
Some error points about pointers:
The address of the first element is passed, not the whole array.
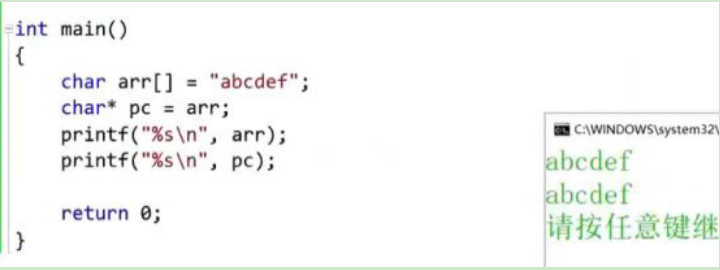
You can use an array or a pointer to print. The pointer will look for the last '\ 0' as the end.
The first dereference print is c. the following print is abcdef
It cannot be modified. The modification is wrong. Some compilers do not recognize errors, such as vs
Easy to see errors:
The method to solve the modification of constant string: add const.
int main() { char ch = 'w'; char *pc = &ch; *pc = 'w'; return 0; }
There is another way to use it as follows: code char* pstr = "hello bit."; It's especially easy for students to think it's a string hello bit Put character pointer pstr in Yes, but/The essence is to put the string hello bit. The address of the first character is put in pstr Yes. int main() { char* pstr = "hello bit.";//Did you put a string into the pstr pointer variable? printf("%s\n", pstr); return 0; }
Array pointers: essentially pointers.
Let's look at some concepts first
The above shows an array with four pointers.
Similarly, an array has four pointers, but only the addresses of the first elements of the four arrays.
If you want to print, how to print:
parr[i] found an element of this array. It is equivalent to finding a pointer, and then printing 5 elements through this pointer.
Through the above, you can clearly understand the array pointer.
Do a few small exercises to write array pointers:
How to print array pointers?
Pointer dereference to find the array, and then print it by using the properties of the array.
Compare the printing of ordinary arrays:
Use of an array pointer:
#include <stdio.h> void print_arr1(int arr[3][5], int row, int col) { int i = 0; for(i=0; i<row; i++) { for(j=0; j<col; j++) { printf("%d ", arr[i][j]); } printf("\n"); } } void print_arr2(int (*arr)[5], int row, int col) { int i = 0; for(i=0; i<row; i++) { for(j=0; j<col; j++) { printf("%d ", arr[i][j]); } printf("\n"); } } int main() { int arr[3][5] = {1,2,3,4,5,6,7,8,9,10}; print_arr1(arr, 3, 5); //The array name arr represents the address of the first element //But the first element of the two-dimensional array is the first row of the two-dimensional array //So the arr passed here is actually equivalent to the address of the first line, which is the address of a one-dimensional array //You can use array pointers to receive print_arr2(arr, 3, 5); return 0; }
If you print the following with an array pointer,
Printing rules: first look at the one-dimensional array.
Different forms of discrimination
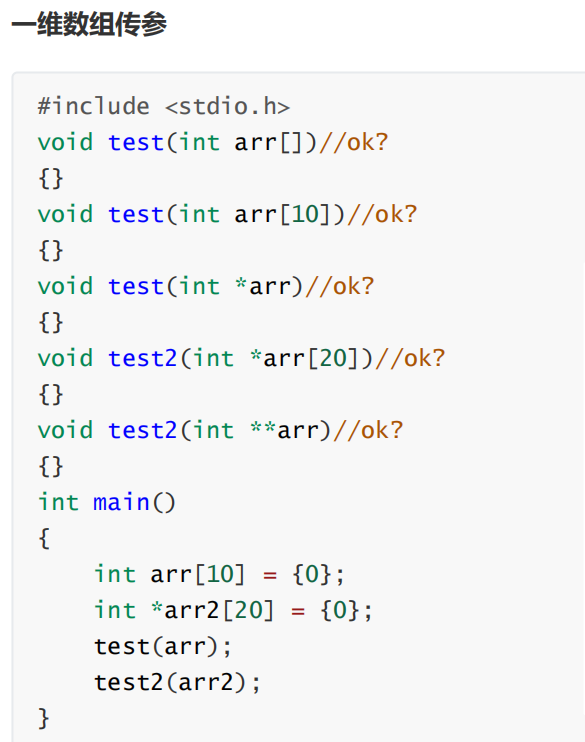
The array name is the address of the first element. The first element is a pointer of type int * and the variables storing it use secondary pointers.
