Spring Security login is mainly composed of a series of filters. If we need to modify the login verification logic, we only need to add and modify the relevant logic on the filter link. Here, we mainly understand the relevant authentication login logic through the source code of Spring Security.
1. Authentication process of spring security
Main analysis:
- Process for authenticating users
- How to perform authentication verification
- How to obtain user information after successful authentication
The specific filter links are as follows:
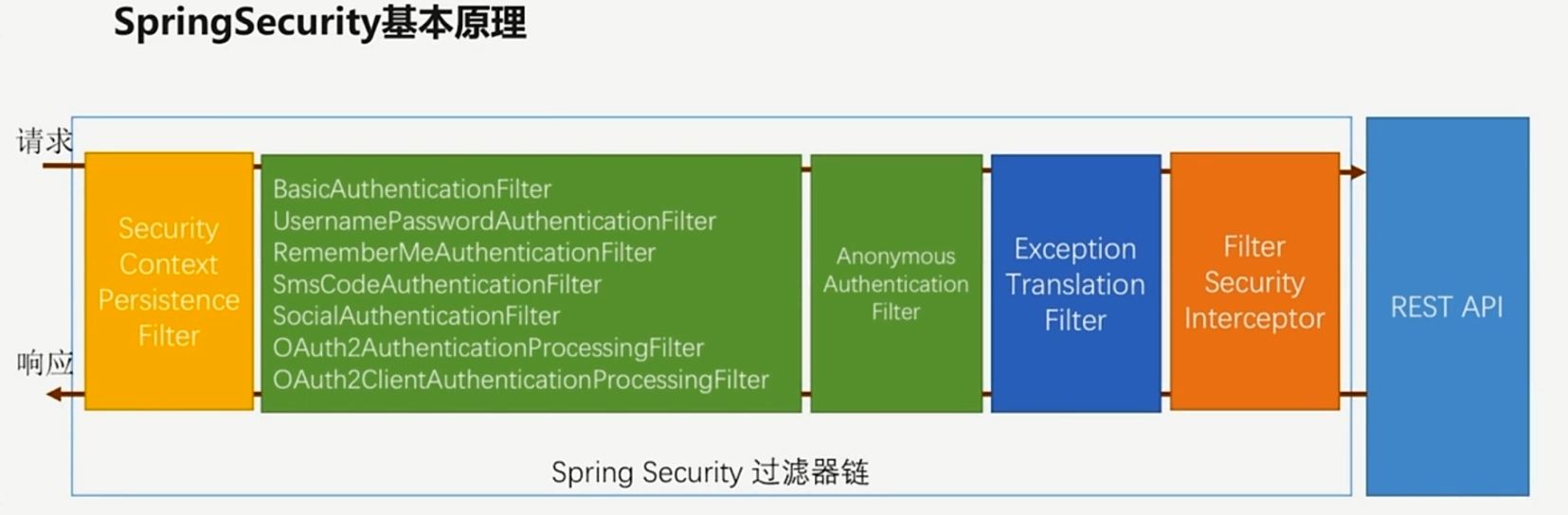
The authentication flow chart of Spring Security is as follows. The main authentication processes include:
- The user submits the user name and password, and then encapsulates them through the UsernamePasswordAuthenticationFilter to become the UsernamePasswordAuthenticationToken object. This is a subclass of AbstractAuthenticationToken, and AbstractAuthenticationToken is an implementation of Authentication, so you can see that all subsequent object instances of Authentication type are obtained;
- Pass the UsernamePasswordAuthenticationToken object in the first step to the AuthenticationManager;
- Through the retrieveUser method of DaoAuthenticationProvider, the default implementation class of AbstractUserDetailsAuthenticationProvider, this method will call the loadUserByUsername method of UserDetailsService to judge the user name and password, and use the default logic for processing;
- Put the user information after successful authentication into the SecurityContextHolder, and then you can obtain the user's relevant information through the SecurityContext.
