A friend suddenly asked me a question about the probability of winning in a row. I'll study it myself, share it with you and record it at the same time.
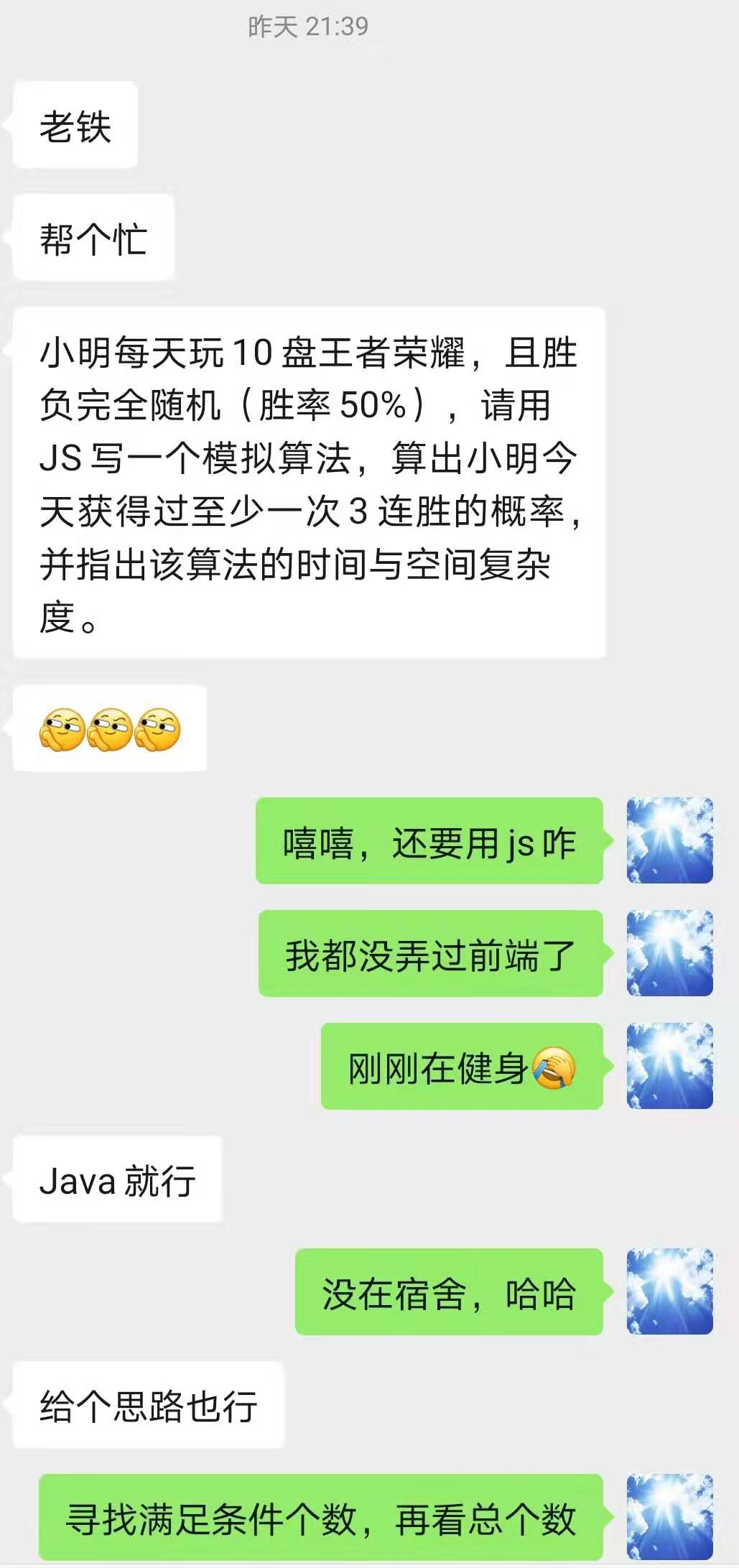
Problem description
Xiao Ming plays 10 sets of King glory every day, and the winning and losing are completely random (the winning rate is 50%). Please write a simulation algorithm with JS to calculate the probability that Xiao Ming has won at least one three game winning streak today, and point out the time and space complexity of the algorithm.
