In fact, we have used the XxxAware interface before. For example, the Employee class we created earlier implements the ApplicationContextAware interface. The source code of the Employee class is as follows.
package io.mykit.spring.plugins.register.bean; import org.springframework.beans.BeansException; import org.springframework.context.ApplicationContext; import org.springframework.context.ApplicationContextAware; import org.springframework.stereotype.Component; /** * @author binghe * @version 1.0.0 * @description Test ApplicationContextAware */ @Component public class Employee implements ApplicationContextAware { private ApplicationContext applicationContext; @Override public void setApplicationContext(ApplicationContext applicationContext) throws BeansException { this.applicationContext = applicationContext; } }
It can be seen from the source code of the Employee class that if the ApplicationContextAware interface is implemented, the setApplicationContext() method needs to be implemented. When the IOC container starts and creates the employee object, Spring will call the setApplicationContext() method and pass the ApplicationContext object into the setApplicationContext() method. We only need to define a member variable of ApplicationContext type in the Employee class to receive the parameters of the setApplicationContext() method, You can use the ApplicationContext object.
In fact, there are many designs similar to ApplicationContextAware interfaces in Spring. In essence, xxaware interfaces in Spring inherit Aware interfaces. Let's take a look at the source code of Aware interfaces, as shown below.
package org.springframework.beans.factory; /** * @author Chris Beams * @author Juergen Hoeller * @since 3.1 */ public interface Aware { }
You can see that the Aware interface is an interface introduced in spring version 3.1. In the Aware interface, no method is defined.
Next, let's see which interfaces inherit the Aware interface, as shown below.
Xxaware interface case
Next, we will select several commonly used xxaware interfaces for simple description.
The ApplicationContextAware interface is widely used. Let's talk about this interface first. Through the ApplicationContextAware interface, we can get the IOC container.
First, we create a Blue class, implement the ApplicationContextAware interface, and output the ApplicationContext in the implemented setApplicationContext(), as shown below.
package io.mykit.spring.plugins.register.bean; import org.springframework.beans.BeansException; import org.springframework.context.ApplicationContext; import org.springframework.context.ApplicationContextAware; /** * @author binghe * @version 1.0.0 * @description Testing the ApplicationContextAware interface */ public class Blue implements ApplicationContextAware { private ApplicationContext applicationContext @Override public void setApplicationContext(ApplicationContext applicationContext) throws BeansException { System.out.println("Incoming ioc: " + applicationContext); this.applicationContext = applicationContext; } }
We can also implement several xxaware interfaces for the Blue class at the same time. For example, make the Blue class implement another BeanNameAware interface. We can obtain the name of the current bean in the Spring container through the BeanNameAware interface, as shown below.
package io.mykit.spring.plugins.register.bean; import org.springframework.beans.BeansException; import org.springframework.beans.factory.BeanNameAware; import org.springframework.context.ApplicationContext; import org.springframework.context.ApplicationContextAware; /** * @author binghe * @version 1.0.0 * @description Testing the ApplicationContextAware interface */ public class Blue implements ApplicationContextAware, BeanNameAware { private ApplicationContext applicationContext @Override public void setApplicationContext(ApplicationContext applicationContext) throws BeansException { System.out.println("Incoming ioc: " + applicationContext); this.applicationContext = applicationContext; } @Override public void setBeanName(String name) { System.out.println("current bean Name of"); } }
Next, we implement an EmbeddedValueResolverAware interface. We can get the StringValue parser through the EmbeddedValueResolverAware interface. As shown below.
package io.mykit.spring.plugins.register.bean; import org.springframework.beans.BeansException; import org.springframework.beans.factory.BeanNameAware; import org.springframework.context.ApplicationContext; import org.springframework.context.ApplicationContextAware; import org.springframework.context.EmbeddedValueResolverAware; import org.springframework.util.StringValueResolver; /** * @author binghe * @version 1.0.0 * @description Testing the ApplicationContextAware interface */ public class Blue implements ApplicationContextAware, BeanNameAware, EmbeddedValueResolverAware { private ApplicationContext applicationContext; @Override public void setApplicationContext(ApplicationContext applicationContext) throws BeansException { System.out.println("Incoming ioc: " + applicationContext); this.applicationContext = applicationContext; } @Override public void setBeanName(String name) { System.out.println("current bean Name of"); } @Override public void setEmbeddedValueResolver(StringValueResolver resolver) { String resolveStringValue = resolver.resolveStringValue("Hello ${os.name} Age:#{20*18}"); System.out.println("The parsed string is:" + resolveStringValue); } }
Next, we need to annotate the @ Component annotation on the Blue class and add the Blue class to the IOC container, as shown below.
