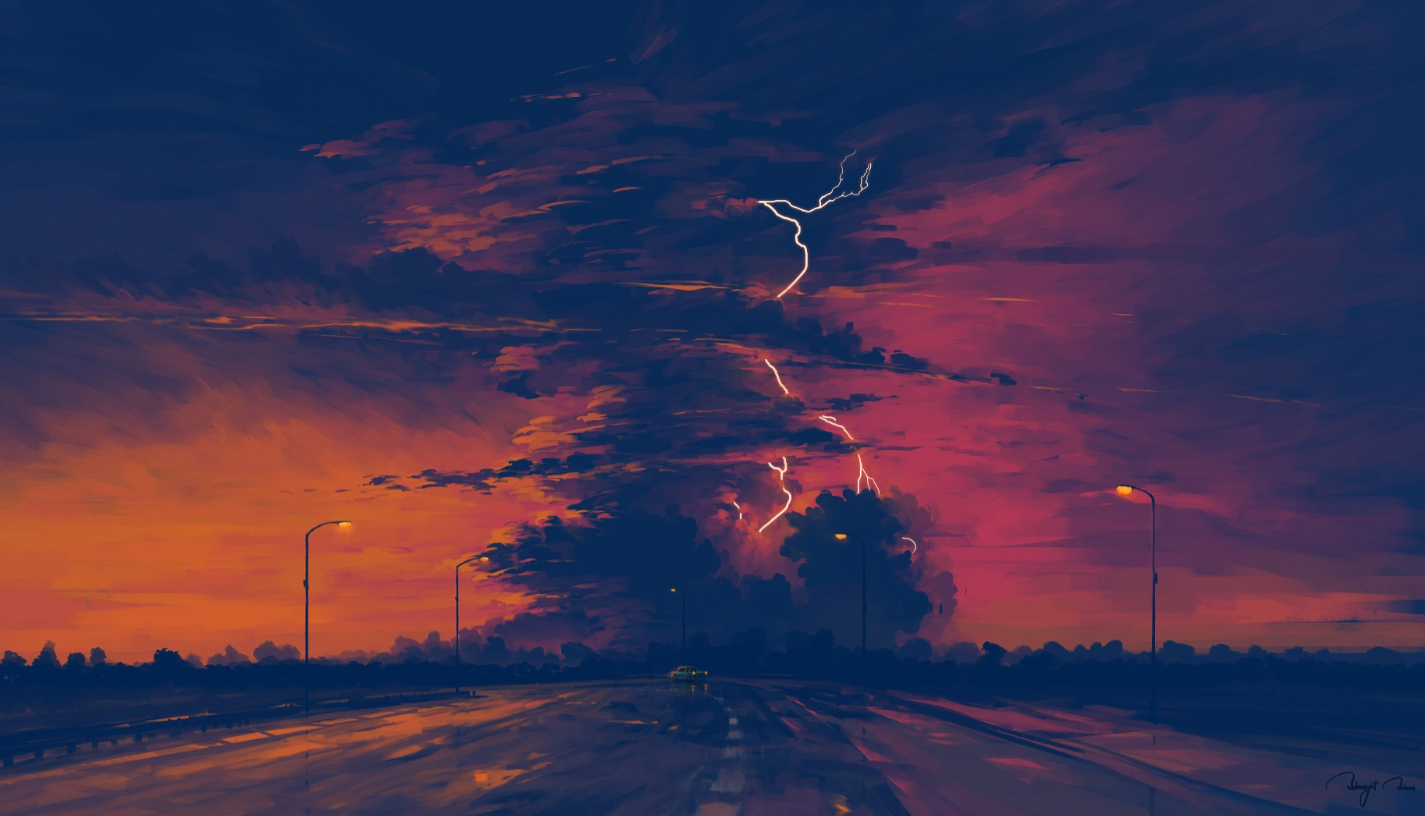
filter and map
Filter filter data (same as data)
The filter does not change the original array and returns a new array
return judge true and false
Returns the children of return true
Children of the original array are not modified
- Length: less than or equal to
- Data: subsets
Example 1:
let array1=['yma16','china'] let array2=array1 // Filter original array let array3=array2.filter((item,index)=>{ if(item=='china'){ item='changed' console.log('Original child',array2[index]) return true } }) console.log('array1',array1) console.log('array2',array2) console.log('array3',array3)
Do not modify the original array to generate children of the original array
The returned array is a subset of the original array
Example 2:
Even if the data is modified, the modified subset of the data is returned
let array1=['yma16','china'] let array2=array1 // Filter and modify children of the original array let array3=array2.filter((item,index)=>{ if(item=='china'){ console.log('Original child',array2[index]) array2[index]='changed' return true } }) console.log('array1',array1) console.log('array2',array2) console.log('array3',array3)
Since the assignment is a shallow copy, array2 is passed as a reference. When array2 is modified, array1 is also modified
Example 3:
If the sub item is a dictionary, modify the dictionary data,
let array1=[{name:'yma16',country:'china'}] let array2=array1 // Filter the original array let array3=array2.filter((item,index)=>{ item.age=18 return true }) console.log('array1',array1) console.log('array2',array2) console.log('array3',array3)
map returns an array object of the same length
The array map does not necessarily return its children
- Data: customizable
- Length: consistent with the original array
Example 1:
map does not filter the array. If no data is returned, undefined is returned as the child by default
let array1=['yma16','china'] let array2=array1 // Returns a new array let array3=array2.map((item,index)=>{ if(item=='china'){ item='changed' console.log('Original child',array2[index]) return item } }) console.log('array1',array1) console.log('array2',array2) console.log('array3',array3)
Deep and shallow copy
Shallow copy
Ordinary assignment is shallow copy, and reference is passed
Example 1:
let array1=['yma16','china'] let array2=array1 // Shallow copy array2.map((o,index)=>{ array2[index]='I am array2' }) console.log('array1',array1) console.log('array2',array2)
Deep copy of array
Deep copy of array
JSON.stringify resolves an object to a string
JSON.parse parses a string into key value pairs
exist side by side and play a part together
Example 1:
Deep copy array with addresses pointing to different
let array1=['yma16','china'] let array2=JSON.parse(JSON.stringify(array1)) // Deep copy array2.map((o,index)=>{ array2[index]='I am array2' }) console.log('array1',array1) console.log('array2',array2)
Strange tricks
Expand Syntax
Expand syntax and object The behavior of assign () is consistent. It executes shallow copies (only traverses one layer). You can expand the array expression or string at the syntax level during function call / array construction; You can also expand the object expression by key value when constructing a literal object
Example: expand syntax deep copy array
let array1=['yma16','china'] let array2=[...array1] // Deep copy array2.map((o,index)=>{ array2[index]='I am array2' }) console.log('array1',array1) console.log('array2',array2) VM1696:7 array1 (2) ["yma16", "china"] VM1696:8 array2 (2) ["I am array2", "I am array2"]
Array de duplication
function noRepeat(arr) { return [...new Set(arr)] }
function noRepeat(arr) { return Array.from(new Set(arr)) }
Array max, min
maximum
function arrayMax(arr) { return Math.max(...arr); }
minimum
function arrayMin(arr) { return Math.min(...arr); }
The number of occurrences of a value in the array
function countOccurrences(arr, value) { return arr.reduce((a, v) => v === value ? a + 1 : a + 0, 0); }
Returns the different elements of two arrays
function diffrence(arrA, arrB) { return arrA.filter(v => !arrB.includes(v)) }
Returns the same elements of two arrays
function intersection(arr1, arr2) { return arr2.filter(v => arr1.includes(v)) }