1, $. get() method
- jQuery.get(url, [data], [callback], [type])
- Load information through a remote HTTP GET request.
- This is a simple GET request function to replace complex $. ajax. The callback function can be called when the request is successful. If you need to execute a function when an error occurs, use $. ajax.
- jQuery.post and jQuery.get in jQuery 1.12 support object parameters, which has many advantages. For example, setting the context of callback function or cross domain post can withCredential: true. Refer to the last example for usage.
- parameter
- (1) URL: the URL address of the page to be loaded
- (2) data: Key/value parameter to be sent.
- (3) Callback: callback function when loading is successful.
- (4) type: return content format, XML, HTML, script, JSON, text_ default.
1. No need to transfer data
//Analog get interface
router.get('/getUser',(req,res)=>{
//Simulate backend data
let data = {
ststus: 'ok',
code: 200,
result: [
{ id: 1, name: "Xiao Zhao", age: 20, sex: 'male' },
{ id: 2, name: "Xiao Yang", age: 18, sex: 'male' },
{ id: 3, name: "petty thief", age: 21, sex: 'female' },
{ id: 4, name: "Xiao Chen", age: 22, sex: 'male' },
{ id: 5, name: "Xiao Ruan", age: 23, sex: 'female' },
{ id: 6, name: "Xiao Hong", age: 17, sex: 'female' }
]
};
res.json(data);
})
//Any interface involves cross domain issues
//get
//Just get data, no need to transfer data
var url="http://127.0.0.1:8080/getUser";
$.get(url,function(res){
console.log(res);
})
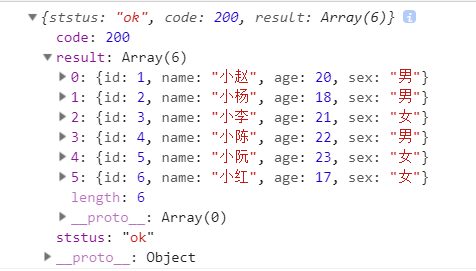
- Cross domain * is to allow access to all domain names
- res.setHeader("Access-Control-Allow-Origin",'*');
2. Need to transfer data
//Simulate the get interface for passing parameters. / / simulate user login and pass the account and password
router.get('/sendparams',(req,res)=>{
let query=req.query;
let id=query.id;
let pwd=query.pwd;
let result={};
if(id=="asd_1234"&&pwd=="abc_1234"){
result={
status:'OK',
code:200,
result:{
effectRows:1
}
}
}
else{
result={
status:'OK',
code:200,
result:{
effectRows:0
}
}
}
res.json(result);
})
//To get data, you need to transfer the api of the data
var src="http://127.0.0.1:8080/sendparams";
var info={id:"asd_1234",pwd:'abc_1234'};
$.get(src,info,function(res){
console.log(res);
});
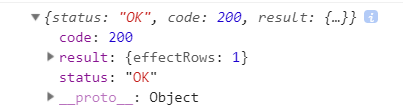
2, $. post() method
- jQuery.post(url, [data], [callback], [type])
- Load information via remote HTTP POST request.
- This is a simple POST request function to replace complex $. ajax. The callback function can be called when the request is successful. If you need to execute a function when an error occurs, use $. ajax.
- jQuery.post and jQuery.get in jQuery 1.12 support object parameters, which has many advantages. For example, setting the context of callback function or cross domain post can withCredential: true. Refer to Example 9 for usage.
- parameter
- (1) url: send request address.
- (2) data: Key/value parameter to be sent.
- (3) Callback: callback function when sending successfully.
- (4) type: return content format, XML, HTML, script, JSON, text_ default.
- Rewrite the back-end code and write the get() method and post() method together.
