Without programming, generate CRUD, add, delete, modify and check RESTful API interface based on PostgreSQL zero code
review
In the previous article, the main functions and usage of crudapi have been introduced. crudapi 1.2.0 only supports MySQL database. In order to support more databases, the code is reconstructed and the abstract factory design mode is adopted to seamlessly switch different types of databases. Starting from crudapi 1.3.0, the support for elephant database PostgreSQL is added.
Abstract factory pattern
Abstract Factory Pattern is to create other factories around a super factory. The super factory is also known as the factory of other factories. This type of design pattern is a creation pattern, which provides the best way to create objects. In the Abstract Factory Pattern, an interface is a factory responsible for creating a related object, and there is no need to explicitly specify their classes. Each generated factory can provide objects according to the factory pattern.
UI interface
Taking the student object as an example, without programming, based on the PostgreSQL database, the addition, deletion, modification and query of CRUD, RESTful API interface and management UI are realized by configuring zero code.
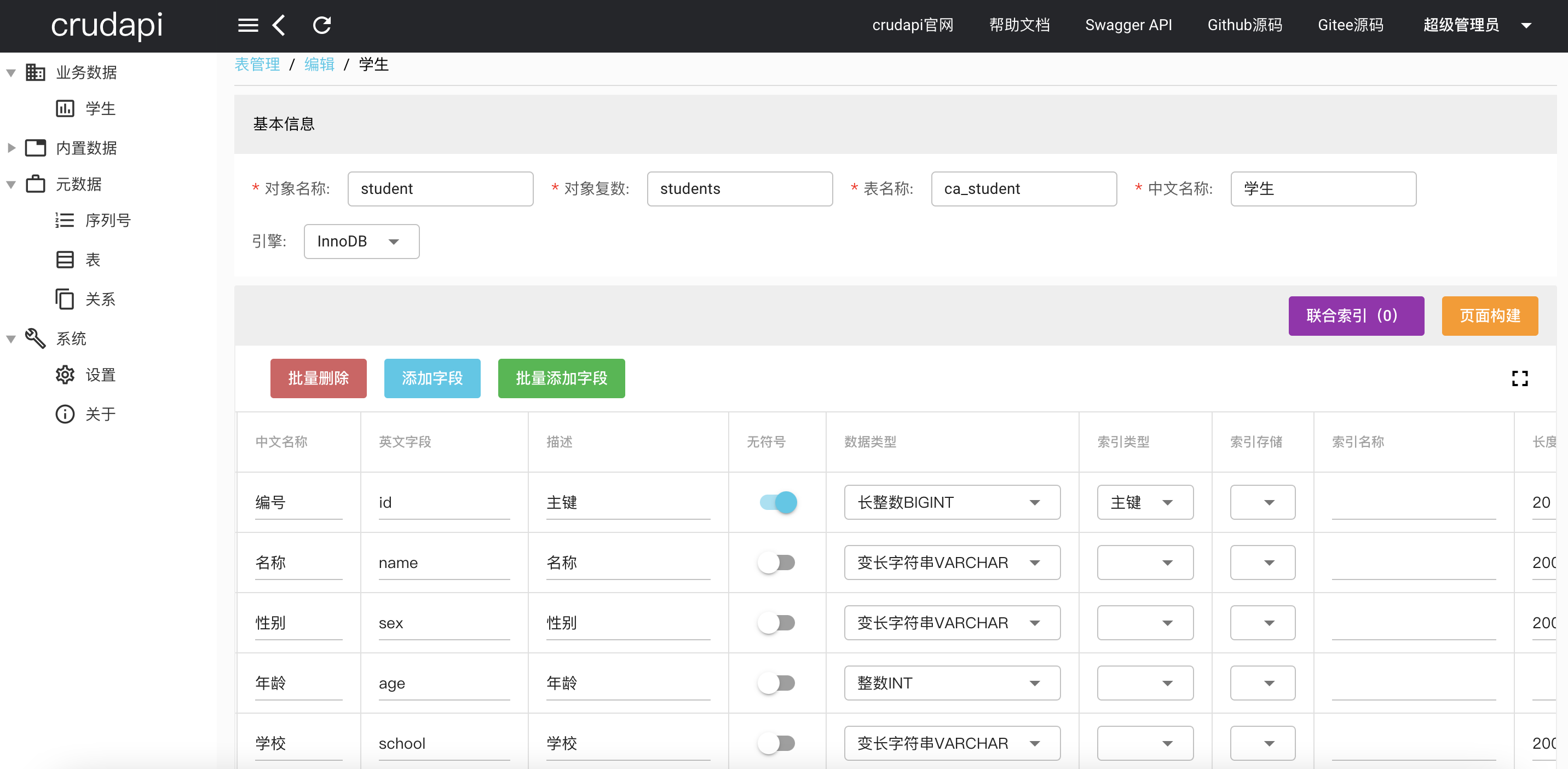
Create student table
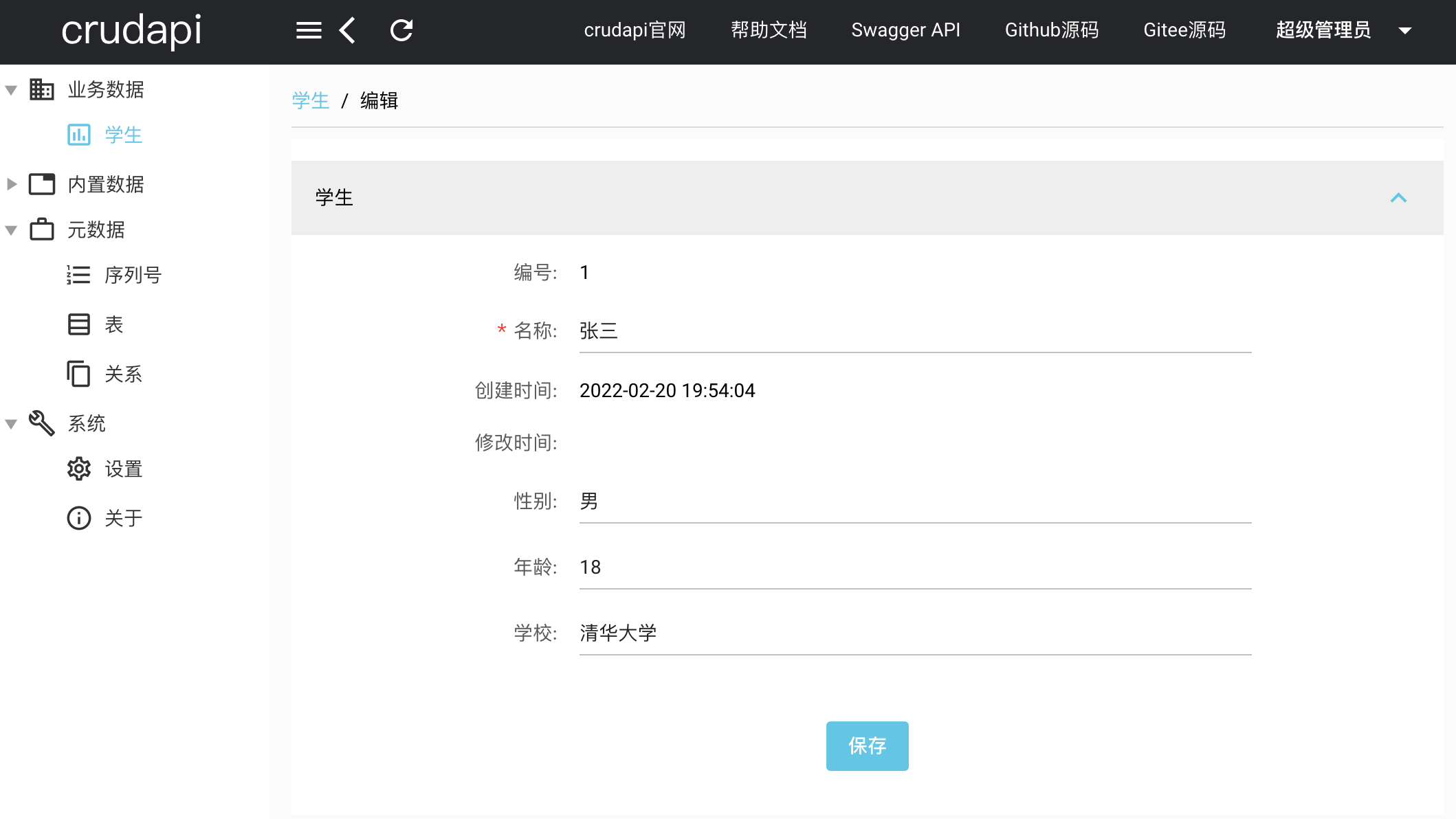
Edit student data
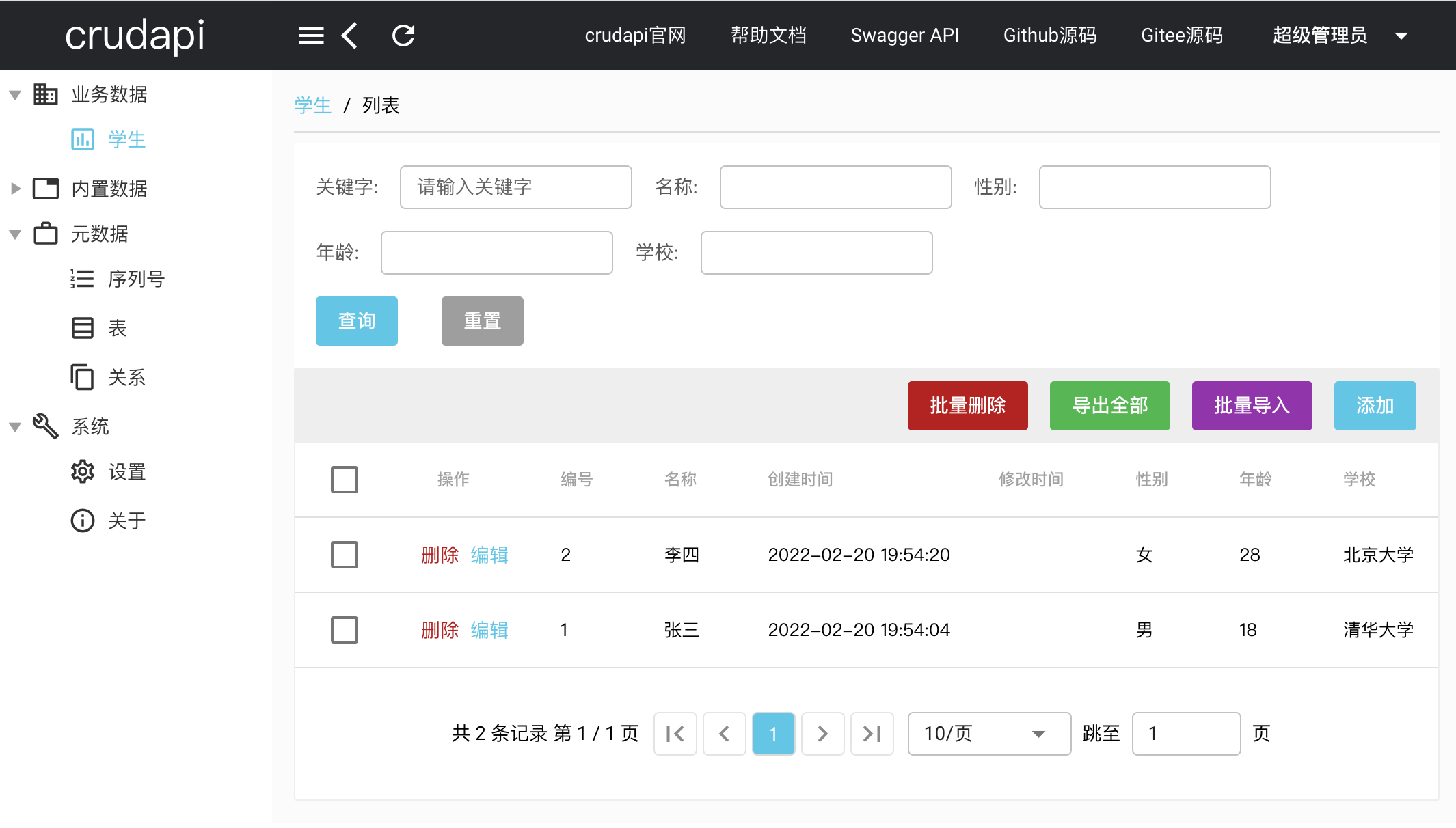
Student data list
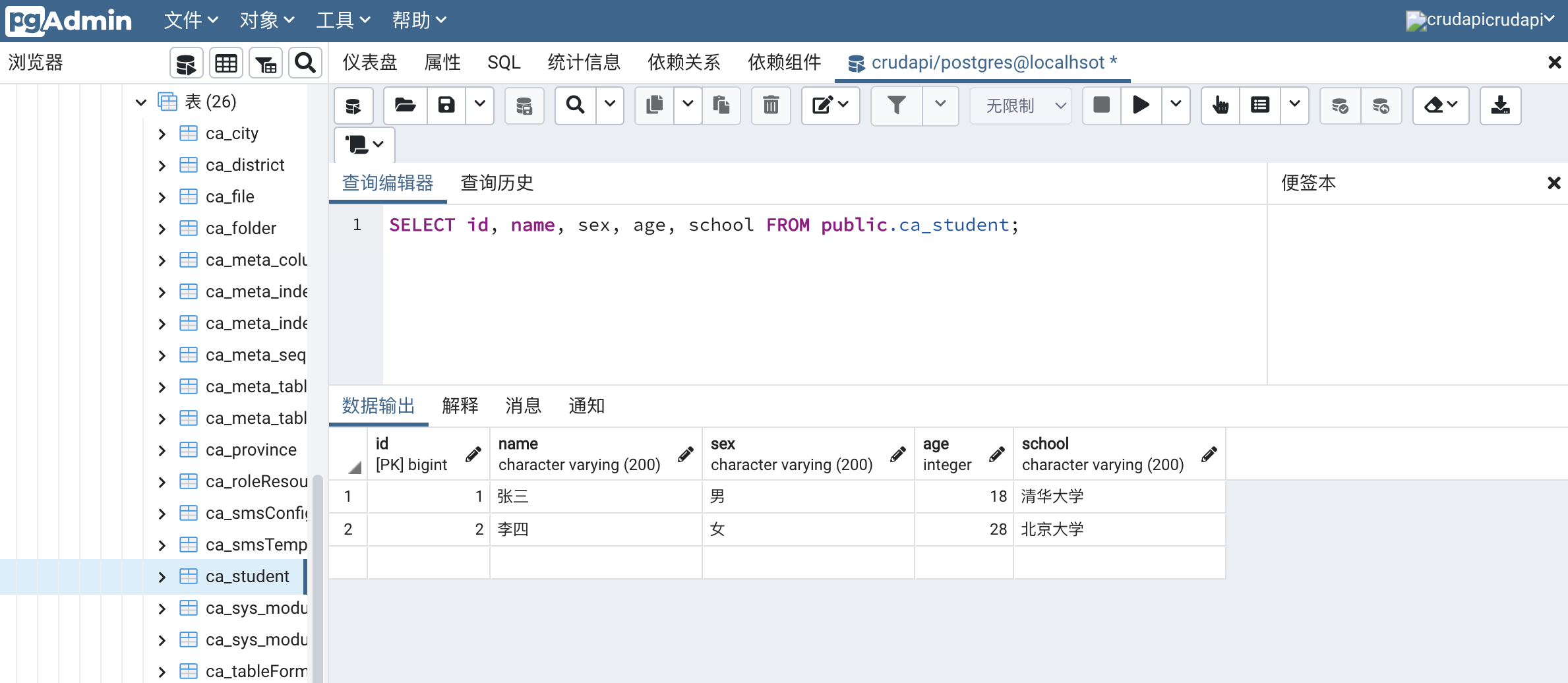
Query postsql data through pgadmin
Implementation principle
Base class
