The code is placed directly in the Github repository[ https://github.com/Damaer/Mybatis-Learning/tree/master/mybatis-06-resultMap ], it can be run directly, so it doesn't take up space.
Many times, we have such requirements. The field name of the database is inconsistent with the attribute name of the entity class. What do we need to do at this time? There are two solutions:
- Directly use the alias when querying, and set the alias to be consistent with the attribute name of the entity class.
- Use resultType to define the mapping relationship by yourself.
The contents of the whole project are as follows:

First, we need to build a mysql database environment (test. SQL). ID is written as sid,name is written as sname,age is written as sage:
#Create database CREATE DATABASE `test` DEFAULT CHARACTER SET utf8 COLLATE utf8_general_ci; #Create data table CREATE TABLE `student` ( `sid` INT NOT NULL AUTO_INCREMENT , `sname` VARCHAR(20) NOT NULL , `sage` INT NOT NULL , `score` DOUBLE NOT NULL , PRIMARY KEY (`sid`)) ENGINE = MyISAM;
Create student Class entity class:
public class Student { private Integer id; private String name; private int age; private double score; public Student(){ } public Student(String name, int age, double score) { super(); this.name = name; this.age = age; this.score = score; } public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public double getScore() { return score; } public void setScore(double score) { this.score = score; } @Override public String toString() { return "Student [id=" + id + ", name=" + name + ", age=" + age + ", score=" + score + "]"; } }
maven dependent POM xml:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.test</groupId> <artifactId>test</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <!-- mybatis Core package --> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>3.3.0</version> </dependency> <!-- mysql Driver package --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.29</version> </dependency> <!-- junit Test package --> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.11</version> <scope>test</scope> </dependency> <!-- Log file management pack --> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>1.2.17</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>1.7.12</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> <version>1.7.12</version> </dependency> </dependencies> </project>
Main configuration file mybatis xml:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!-- Configuration database file --> <properties resource="jdbc_mysql.properties"> </properties> <!-- Alias. The full name of data object operation is too long. Alias is required --> <typeAliases> <!--<typeAlias type="bean.Student" alias="Student"/>--> <!--You can use the class name directly. For the path configuration (alias) of the whole package, it is simple and fast --> <package name="bean"/> </typeAliases> <!-- Configure the running environment --> <!-- default It indicates which environment is used by default, and multiple can be configured, such as test environment during development, formal environment after online, etc --> <environments default="mysqlEM"> <environment id="mysqlEM"> <transactionManager type="JDBC"> </transactionManager> <dataSource type="POOLED"> <property name="driver" value="${jdbc.driver}"/> <property name="url" value="${jdbc.url}"/> <property name="username" value="${jdbc.user}"/> <property name="password" value="${jdbc.password}"/> </dataSource> </environment> </environments> <!-- Register mapping file --> <mappers> <mapper resource="mapper/mapper.xml"/> </mappers> </configuration>
Database configuration file (jdbc_mysql.properties):
jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql://localhost:3306/test jdbc.user=root jdbc.password=123456
Log configuration file log4j prperties:
log4j.prpp log4j.rootLogger=DEBUG, stdout log4j.appender.stdout=org.apache.log4j.ConsoleAppender log4j.appender.stdout.layout=org.apache.log4j.PatternLayout log4j.appender.stdout.layout.ConversionPattern=[service] %d - %c -%-4r [%t] %-5p %c %x - %m%n log4j.logger.java.sql.Statement = debug log4j.logger.java.sql.PreparedStatement = debug log4j.logger.java.sql.ResultSet =debug