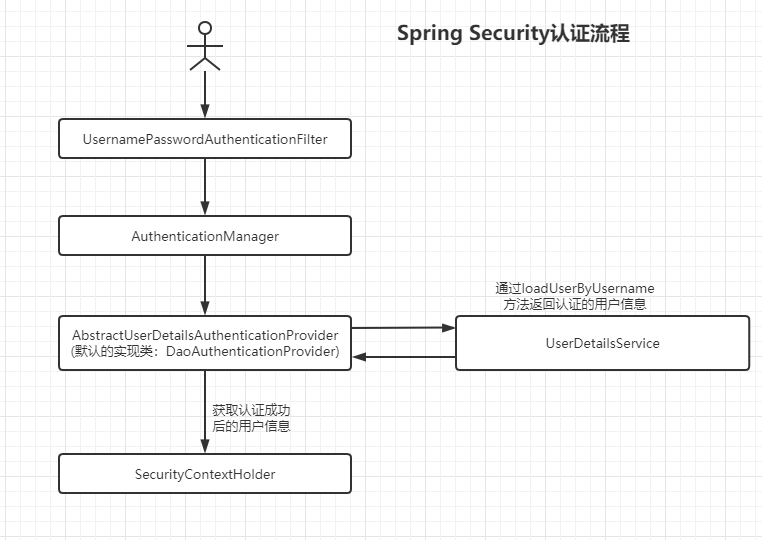
Spring security source code download address:
https://github.com/spring-projects/spring-security
2. Analysis of authentication source code of spring security
2.1 set up the project and visit
First, we set up a Spring Security project, which can be used for integrated development easily. We can mainly introduce the following dependencies (of course, you can also check the official website and select the appropriate version):
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
After starting the project, a password string will be generated randomly. Here, you need to copy and save it for login:

Access login address:
http://localhost:8080/login
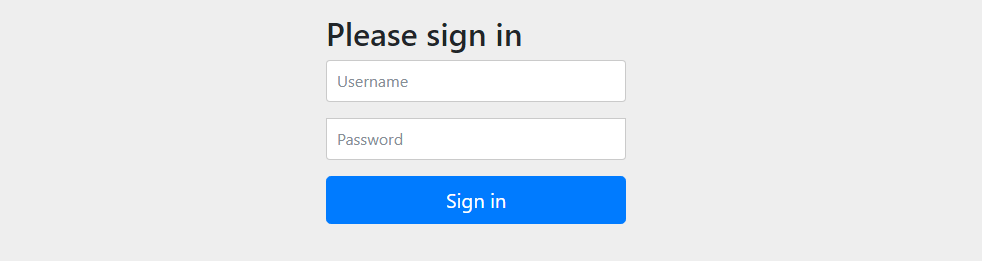
Default account name and password:
Account name: user password: Password string generated when the project starts
2.2 source code analysis
- After breaking the point, it will be found that UsernamePasswordAuthenticationFilter attemptAuthentication(HttpServletRequest request, HttpServletResponse response) method will first be encapsulated as a UsernamePasswordAuthenticationToken object by user name and password, and then this. will be called. getAuthenticationManager(). The authenticate (authrequest) method enters the AuthenticationManager. The source code of the attemptAuthentication method is as follows:
@Override public Authentication attemptAuthentication(HttpServletRequest request, HttpServletResponse response) throws AuthenticationException { if (this.postOnly && !request.getMethod().equals("POST")) { throw new AuthenticationServiceException("Authentication method not supported: " + request.getMethod()); } String username = obtainUsername(request); username = (username != null) ? username : ""; username = username.trim(); String password = obtainPassword(request); password = (password != null) ? password : ""; UsernamePasswordAuthenticationToken authRequest = new UsernamePasswordAuthenticationToken(username, password); // Allow subclasses to set the "details" property setDetails(request, authRequest); return this.getAuthenticationManager().authenticate(authRequest); }