- Android design pattern Github project address: Click jump
0. What is the builder model?
1. Separate a complex object and use multiple simple objects to build a complex object step by step.
1. Advantages and disadvantages
advantage:
1. The complex creation process is encapsulated inside. For external callers, they only need to pass in the builder and construction tools. For how the interior is built into a finished product, the caller does not need to care about or know. It has good encapsulation, so that the client does not need to know the details of the internal composition of the product.
2. The builder is independent and easy to expand. Decoupling.
Disadvantages:
1. Products must have common ground and limited scope. 2. If the internal changes are complex, there will be many construction classes.
Usage scenario:
1. The object to be generated has a complex internal structure.
2. The internal properties of the object to be generated depend on each other.
2. Which libraries or methods are implemented using the builder pattern?
AlterDialog,Notification,Gson,Okhttp
3. Example - customers order at McDonald's
1. The food products in McDonald's can be regarded as simple objects
2. McDonald's combines several food products (simple objects) into a set meal, and provides customers with additional food products and ways to pay.
3. This set of processes forms a ordering mechanism, which can be regarded as a complex object.
4. Customers don't have to know how simple objects are made, they just need to order according to the process.
1. Food types (complex objects) include the following simple objects - > packaging types
2. Food commodity (complex object) includes the following simple objects - > food type (encapsulated, so it can be regarded as a simple object), price and name
3. The complete ordering mechanism (complex objects) is as follows: simple objects - > food commodities (encapsulated, so they can be regarded as simple objects). Pay the bill
Food type: hamburger, beverage
Food packaging type: paper and plastic bottles
The attributes of food commodities: food type, food packaging type, name and price
Food commodities: [spicy chicken burger] [Big Mac hamburger] [Cola] [beverage]
Basic package: Spicy drumstick burger package (spicy drumstick burger + cola), Big Mac burger package (Big Mac burger + Sprite)
Define and implement food packaging types
- 1-1.FoodPackingStyle
/** * Abstract class of food packaging */ public interface FoodPackingStyle { String packStyle(); }
- 1-2.BottlePacking
/** * Specific implementation of food packaging - plastic bottle packaging */ public class BottlePacking implements FoodPackingStyle { @Override public String packStyle() { return "Plastic bottle packaging"; } }
- 1-3.WrapperPacking
/** * Specific implementation of food packaging - paper packaging */ public class WrapperPacking implements FoodPackingStyle { @Override public String packStyle() { return "Paper packaging"; } }
Define and implement food types
- 2-1.FoodItem
/** * Abstract class of food commodity * As a commodity, a food has defined attributes such as [name, packaging type, price] */ public interface FoodItem { //Food name String foodName(); //Food packaging type - there are [paper packaging, plastic box packaging] FoodPackingStyle foodPackingStyle(); //Food price float foodPrice(); }
- 2-2.FoodBurger
/** * Food - Hamburger - abstract class (there can be many kinds of hamburgers) */ public abstract class FoodBurger implements FoodItem { //Return the implementation class of specific food packaging type. Hamburgers are all paper packaging @Override public FoodPackingStyle foodPackingStyle() { return new WrapperPacking(); } }
- 2-3.FoodDrink
/** * Food beverage abstract class (there can be many kinds of drinks) */ public abstract class FoodDrink implements FoodItem{ //Return the implementation class of specific food packaging type. All drinks are packaged in plastic bottles @Override public FoodPackingStyle foodPackingStyle() { return new BottlePacking(); } }
Define and implement food commodities
- 3-1.BigMacBurger