@Component public class Blue implements ApplicationContextAware, BeanNameAware, EmbeddedValueResolverAware { **Java Interview core knowledge notes** Which includes JVM,Lock, concurrency Java Reflection Spring Principles, microservices Zookeeper,A large number of knowledge points such as database and data structure. 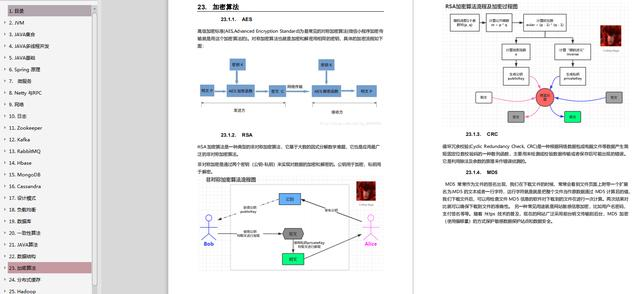 **Java Arrangement of high frequency examination sites for middle and senior interview** 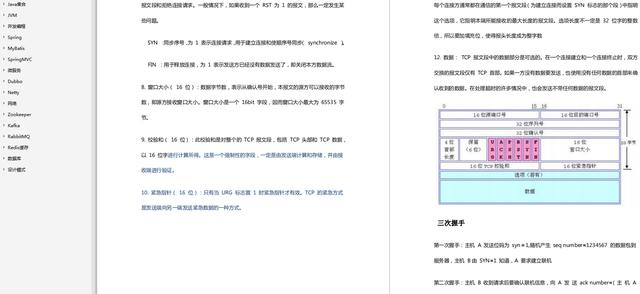 **more Java Advanced knowledge notes and document sharing are good learning materials for both interview and learning** **[Friends in need can poke here and get it for free](https://gitee.com/vip204888/java-p7)** 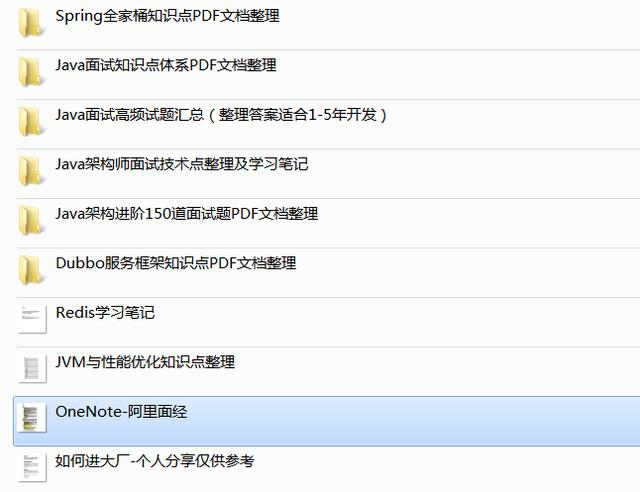 **Finally, share Java Video teaching for advanced learning and interview** 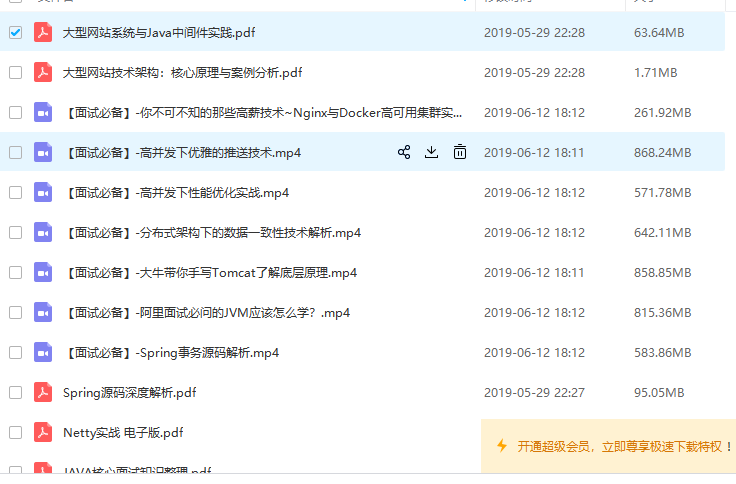 If you want, you can poke here and get it for free](https://gitee.com/vip204888/java-p7)** [External chain picture transfer...(img-CvxtUc1G-1628287950746)] **Finally, share Java Video teaching for advanced learning and interview** [External chain picture transfer...(img-tGN8msB1-1628287950748)]