Problem solving ideas
Enumerating rules
Seeing that there are too many 10 sets, let's take a look at 3 sets, 4 sets, 5 sets and so on to see what the rules are. As we learn about computers, in fact, all the data of computers are composed of 0 and 1. Here, individuals use 1 to represent victory, 0 to represent defeat, and the winning rate is 50%
Therefore, the probability of three consecutive wins in three sets is as follows
111 == 1/2^3=0.125
The probability of winning three sets in a row is as follows
1110 == 1/2^4
0111 == 1/2^4
1111 == 1/2^4
sum = 1/2^34+ 1/2^4 + 1/2^4 = 3/2^3=0.1875
Situation and probability of winning three sets in a row
1110* == 1/2^4
01110 == 1/2^5
*0111 == 1/2^4
11110 == 1/2^5
01111 == 1/2^5
11111 == 1/2^5
sum = 1/2^4 + 1/2^5 + 1/2^4 + 1/2^5 + 1/2^5 + 1/2^5= 1/2^4 * 2+ 1/2^5 * 4 =0.25
Enumeration deduction
We know that a winning streak is 1, that is, how many two wins, that is, how many one moves in parallel. However, to prevent exceeding its own winning streak, the next winning streak is 0 That is, the total number of wins in 10 sets is
Ten unknowns**********
Three winning streak reasoning, 01110 five numbers, shifted from the left, the total number of moves (10-5) + 1 will have four kinds, plus the boundary problem, there are two kinds 11100111, that is, the three winning streak probability 1 / 2 ^ 5 * (10-5 + 1) + 2 / 2 ^ 4
Four consecutive victories analogy 1 / 2 ^ 6 * (10-6 + 1) + 2 / 2 ^ 5
Therefore, by analogy, set the above as N sets, and the probability of success in a row
∑
i
=
s
u
c
c
e
s
s
N
\sum_{i=success}^N
∑i=successN (1/2^(i+2) * (N-(i+2)+1)+2 * 1/(i+1)) + 1/2^N
It is realized by computer, as shown in the figure below
/** * @param success Winning streak * @param count Total number, count > m + 2 * @return */ public static double rateResult(int success, int count) { if (count < success) { return 0; } double sum = 0; for (int i = success; i < count; i++) { sum += Math.pow(1.0 / 2, i + 2) * (count - i - 1) + Math.pow(1.0 / 2, i + 1) * 2; // i-2 winning streak probability } sum += Math.pow(1.0 / 2, count); // n winning streak return sum; }
Verification reasoning
From the above enumeration, we deduce from two groups of data. Let's verify it
public static void main(String[] args) { System.out.println(rateResult(3, 3)); // 0.125 System.out.println(rateResult(3, 4)); // 0.1875 System.out.println(rateResult(3, 5)); // 0.25 }
In other words, if the conditions are met, whether there is a mistake remains to be studied
Algorithm optimization
N sets, the winning streak is success, the winning probability is rate, and the algorithm is optimized.
/** * @param success Winning streak * @param count Number of General Administration * @param rate Winning streak probability * @return */ public static double rateResult(int success, int count, double rate) { if (count < success) { return 0; } double sum = 0; for (int i = success; i < count; i++) { sum += Math.pow((1 - rate), 2) * Math.pow(rate, i) * (count - i - 1) + (1 - rate) * Math.pow(rate, i) * 2; // i-2 winning streak probability } sum += Math.pow(1.0 / 2, count); // n winning streak return sum; }
practical application
The question is, how can we play the game and play the king?
1. For example, if the game is played in a balanced way, there will be more than three consecutive games. How many games will it take to play.
int N = 3; while (true) { if (rateResult(3, N++, 0.5) > 1) { System.out.println(--N); break; } }
Output 18, that is, when we play 18 games, there will be three consecutive wins.
2. We further calculate the evolution. If we win 10 consecutive games, how many games will we play the king game?
int N = 3; while (true) { if (rateResult(10, N++, 0.5) > 1) { System.out.println(--N); break; } }
The result is 2057. Isn't it surprising that those who win more than 15 games in a row must play a lot of games.
3. Continue to practice, but the game design rules, in fact, the basic games are scored according to 50% of the players' ordinary summary ability. Of course, there are many human factors. In fact, there are also hand feel. Different mobile phones or different times give people different playing states. At this time, we can dynamically generate the winning rate factor rate. In addition, the subconscious design rules of the game can not make players unable to get on the stage all the time. Therefore, there is the problem of reward points, that is, if you lose, you will also be rewarded with points. As long as you play a certain number of games, It will be possible to become a king. Unless your wisdom is below this level, you can basically become a king. As for how to count this algorithm, it is more complex. Because your victory rate in the last stage will be lower. To win points again, you need to constantly learn and improve yourself. This is the subconscious rule of the game.