When learning a programming language, we have learned to configure the integrated development environment, basic syntax and basic programming skills. At this time, we will always encounter the bottleneck of in-depth learning of this programming language. When I was learning python programming, I always lingered in the above process for a period of time. This time, I worked hard to solve the first bottleneck I encountered - object-oriented python programming.
- When we can use git to clone ready-made programs from github for local development, and then use miniconda and docker to build the corresponding virtual environment for cloning different programs.
- However, in the face of hundreds of open source code modules from a team or company, how can we skillfully apply them in a short time? The secret lies in the in-depth understanding of Python object-oriented programming. Only by mastering object-oriented programming can we make full use of pychar class object operation function, which is very convenient for us to complete the development of Python.
1, Introduction to Python object orientation
The original intention of Python language design is an object-oriented programming language. Therefore, it is easy to create a class and object in Python compared with other languages. Below, we will experience the connotation of Python object-oriented programming through some examples of some basic terms of object-oriented programming.
First, give the simplest class:
# The keyword for defining a class in Python is' class'. If no class is defined, # You can enter pass directly. class Dog: pass dog_1 = Dog() # Create first instance of Dog() class ` dog_1` dog_2 = Dog() # Create second instance of Dog() class ` dog_2` # The following dynamically assigns the attribute 'name' to the first instance: d1.name = 'Xiaoliang' # Output the 'name' attribute of the first instance: print(dog_1.name)
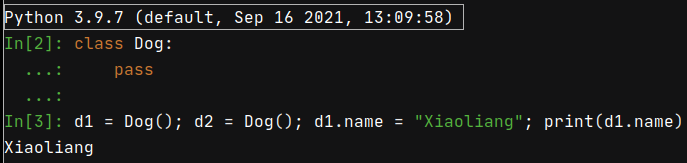

2, Variable
Python classes include variables and variables.
2.1 instance variables
The above dynamic adding variables to the class will be very inconvenient in practical application. If we can directly add Dog class to the class, the attribute name is also called instance attribute.
Python class instance variables are added through__ init__ Function (a construction method). It is assumed that the instance attributes of a dog include: name, height, blood and power. The implementation code is as follows:
class Dog: # The constructor is executed first when instantiating with the 'Dog' class`__ init__` function def __init__(self, name, height, blood, power): # When instantiating, the following instructions can assign the defined attributes to themselves; # `self.name ` is the name of each instance, and ` = ` is followed by ` name '` # Is an instance variable, which assigns different values according to different instance properties. self.name = name self.height = height self.blood = blood self.power = power dog_1 = Dog('Xiaoliang', 0.6, 9, 5) # Create first instance ` dog_1` dog_2 = Dog('Xiaowang', 0.7, 7, 3) # Create first instance ` dog_2` print(dog_1); print(dog_2); print(dog_1.name); print(dog_2.name)
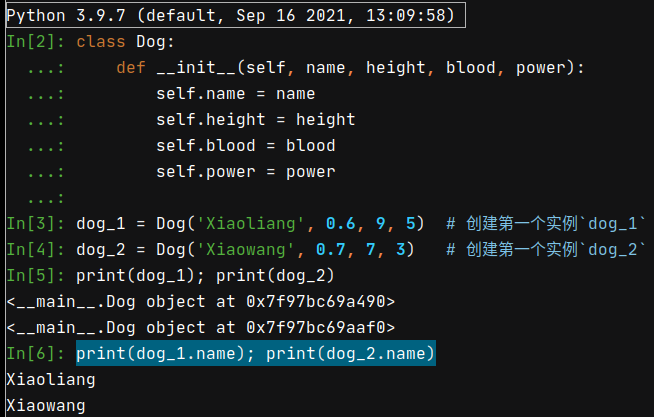
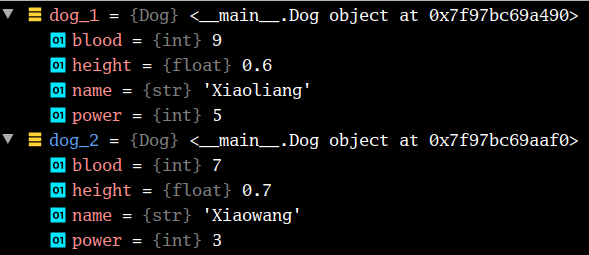
Note: the instance variable of a class is represented by the self keyword. The main function of self is to indicate that this variable is a variable related to the instance attribute.
