About promise of ES6 and yield of ES7
1.promise
1 Basic Concepts
-
name:It is translated as "commitment", which expresses the operation that will be executed in the future, representing asynchronous operation;
-
Status:There are three states: pending, completed, and rejected.
-
characteristic:(1) Only asynchronous operation can determine the current state, and any other operation cannot change this state;(2) Once the state changes, it won't change. The process of state change can only be: from pending to fully and from pending to rejected. If the state does not change after the above changes, it is called resolved
2. How to create a promise
How to create a promise (direct execution)
const promise = new Promise((resolve,reject) => {
if(sucess) {
resolve(value);
}else {
reject(error)
}
)
Note: for promose objects, {if you use new directly, it will be executed directly. If you want to define it, you can use functions to define such an asynchronous operation
Method 2 (asynchronous operation will not be executed immediately)
Function runasync() {var P = new promise (function (resolve, reject) {/ / do some asynchronous operations setTimeout (function() {console. Log ('execution completed '); resolve('whatever data');}, 2000); }); return p; } runAsync()
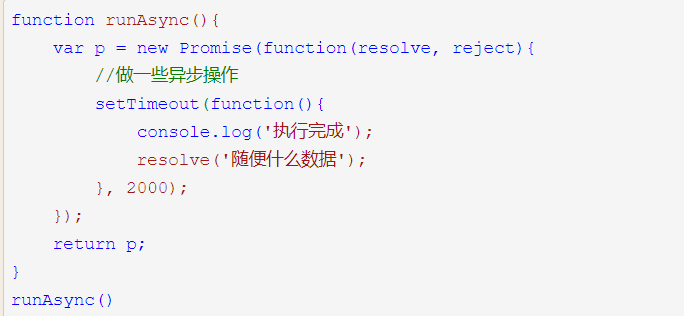
What is the relationship between resolve and reject and then
For example, we defined in the resolve function
function runAsync(){
var p = new Promise(function(resolve, reject){
/ / do some asynchronous operations
setTimeout(function(){
console.log('execution completed ');
resolve('Any data ');
}, 2000);
});
return p;
}
runAsync()
After executing runAsync asynchronously, if it is used again
runAsync().then(res=>{
console.log(res);
})
'whatever data' will be printed out
The callback embodiment of reject and resolve of asynchronous operation in then
function runAsync(){ let p = new Promise(function(resolve, reject){ //Do some asynchronous operations setTimeout(function (){ let num=Math.ceil(Math.random()*10); if(num<5) { resolve(num); }else{ reject('The number is too big!') } }, 2000); }); return p; }; runAsync().then(function(data){ console.log('resolve'); console.log(data) },function(data) { console.log('reject'); console.log(data) });
Finally, if the number is greater than 5, it will be printed out
Promise {<pending>}39787b1918052d26d1c92ea7ecfacad86e8dbfa2
VM296:19 reject
VM296:20 the number is too big!
3 promise.all
-
If all are successful, all callbacks that successfully execute resolve will be returned
var p1 = new Promise((resolve, reject) => { setTimeout(resolve, 1000, 'one'); }); var p2 = new Promise((resolve, reject) => { setTimeout(resolve, 2000, 'two'); }); var p3 = new Promise((resolve, reject) => { setTimeout(resolve, 3000, 'three'); }); var p4 = new Promise((resolve, reject) => { resolve('p4 reject!'); }); var p5 = new Promise((resolve, reject) => { resolve('p5 resolve!'); }); Promise.all([p1, p2, p3, p4, p5]).then(values => { console.log(values); }, reason => { console.log(reason) });
Promise {<pending>}
VM412:18 (5) ["one", "two", "three", "p4 reject!", "p5 resolve!"]
When any promise in the parameter fails to return, the whole immediately returns the failure, and the returned error message is the first failed promise result.
var p1 = new Promise((resolve, reject) => { setTimeout(resolve, 1000, 'one'); }); var p2 = new Promise((resolve, reject) => { setTimeout(resolve, 2000, 'two'); }); var p3 = new Promise((resolve, reject) => { setTimeout(resolve, 3000, 'three'); }); var p4 = new Promise((resolve, reject) => { reject('p4 reject!'); }); var p5 = new Promise((resolve, reject) => { reject('p5 reject!'); }); Promise.all([p1, p2, p3, p4, p5]).then(values => { console.log(values); }, reason => { console.log(reason) }); VM425:20 p4 reject!
4 promise.race
Race has the translation of race, so the returned new instance state is the instance that changes the state first in the following parameters; If it is not a Promise instance, use Promise first Resolve method, and then further processing after conversion.
If the passed iteration is empty, the returned Promise will wait forever
var p1 = new Promise((resolve, reject) => { setTimeout(resolve, 1000, 'one'); }); var p2 = new Promise((resolve, reject) => { setTimeout(resolve, 2000, 'two'); }); var p3 = new Promise((resolve, reject) => { setTimeout(resolve, 3000, 'three'); }); Promise.race([p1, p2, p3]).then(values => { console.log(values); }, reason => { console.log(reason) }); Promise {<pending>} VM446:12 one
2.async and await
1 Basic Concepts https://www.haorooms.com/post/es67application
Simple pause execution
var sleep = function (time) { return new Promise(function (resolve, reject) { setTimeout(function () { resolve(); }, time); })}; var start = async function () { console.log('start'); await sleep(3000); console.log('end');}; start();
Get return value
var sleep = function (time) { return new Promise(function (resolve, reject) { setTimeout(function () { // Return to 'ok' resolve('ok'); }, time); })}; var start = async function () { let result = await sleep(3000); console.log(result); // Received 'ok'}; start()
Capture error
var sleep = function (time) { return new Promise(function (resolve, reject) { setTimeout(function () { // Simulation error, return 'error' reject('error'); }, time); })}; var start = async function () { try { console.log('start'); await sleep(3000); // Here you get a return error // So the following code will not be executed console.log('end'); } catch (err) { console.log(err); // Error caught here ` error` }}; start()
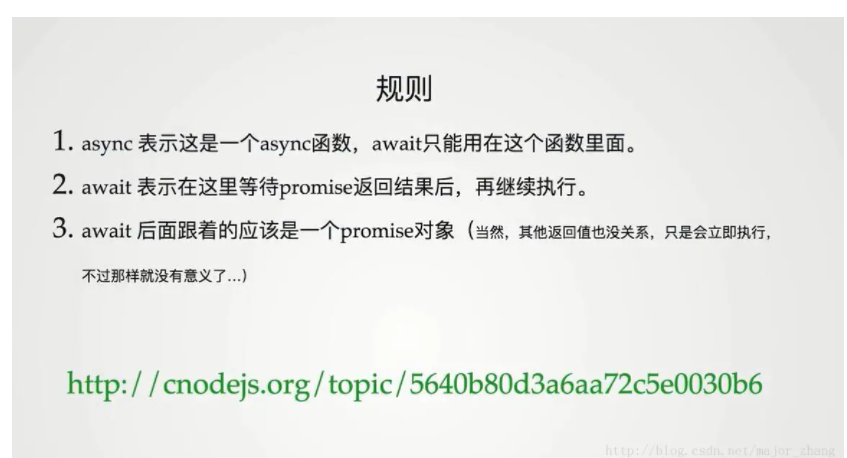