CrudAbstractRepository is an abstract class. Its main function is to add, delete, modify and query the database table.
public abstract class CrudAbstractRepository { public Long create(String tableName, Map<String, Object> map) { log.info("CrudAbstractRepository->create"); } }
CrudAbstractFactory is a factory class used to create CrudAbstractRepository.
public abstract class CrudAbstractFactory { public abstract CrudAbstractRepository getCrudRepository(); public Long create(String tableName, Map<String, Object> map) { log.info("CrudAbstractFactory->create"); CrudAbstractRepository repository = this.getCrudRepository(); return repository.create(tableName, map); } }
MySql subclass
CrudAbstractRepository implements the general database processing function. If there are different processing methods in MySql, you can copy the corresponding methods through Override, and finally the subclass overrides the parent method. For example, MySql crudrepository re implements the create add data function.
@Component public class MySqlCrudRepository extends CrudAbstractRepository { @Override public Long create(String tableName, Map<String, Object> map) { log.info("MySqlCrudRepository->create"); return super.create(tableName, map); } }
public class MySqlCrudFactory extends CrudAbstractFactory { @Autowired private MySqlCrudRepository mySqlCrudRepository; @Override public CrudAbstractRepository getCrudRepository() { return mySqlCrudRepository; } }
PostSql subclass
Similar to MySql, if there is a part in PostSqlCrudRepository that needs to be rewritten, it can directly overwrite the method with the same name.
