function
call
- Syntax: FN call(obj,…args)
- Function: execute fn, make this obj, and pass the following n parameters to fn
Function.prototype.myCall = function (obj, ...args) { if (obj == undefined || obj == null) { obj = globalThis } obj.fn = this let res = obj.fn(...args) delete obj.fn return res } value = 2 let foo = { value: 1, } let bar = function (name) { console.log(name, this.value) } bar.myCall(foo, 'HearLing', 18) //HearLing 18 1 bar.myCall(null, 'HearLing', 18) //HearLing 18 2 Copy code
apply
- Syntax: FN apply(obj,arr)
- Function: execute fn, make this obj, and pass the elements in the arr array to fn
Function.prototype.myAplly = function (obj, arr) { if (obj == undefined || obj == null) { obj = globalThis } obj.fn = this let res = obj.fn(...arr) delete obj.fn return res } value = 2 let foo = { value: 1, } let bar = function (name, age) { console.log(name, age, this.value) } bar.myAplly(foo, ['HearLing', 18]) //HearLing 18 1 bar.myAplly(null, ['HearLing', 18]) //HearLing 18 2 Copy code
bind
- Syntax: FN bind(obj,…args)
- Function: return a new function, bind this to obj for fn, and specify the parameters as the following n parameters
Function.prototype.myBind = function (obj, ...args) { let that = this let fn = function () { if (this instanceof fn) { return new that(...args) } else { return that.call(obj, ...args) } } return fn } value = 2 let foo = { value: 1, } let bar = function (name, age) { console.log(name, age, this.value) } let fn = bar.myBind(foo, 'HearLing', 18) //fn() //HearLing 18 1 let a = new fn() //HearLing 18 undefined console.log(a.__proto__)//bar {} Copy code
Difference call()/apply()/bind())
call(obj)/apply(obj): call the function, specify this in the function as the value of the first parameter, bind(obj): return a new function, the original function will be called inside the new function, and this is the value of the first parameter specified by bind()
throttle
- Understanding: when a function is triggered frequently for many times, the function will be executed for the second time only after it is greater than the set execution cycle
- Scenario: page scroll ing, mousemove of DOM elements, click of snapping up, playing events and calculating progress information
- Function: the throttling function can be executed at most once within the set delay milliseconds (in short, I lock it. No matter how many times you click, I unlock it when the time is up)
function throttle(fn, delay) { let flag = true return (...args) => { if (!flag) return flag = flase setTimeout(() => { fn.apply(this, args) flag = true }, delay) } } Copy code
Anti shake
- Understanding: when the function is triggered frequently, only the last time will take effect within the specified time
- Scenario: search box real-time keyup/input, button click too fast, multiple requests (login, SMS), window adjustment (resize)
- Function: the anti shake function is called after the delay of delay milliseconds after being invoked. No time to delay, you click again and clear the timer to re count until you really wait for delay for so many seconds.
function debounce(fn, delay) { let timer = null return (...args) => { clearTimeout(timer) timer = setTimeout(() => { fn.apply(this, args) }, delay) } } Copy code
Difference between throttling and anti chattering