- back-end
//Simulate backend data
let data = {
ststus: 'ok',
code: 200,
result: [
{ id: 1, name: "Zhang San", age: 20, sex: 'male' },
{ id: 2, name: "Li Si", age: 18, sex: 'male' },
{ id: 3, name: "Wang Wu", age: 21, sex: 'female' },
{ id: 4, name: "quick", age: 22, sex: 'male' },
{ id: 5, name: "floret", age: 23, sex: 'female' },
{ id: 6, name: "Xiao Hong", age: 17, sex: 'female' }
]
};
//Analog get interface
router.route('/getUser').get((req, res) => {
res.json(data);
}).post((req, res) => {
res.json(data);
});
//Simulate the get interface for passing parameters
//Simulate user login and pass account and password
router.route('/sendparams').get((req, res) => {
//Get get value
let query = req.query;
let id = query.id;
let pwd = query.pwd;
let result = {};
if (id == "asd_1234" && pwd == "abc_1234") {
result = {
status: 'OK',
code: 200,
result: {
effectRows: 1
}
}
}
else {
result = {
status: 'OK',
code: 200,
result: {
effectRows: 0
}
}
}
res.json(result); }).post((req, res) => {
//post value transfer
let body = req.body;
let id = body.id;
let pwd = body.pwd;
let result = {};
if (id == "asd_1234" && pwd == "abc_1234") {
result = {
status: 'OK',
code: 200,
result: {
effectRows: 1
}
}
}
else {
result = {
status: 'OK',
code: 200,
result: {
effectRows: 0
}
}
}
res.json(result);
});
$.post(url,function(res){
console.log(res);
});
$.post(src,info,function(res){
console.log(res);
});
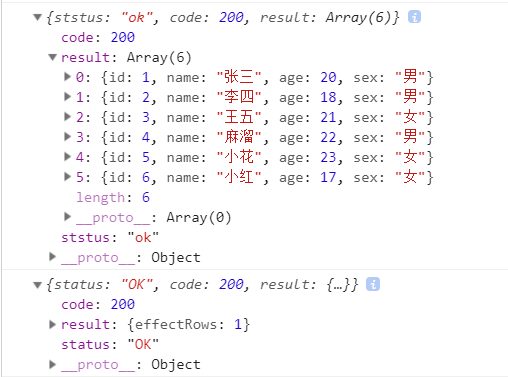
3, $. ajax() method
- jQuery.ajax(url,[settings])
- Load remote data via HTTP request.
- jQuery underlying AJAX implementation. $ ajax() returns the XMLHttpRequest object it created. In most cases, you don't need to operate the function directly, unless you need to operate infrequent options for more flexibility.
- In the simplest case, $. ajax() can be used directly without any parameters.
- Note that all options can be set globally through the $. ajaxSetup() function.
- Callback function
- If you want to process the data from $. ajax(), you need to use the callback function. beforeSend,error,dataFilter,success,complete.
- parameter
- (1) URL: a URL string used to contain the sending request.
- (2) settings:AJAX request settings. All options are optional.
- settings: Options
- (1)jsonp
- Rewrite the name of the callback function in a jsonp request. This value is used to replace the "callback" part of the URL parameter in GET or POST requests such as "callback =?". For example, {jsonp: 'onJsonPLoad'} will cause "onJsonPLoad =?" to be passed to the server.
- (2)jsonpCallback
- Specify a callback function name for the jsonp request. This value will be used to replace the random function name automatically generated by jQuery. This is mainly used to make jQuery generate unique function names, which makes it easier to manage requests and provide callback functions and error handling. You can also specify the callback function name when you want the browser to cache the GET request.
