background
In yesterday's product requirements review, the product manager received feedback from the user. There are some text fields in the system. The user hopes that the text content and format filled in the text can be retained. At present, only the text content can be saved. The line breaks and spaces in the text field are not displayed correctly.
After receiving this request, I searched. Most of the content in the text field on the Internet is obtained, and the line breaks and spaces in it are replaced with html tags, such as < br >, which are processed by JavaScript. Then, after these data are transformed, the saved back end uses innerHTML to display in a div or p tag. A big security risk here is script injection. If the user enters some scripts and these are not processed, they can be directly displayed on the page using innerHTML, which is very dangerous.
So I sought another solution.
Finally, I found that white space with element style can correctly display line breaks and spaces in text fields.
White space attribute interpretation
Here are some values of white space and their explanations
attribute | Line feed | Spaces and tabs | Text wrap | End of line space |
---|---|---|---|---|
normal | merge | merge | Line feed | delete |
nowrap | merge | merge | nowrap | delete |
pre | retain | retain | nowrap | retain |
pre-wrap | retain | retain | Line feed | Hang |
pre-line | retain | merge | Line feed | delete |
break-spaces | retain | retain | Line feed | Line feed |
normal
Consecutive whitespace characters are merged and line breaks are treated as whitespace characters. Line breaks are necessary when filling line boxes.
nowrap
Like normal, consecutive whitespace characters are merged. However, the newline in the text is invalid.
pre
Consecutive whitespace characters are retained. Line breaks or Element.
pre-wrap
Consecutive whitespace characters are retained. Line breaks or Element, or to fill in the "line boxes" before line wrapping.
pre-line
Consecutive whitespace characters are merged. Line breaks or Element, or when it is necessary to fill the "line boxes", the line will wrap.
break-spaces
The behavior is the same as that of pre wrap, except:
Any reserved blank sequence always takes up space, including at the end of the line.
There is a line break after each reserved space character, including between space characters.
This reserved space takes up space without hanging, which affects the inherent size of the box (minimum content size and maximum content size).
demo
In addition, I also specially wrote a simple demo to demonstrate the use of white space to correctly display the text format in the text field.
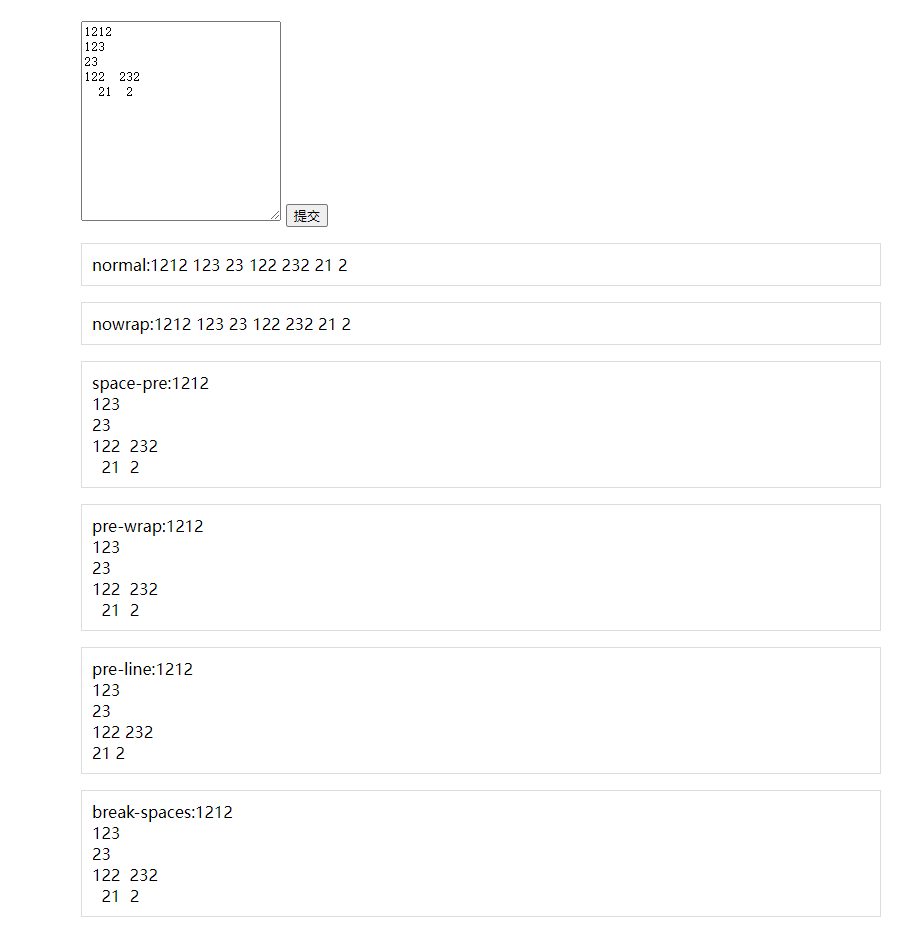
As can be seen from the above figure, setting the white space attribute of the element to space pre, pre wrap, pre line and break space can achieve good results
demo code
<html> <head> <title>Save text field formatting</title> <style type="text/css"> .showtext { padding: 10px; border: 1px solid #ddd; } .white-space-normal { white-space: normal; } .white-space-nowrap { white-space: nowrap; } .white-space-pre { white-space: pre; } .white-space-pre-wrap { white-space: pre-wrap; } .white-space-pre-line { white-space: pre-line; } .white-space-break-spaces { white-space: break-spaces; } </style> </head> <body> <div style="width: 800px; margin: 40px auto;"> <textarea id="myinput" style="height: 200px; width: 200px;"></textarea> <button onclick="submit()">Submit</button> <p id="normal" class="showtext white-space-normal">normal:</p> <p id="nowrap" class="showtext white-space-nowrap">nowrap:</p> <p id="pre" class="showtext white-space-pre">space-pre:</p> <p id="pre-wrap" class="showtext white-space-pre-wrap">pre-wrap:</p> <p id="pre-line" class="showtext white-space-pre-line">pre-line:</p> <p id="break-spaces" class="showtext white-space-break-spaces">break-spaces:</p> </div> <script> function submit() { const inputValue = document.querySelector('#myinput').value console.log('Text field content', inputValue); [...document.querySelectorAll('.showtext')].forEach(x => { x.append(inputValue) }) } </script> </body> </html>
reference
https://developer.mozilla.org/zh-CN/docs/Web/CSS/white-space