Class 2.2 variables
1. Methods for adding class variables to classes
Here, we use instance variables to understand class variables and class variables. For example, in the following code, there is an additional class attribute num_of_dogs:
class Dog: num_of_dogs = 0 #Class properties # Construction method: add instance properties and do other initial work def __init__(self, name, height, blood, power): self.name = name self.height = height self.blood = blood self.power = power dog_1 = Dog('Xiaoliang', 0.6, 9, 5) # Create first instance ` dog_1` dog_2 = Dog('Xiaowang', 0.7, 7, 3) # Create first instance ` dog_2` # Methods to access class properties: # (1) Access by class name print(Dog.num_of_dogs) # (2) Access by instance print(dog_1.num_of_dogs)
2. Methods to access class variables
(1) Access dog. By class name num_ of_ dogs;
(2) Accessing dog through instance_ 1.num_ of_ dogs.
3. Methods of modifying class variables
There are also two modification methods:
(1) Modify by class name:
Dog.num_of_dogs = 10 #Modify by class name print(Dog.num_of_dogs) print(dog_1.num_of_dogs, dog_2.num_of_dogs)
Reasons for this result:
This is due to Dog num_of_dogs = 10. After execution, the class attribute of Dog class is num_of_dogs becomes 10. Since the scope of class attributes is global in the class, the instance dog_1 and dog_2. Access the class attribute num_of_dogs will also output 10.
(2) Modify by instance
dog_1.num_of_dogs = 10 print(Dog.num_of_dogs) print(dog_1.num_of_dogs, dog_2.num_of_dogs)
The output result of modifying class attributes through instances is different from that of modifying class names. There is only dog_1.num_of_dogs output 10, while other class attributes are still 0.
Reasons for this result:
This is due to dog_1.num_of_dogs = 10. After execution, a dog is generated_ 1 instance an instance attribute num_of_dogs, so dog_1.num_ of_ When dogs output, the first access is dog_1.num_of_dogs is the instance attribute, not the class attribute dog num_of_dogs.
4. Role of class variables
Class variable num_of_dogs can be used to count how many instances of Dog have been created_ x. Can be in a class__ init__ Function implementation, the code is as follows:
class Dog: num_of_dogs = 0 def __init__(self, name, height, blood, power): ...... num_of_dogs = num_of_dogs + 1
3, Method
3.1 example method
Except up there__ init__ For the attributes of Dog class added in the function, you can also add the method of class through the def function method. Compared with Dog class, it not only has the attributes in the above four, but also assumes that they can speak human words. Next, add the speak() method through the def function:
class Dog: def __init__(self, name, height, blood, power): self.name = name self.height = height self.blood = blood self.power = power def speak(self): # Construct 'speak()' method: # self.xx is the attribute of each instance; So the properties of the output instance, # Self. Is required xx print(f'I am{self.name}, height{self.height}, Blood volume{self.blood}, aggressivity{self.power}') dog_1 = Dog('Xiaoliang', 0.6, 9, 5) # Create first instance ` dog_1` dog_2 = Dog('Xiaowang', 0.7, 7, 3) # Create first instance ` dog_2` # Next, call the class method of each instance: print(dog_1.speak()); print(dog_2.speak())
Class 3.2 method
Suppose we have such an application scenario: we not only need to be able to get the total number of instances instantiated, but also need to be able to output the objects of all instances. The program to solve this problem using class method (the flag is @ classmethod) is as follows:
class Dog: # First, define a class list variable to store class instances dogs = [] # Build a class method to count the total number of instance objects @classmethod #This keyword indicates that the following functions are class methods def num_of_dogs(cls): # cls indicates that the input is a class object return len(cls.dogs) def __init__(self, name) self.name = name # The following instructions are implemented: each time an instance is instantiated, the instance is placed in the # Class variable dogs [] list. Note: ` self 'indicates the current instance object Dog.dogs.append(self) dog_1 = Dog('Xiaoliang'); dog_2 = Dog('Xiaowang') dog_3 = Dog('Xiaoli'); dog_4 = Dog('Xiaozhao') # Call the class method num of the Dog class with the class name_ of_ dogs print(Dog.num_of_dogs) # Of course, we can also use instances to call the class method num_of_dogs print(dog_1.num_of_dogs)
Note: class methods cannot access instance variables, that is, variables related to self. They can only access class variables, so class methods are designed only for classes.