First, explain the difference in concept, what is anti shake throttling; Then there are different usage scenarios; Classic distinguishing picture: [picture uploading... (image-7eb6d7-1612680062643-0)]
curry
function mycurry(fn, beforeRoundArg = []) { return function () { let args = [...beforeRoundArg, ...arguments] if (args.length < fn.length) { return mycurry.call(this, fn, args) } else { return fn.apply(this, args) } } } function sum(a, b, c) { return a + b + c } let sumFn = mycurry(sum) console.log(sumFn(1)(2)(3))//6 Copy code
array
Array de duplication
function unique(arr) { const res = [] const obj = {} arr.foreach((item) => { if (obj[item] === undefined) { obj[item] = true res.push(item) } }) return res } //Other methods //Array.from(new Set(array)) //[...new Set(array)] Copy code
Flatten arrays
// Recursive expansion function flattern1(arr) { let res = [] arr.foreach((item) => { if (Array.isArray(item)) { res.push(...flattern1(item)) } else { res.push(item) } }) return res } Copy code
object
new
function newInstance (Fn, ...args) { const obj = {} obj.__proto__ = Fn.prototype const result = Fn.call(obj, ...args) // If Fn returns an object type, it will no longer return obj, but the object returned by Fn. Otherwise, it will return obj return result instanceof Object ? result : obj } Copy code
instanceof
function instance_of(left, right) { let prototype = right.prototype while (true) { if (left === null) { return false } else if (left.__proto__ === prototype) { return true } left = left.__proto__ } } let a = {} console.log(instance_of(a, Object))//true Copy code
Object array copy
Shallow copy
// Shallow copy method //Object.assign(target,...arr) // [...arr] // Array.prototype.slice() // Array.prototype.concate() function cloneShallow(origin) { let target = {} for (let key in origin) { if (origin.hasOwnProperty(key)) { target[key] = origin[key] } } return target } let obj = { name: 'lala', skill: { js: 1, css: 2, }, } let newobj = cloneShallow(obj) newobj.name = 'zhl' newobj.skill.js = 99 console.log(obj)//{ name: 'lala', skill: { js: 99, css: 2 } } console.log(newobj)//{ name: 'zhl', skill: { js: 99, css: 2 } } Copy code
Deep copy
// Shallow copy method //JSON.parse(JSON.stringify(obj)) function deepClone(source, hashMap = new WeakMap()) { let target = new source.constructor() if (source == undefined || typeof source !== 'object') return source if (source instanceof Date) return source(Date) if (source instanceof RegExp) return source(RegExp) hashMap.set(target, source)//Resolve circular references for (let key in source) { if (source.hasOwnProperty(key)) { target[key] = deepClone(source[key], hashMap) } } return target } let obj = {//More complex data should be considered name: 'lala', skill: { js: 1, css: 2, }, } let newobj = deepClone(obj) newobj.skill.js = 100 console.log(obj)//{ name: 'lala', skill: { js: 1, css: 2 } } console.log(newobj)//{ name: 'lala', skill: { js: 99, css: 2 } } Copy code
last
Analysis of front-end school recruitment interview Click here for free Full pdf view
{
js: 1,
css: 2,
},
}
let newobj = deepClone(obj)
newobj.skill.js = 100
console.log(obj)//{ name: 'lala', skill: { js: 1, css: 2 } }
console.log(newobj)//{ name: 'lala', skill: { js: 99, css: 2 } }
Copy code
# ### last **Analysis of front-end school recruitment interview[Click here for free](https://gitee.com/vip204888/web-p7) full pdf view** >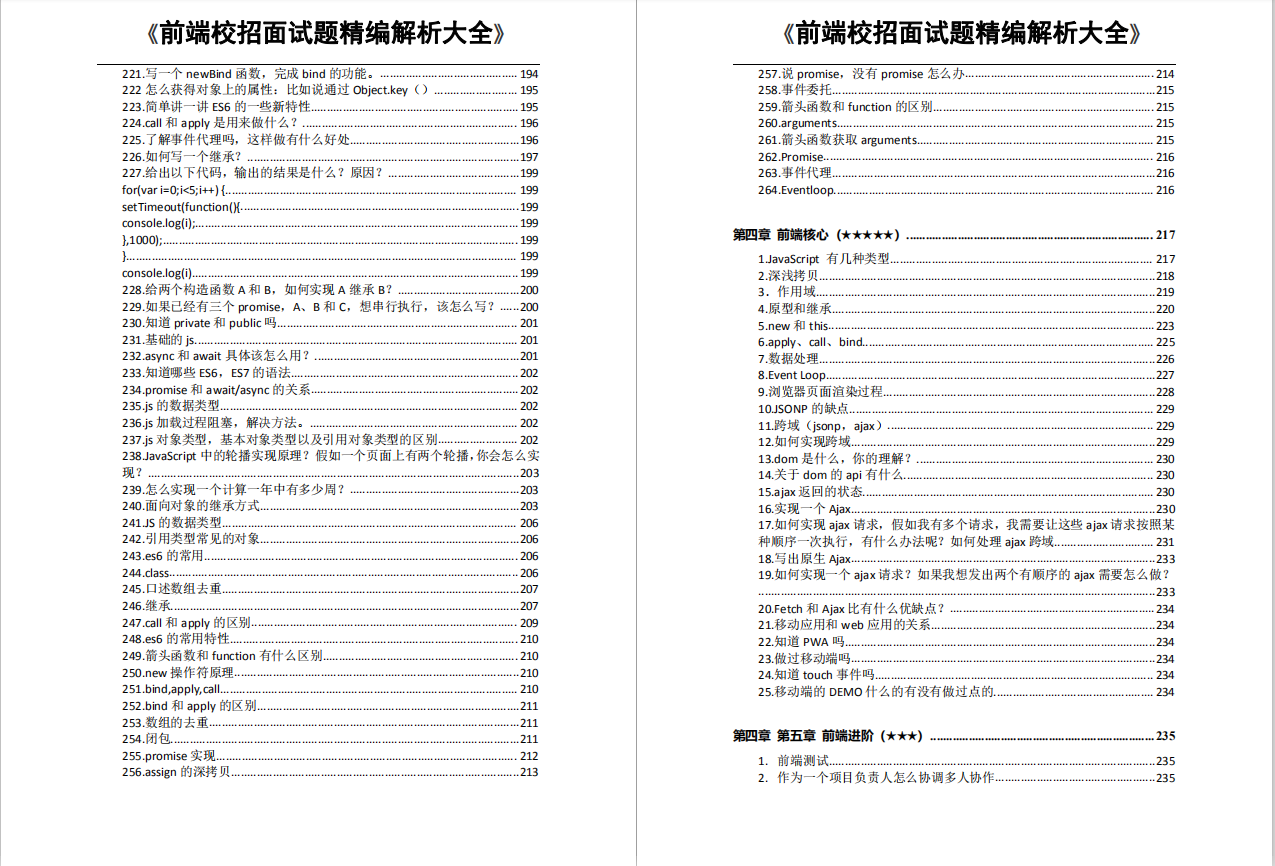