Tool class used (MyBatisUtils.java):
public class MyBatisUtils { static private SqlSessionFactory sqlSessionFactory; static public SqlSession getSqlSession() { InputStream is; try { is = Resources.getResourceAsStream("mybatis.xml"); if (sqlSessionFactory == null) { sqlSessionFactory = new SqlSessionFactoryBuilder().build(is); } return sqlSessionFactory.openSession(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } return null; } }
Interface definition (IStudentDao.java):
public interface IStudentDao { // Return the information List of all students public List<Student> selectAllStudents(); // Find students by id public Student selectStudentById(int id); }
Interface implementation class (StudentDaoImpl.class):
public class StudentDaoImpl implements IStudentDao { private SqlSession sqlSession; public List<Student> selectAllStudents() { List<Student> students ; try { sqlSession = MyBatisUtils.getSqlSession(); students = sqlSession.selectList("selectAllStudents"); //Queries do not need to be modified, so transactions do not need to be committed } finally { if (sqlSession != null) { sqlSession.close(); } } return students; } public Student selectStudentById(int id) { Student student=null; try { sqlSession=MyBatisUtils.getSqlSession(); student=sqlSession.selectOne("selectStudentById",id); sqlSession.commit(); } finally{ if(sqlSession!=null){ sqlSession.close(); } } return student; } }
Main mapper files: Aliases can be used directly:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="abc"> <select id="selectAllStudents" resultType="Student"> select sid as id,sname as name,sage as age,score from student </select> <select id="selectStudentById" resultType="Student"> select sid as id,sname as name,sage as age,score from student where sid=${value} </select> </mapper>
Or you can define your own mapping:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="abc"> <resultMap id="StudentMapper" type="Student"> <id column="sid" property="id"/> <result column="sname" property="name"/> <result column="sage" property="age"/> </resultMap> <select id="selectAllStudents" resultMap="StudentMapper"> select sid as id,sname as name,sage as age,score from student </select> <select id="selectStudentById" resultMap="StudentMapper"> select sid as id,sname as name,sage as age,score from student where sid=${value} </select> </mapper>
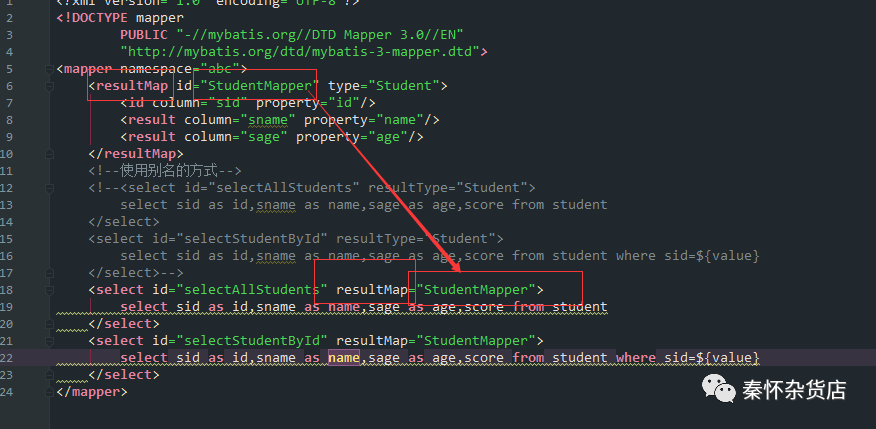
Points needing attention:
- There is an id attribute, which is the id used in other places
- Type - entity class. Alias can be written, or class name with full path should be written
- ID - tags are used to mark the results as IDS, which can help improve overall performance
- Result – the normal result injected into a field or JavaBean property
- association – a complex type of association; Many results will be wrapped into this type of nested result mapping – associations can be specified as a resultMap element or reference a
- Collection – a nested result map of a complex type of collection – a collection can be specified as a resultMap element or referenced as a result map element
- discriminator – use the result value to decide which resultMap case to use – result mapping based on some values nested result mapping – a case is also the result of its own mapping, so it can contain many same elements, or it can refer to an external resultMap. If the object name is consistent with the attribute name, we can not write it to < resultMap > < / resultMap >.
Test class mytest class:
public class MyTest { private IStudentDao dao; @Before public void Before(){ dao=new StudentDaoImpl(); } /* * Query list * */ @Test public void testselectList(){ List<Student> students=dao.selectAllStudents(); if(students.size()>0){ for(Student student:students){ System.out.println(student); } } } /* * Query student by id * */ @Test public void testselectStudentById(){ Student student=dao.selectStudentById(1); System.out.println(student); } }