outline
Introduction to AOP
II. Actual Operations of Spring
3. Common labels of AOP
IV. Project Source Code and Reference Download
5. Reference Articles
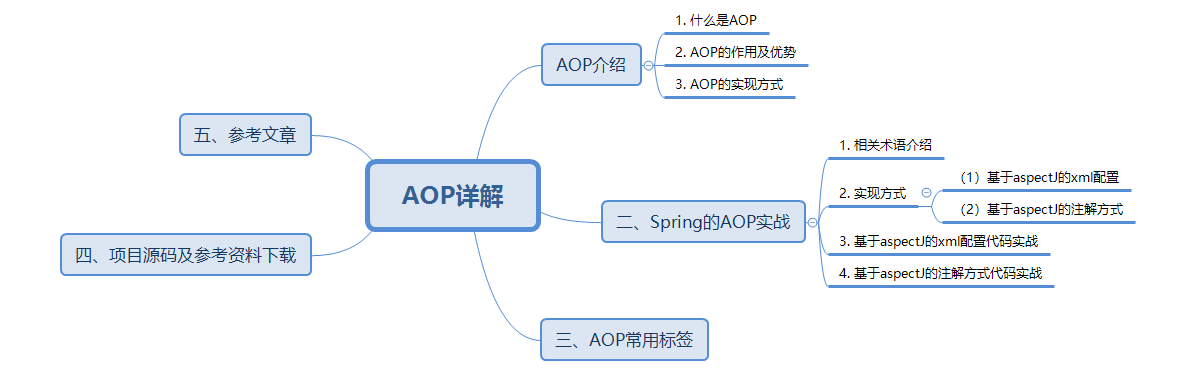
Introduction to AOP
1. What is AOP
In the software industry, AOP stands for Aspect Oriented Programming, which means: AoP Through precompile A technique of unified maintenance of program functions by means of mode and run-time dynamic agent. AOP is OOP The continuation of software development is a hotspot as well as a hotspot. Spring An important part of the framework is Functional Programming A derivative paradigm. Using AOP, the parts of business logic can be isolated, so that the parts of business logic can be separated from each other. Coupling degree It reduces the reusability of programs and improves the efficiency of development.
_Simply speaking, it is to extract the duplicate code of our program, and when it needs to be executed, we use dynamic proxy technology to enhance our existing methods without modifying the source code.
2. The Role and Advantage of AOP
Function: Enhance the existing methods without modifying the source code during the running of the program.
Advantage: Reduce duplicate code, improve development efficiency and maintain convenience
3. Implementation of AOP
_Using Dynamic Agent Technology
II. Actual Operations of Spring
1. Introduction of related terms
(1) Joinpoint: The so-called join points refer to those intercepted points. In spring, these points refer to methods, because spring only supports join points of method type.
(2) PointCut: The point of entry is the definition of which Joinpoint s we want to intercept.
(3) Advice (Notification/Enhancement): Notification refers to what is to be done after intercepting Joinpoint. Notification is divided into relocation notification, post-notification, exception notification, final notification, surround notification (all functions to be completed)
_Pre-notification: Execution before method
Post-notification: Execution after method
_exception notification: method exception
_Final Notification: Execution after Postponement
_Circumferential Notification: Executed before and after the method
(4) Aspect: It is a combination of entry point and notification (introduction). The process of applying enhancement to specific methods is called aspect. That's the process of applying enhancements to entry points
2. Realization
(1) xml configuration based on aspectJ
(2) Annotation based on aspectJ
3. Actual implementation of xml configuration code based on aspectJ
Create the test class Book.java
package aop;
public class Book {
public void add() {
System.out.println("add.......");
}
}
Create the Enhanced and Notification Class MyBook.java
package aop;
import org.aspectj.lang.ProceedingJoinPoint;
public class MyBook {
public void before1() {
System.out.println("Preamplifier...");
}
public void after1() {
System.out.println("Post enhancement...");
}
//Around Advice
public void around1(ProceedingJoinPoint proceedingJoinPoint) {
//Before method
System.out.println("Before method...");
//Execute enhanced methods
try {
proceedingJoinPoint.proceed();
} catch (Throwable e) {
e.printStackTrace();
}
//After method
System.out.println("After method...");
}
}
Configuration file bean.xml under src
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> <!-- bean definitions here -->
<!-- Configuring objects of two classes -->
<bean id="book" class="aop.Book"></bean>
<bean id="myBook" class="aop.MyBook"></bean>
<!-- To configure aop operation -->
<aop:config>
<!-- Configuration entry point expression It's about writing expressions. id That's the entry point name. -->
<aop:pointcut expression="execution(* aop.Book.*(..))" id="pointcut1"/>
<!-- Configure sections to apply enhancements to methods ref Is an object that enhances classes -->
<aop:aspect ref="myBook">
<!-- Configuration Pre-Enhancement method Represents which method is used as the front-end in the enhancement class pointcut-ref Represents which entry point to configure the enhancement method-->
<aop:before method="before1" pointcut-ref="pointcut1"/>
<!-- Configuration Post Enhancement -->
<aop:after method="after1" pointcut-ref="pointcut1"/>
<!-- Configuration Ring Enhancement -->
<aop:around method="around1" pointcut-ref="pointcut1"/>
</aop:aspect>
</aop:config>
</beans>
The test code is as follows
package aop;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class TestAop {
@Test
public void testUser() {
//Load the spring configuration file to create objects based on content
ApplicationContext context = new ClassPathXmlApplicationContext("bean.xml");
Book book = (Book) context.getBean("book");
book.add();
}
}
The results are as follows.