3.3 static method
Instance method is a method designed for instance variables (marked with self), while class method is a method designed for class variables (marked with cls). However, in practice, sometimes we need to design a kind of method to process neither instance variables nor class variables. This is the so-called static method, and its flag is @ staticmethod. For example, we need to add an explanatory language to the class:
class Dog: @staticmethod def description() print("This is a Dog class!") def __init__(self, name): self.name = name
4, Three characteristics of object-oriented
4.1 succession
Although the Dog class method was designed earlier, the instantiated variables are name, height, blood, power and the class method speak(). However, as shown in the figure below, for different types of dogs, they have various attributes of Dog, but they have their own attributes and methods.
![]() |
![]() |
![]() |
Therefore, only using dog class can not well represent all dog objects, so we need to introduce a very important concept of object-oriented programming - class inheritance. Using class inheritance, we can realize the representation of pet dogs, shepherds and police dogs on Dog class methods. The following takes the pet dog as an example to explain the inheritance of the class. The code is as follows:
class Dog: def __init__(self, name, height, power): self.name = name self.height = height self.power = power def speak(self): print(f'I am{self.name}, height{self.height}, aggressivity{self.power}') # Add a bracket after pet Dog PetDog and write the parent Dog internally # This implements the PetDog class that inherits the Dog class: class PetDog(Dog): # For the unique instance attribute price of pet dog, it needs to be defined in # __ init__ () defined in function def __init__(self, name, height, power, price): # super() keyword indicates that the PetDog class inherits the parameters of the parent class! super().__init__(name, height, power) # After executing the above instructions, you only need to assign pet dog PetDog # The unique instance variable price is enough. self.price = price # Now create a pet dog instance pet_dog = PetDog('Xiaoxin', 0.6, 1, 10000) # Pet created based on PetDog class_ Dog instance. Due to the function of super(), # It also inherits the name, height and power instance variables of the parent class. Of course, it also inherits # Has its own unique instance variables. print(pet_dog.price, pet_dog.name, pet_dog.height, pet_dog.power) # pet_dog also inherits the speak() method of the parent class! pet_dog.speak()
4.2 packaging
Encapsulation can only be accessed inside the class, and external access to attributes or methods will report exceptions. Encapsulation in Python is very simple. Just add two underscores in front of attributes or method names, which completes the privatization of attributes and methods, that is, encapsulation. In fact, python language does not have private attributes in a strict sense. Even if private attributes are defined in a class, we can output results through class methods.
The following is a Python class encapsulation example, in which the instance variable price is set as a private attribute, but we can output the private attribute through the get(self) class method; In addition, set a private class function secret_language and set a common class method speak:
class Dog: def __init__(self, name, price): self.name = name # Private instance variable self.__price = price # Define a class method that can be called externally to output private instance variables def get(self): print(self.__price) dog_1 = Dog("Xiaoliang", 1000) print(dog_1.name) # Common variable normal output "Xiaoliang" print(dog_1.__price) # Private instance variables cannot be directly output externally print(dog_1.get()) # Normally output the value of private instance variable 'price'
The verification output results are shown in the figure below:
4.3 polymorphism
Python does not have the idea of true polymorphism in essence, but there is such an idea of polymorphism in form.