/** * Big Mac leg fort - food commodity - specific implementation class of Hamburg */ public class BigMacBurger extends FoodBurger { @Override public String foodName() { return "Big Mac burger"; } @Override public float foodPrice() { return 27; } }
- 3-2.HotSpicyChickenBurger
/** * Spicy chicken drumstick Burger - food commodity - specific implementation class of hamburger */ public class HotSpicyChickenBurger extends FoodBurger { @Override public String foodName() { return "Zinger Burger"; } @Override public float foodPrice() { return 30; } }
- 3-3.CocaColaDrink
/** * Coca Cola - food commodity - Beverage - specific implementation class */ public class CocaColaDrink extends FoodDrink { @Override public String foodName() { return "Coca Cola"; } @Override public float foodPrice() { return 10; } }
- 3-4.SpriteDrink
/** * Sprite - food commodity - Beverage - specific implementation class */ public class SpriteDrink extends FoodDrink { @Override public String foodName() { return "Sprite"; } @Override public float foodPrice() { return 10; } }
Builder class, which can be used by customers to create menu calls
- 4-1.MealBuilder
/** * Create package Builder class */ public class MealBuilder { private List<FoodItem> itemsList = new ArrayList<FoodItem>(); private String name; //You must bring the customer's name when ordering public MealBuilder(String name){ this.name = name; } //The spicy chicken drumstick burger set meal must contain one spicy chicken drumstick burger and one coke public MealBuilder hotSpicyChickenBurgerMealComb(){ itemsList.add(new HotSpicyChickenBurger()); itemsList.add(new CocaColaDrink()); return this; } //The Big Mac package must contain a big Mac hamburger and a sprite public MealBuilder bigMacBurgerMealComb(){ itemsList.add(new BigMacBurger()); itemsList.add(new SpriteDrink()); return this; } //Provide methods for adding food products to the outside world. If guests feel that it is not enough, they can add other food freely public MealBuilder addFoodProduct(FoodItem foodItem){ itemsList.add(foodItem); return this; } //Calculate price private float getCost(){ float cost = 0.0f; for (FoodItem item : itemsList) { cost += item.foodPrice(); } return cost; } //Pay the bill and show all the product information in the package public void doCheck(){ System.out.println("Builder pattern :>> "+name+" Your package includes the following foods>> "); for (FoodItem item : itemsList) { System.out.println("Builder pattern :>> name:"+item.foodName()+" packing:"+item.foodPackingStyle().packStyle()+" Unit Price:"+item.foodPrice()); } System.out.println("Builder pattern :>> The total price you need to pay is "+getCost()+" RMB. "); System.out.println("Builder pattern :>> end."); } }
- 4-2.BuildPatternActivity
public class BuildPatternActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_build_pattern); /** * Xiao Ming starts ordering - > chooses a [spicy chicken drumstick burger package] - [pay the bill */ MealBuilder mealBuilderA = new MealBuilder("Xiao Ming").hotSpicyChickenBurgerMealComb(); mealBuilderA.doCheck(); /** * Wang Laoer started ordering - > chose a [Big Mac hamburger set meal] - > felt it was not enough to eat, and ordered a single [spicy chicken drumstick Castle] - > to pay the bill */ MealBuilder mealBuilderB = new MealBuilder("Wang Laoer").bigMacBurgerMealComb().addFoodProduct(new HotSpicyChickenBurger()); mealBuilderB.doCheck(); } }
The result after running is:
4. Difference from factory mode
Generally speaking, the builder mode is handed over to the client, which can freely combine simple objects step by step, and finally build a complex object and generate instances of complex objects.
The factory pattern can also be understood as a combination of simple objects, and finally create a complex object. The difference is that the factory defines the whole process of combining to build a complex object internally, and finally allows the client to directly create and produce an instance of a complex object.
5. Android technology life exchange
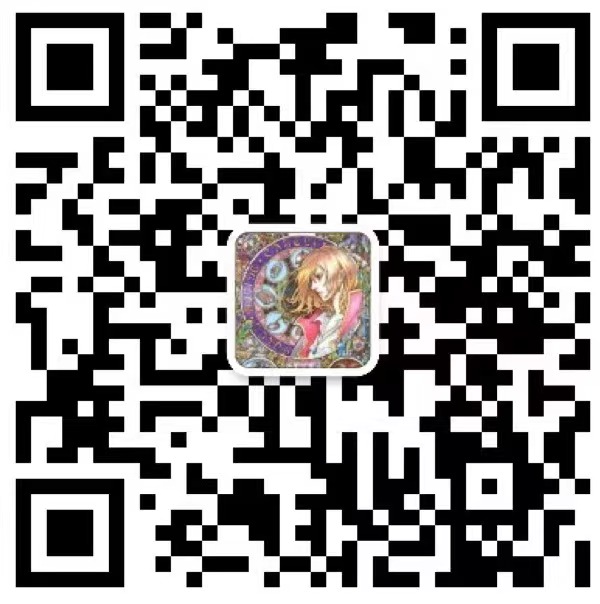
[1]* Reference articles
[2]* Reference articles
[3]* Reference articles
[4]* Reference articles
[5]* Reference articles