//Data type has a value jsonp similar to dataType
$.ajax({
method:'post',
url:src,
data:info,
contentType:'application/x-www-form-urlencoded',
success:function(res){
console.log(res);
},
error:function(err){
},
async:true
});
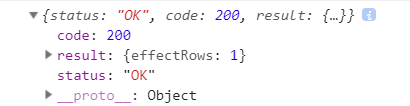
//Simulate jsonp cross domain
router.get("/getUserJsonp", (req, res) =>{
let query = req.query;
let cb = query.cb;
res.send(`${cb}('a')`);
});
//The dataType value sets jsonp, which involves cross domain jsonp
//jsonp cross domain can only be get requests
$.ajax({
method:'get',
url:'http://127.0.0.1:8080/getUserJsonp',
dataType:'jsonp',//The form is jsonp cross domain request
jsonp:'cb',
jsonpCallback:'getData',//Set the callback function of jsonp
success:function(res){
console.log(res);//a
}
});
- Set the allowed request header to allow that property to appear
- res.setHeader("Access-Control-Allow-Headers","Content-Type,userid,userpwd");
- front end
$.ajax({
method:'get',
url:'http://127.0.0.1:8080/getUser',
//Set the headers request header and send it to the server with some relevant parameters
headers:{
userid:'abc123',
userpwd:'abc'
},
success:function(res){
console.log(res);
}
})
let data = {
ststus: 'ok',
code: 200,
result: [
{ id: 1, name: "Zhang San", age: 20, sex: 'male' },
{ id: 2, name: "Li Si", age: 18, sex: 'male' },
{ id: 3, name: "Wang Wu", age: 21, sex: 'female' },
{ id: 4, name: "quick", age: 22, sex: 'male' },
{ id: 5, name: "floret", age: 23, sex: 'female' },
{ id: 6, name: "Xiao Hong", age: 17, sex: 'female' }
]
};
//Analog get interface
router.route('/getUser').get((req, res, next) => {
//testing
let headers = req.headers;
if (headers.userid == "abc123" && headers.userpwd == "abc") {
next();
}
else {
res.json({
status: 'error',
code: 402
});
}
}, (req, res) => {
res.json(data);
}).post((req, res) => {
res.json(data);
});
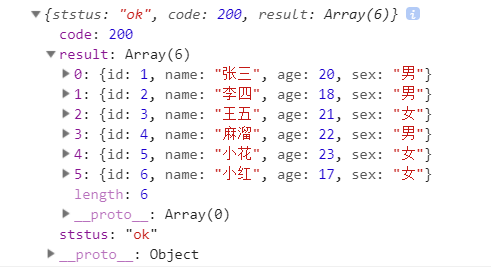
4, $. getJSON()
- jQuery.getJSON(url, [data], [callback])
- Load JSON data through HTTP GET request.
- In jQuery 1.2, you can load JSON data of other domains by using a callback function in the form of JSON, such as "myurl?callback =?". JQuery will automatically replace? Give the correct function name to execute the callback function. Note: the code after this line will be executed before the callback function is executed.
- parameter
- (1) url: send request address.
- (2) data: Key/value parameter to be sent.
- (3) Callback: callback function when loading is successful.
- front end
//getJSON, return json
$.getJSON('http://127.0.0.1:8080/stu',function(res){
console.log(res);
})
//get interface of getJSON
router.get("/stu", (req, res) => {
let stu = { id: 1, name: "Xiao Zhao" }
res.send(JSON.stringify(stu));
});
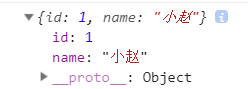
5, $. getScript()
- jQuery.getScript(url, [callback])
- Load and execute a JavaScript file through an HTTP GET request.
- Before jQuery version 1.2, getScript can only call JS files in the same domain. In 1.2, you can call JavaScript files across domains.
- parameter
- (1) url: address of JS file to be loaded.
- (2) Callback: callback function after successful loading.
$.getScript("./js/test.js", function(){
alert("Script loaded and executed.");
});
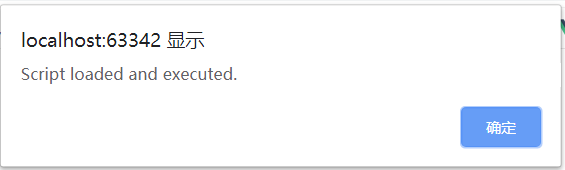