1, Foreword
The interface request body has a sign signature parameter. The sign signature is generated by splicing the request parameters and finally md5 encrypting the request parameters after removing the sign parameter from the request body
Using jmeter to test the interface, we hope to modify the value of the sign parameter in the post body to the value of the signature before the request
2, sign signature
The implementation of signature is to add BeanShell preprocessor, generate sign value, set a variable, and then reference the variable in the requested body
Refer to the previous article: https://blog.csdn.net/x2waiwai/article/details/122843324
Next, let's talk about another implementation method. In the BeanShell preprocessor, first obtain the body of the request, sign, re assign the sign parameter, and then send a new request body
The overall implementation idea is as follows
1. Get the request body value first
2.body to JSONObject object
3. According to the sign signature rules, traverse the values of JSONObject objects, and then sort them
4. After sorting, splice the signature key to generate a new string
5.md5 encryption to obtain the sign signature value
6. Add the sign attribute to the jsonObject object
7.JSONObject to json string
8. Reassign the requested body
Add the preprocessing of the above functions before sending the request to realize the automatic signature of the body parameter
3, BeanShell preprocessor
HTTP request samples in the body can be signed without the sign parameter
Add BeanShell preprocessor
The overall code is implemented as follows
import org.apache.jmeter.config.Arguments; import org.apache.jmeter.config.Argument; import org.json.JSONObject; import org.json.JSONArray; import org.apache.commons.codec.digest.DigestUtils; //Import md5 encrypted package Arguments arguments = sampler.getArguments(); Argument arg = arguments.getArgument(0); // 1. Get the request body value String body = arg.getValue(); log.info(body); // 2.body to json object JSONObject jsonObject = new JSONObject(body); String user = jsonObject.getString("username"); String psw = jsonObject.getString("password"); log.info(user); //3. The obtained json object removes the parameters of sign itself, splicing parameters, sorting and splicing key // This section will not be implemented automatically with java code for the time being String a = "username" + user; log.info(a); String b = "password" + psw; log.info(b); String key = "12345678"; log.info(key); // 4. Concatenate signature key string after sorting c = b+a+key; log.info(c); // 5.md5 encryption to obtain the sign signature value String md5_after = DigestUtils.md5Hex(c); // md5 encryption log.info(md5_after); // 6. Add the sign parameter to the jsonObject object jsonObject.put("sign", md5_after); // 7.JSONObject to string String postData = jsonObject.toString(); log.info(postData); // 8. Reassign the body parameter of the request arg.setValue(postData);
Partial log after operation
2021-01-04 22:31:15,783 INFO o.a.j.t.JMeterThread: Thread started: Thread group 1-1 2021-01-04 22:31:15,786 INFO o.a.j.u.BeanShellTestElement: {"username": "test", "password": "123456", "mail": ""} 2021-01-04 22:31:15,788 INFO o.a.j.u.BeanShellTestElement: test 2021-01-04 22:31:15,788 INFO o.a.j.u.BeanShellTestElement: usernametest 2021-01-04 22:31:15,788 INFO o.a.j.u.BeanShellTestElement: password123456 2021-01-04 22:31:15,789 INFO o.a.j.u.BeanShellTestElement: 12345678 2021-01-04 22:31:15,790 INFO o.a.j.u.BeanShellTestElement: password123456usernametest12345678 2021-01-04 22:31:15,791 INFO o.a.j.u.BeanShellTestElement: 1aca01806e93bb408041965a817666af 2021-01-04 22:31:15,791 INFO o.a.j.u.BeanShellTestElement: {"password":"123456","mail":"","sign":"1aca01806e93bb408041965a817666af","username":"test"} 2021-01-04 22:31:15,929 INFO o.a.j.t.JMeterThread: Thread is done: Thread group 1-1
4, View result tree
View the result tree and run the result request. The body will bring the sign value
The response was successful
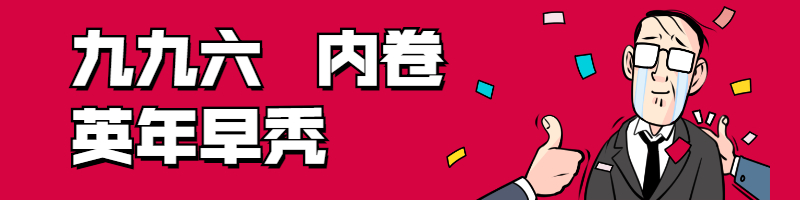
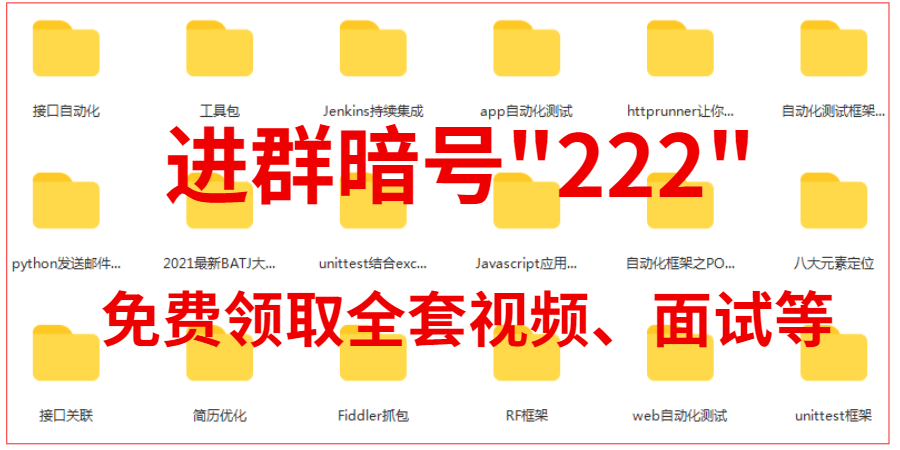
1. Complete project source code and environment necessary for self-study development or testing
2. All templates in test work (test plan, test case, test report, etc.)
3. Classic interview questions of software testing
4. Python/Java automated test practice pdf
5. Jmeter/postman interface test full set of video acquisition
I have personally sorted out some technical materials of my software testing career in recent years, including e-books, resume modules, various work templates, interview scriptures, self-study projects, etc. If you encounter problems in your study or work, you can directly Click this link If you enter the group and ask, there will also be great gods in the group to help answer, or you can add the group number manually 743262921 Remarks 222