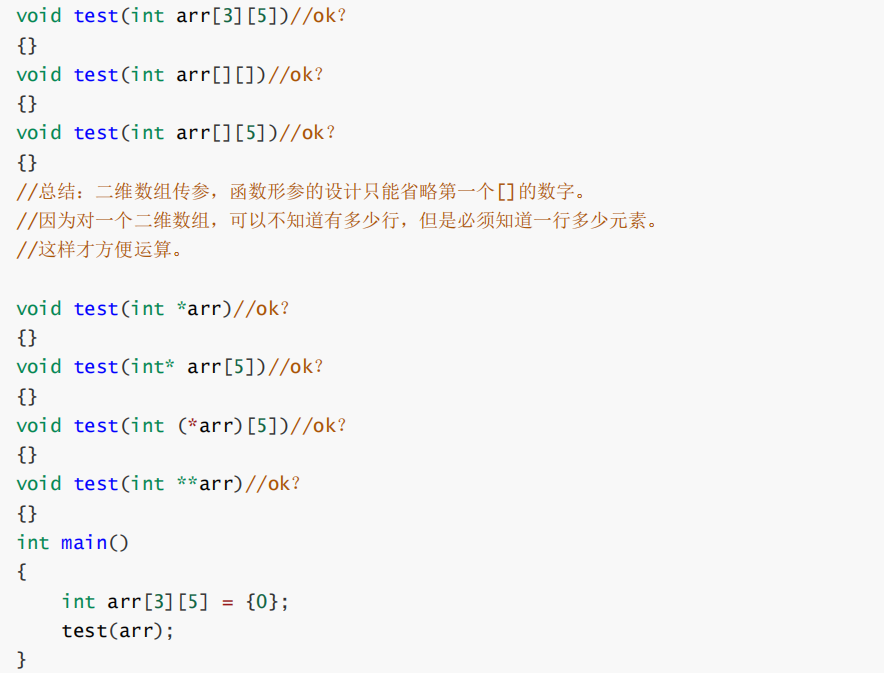
The above two-dimensional array parameters cannot omit columns.
The array name is the address of the first element. The address of a one-dimensional array cannot be placed in the integer pointer or in the secondary pointer. The secondary pointer is used to store the address of the primary pointer variable. Only the third below can be used.
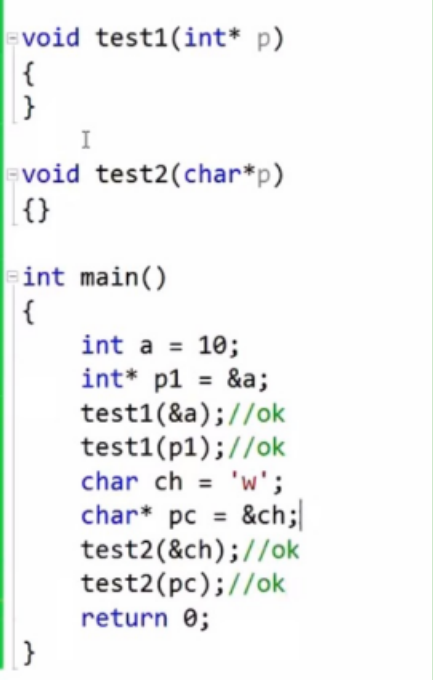
Function pointer:
Dereference of function pointer:
Use the function pointer to build a calculator:
#include <stdio.h> int add(int a, int b) { return a + b; } int sub(int a, int b) { return a - b; } int mul(int a, int b) { return a*b; } int div(int a, int b) { return a / b; } int main() { int x, y; int input = 1; int ret = 0; do { printf( "*************************\n" ); printf( " 1:add 2:sub \n" ); printf( " 3:mul 4:div \n" ); printf( "*************************\n" ); printf( "Please select:" ); scanf( "%d", &input); switch (input) { case 1: printf( "Input operand:" ); scanf( "%d %d", &x, &y); ret = add(x, y); printf( "ret = %d\n", ret); break; case 2: printf( "Input operand:" ); scanf( "%d %d", &x, &y); ret = sub(x, y); printf( "ret = %d\n", ret); break; case 3: printf( "Input operand:" ); scanf( "%d %d", &x, &y); ret = mul(x, y); printf( "ret = %d\n", ret); break; case 4: printf( "Input operand:" ); scanf( "%d %d", &x, &y); ret = div(x, y); printf( "ret = %d\n", ret); break; case 0: printf("Exit program\n"); breark; default: printf( "Selection error\n" ); break; } } while (input); return 0; }
Function pointer:
#include <stdio.h> int add(int a, int b) { return a + b; } int sub(int a, int b) { return a - b; } int mul(int a, int b) { return a*b; } int div(int a, int b) { return a / b; } int main() { int x, y; int input = 1; int ret = 0; int(*p[5])(int x, int y) = { 0, add, sub, mul, div }; //Transfer table while (input) { printf( "*************************\n" ); printf( " 1:add 2:sub \n" ); printf( " 3:mul 4:div \n" ); printf( "*************************\n" ); printf( "Please select:" ); scanf( "%d", &input); if ((input <= 4 && input >= 1)) { printf( "Input operand:" ); scanf( "%d %d", &x, &y); ret = (*p[input])(x, y); } else printf( "Incorrect input\n" ); printf( "ret = %d\n", ret); } return 0; }
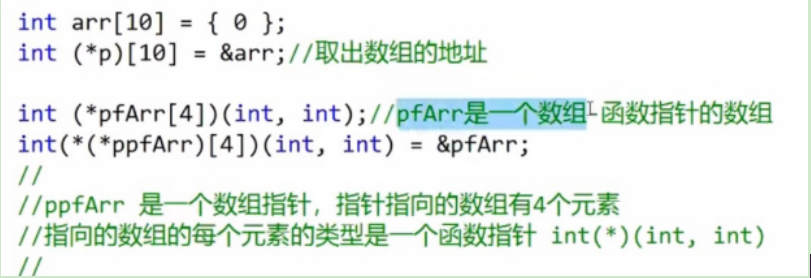
Callback function:
Create a sort function:
About void parameters:
Use of sorting function:
-----------------------------------------------------------------------------------------------------------------------------
Pointer example:
Example:
There are different types between them. The compiler will have a warning.
Find the dereference in the second line, which is equivalent to getting the array name in the second line.
Printed at.