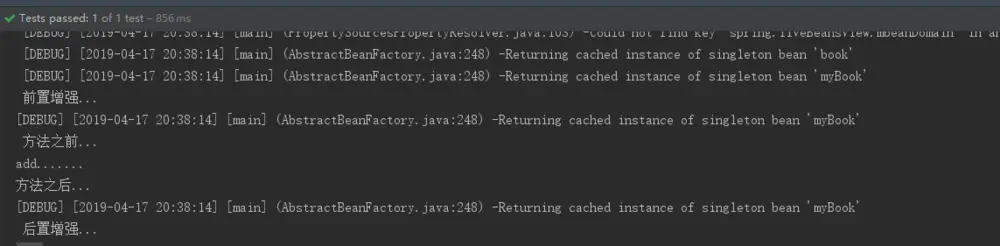
4. Code Practice of Annotation Mode Based on aspectJ
Create the test class Book.java
_code is the same as in xml configuration
Create the Enhanced and Notification Class MyBook.java
package aop2;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
//Spring can find the section annotated with @Aspect and apply it to the target object.
@Aspect
public class MyBook {
//Enhanced configuration using annotations on Methods
@Before(value="execution(* aop2.Book.*(..))")
public void before1() {
System.out.println("Preamplifier...");
}
@After(value="execution(* aop2.Book.*(..))")
public void after1() {
System.out.println("Post enhancement...");
}
@Around(value="execution(* aop2.Book.*(..))")
//Around Advice
public void around1(ProceedingJoinPoint proceedingJoinPoint) {
//Before method
System.out.println("Before method...");
//Execute enhanced methods
try {
proceedingJoinPoint.proceed();
} catch (Throwable e) {
e.printStackTrace();
}
//After method
System.out.println("After method...");
}
}
Configuration file bean2.xml under src
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> <!-- bean definitions here -->
<!-- open aop operation -->
<aop:aspectj-autoproxy></aop:aspectj-autoproxy>
<!-- create object -->
<bean id="book" class="aop2.Book"></bean>
<bean id="myBook" class="aop2.MyBook"></bean>
</beans>
The test code is as follows
package aop2;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class TestAop {
@Test
public void testUser() {
//Load the spring configuration file to create objects based on content
ApplicationContext context = new ClassPathXmlApplicationContext("bean2.xml");
Book book = (Book) context.getBean("book");
book.add();
}
}
The results are as follows.
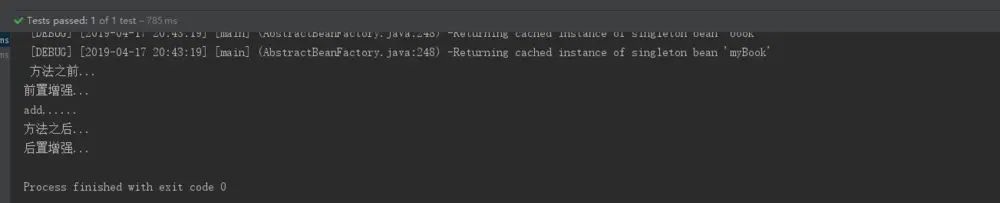
3. Common labels of AOP
(1)<aop:config>
_is used to declare the configuration of starting aop
(2)<aop:aspect>
Function: Used to configure sections.
Attributes:
_id: Provide a unique identification for the cut surface.
ref: Refers to the id of the configured notification class bean.
(3)<aop:pointcut>
_Role: Used to configure pointcut expressions
Attributes:
_expression: Used to define pointcut expressions.
id: Used to provide a unique identifier for pointcut expressions.
(4)<aop:before>
Role: Used to configure pre-notifications
Attributes:
_method: Specify the name of the method in the notification.
_pointct: Defining pointcut expressions
_pointcut-ref: Reference to specified pointcut expressions
(5)<aop:after-returning>
Function: Used to configure post-notification
Attributes:
_method: Specify the name of the method in the notification.
_pointct: Defining pointcut expressions
_pointcut-ref: Reference to specified pointcut expressions
(6)<aop:after-throwing>
Role: Used to configure exception notifications
Attributes:
_method: Specify the name of the method in the notification.
_pointct: Defining pointcut expressions
_pointcut-ref: Reference to specified pointcut expressions
(7)<aop:after>
Role: Used to configure final notifications
Attributes:
_method: Specify the name of the method in the notification.
_pointct: Defining pointcut expressions
_pointcut-ref: Reference to specified pointcut expressions
(8)<aop:around>
Role: Used to configure surround notifications
Attributes:
_method: Specify the name of the method in the notification.
_pointct: Defining pointcut expressions
_pointcut-ref: Reference to specified entry point expression
IV. Project Source Code and Reference Download
Links: https://pan.baidu.com/s/1mU6ktTrwggh9SVmsoucJRg
Extraction code: jep8