@Component public class PostSqlCrudRepository extends CrudAbstractRepository { @Override public Long create(String tableName, Map<String, Object> map) { log.info("PostSqlCrudRepository->create"); return super.create(tableName, obj); } }
public class PostSqlCrudFactory extends CrudAbstractFactory { @Autowired private PostSqlCrudRepository postSqlCrudRepository; @Override public CrudAbstractRepository getCrudRepository() { return postSqlCrudRepository; } }
CrudTemplate
Read spring.com from CrudDatasourceProperties datasource. driverClassName
@ConfigurationProperties(prefix = "spring.datasource") @Component public class CrudDatasourceProperties { private String driverClassName; public String getDriverClassName() { return driverClassName; } public void setDriverClassName(String driverClassName) { this.driverClassName = driverClassName; } }
According to spring datasource. The value of driverclassname, which dynamically creates MySQL crudfactory or PostSqlCrudFactory factory objects through reflection,
@Configuration public class CrudTemplateConfig { public static final String MYSQL_DRIVER_NAME = "com.mysql.cj.jdbc.Driver"; Map<String, String> driverClassNameMap = new HashMap<String, String>() { private static final long serialVersionUID = 1L; { put("com.mysql.cj.jdbc.Driver", "cn.crudapi.core.repository.mysql.MySqlCrudFactory"); put("org.postgresql.Driver", "cn.crudapi.core.repository.postsql.PostSqlCrudFactory"); } }; @Autowired private CrudDatasourceProperties crudDatasourceProperties; @Bean public CrudTemplate crudTemplate(CrudAbstractFactory factory) { CrudTemplate crudTemplate = new CrudTemplate(factory); return crudTemplate; } @Bean public CrudAbstractFactory crudAbstractFactory() { CrudAbstractFactory crudAbstractFactory = null; String driverClassName = crudDatasourceProperties.getDriverClassName(); log.info("CrudTemplateConfig->driverClassName: " + driverClassName); try { String factoryClassName = driverClassNameMap.get(driverClassName); if (factoryClassName == null) { factoryClassName = driverClassNameMap.get(MYSQL_DRIVER_NAME); } log.info("CrudTemplateConfig->factoryClassName: " + factoryClassName); Class<?> cls = Class.forName(factoryClassName); Object obj = cls.newInstance(); crudAbstractFactory = (CrudAbstractFactory)obj; } catch (Exception e) { e.printStackTrace(); } return crudAbstractFactory; } }
Similar to RestTemplate, CrudTemplate finally realizes the function of crud addition, deletion, modification and query
public class CrudTemplate { @Nullable private volatile CrudAbstractFactory crudFactory; public CrudTemplate() { super(); log.info("CrudTemplate->Constructor"); } public CrudTemplate(CrudAbstractFactory crudFactory) { super(); log.info("CrudTemplate->Constructor crudFactory"); this.crudFactory = crudFactory; } public Long create(String tableName, Map<String, Object> map) { log.info("CrudTemplate->create"); return crudFactory.create(tableName, map); } }
application.properties
You need to configure the database connection driver as needed. You can switch between different databases without republishing.
#mysql spring.datasource.driverClassName=com.mysql.cj.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3306/crudapi spring.datasource.username= spring.datasource.password= #postgresql spring.datasource.driverClassName=org.postgresql.Driver spring.datasource.url=jdbc:postgresql://localhost:5432/crudapi spring.datasource.username= spring.datasource.password=
Summary
This paper mainly introduces the implementation principle of CRUD API supporting multiple databases. Taking student objects as an example, zero code implements CRUD addition, deletion, modification and query RESTful API. The follow-up plan supports more databases, such as Oracle, MSSQL Server, Mongodb, etc.
Implementation mode | Code quantity | time | stability |
---|---|---|---|
Traditional development | About 1000 lines | 2 days / person | About 5 bug s |
Crud API system | 0 rows | 1 minute | Basically 0 |
To sum up, using crudapi system can greatly improve work efficiency and save cost, and make data processing easier!
Introduction to crud API
Crud API is a combination of crud+api, which means the interface of adding, deleting, modifying and querying. It is a zero code configurable product. Using crud API can bid farewell to the boring addition, deletion, modification and query code, make you more focused on business, save a lot of costs, and improve work efficiency.
The goal of crud API is to make it easier to process data, and everyone can use it for free!
Without programming, crud is automatically generated through configuration, and RESTful API is added, deleted, modified and queried to provide background UI management business data. Based on the mainstream open source framework, it has independent intellectual property rights and supports secondary development.
Demo demo
Crud API is a product level zero code platform, which is different from automatic code generator. It does not need to generate business codes such as Controller, Service, Repository and Entity. The program can be used when running. The real 0 code can cover the basic CRUD RESTful API irrelevant to business.
Official website address: https://crudapi.cn
Test address: https://demo.crudapi.cn/crudapi/login
Source code address attached
GitHub address
https://github.com/crudapi/crudapi-admin-web
Gitee address
https://gitee.com/crudapi/crudapi-admin-web
Due to network reasons, GitHub may be slow. Just visit Gitee and update the code synchronously.